BulkWrite vs. Iterative Updates
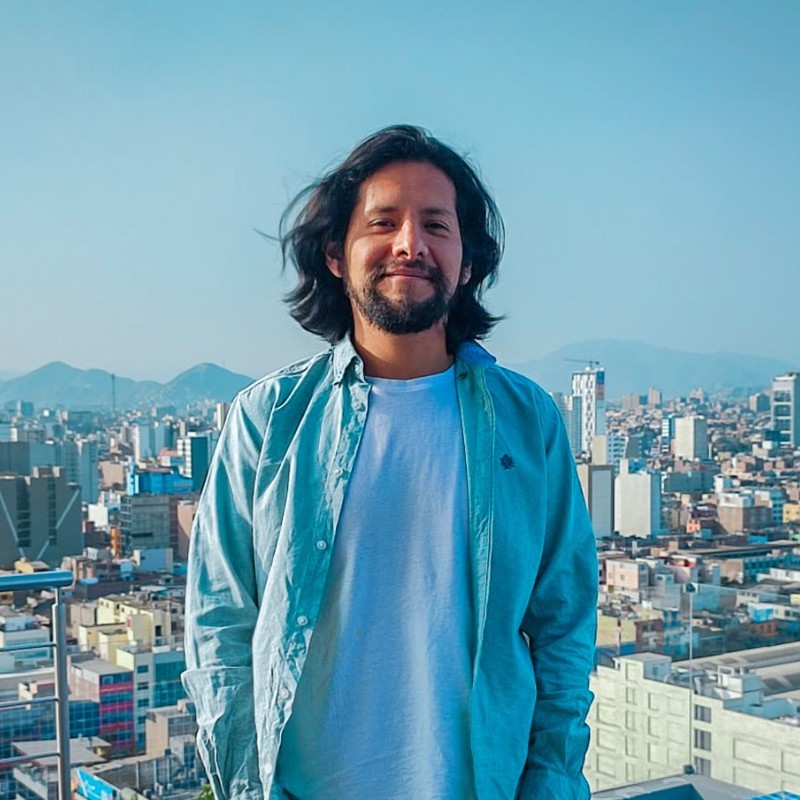
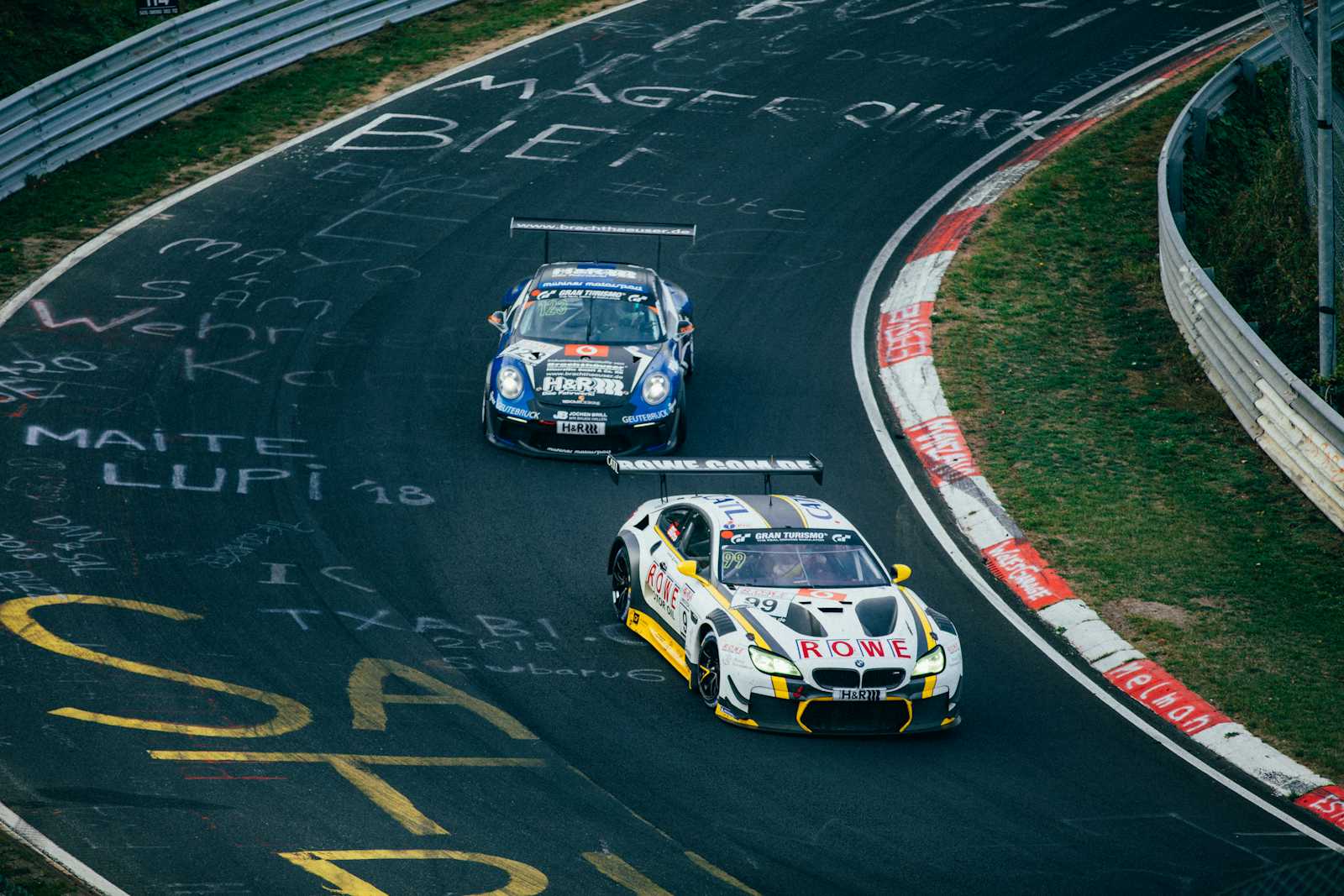
We often encounter scenarios where we need to update multiple documents in a MongoDB collection. One common approach is to iterate through the documents and execute individual update statements. However, MongoDB provides a more efficient alternative: the bulkWrite
function.
Let's delve into the reasons why bulkWrite
reigns supreme over iterative updates:
1. Performance Boost:
Reduced Network Overhead: Iterative updates involve multiple round trips to the database, each requiring a separate network request.
bulkWrite
, on the other hand, bundles all update operations into a single request, significantly reducing network latency.Optimized Database Operations: MongoDB can optimize the execution of bulk operations, processing multiple updates in a single transaction. This minimizes the overhead associated with individual update operations.
2. Improved Consistency:
- Atomic Updates:
bulkWrite
ensures that all update operations within a batch are executed atomically. This means that either all updates succeed or none of them do, guaranteeing data consistency. Iterative updates, however, can lead to inconsistencies if an update fails mid-process.
3. Enhanced Error Handling:
- Centralized Error Management:
bulkWrite
provides a single point of error handling. You can catch any errors that occur during the bulk operation and handle them accordingly. With iterative updates, you need to handle errors individually for each update, making error management more complex.
4. Simplified Code:
- Concise and Readable:
bulkWrite
offers a more concise and readable way to perform multiple updates. It eliminates the need for complex loops and error handling logic, making your code cleaner and easier to maintain.
Example:
Let's consider a scenario where we need to update the "tenancyName" field in multiple "posts" documents.
Iterative Approach:
content_copyaddcompare_arrowsopen_in_fullfor (const post of posts) {
await postsCollection.updateOne(
{ _id: post._id },
{ $set: { tenancyName: post.tenancyName } },
);
}
BulkWrite Approach:
content_copyaddcompare_arrowsopen_in_fullconst bulkOperations = posts.map((post) => ({
updateOne: {
filter: { _id: post._id },
update: { $set: { tenancyName: post.tenancyName } },
},
}));
await postsCollection.bulkWrite(bulkOperations);
As you can see, the bulkWrite
approach is significantly more concise and efficient.
Conclusion:
While iterative updates might seem intuitive, bulkWrite
offers a superior solution for updating multiple documents in MongoDB. It provides significant performance gains, improved consistency, enhanced error handling, and simplified code. Embrace the power of bulkWrite
and streamline your MongoDB update operations!
Subscribe to my newsletter
Read articles from Ernesto Yanac Huertas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
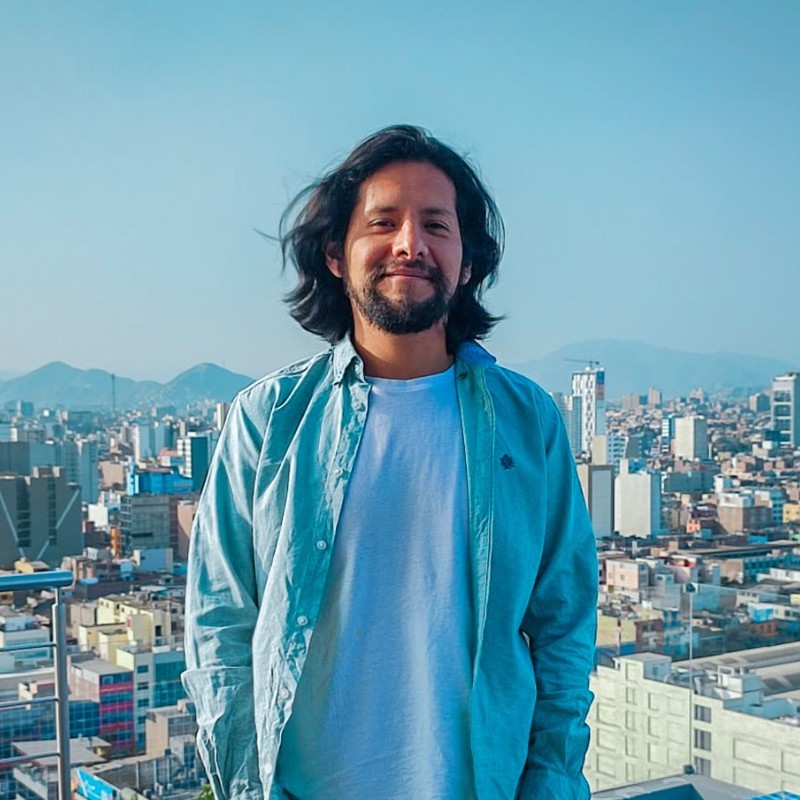
Ernesto Yanac Huertas
Ernesto Yanac Huertas
Developing web/mobile applications, from the server-side to the client-side. With 6 years of experience in software development. I am skilled in using a range of data mining language and technologies working as Big Data Engineer for 4 years.