Understand CORS and how to fix a CORS error

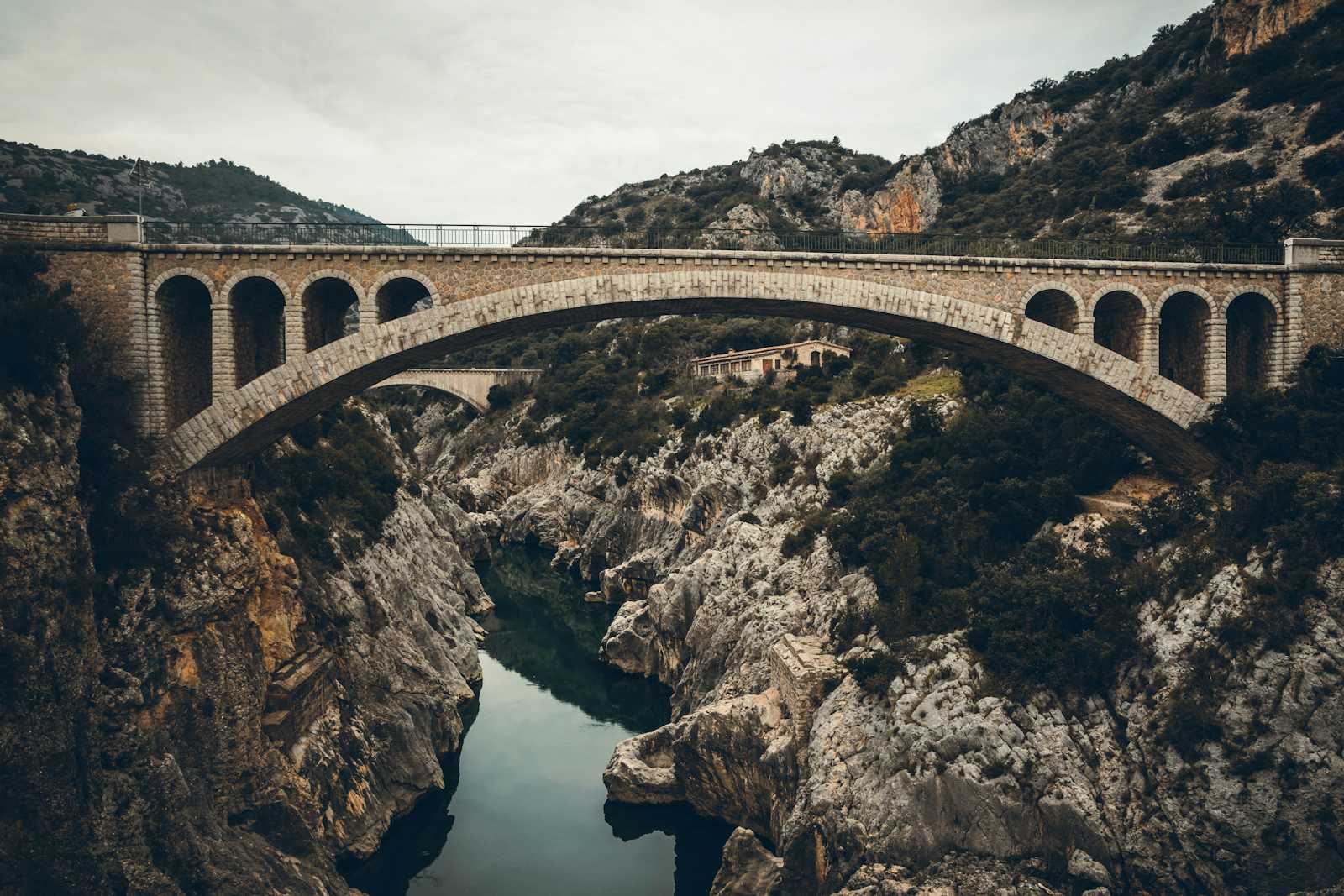
What is CORS?
CORS stands for Cross-Origin-Resource-Sharing.
Let us say your backend is an API-only app and it is deployed on Heroku. Your frontend is deployed on Netlify. So your frontend will be communicating with your backend which is on a different origin. This would be termed as Cross-Origin-Resource-Sharing.
Similarly, let us say that your full-stack application is hosted on www.example.com
. It is making a request to a public API, say this GOT API which is hosted on www.thronesapi.com
. This would be a cross-origin resource sharing.
CORS error
CORS error occurs when the server receiving the request is not allowed to share the resource with a different origin.
For instance, let's say that www.thronesapi.com
is not allowed to respond to any request coming from an origin other than thronesapi.com
. In that case, when we make a request to the API from a browser, we will see a CORS error.
See the CORS error in action
To see the CORS error in action, carry out the following steps -
Open a new tab and visit
guptashreyansh.github.io
.Open the browser console.
Make a request to the following URL -
https://shreyanshgupta.hashnode.dev/rss.xml
.
You can simply copy this code and paste it in the console -
fetch("https://shreyanshgupta.hashnode.dev/rss.xml")
.then(res => res.text())
.then(console.log)
You will see the following error -
Why does a CORS error occur?
The CORS error is a security measure that is implemented in the browsers. If the browsers do not find an Access-Control-Allow-Origin
header (with the current origin whitelisted) in the response, it will block the response from being loaded. In the case of preflight requests, the absence of the required value will simply block the actual request from being executed.
How to fix the CORS error?
To fix the CORS error, we need to set the Access-Control-Allow-Origin
header in the response.
A value like this - Access-Control-Allow-Origin: https://guptashreyansh.github.io
- will allow us to query Hashnode's RSS XML page on my website.
A value like this - Access-Control-Allow-Origin: *
- will allow us to query Hashnode's RSS XML page on any website.
This value needs to be set on shreyanshgupta.hashnode.dev
server.
We did not try requesting the thronesapi
because it has CORS enabled. You can try it out by making a fetch
request to this API from any origin -
fetch("https://thronesapi.com/api/v2/characters")
.then(res => res.json())
.then(console.log)
You can check out more public APIs, and whether or not CORS is enabled for them, on this page.
Using a CORS proxy
One way to bypass CORS is to simply update the response's headers on the server. But what if we don't have access to the server? For instance, we are querying the RSS XML page from Hashnode but we don't have access to Hashnode's server. So we can't update the response's headers.
In this case, we can use a CORS proxy.
CORS blocking is implemented in the browsers. This means that if you were to make the same request from a server, you'd load the response without any problems.
In CORS proxy, we set up a proxy server. We provide that server with the URL we want to query from. The proxy server queries the response and forwards it to us with appropriate headers.
Make a request through a CORS proxy
Let us make the same request through a CORS proxy. Follow the following steps -
Visit -
https://cors-anywhere.herokuapp.com/corsdemo
- and request temporary access to the demo server.Open a new tab and visit
guptashreyansh.github.io
.Open the browser console.
Make a request to the following URL -
https://shreyanshgupta.hashnode.dev/rss.xml
- this time through the CORS proxy.
fetch("https://cors-anywhere.herokuapp.com/https://shreyanshgupta.hashnode.dev/rss.xml")
.then(res => res.text())
.then(console.log)
You'll see that the response loads successfully.
Set up your own CORS proxy
This is the technique that I am using to query and render the Hashnode articles on my website. But I am not using https://cors-anywhere.herokuapp.com
since it is a test-only server.
Instead, I deployed my CORS proxy on Cloudflare (since Heroku does not have a free tier anymore). Cloudflare provides free-tier limits that are good enough for me but YMMV.
Take a look at the following repository to deploy your Cloudflare proxy. If you'd like to deploy on Heroku, you can check out the cors-anywhere repository.
Further readings
If you'd like to know more about CORS, I recommend checking out this MDN article - https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS
.
Subscribe to my newsletter
Read articles from Shreyansh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
