Actions Class In Selenium
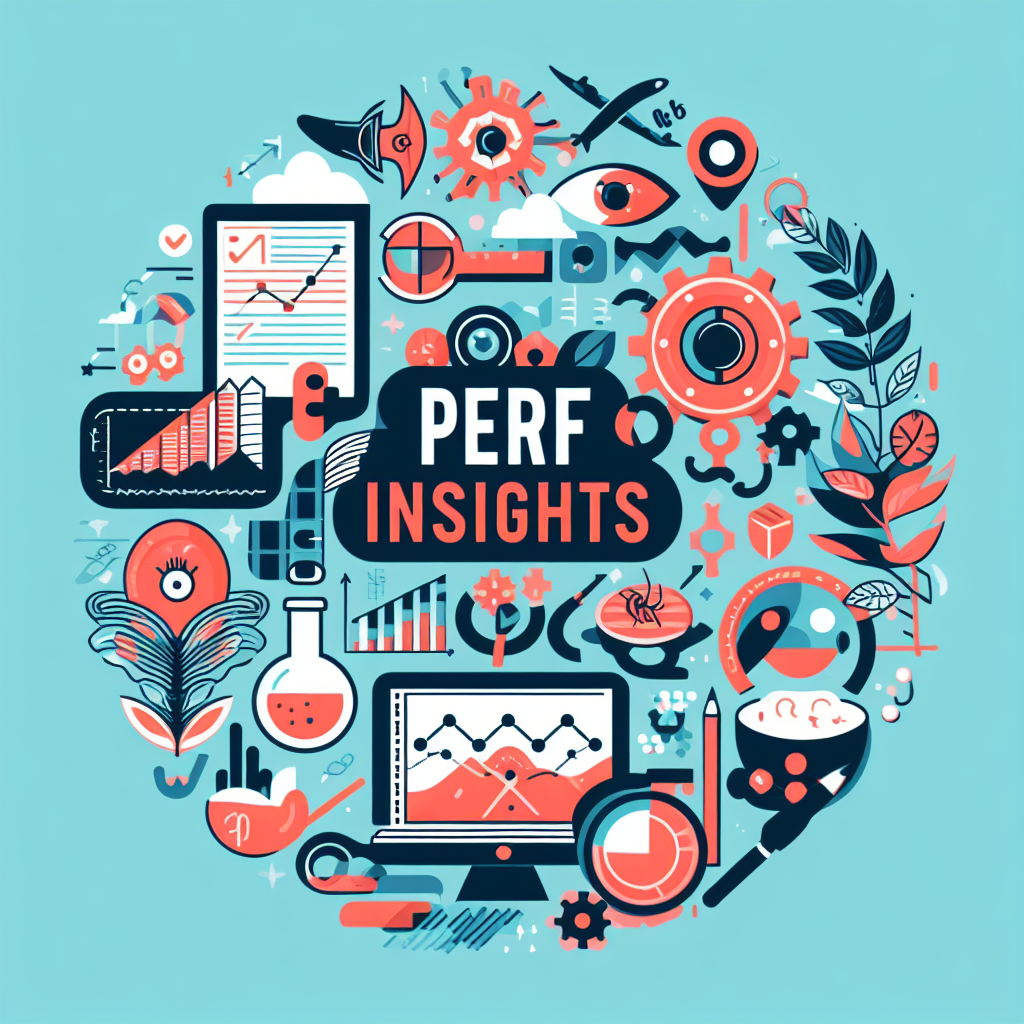
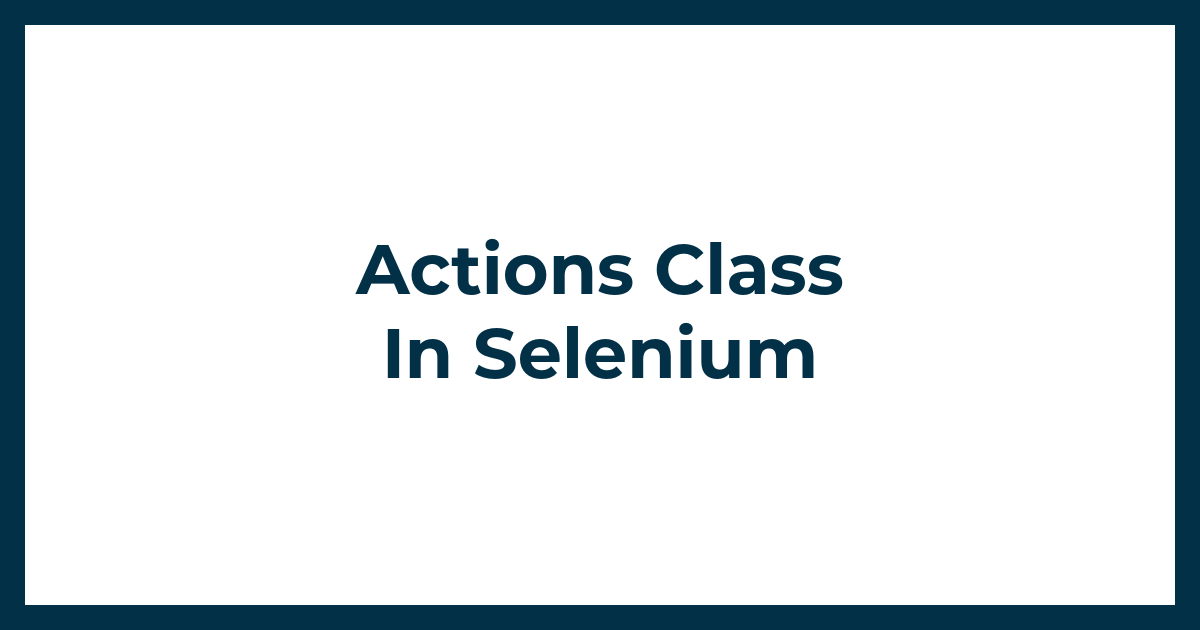
Introduction
Actions class in Selenium helps us in automating the mouse and keyboard actions. Basic actions like click and enter text in a field can be handled using basic Selenium command. We need Actions class in selenium to perform all the keyboard and mouse actions.
Commonly used Actions class methods
moveToElement(WebElement element)
If you wants to move your mouse over an element you can use this method
actions.moveToElement(element).build().perform();
doubleClick(WebElement element)
To perform the double click action of mouse you can use this method
actions.doubleClick(element).build().perform();
dragAndDrop(WebElement source, WebElement dest)
To perform the click and hold the source element and release it on target element, that can be performed with the help of dragAndDrop() method.
actions.dragAndDrop(source, dest).build().perform();
dragAndDropBy(WebElement source, int xOffset, int yOffset)
To perform dragAndDrop action on a given offset then you can use this method.
actions.dragAndDropBy(source, xOffset, yOffset).build().perform();
contextClick(WebElement element)
To perform the right click action of mouse we can use this method
actions.contextClick(element).build().perform();
keyDown(Keys.key)
To perform keyboard actions we can use this method, like if you want to perform
ctrl+A
action then this method can be used to perform that.actions.keyDown(Keys.CONTROL).sendKeys("a").build().perform();
build() and perform()
The method build()
and perform()
in Actions class are used to compile all the actions and execute the same.
build() : this method is basically used to compile all the actions, and
perform() : this method is used to execute the compiled action that were built using
build()
.
Implementing Actions Class
In below code I have performed few action :
Move to and click
Hold down the Control key and click
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
import org.testng.annotations.Test;
import java.time.Duration;
public class ActionsBlog {
@FindBy(xpath = "//a[text()='More']//parent::li")
WebElement elementForMoreDropDown;
@FindBy(xpath = "//a[text()='Dynamic Data']//parent::li")
WebElement elementForDynamicData;
@FindBy(xpath = "//a[text()='WebTable']/parent::li")
WebElement elementForWebTable;
WebDriver driver;
Actions actions;
@Test
public void actionsClass() throws Exception{
driver = new FirefoxDriver();
PageFactory.initElements(driver, this);
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.get("https://demo.automationtesting.in/Windows.html");
actions = new Actions(driver);
actions.moveToElement(elementForMoreDropDown).click(elementForDynamicData).build().perform();
Thread.sleep(2000);
actions.keyDown(Keys.CONTROL).click(elementForWebTable).build().perform();
Thread.sleep(5000);
driver.quit();
}// test
}// class
Conclusion
Actions class in Selenium is a powerful tool that allows you to automate the mouse and keyboard action and helps you in creating more realistic test scripts.
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
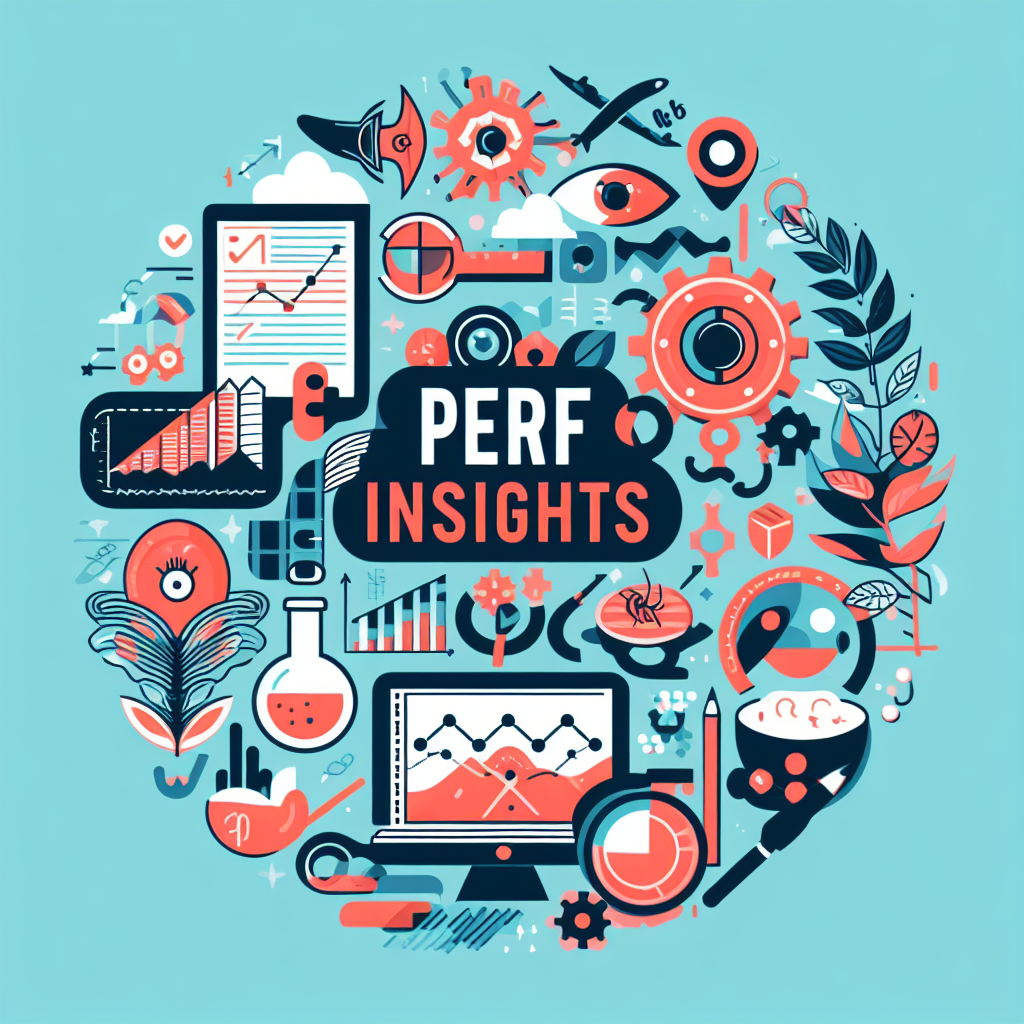
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.