APIs: Your Developer Superpower Toolkit
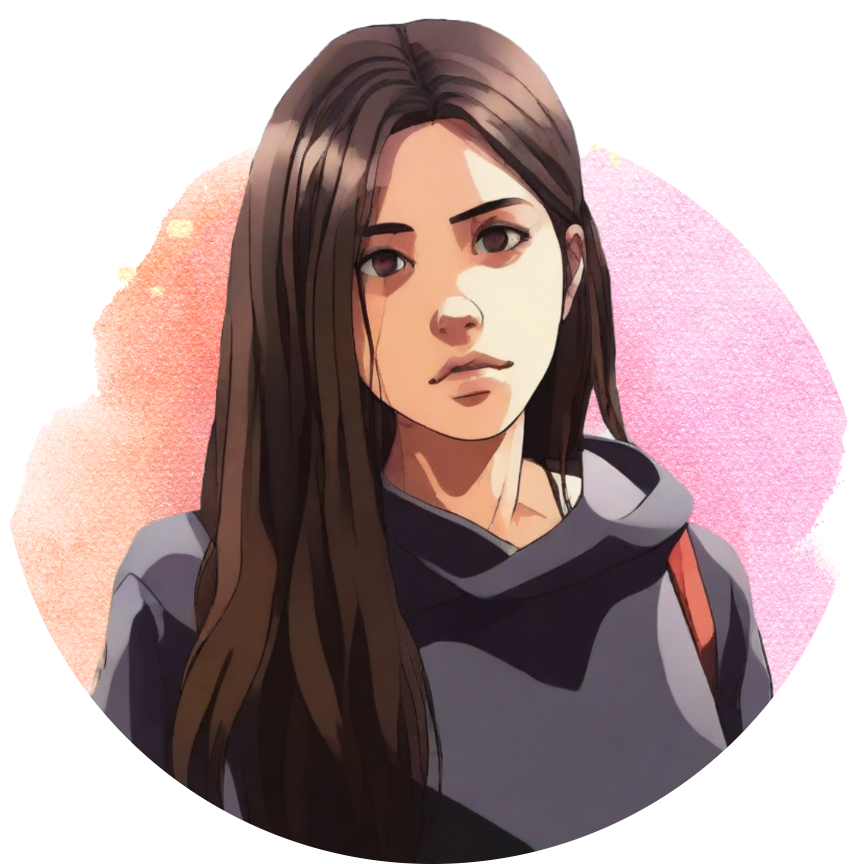
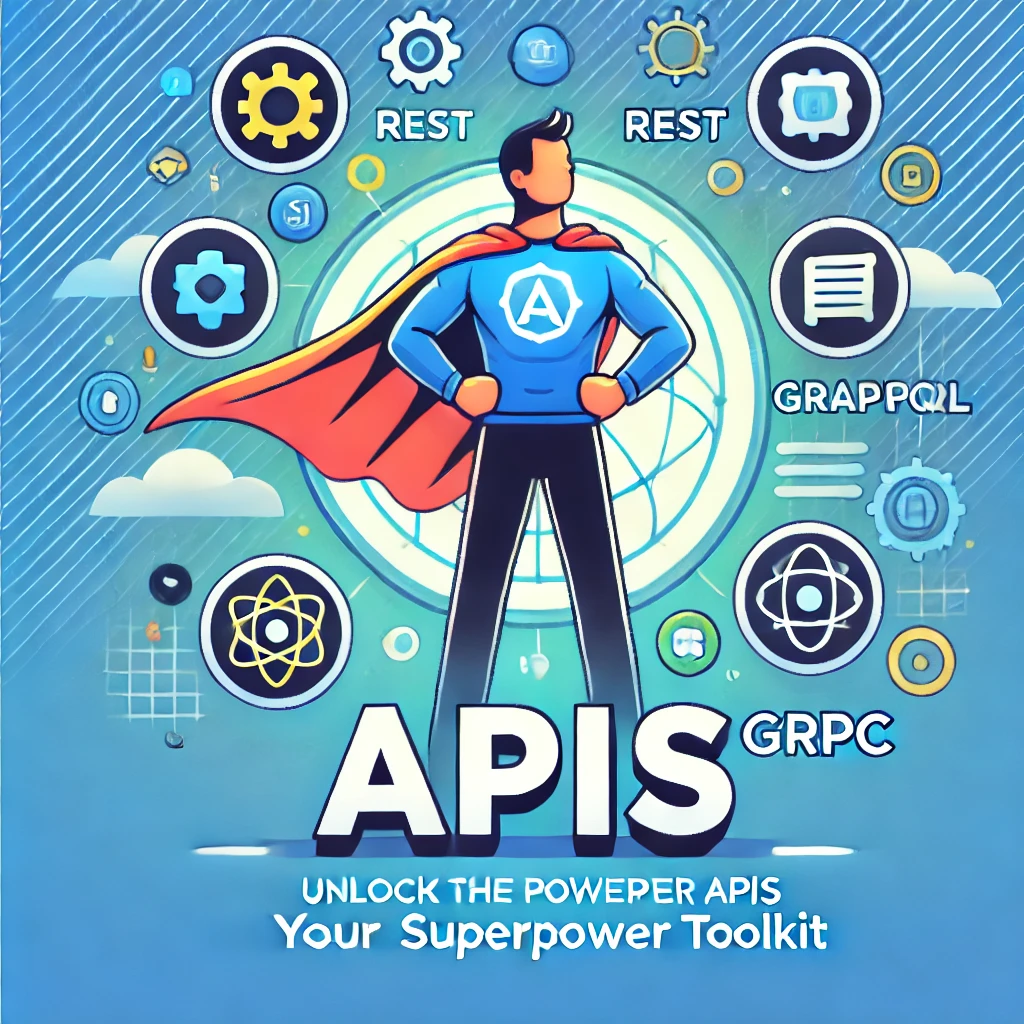
APIs are indeed superpowers for the developers. They grant developers incredible power to build applications that interact with a vast array of functionalities, from seamlessly integrating with third-party services to harnessing complex machine learning algorithms with a few lines of code. With APIs, developers can wield the power to create applications that are more innovative, efficient, and versatile than ever before. But.. what exactly are APIs, and how do they work?
What are APIs?
An API, which stands for Application Programming Interface, acts as a middleman between your application and another system, whether it's hardware or software. It provides a set of instructions and tools for developers to interact with that system, hiding the complex inner workings and exposing only the necessary functionalities. This allows developers to focus on building their applications without worrying about low-level details.
Think of APIs as a waiter who takes your order (request) to the kitchen (server) and brings back your food (response) without you needing to know how to cook (or, in my case, burn water).
Did You Know? APIs Are Everywhere!
APIs are more pervasive than you might think. Even seemingly standalone tools like Software Development Kits (SDKs) are essentially collections of APIs. An SDK provides pre-written code snippets and functions that interact with a specific platform or service, allowing developers to easily integrate those functionalities into their applications.
Types of APIs:
Local APIs: These allow applications to interact with your device's hardware, like the camera or microphone.
Remote Procedure Calls (RPC): These APIs let you control remote objects like drones or traffic lights or access services like real-time translation.
Messaging APIs: These enable applications to send and receive messages with other applications or services.
Database APIs: These provide access to data stored in databases.
Web APIs: These are the most popular type, allowing applications to interact with data and functionalities on the web.
The Power of Abstraction
APIs offer a powerful concept called abstraction. They hide the complexity of the underlying system and present a simplified interface for developers to work with. Imagine trying to convert a string to uppercase by manipulating bits in each letter. With an API, you can simply use a single function like .upper()
in Python. This makes development faster, easier, and less error-prone.
Without abstraction, programming would be like explaining quantum physics to a cat. You'd be stuck manually flipping bits and hoping your code doesn't explode. Abstraction saves you from being a digital caveman, reinventing the wheel every time.
APIs: The Building Blocks of the Web
The superpower of APIs extends far beyond individual applications. They are the glue that holds the web together. Different browsers can display the same web pages because they all rely on a set of web APIs. APIs power everything from playing music on your media player to accessing weather data.
REST APIs: A Popular Approach
The most common type of web API is the REST API, which stands for REpresentational State Transfer. REST APIs leverage existing web technologies like HTTP and utilize a standardized approach for making requests and receiving responses. This ensures consistent and predictable communication between applications.
How APIs Work
APIs work through a series of steps involving the client application, the server, and a well-defined communication protocol. Here's a breakdown of the process:
Client Makes a Request: The client application initiates the interaction by sending a request to the server. This request specifies what data or action the client needs. The request typically includes:
URL: The location of the resource on the server.
HTTP Verb: A verb like GET, POST, PUT, PATCH, or DELETE indicating the desired action (e.g., GET to retrieve data, POST to create a new resource).
Headers (Optional): Additional information about the request, such as authentication credentials or data format preferences.
Body (Optional): Data to be sent to the server, often in a format like JSON (JavaScript Object Notation).
Server Processes the Request: The server receives the request and uses the API logic to process it. This may involve accessing databases, performing calculations, or interacting with other systems.
Server Sends a Response: The server sends a response back to the client application. The response typically includes:
Status Code: A numeric code indicating the success or failure of the request (e.g., 200 for success, 404 for not found).
Headers (Optional): Additional information about the response, such as the content type of the data.
Body (Optional): The requested data or the result of the action performed by the server.
Client Receives the Response: The client application receives the response from the server and parses the data according to the API specifications. It then uses the retrieved data or acts upon the results to continue its execution.
API Architectures: The Rules of Engagement
While APIs provide a way to interact with a system, different architectural styles define how that interaction happens. These architectures establish guidelines for how data is structured, how requests are formatted, and how responses are delivered. Here are some of the most common API architectures:
REST (REpresentational State Transfer): As mentioned earlier, REST is the dominant architecture for web APIs. It leverages HTTP verbs (GET, POST, PUT, DELETE), URLs, and standard data formats (JSON, XML) to create a simple and flexible interface.
SOAP (Simple Object Access Protocol): SOAP is a more heavyweight architecture that uses XML for both requests and responses. It offers a higher level of security and control but can be more complex to implement compared to REST.
GraphQL: This newer architecture allows clients to request specific data in a single query, improving efficiency and reducing the number of roundtrips needed between client and server.
gRPC (gRPC Remote Procedure Calls): gRPC is a high-performance protocol based on Google's Protocol Buffers for data serialization. It's ideal for real-time communication and microservices architectures.
WebSockets: WebSockets provide a persistent, two-way communication channel between client and server. This enables real-time data exchange, making them suitable for chat applications or live data feeds.
Choosing the right API architecture depends on the specific needs of the application. REST remains popular for its simplicity and wide adoption, while GraphQL and gRPC offer advantages for complex data queries and performance-critical scenarios. WebSockets shine in real-time communication needs.
API Verbs: The Potent Words
Just like we use words to communicate, APIs rely on specific verbs to indicate the desired action within a request. These verbs are known as HTTP verbs. Here are the most common ones and how they correspond to CRUD operations (Create, Read, Update, Delete):
GET: This verb retrieves data from a resource on the server. It's analogous to the "Read" operation in CRUD.
POST: This verb creates a new resource on the server. It maps to the "Create" operation in CRUD.
PUT: This verb is used to update an existing resource on the server. It aligns with the "Update" operation in CRUD.
PATCH: Similar to PUT, PATCH updates a resource but allows for partial modifications.
DELETE: This verb removes a resource from the server, corresponding to the "Delete" operation in CRUD.
Beyond REST: Exploring New Frontiers
While REST APIs remain dominant, the world of APIs is constantly evolving. New approaches like GraphQL and Async APIs are emerging, offering additional flexibility and efficiency for specific use cases. This blog post is just a glimpse into the fascinating world of APIs. Stay tuned for future installments where we'll delve deeper into specific types of APIs, explore the latest trends, and unlock even more superpower for developers!
The Future of APIs
The world of APIs is constantly evolving. As application needs become more diverse, new architectures and tools are emerging to address them. Here are some trends to watch:
Microservices Architecture: This approach breaks down applications into smaller, independent services that communicate through APIs. This promotes modularity, scalability, and faster development cycles.
API Security: As APIs become more ubiquitous, security concerns become paramount. Techniques like authentication, authorization, and encryption are crucial to protect sensitive data and prevent unauthorized access.
API Documentation and Tools: Comprehensive documentation and user-friendly tools are essential for developers to discover, understand, and use APIs effectively.
Beyond the Basics: Exploring Different Types of APIs
We've covered the fundamental concepts of APIs, but there's a vast landscape to explore. Here's a glimpse into some of the different categories of APIs based on their functionality:
Public vs. Private APIs: Public APIs are available for anyone to use, while private APIs are restricted to authorized developers or within an organization.
Open APIs vs. Closed APIs: Open APIs have freely available specifications and documentation, while closed APIs have limited accessibility or require specific permissions.
Data APIs: These APIs provide access to specific data sets, enabling applications to retrieve and utilize the data for various purposes.
Payment APIs: These APIs facilitate secure online transactions, allowing applications to integrate payment processing functionalities.
Social Media APIs: These APIs allow applications to connect with social media platforms, enabling features like sharing content or user logins.
Machine Learning APIs: These APIs provide access to pre-trained machine learning models, allowing developers to incorporate intelligent capabilities into their applications without building the models from scratch.
This is just a starting point. There are countless other types of APIs catering to specific industries and functionalities.
In conclusion, APIs are crucial for modern software development. They enable smooth communication between applications and simplify complex functions, helping developers create strong applications efficiently. APIs drive our interconnected digital world, from local interactions to global web services. As technology evolves, APIs will advance, enhancing developer capabilities and shaping the future of software development. Embracing APIs opens up endless possibilities in the digital landscape.
Subscribe to my newsletter
Read articles from Annanya Sah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
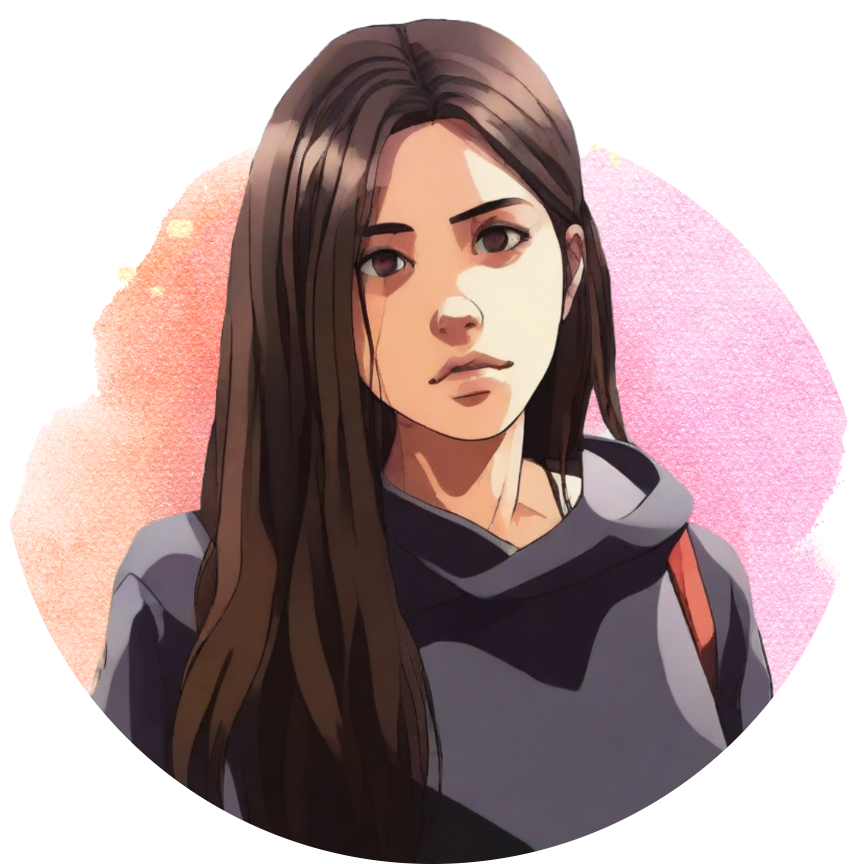
Annanya Sah
Annanya Sah
Passionate coder and avid blogger. Merging software engineering with creative writing. Brainstorming innovative solutions, writing aesthetic code, and turning deadlines into guidelines. Exploring philosophical musings and sharing experiences.