How to Dockerize Node.js Applications A Step-by-Step Guide

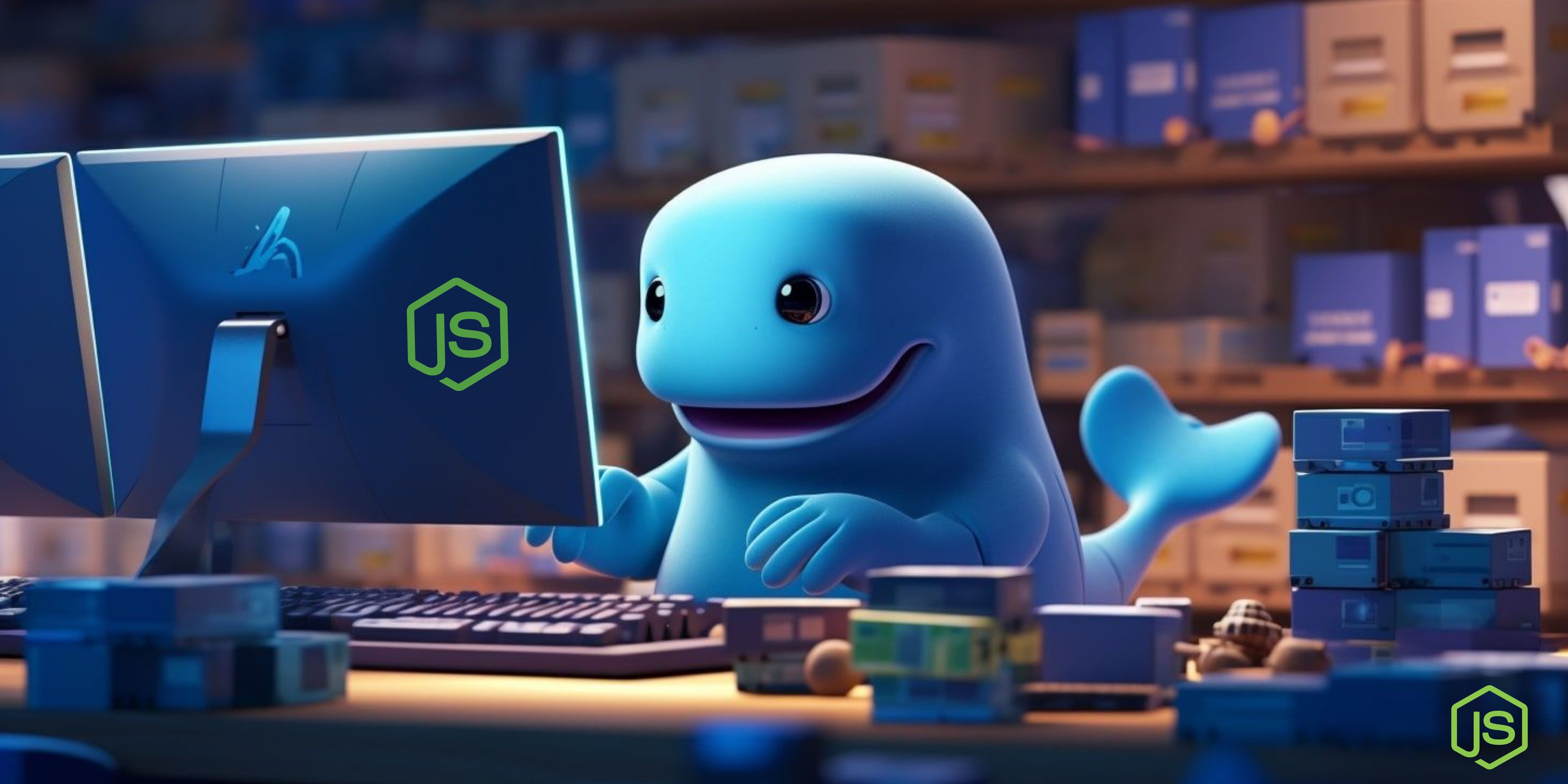
Docker containerization has transformed application development, offering consistency, scalability, and simplified dependency management. This guide provides a step-by-step approach to Dockerizing Node.js applications using npm.
What is Docker?
Docker is a platform that uses containers to run applications in isolated environments. Key components include Docker images, containers, and Dockerfiles. Containers are lightweight and share the host OS, making them more efficient than virtual machines.
Why Use Docker?
Consistency: Ensure the same environment across development, testing, and production.
Isolation: Secure and separate applications.
Scalability: Easily scale applications and manage dependencies.
Getting Started with Docker
Install Docker on your system from the official Docker website.
Basic Docker Commands:
docker run
: Run a container.docker ps
: List running containers.docker stop
: Stop a container.docker rm
: Remove a container.docker build
: Build an image from a Dockerfile.
Basic Docker Compose Commands:
docker-compose up
: Build, (re)create, start, and attach to containers.docker-compose down
: Stop and remove containers, networks, images, and volumes.docker-compose ps
: List containers.docker-compose stop
: Stop running containers.docker-compose rm
: Remove stopped service containers.docker-compose build
: Build or rebuild services.docker-compose logs
: View output from containers.
Dockerizing your Node.js Application
Step 1: Create .dockerignore
# See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
# dependencies
/node_modules
/.pnp
.pnp.js
# testing
/coverage
# production
/build
# misc
.DS_Store
.env
.env.local
.env.development.local
.env.test.local
.env.production.local
npm-debug.log*
yarn-debug.log*
yarn-error.log*
Step 2: Create a Dockerfile
: (if using yarn)
FROM node:20-alpine AS builder
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
FROM node:20-alpine
WORKDIR /app
COPY --from=builder /app/node_modules ./node_modules
COPY --from=builder /app ./
EXPOSE 8000
CMD ["yarn", "start"]
If using npm :
# Stage 1: Build the application
FROM node:20-alpine AS builder
WORKDIR /app
COPY package.json package-lock.json ./
RUN npm install
COPY . .
# Stage 2: Run the application
FROM node:20-alpine
WORKDIR /app
COPY --from=builder /app/node_modules ./node_modules
COPY --from=builder /app ./
EXPOSE 3000
CMD ["npm", "start"]
Step 3: Create a docker-compose.yml
file:
version: "3"
services:
easy-express: #keep the name accordingly your application
build:
dockerfile: Dockerfile
context: ./
container_name: easy-express #keep the name accordingly your application
ports:
- "8000:8000"
restart: always
volumes:
- .:/app
- /app/node_modules
env_file:
- .env
dns:
- 8.8.8.8
- 8.8.4.4
networks:
- app-network
networks:
app-network:
Step 4: Build and Run the Docker Container
docker-compose up --build
Step 5: List containers.
docker ps
Step 6: Stop and remove containers, networks, images, and volumes.
docker-compose down
Output :
Subscribe to my newsletter
Read articles from Ashim Rudra Paul directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ashim Rudra Paul
Ashim Rudra Paul
I am a Software Engineer at SJ Innovation with over 2 years of experience specializing in the MERN stack. My expertise spans TypeScript, Next.js, React.js, React Native, Express.js, Firebase, Supabase, MongoDB, PostgreSQL, and MySQL. I hold a degree in Computer Science and Engineering from Sylhet Polytechnic Institute and have earned certifications in MERN stack web development and JavaScript, C, C++, Python programming. Previously a Team Lead at elPixala, I excel in collaborating with product and design teams to create seamless user experiences. I prioritize rigorous testing and debugging to ensure high-quality performance. What sets me apart is my passion for coding and problem-solving. I thrive on crafting innovative solutions to complex challenges. Recently venturing into competitive programming on platforms like HackerRank and Codeforces has further sharpened my skills. Choose me for my proven track record of delivering dynamic web applications that combine functionality with visual appeal. My commitment to quality ensures top-notch results every time.