Exploring Random Forests: A Beginner's Guide to Ensemble Learning

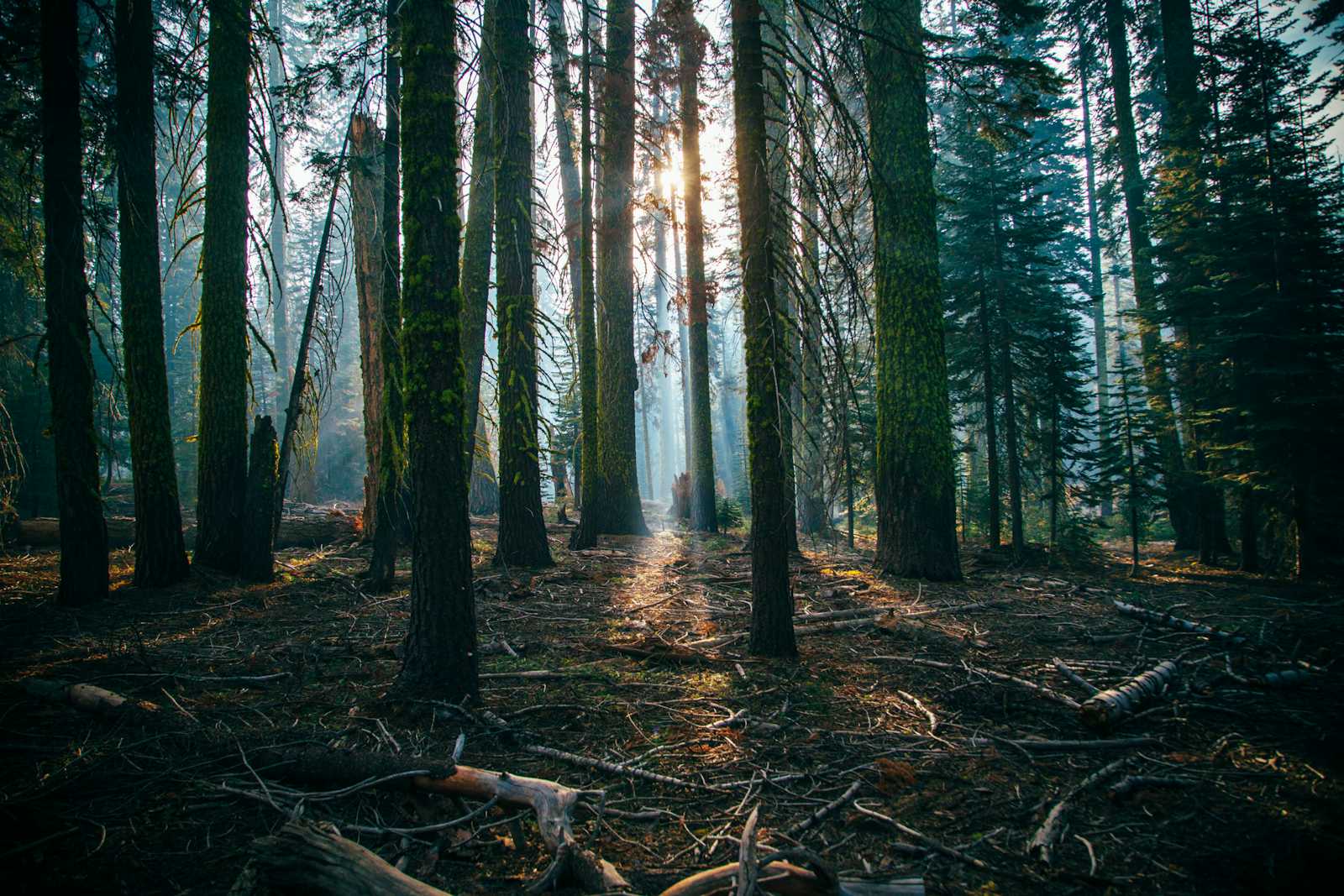
In my previous blog, we explored the fascinating world of Decision Trees, a fundamental algorithm in machine learning. Today, we will take a step further into the realm of ensemble learning by diving into Random Forests. This blog aims to provide a comprehensive understanding of Random Forests, enriched with examples, code snippets, and resources to help you on your machine learning journey.
Why Move Beyond Decision Trees?
While Decision Trees are powerful and intuitive, they have their limitations, particularly in terms of overfitting and variance. This is where ensemble learning techniques like Random Forests come into play. Ensemble learning combines multiple models to improve overall performance and robustness.
What is a Random Forest?
A Random Forest is an ensemble learning method that constructs multiple decision trees during training and outputs the mode of the classes (classification) or mean prediction (regression) of the individual trees. The key idea is to reduce overfitting and improve generalization by averaging the results of many decision trees.
Some Video Links For Quick Understanding Of Concept:
To enhance your understanding of Random Forests, here are some video resources that provide detailed explanations:
StatQuest with Josh Starmer: This video offers a clear and engaging explanation of Random Forests, breaking down the concepts into easy-to-understand segments. Watch here
Simplilearn: This tutorial covers the basics of Random Forests, including how they work and their applications in machine learning. Watch here
Edureka: This video provides an in-depth look at Random Forests, including practical examples and code demonstrations. Watch here
How Does Random Forest Work?
Bootstrap Sampling: Random Forests use a technique called bootstrap sampling to create multiple subsets of the training data. Each subset is used to train a different decision tree.
Random Feature Selection: At each split in the decision tree, a random subset of features is selected. This ensures that the trees are diverse and reduces the correlation between them.
Aggregation: The final prediction is made by aggregating the predictions of all the individual trees. For classification, it’s the majority vote, and for regression, it’s the average prediction.
Implementing Random Forests: A Step-by-Step Guide
Let's walk through the process of implementing a Random Forest using Python and the scikit-learn library.
Step 1: Import Libraries
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
Step 2: Load and Prepare Data
For this example, we’ll use the famous Iris dataset.
# Load dataset
data = pd.read_csv('iris.csv')
# Split data into features and target
X = data.drop('species', axis=1)
y = data['species']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 3: Train the Random Forest Model
# Initialize the model
rf_model = RandomForestClassifier(n_estimators=100, random_state=42)
# Train the model
rf_model.fit(X_train, y_train)
Step 4: Make Predictions and Evaluate
# Make predictions
y_pred = rf_model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy * 100:.2f}%')
Visualizing the Random Forest
Visualizing individual trees in a Random Forest can be insightful. Here’s how you can visualize one of the trees:
from sklearn.tree import export_graphviz
import graphviz
# Export one of the trees
tree = rf_model.estimators_[0]
dot_data = export_graphviz(tree, out_file=None, feature_names=X.columns, class_names=y.unique(), filled=True, rounded=True, special_characters=True)
graph = graphviz.Source(dot_data)
graph.render("iris_tree")
Advantages of Random Forests
Robustness: Random Forests are less likely to overfit compared to individual decision trees.
Versatility: They can handle both classification and regression tasks.
Feature Importance: Random Forests provide insights into feature importance, helping in feature selection.
Challenges and Considerations
While Random Forests are powerful, they are not without challenges. They can be computationally intensive and may require tuning of hyperparameters like the number of trees, maximum depth, and the number of features to consider at each split.
Resources and Further Reading
Conclusion
Transitioning from Decision Trees to Random Forests is a significant milestone in the machine learning journey. The ability to build robust models that generalize well to new data is invaluable. I hope this blog has provided you with a clear understanding of Random Forests and inspired you to explore this powerful algorithm further.
Happy "Code Inferno" !!
Happy Coding !!
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
👋 Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.