Introduction to Design Patterns in Java

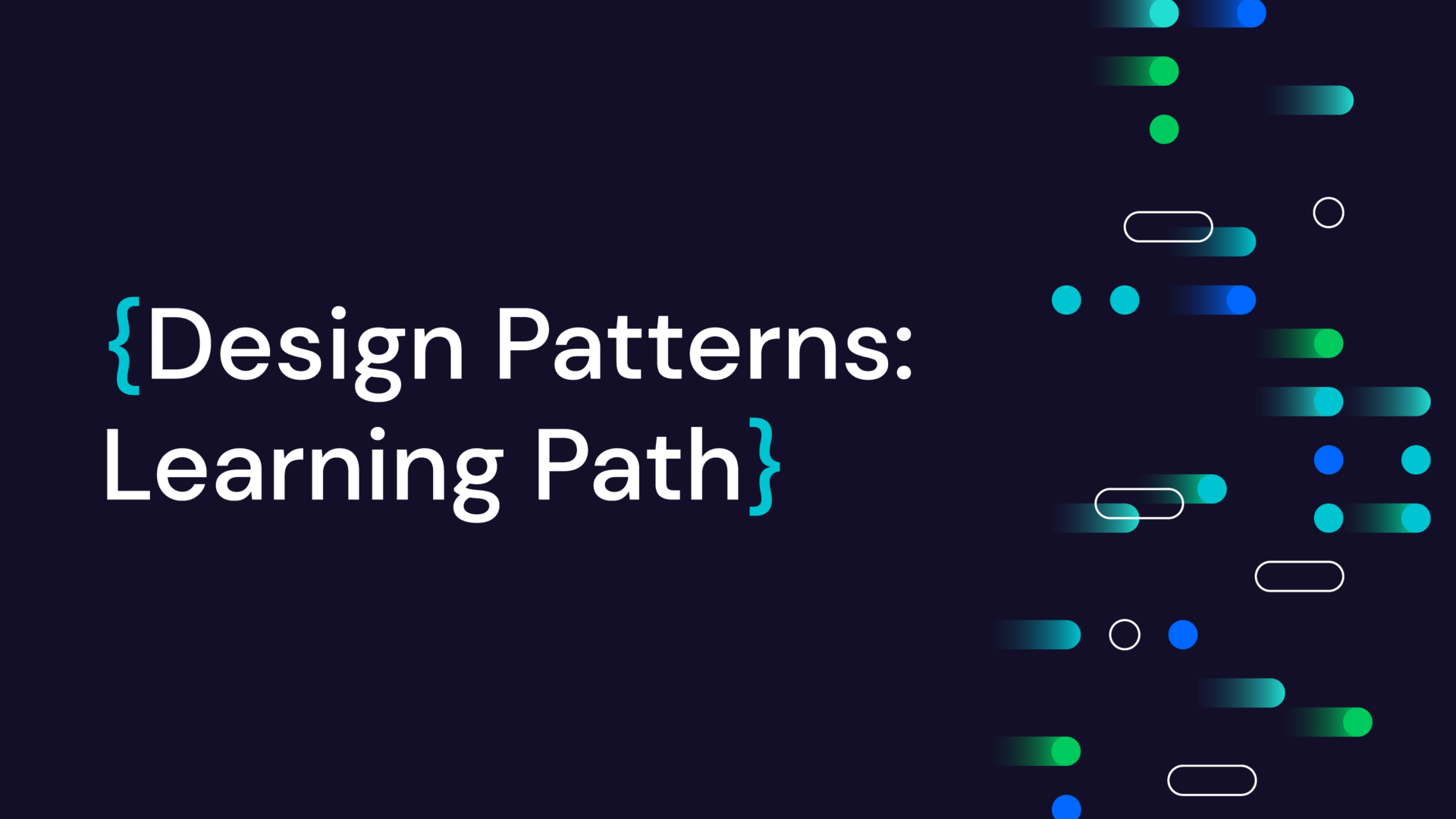
Introduction
Definition of Design Patterns
Design patterns are reusable solutions to common problems that occur in software design. They are best practices that provide a template for solving recurring design issues in a way that is effective, efficient, and maintainable. Design patterns can be classified into three main categories: Creational, Structural and Behavioral design pattern.
Design patterns are essential tools that enhance the efficiency, quality, and maintainability of software projects, ultimately leading to better outcomes in software development.
Importance of Design Patterns in Software Development
Design patterns play a crucial role in software development for several reasons:
1. Reusability
Design patterns provide proven solutions that can be reused across different projects, reducing development time and effort.
2. Standardization
They offer a common language and framework for developers, facilitating clearer communication about design decisions and promoting consistency in coding practices.
3. Maintainability
Patterns help create code that is easier to understand and maintain. By following established patterns, developers can create a more predictable structure, making it easier to update and enhance applications over time.
4. Flexibility and Scalability
Design patterns promote flexibility by encouraging modular architecture. This allows developers to change components without affecting the entire system, making it easier to scale applications.
5. Problem-Solving
They provide a catalog of solutions to common problems, helping developers avoid reinventing the wheel and instead focus on higher-level design concerns.
6. Improved Quality
Using design patterns can lead to higher-quality software by encouraging best practices, reducing bugs, and enhancing the overall robustness of the system.
7. Facilitate Collaboration
Patterns enable better collaboration among team members by providing a shared understanding of system architecture and design principles.
8. Encourage Good Design Principles
Design patterns embody principles such as SOLID, DRY (Don't Repeat Yourself), and KISS (Keep It Simple, Stupid), promoting good design practices in software development.
Types of Design Patterns
Creational Patterns
Singleton
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is used when exactly one object is needed to coordinate actions across a system. It's particularly useful in scenarios like logging, driver objects, caching, thread pools, and database connections. Read more
Factory Method
The Factory Method Pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. It promotes loose coupling by eliminating the need to bind application-specific classes into the code. The pattern helps in creating objects without having to specify the exact class of the object that will be created. Read more
Abstract Factory
The Abstract Factory Pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. This pattern is useful for creating complex objects that require extensive setup and coordination. It allows the interchangeability of product families without altering the client code.
Builder
The Builder Pattern separates the construction of a complex object from its representation. By using different builders, the same construction process can create different representations. This pattern is often used when objects need to be created in multiple steps or when an object needs to be constructed in a specific sequence.
Prototype
The Prototype Pattern is used to create new objects by copying an existing object, known as the prototype. This pattern is beneficial when the process of creating a new instance is more expensive than copying an existing one. It's particularly useful when the initialization of an object is complex or when the object creation requires significant resources.
Structural Patterns
Adapter
The Adapter Pattern allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces by converting the interface of a class into another interface that a client expects. This pattern is particularly useful when integrating new components into an existing system that was not designed to work with them.
Bridge
The Bridge Design Pattern is a structural design pattern that separates an object's abstraction from its implementation so that the two can vary independently. This pattern is particularly useful in scenarios where there are multiple dimensions of variability in an object, allowing for greater flexibility and scalability in the system's design.
Composite
The Composite Pattern allows you to compose objects into tree structures to represent part-whole hierarchies. This pattern enables clients to treat individual objects and compositions of objects uniformly. It's commonly used for representing hierarchies of user interface components or filesystems.
Decorator
The Decorator Pattern allows behavior to be added to an individual object, dynamically, without affecting the behavior of other objects from the same class. This pattern is useful for extending the functionalities of objects in a flexible and reusable way. It's often used for adhering to the Single Responsibility Principle by dividing functionality among classes with unique areas of concern.
Facade
The Facade Pattern provides a simplified interface to a complex subsystem. It defines a higher-level interface that makes the subsystem easier to use. This pattern is used to reduce dependencies and simplify the interface for interacting with a complex system, making it easier for clients to use and understand.
Flyweight
The Flyweight Design Pattern is a structural design pattern that aims to minimize memory usage by sharing as much data as possible with other similar objects. It is particularly useful in systems where a large number of similar objects are used, as it helps to reduce the overhead associated with object creation and memory consumption.
Proxy
The Proxy Pattern provides a surrogate or placeholder for another object to control access to it. This pattern is used to create a representative object that controls access to another object, which might be remote, expensive to create, or in need of secure access. Proxies are often used in lazy initialization, access control, and remote method invocation.
Behavioral Patterns
Chain of Responsibility
The Chain of Responsibility Design Pattern is a behavioral design pattern that allows an object to pass a request along a chain of potential handlers until the request is handled. Each handler in the chain either handles the request or passes it to the next handler in the chain. This pattern decouples the sender of a request from its receiver, giving more flexibility in assigning responsibilities to objects.
Command
The Command Pattern encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. It also provides support for undoable operations. This pattern is useful for implementing callback functions, queuing requests, and handling multi-level undo/redo mechanisms.
Interpreter
The Interpreter Design Pattern is a behavioral design pattern that defines a representation for a language's grammar along with an interpreter that uses this representation to interpret sentences in the language. It is particularly useful for designing and implementing domain-specific languages.
Iterator
The Iterator Pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. This pattern is useful for traversing collections of objects, such as lists or arrays, in a consistent manner, providing a standard way to loop through different data structures.
Mediator
The Mediator Design Pattern is a behavioral design pattern that defines an object (the mediator) to encapsulate how a set of objects interact. This pattern promotes loose coupling by preventing objects from referring to each other explicitly, allowing their interaction to be varied independently.
Memento
The Memento Design Pattern is a behavioral design pattern that allows you to capture and externalize an object's internal state so that it can be restored to this state later without violating encapsulation. This pattern is particularly useful for implementing undo mechanisms.
Observer
The Observer Pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is useful for implementing distributed event-handling systems and is commonly used in scenarios like user interface frameworks, event-driven systems, and notification services.
State
The State Pattern allows an object to alter its behavior when its internal state changes. This pattern encapsulates state-specific behavior and changes the behavior of the context object when its state changes. It's particularly useful for implementing state machines and managing state transitions in an organized manner.
Strategy
The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. The strategy pattern allows the algorithm to vary independently from the clients that use it. It's useful for defining a series of algorithms and making them easily interchangeable, promoting the Open/Closed Principle.
Template Method
The Template Method Design Pattern is a behavioral design pattern that defines the skeleton of an algorithm in a method, deferring some steps to subclasses. It allows subclasses to redefine certain steps of an algorithm without changing its structure. This pattern is particularly useful for situations where you have multiple classes performing similar tasks with some variations.
Visitor
The Visitor Design Pattern is a behavioral design pattern that allows you to add further operations to objects without having to modify them. It achieves this by allowing you to separate algorithms from the objects on which they operate. This pattern is particularly useful when you have a complex object structure and you want to perform operations on these objects without changing their classes.
Best Practices for Using Design Patterns
Know Your Patterns: Familiarize yourself with various design patterns and their use cases to apply the most suitable one for your situation.
Keep It Simple: Avoid overcomplicating designs by using patterns unnecessarily; prioritize simplicity and clarity.
Adapt Patterns to Fit: Modify design patterns as needed to better fit your specific requirements rather than adhering strictly to their original forms.
Use Patterns Judiciously: Apply design patterns only when they add value; avoid using them just for the sake of using patterns.
Document Your Choices: Provide clear documentation explaining why a particular pattern was chosen, which can help future developers understand the design decisions.
Prioritize Readability: Ensure that the code remains readable and understandable, even when using design patterns.
Test Extensively: Implement unit tests to validate that the application of the design pattern works as intended and integrates well with other components.
Refactor When Necessary: Be open to refactoring your design if a pattern does not work out as expected or if the application evolves.
Collaborate and Share Knowledge: Encourage discussions and knowledge sharing about design patterns within your team to foster a better understanding and improved implementations.
Conclusion
Recap of Key Points
This article introduces design patterns as generic solutions to common software design problems, emphasizing their importance in creating maintainable, robust, and extensible applications. It provides an overview of design patterns in Java, categorizing them into Creational, Structural, and Behavioral patterns. Key patterns discussed include Singleton, Factory Method, Abstract Factory, Builder, Prototype, Adapter, Bridge, Composite, Decorator, Facade, Flyweight, Proxy, Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method, and Visitor. The article also covers best practices, when to use design patterns, avoiding their overuse, and combining them effectively, concluding with a recap, future trends, and encouragement for ongoing learning.
Subscribe to my newsletter
Read articles from Vishad Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
