A Guide to React's onClick Handler: Preventing Typical Issues

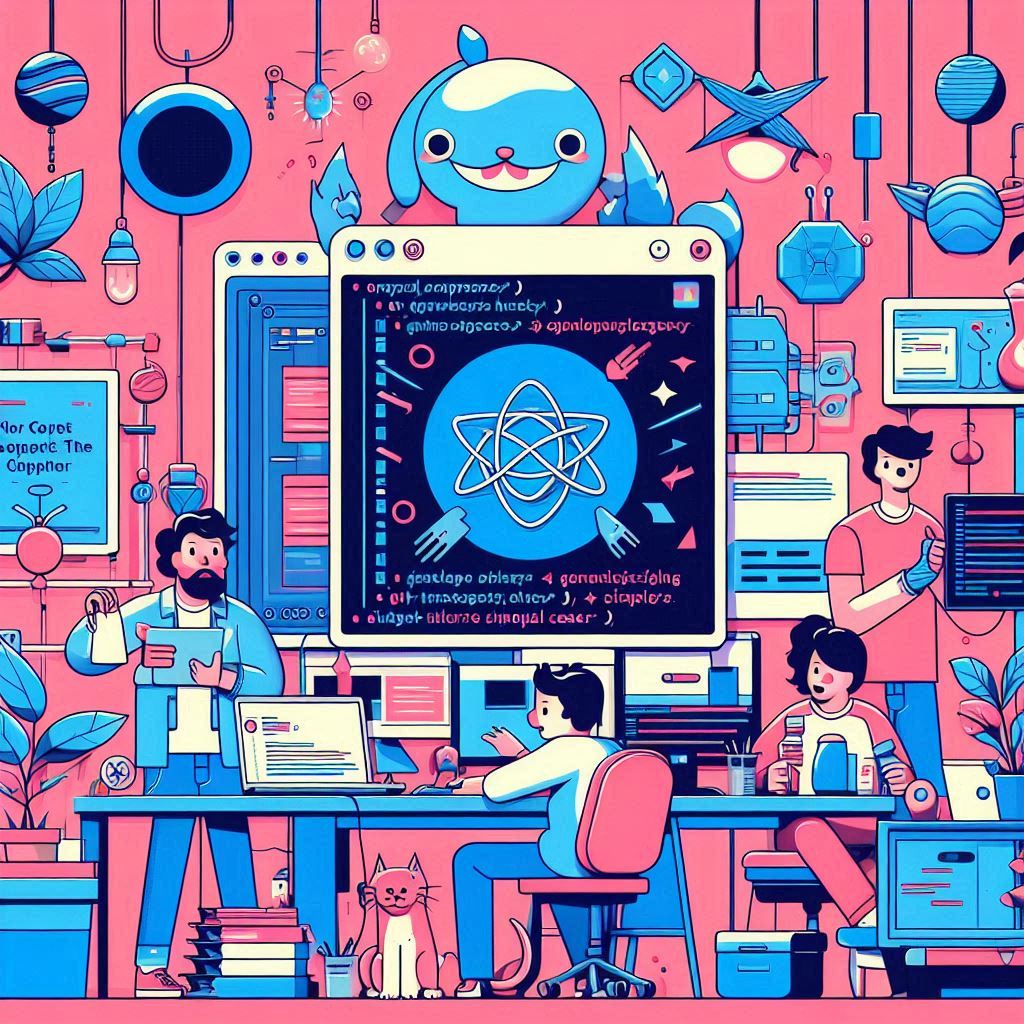
Handling user interactions in React, like button clicks, is pretty straightforward. But there are some subtle issues that can trip you up. Let’s explore a common problem and how to fix it.
The Problem
Take a look at this React component:
import { useState } from 'react';
import './App.css';
function App() {
const [count, setCount] = useState(0);
return (
<div>
<button onClick={setCount(count + 1)}>Counter {count}</button>
</div>
);
}
export default App;
//there is one problem in this code , This problem wont let this component to render
Looks fine, right? You’d expect that clicking the button would increase the count
and display the updated value. But surprisingly, this component doesn’t render properly. Why?
Immediate Execution vs. Function Definition
The issue is with how the onClick
handler is defined. In this problematic version:
Immediate Execution vs. Function Definition
The issue is with how the onClick
handler is defined. In this problematic version:
javascriptCopy code<button onClick={setCount(count + 1)}>Counter {count}</button>
The expression setCount(count + 1)
runs immediately when the component renders. This means setCount
is called during the render phase, updating the state, which triggers a re-render. This causes an infinite loop where React keeps trying to update and re-render the component. React catches this and stops the rendering to prevent crashing.
The Solution: Use a Function for the onClick
Handler
To fix this, make sure the state update only happens when the button is clicked. Pass a function to the onClick
handler:
javascriptCopy codeimport { useState } from 'react';
import './App.css';
function App() {
const [count, setCount] = useState(0);
return (
<div>
<button onClick={() => setCount(count + 1)}>Counter {count}</button>
</div>
);
}
export default App;
In this fixed version, () => setCount(count + 1)
is a function definition. React sets up the event listener with this function, which only runs when the button is clicked.
Why This Works
Deferred Execution: The function
() => setCount(count + 1)
runs only in response to the button click event, not during the render phase.Controlled State Update: The state update happens only when the user clicks the button, avoiding unnecessary and problematic re-renders.
No Infinite Loop: By deferring the state update, we avoid the infinite loop, allowing React to render the component properly.
Another Common Pitfall: Event Handler Binding
Another common mistake involves binding functions to event handlers, especially in class components. Check out this example using a class component:
javascriptCopy codeimport React, { Component } from 'react';
import './App.css';
class App extends Component {
constructor() {
super();
this.state = { count: 0 };
this.handleClick = this.handleClick.bind(this); // Correctly binding the method
}
handleClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<button onClick={this.handleClick}>Counter {this.state.count}</button>
</div>
);
}
}
export default App;
The Binding Issue
In class components, methods must be properly bound to the component instance. If you forget to bind the method in the constructor, like this:
javascriptCopy codeconstructor() {
super();
this.state = { count: 0 };
// this.handleClick = this.handleClick.bind(this); // Missing binding
}
You’ll get an error when the button is clicked because this
inside handleClick
will be undefined
.
The Solution: Arrow Functions
A modern and cleaner approach is to use arrow functions, which automatically bind this
to the component instance:
javascriptCopy codeimport React, { Component } from 'react';
import './App.css';
class App extends Component {
state = { count: 0 };
handleClick = () => {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<button onClick={this.handleClick}>Counter {this.state.count}</button>
</div>
);
}
}
export default App;
Why This Works
Automatic Binding: Arrow functions inherit
this
from the enclosing scope, sothis
is correctly bound to the component instance.Simpler Code: Using arrow functions for class methods eliminates the need for explicit binding in the constructor, making the code cleaner and less error-prone.
Conclusion
Handling events in React requires a careful approach to ensure state updates occur at the right time and functions are properly bound. By understanding the difference between immediate execution and deferred function execution, as well as the importance of binding in class components, you can avoid common pitfalls and create more robust, responsive applications.
Next time you define an onClick
handler, remember to use a function and consider using arrow functions for class methods. These small adjustments can make a big difference in the behavior and performance of your React components. Happy coding!
Subscribe to my newsletter
Read articles from KUNAL VITTHAL DHOBE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
