Day 3: Operators and Expressions in JavaScript
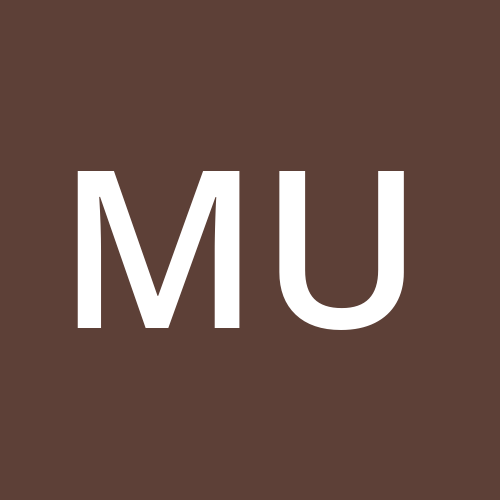
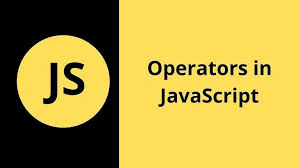
Welcome back to our JavaScript series! Today, we'll learn about operators and expressions, which are essential to performing operations .
Operators
Operators are symbols that perform specific operations on variables and values. Some common types of operators in JavaScript are :-
1. Arithmetic Operators
These operators perform basic mathematical (Arithmetic) operations.
Addition (
+
): Adds two numbers.let sum = 5 + 3; console.log(sum); // Output: 8
Subtraction (
-
): Subtracts one number from another.let difference = 10 - 4; console.log(difference); // Output: 6
Multiplication (
*
): Multiplies two numbers.let product = 4 * 2; console.log(product); // Output: 8
Division (
/
): Divides one number by another.let quotient = 8 / 2; console.log(quotient); // Output: 4
Modulus (
%
): Finds the remainder of division.let remainder = 10 % 3; console.log(remainder); // Output: 1
2. Assignment Operators
These operators assign values to the variables.
Assignment (
=
): Assigns a value to a variable.let x = 5;
Addition Assignment (
+=
): Adds a value to a variable.let y = 3; y += 2; // y = y + 2 console.log(y); // Output: 5
Subtraction Assignment (
-=
): Subtracts a value from a variable.let z = 10; z -= 4; // z = z - 4 console.log(z); // Output: 6
Multiplication Assignment (
*=
): Multiply a value with a variable.let z = 10; z *= 4; // z = z * 4 console.log(z); // Output: 40
3. Comparison Operators
These operators compare two values and return a boolean (true or false).
Equal to (
==
): Checks if only the two values are equal.console.log(5 == '5'); // Output: true
Strict Equal to (
===
): Checks if two values and their datatypes are also equal.console.log(5 === '5'); // Output: false
Not Equal to (
!=
): Checks if two values are not equal.console.log(5 != '5'); // Output: false
Greater than (
>
): Checks if one value is greater than another.console.log(8 > 3); // Output: true
Less than (
<
): Checks if one value is less than another.console.log(2 < 6); // Output: true
4. Logical Operators
These operators are used to combine multiple conditions.
AND (
&&
): Returns true if both conditions are true.console.log(5 > 3 && 8 > 6); // Output: true
OR (
||
): Returns true if at least one condition is true.console.log(5 > 3 || 2 > 6); // Output: true
NOT (
!
): Reverses the boolean value.console.log(!(5 > 3)); // Output: false
5. Conditional (Ternary) Operator
This operator is a shorthand for the if...else
statement.
let age = 18;
let canVote = (age >= 18) ? 'Yes' : 'No';
console.log(canVote); // Output: Yes
Expressions
Expressions are combinations of operands and operators that produce a result on evaluation. For example:
let a = 5;
let b = 10;
let result = a + b;
console.log(result); // Output: 15
Summary
Today, we covered various types of operators and how to use them in expressions. Operators help you perform calculations, assign values, and make comparisons. Tomorrow, we'll dive into control flow, which allows you to make decisions in our code.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
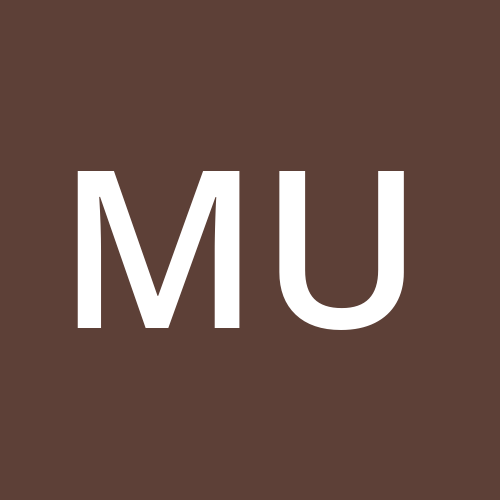