Key Advantages of Zod in TypeScript Validation
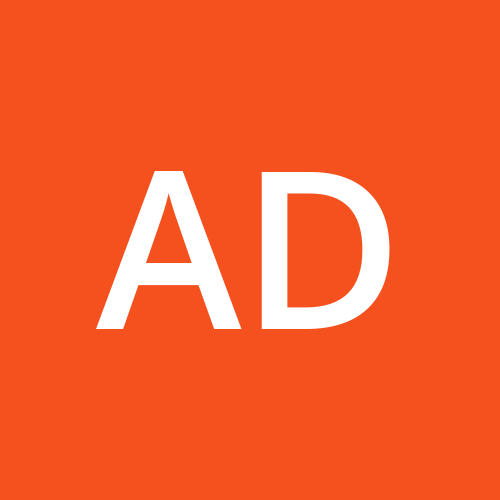
Introduction
In modern web development, validating user input is crucial for ensuring data integrity and security. Various libraries and tools can help with this task, but Zod stands out for its simplicity, type safety, and powerful capabilities. In this article, we'll dive into Zod and explore how to use it for common validation tasks, using real-world examples.
What is Zod?
Zod is a TypeScript-first schema declaration and validation library. It allows you to define schemas for your data and provides validation functions to ensure that the data matches the schema. Zod is known for its developer-friendly API, comprehensive error messages, and strong TypeScript integration.
Getting Started with Zod
First, install Zod in your project:
npm install zod
Validating a Username
Let's start with a simple example: validating a username. The username should be between 2 and 20 characters long and can only contain letters, numbers, and underscores. Here's how you can define this validation using Zod:
import { z } from "zod";
export const usernameValidations = z
.string()
.min(2, 'Username must be at least two characters')
.max(20, 'Username must be no more than twenty characters')
.regex(/^[a-zA-Z0-9_]+$/, 'Username can contain only letters, numbers, and underscores.');
In this code:
z.string()
ensures the value is a string..min(2, 'Username must be at least two characters')
specifies a minimum length of 2 characters..max(20, 'Username must be no more than twenty characters')
specifies a maximum length of 20 characters..regex(/^[a-zA-Z0-9_]+$/, 'Username can contain only letters, numbers, and underscores.')
ensures the username contains only allowed characters.
Creating a Sign-Up Schema
Next, let's create a sign-up schema that includes username, email, and password fields:
import { z } from "zod";
export const signUpSchema = z.object({
username: usernameValidations,
email: z.string().email({ message: "Please enter a valid email address" }),
password: z.string().min(6, { message: 'Password must be at least six characters' })
});
In this code:
username: usernameValidations
reuses the username validation schema.email: z.string().email({ message: "Please enter a valid email address" })
ensures the email is a valid format.password: z.string().min(6, { message: 'Password must be at least six characters' })
specifies a minimum password length of 6 characters.
Creating a Sign-In Schema
For sign-in, we typically need an identifier (which can be a username or email) and a password. Here's how to define this schema:
import { z } from "zod";
export const signInSchema = z.object({
identifier: z.string(),
password: z.string()
});
Creating a Verification Schema
For verification codes, we can specify a fixed length. Here's an example:
import { z } from "zod";
export const verifySchema = z.object({
code: z.string().length(6, 'Verification code must be 6 digits')
});
In this code:
code: z.string().length(6, 'Verification code must be 6 digits')
ensures the verification code is exactly 6 characters long.
Creating a Message Schema
When dealing with messages, we might want to enforce minimum and maximum lengths for the content. Here's how to define a schema for message content:
import { z } from "zod";
export const messageSchema = z.object({
content: z
.string()
.min(10, { message: 'Content must be at least 10 characters' })
.max(300, { message: 'Content must be less than 300 characters' })
});
Creating a Boolean Schema
Finally, let's define a schema for a boolean field, such as whether to accept messages:
import { z } from "zod";
export const AcceptMessageSchema = z.object({
acceptMessages: z.boolean()
});
Conclusion
Zod is a powerful and flexible library for schema declaration and validation in TypeScript. It simplifies the process of ensuring data integrity and provides comprehensive error messages to help developers debug issues quickly. By using Zod, you can create robust validation schemas for various data types and requirements, making your application more reliable and secure.
With the examples provided, you should have a good understanding of how to use Zod for common validation tasks in your projects. Happy coding!
Subscribe to my newsletter
Read articles from Ankit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
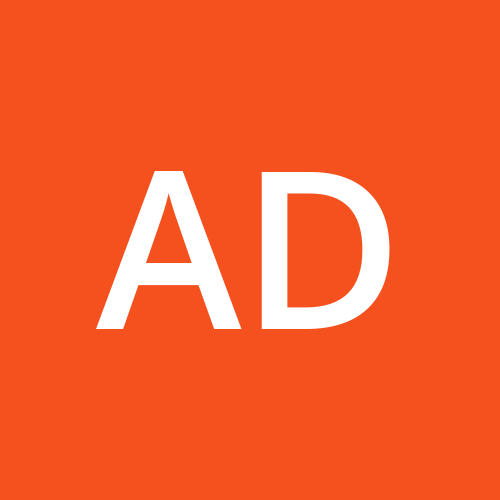