Functions in Dart: Best Practices and Examples

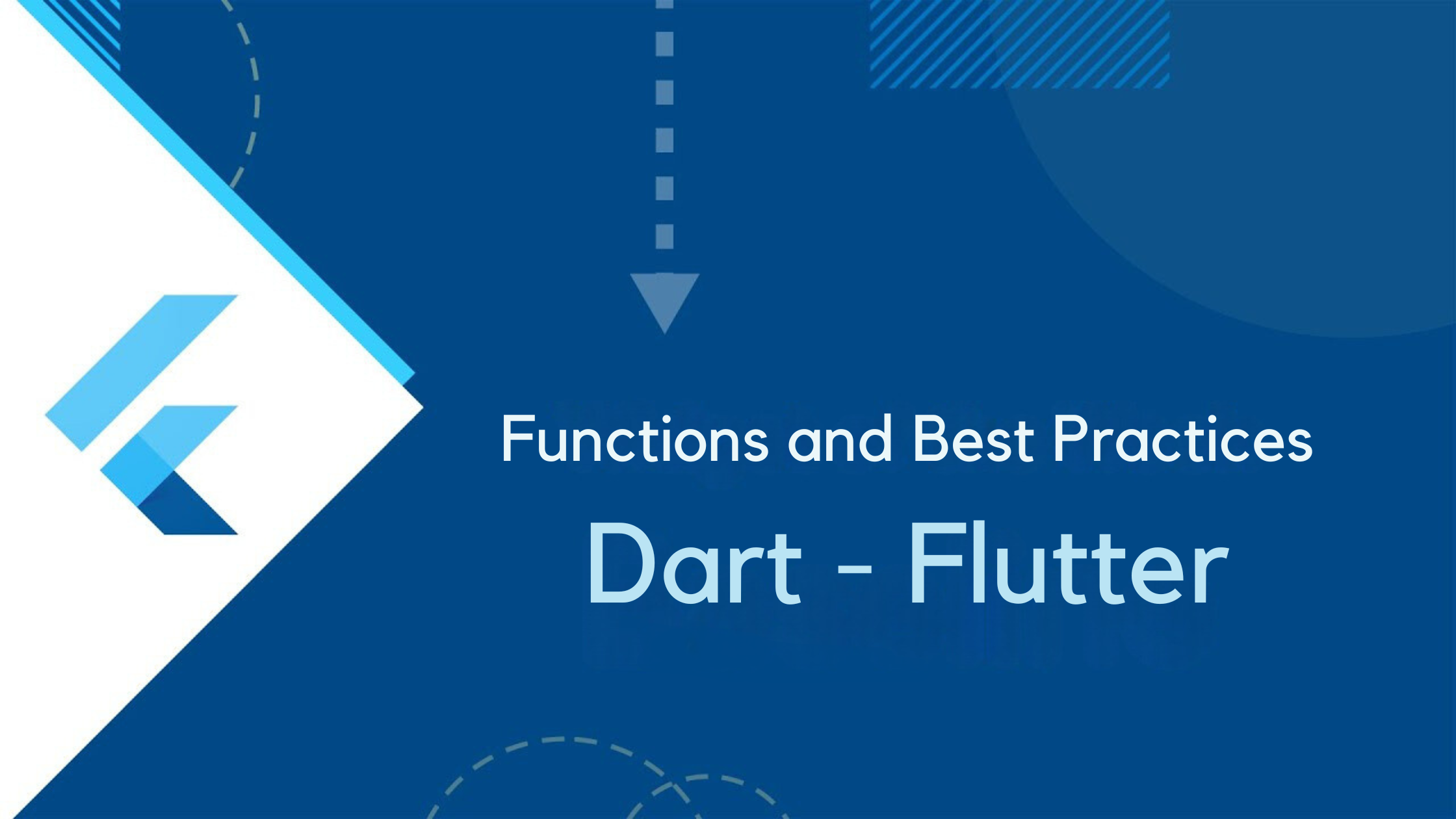
Whether you're just getting started or looking to refine your understanding, this blog is designed to provide you with a clear and detailed overview of Dart functions ๐. We'll explore the different types of functions, best practices, naming conventions, and much more. By the end of this blog, you'll be equipped with the knowledge to write clean, efficient, and maintainable functions in Dart.
What You'll Learn
Basic functions and their syntax
Different return types
Named and positional parameters
Private functions
Anonymous and arrow functions
Asynchronous functions
Using callbacks
Extensions and their utility
Best practices for writing functions
Let's dive in!
Basic Function
Think of a function as a helper. Just like in a kitchen, you have a recipe for making a cake. You follow the recipe's steps to make a delicious cake. Similarly, in programming, a function is a recipe for performing a task. ๐ฐ
void main() {
printHello();
}
void printHello() {
print('Hello, Dart!');
}
Function with Return Type
Functions can return values, making them more versatile. Imagine you ask someone to add two numbers for you and tell you the result. That's what a function with a return type does. ๐งฎ
void main() {
int result = add(3, 5);
print(result); // Output: 8
}
int add(int a, int b) {
return a + b;
}
Function with Named Parameters
Named parameters are like ordering a sandwich with specific ingredients. You explicitly mention what you want. This makes your function calls clear and flexible. ๐ฅช
void main() {
String fullName = getFullName(firstName: 'John', lastName: 'Doe');
print(fullName); // Output: John Doe
}
String getFullName({required String firstName, required String lastName}) {
return '$firstName $lastName';
}
Function with Positional Parameters
Positional parameters are like going to a fast-food restaurant and ordering items by their position on the menu. They are optional and have default values. ๐
void main() {
printGreeting('Hello');
printGreeting('Hello', 'John');
}
void printGreeting(String greeting, [String name = 'Guest']) {
print('$greeting, $name!');
}
Private Functions
Private functions are like secret recipes you keep to yourself. They're only accessible within the same library, ensuring that certain tasks remain internal. ๐คซ
void main() {
_privateFunction();
}
void _privateFunction() {
print('This is a private function.');
}
Anonymous Functions
Anonymous functions, or lambdas, are like writing a quick note without signing it. They're often used for short, one-off tasks. ๐
void main() {
List<String> names = ['John', 'Jane', 'Doe'];
names.forEach((name) {
print(name);
});
}
Arrow Functions
Arrow functions are a shorthand for writing simple functions. Think of them as writing "OK" instead of "Okay." They do the same job but are more concise. ๐ฏ
void main() {
int result = multiply(4, 5);
print(result); // Output: 20
}
int multiply(int a, int b) => a * b;
Asynchronous Functions
Asynchronous functions are like sending an email and waiting for a reply. You don't just sit there doing nothing; you continue with your work and check your email later. ๐ง
void main() async {
String data = await fetchData();
print(data); // Output: Data fetched!
}
Future<String> fetchData() async {
await Future.delayed(Duration(seconds: 2));
return 'Data fetched!';
}
Function with Callbacks
Callbacks are functions passed as arguments to other functions, commonly used for event handling. Imagine setting a timer and telling it to ring a bell when the time's up. โฒ๏ธ
void main() {
performTask(() {
print('Task complete!');
});
}
void performTask(Function callback) {
print('Performing task...');
callback();
}
Examples of Functions with Different Return Types
String Return Type
Here's a function that welcomes a user by returning a greeting message. ๐
void main() {
String message = welcomeMessage('Alice');
print(message); // Output: Welcome, Alice!
}
String welcomeMessage(String name) {
return 'Welcome, $name!';
}
List Return Type
A function that generates a list of numbers. Think of it as making a list of items you need from the grocery store. ๐
void main() {
List<int> numbers = generateNumbers(5);
print(numbers); // Output: [1, 2, 3, 4, 5]
}
List<int> generateNumbers(int count) {
return List<int>.generate(count, (index) => index + 1);
}
Map Return Type
A function that returns a map of names and their scores. It's like creating a scorecard for a game. ๐ฒ
void main() {
Map<String, int> scores = getScores();
print(scores); // Output: {Alice: 90, Bob: 85}
}
Map<String, int> getScores() {
return {'Alice': 90, 'Bob': 85};
}
Using Extensions with Functions
Extensions allow you to add new functionality to existing classes without modifying their source code. This can be especially useful for adding utility functions. ๐ ๏ธ
void main() {
String text = 'hello';
print(text.capitalize()); // Output: Hello
}
extension StringExtension on String {
String capitalize() {
return this[0].toUpperCase() + substring(1);
}
}
Best Practices for Using Functions in Dart
Use Descriptive Names: Function names should clearly describe their purpose. Just like naming your pets, make sure their names make sense! ๐ถ
void main() { int sum = add(5, 3); print(sum); // Output: 8 } int add(int a, int b) { return a + b; }
Keep Functions Small: Each function should do one thing and do it well. Like a single recipe for making cookies, not cookies and cake! ๐ช
void main() { int product = multiply(5, 3); print(product); // Output: 15 } int multiply(int a, int b) { return a * b; }
Use Named Parameters for Clarity: Named parameters make your function calls more understandable. It's like saying exactly what toppings you want on your pizza. ๐
void main() { String fullName = getFullName(firstName: 'John', lastName: 'Doe'); print(fullName); // Output: John Doe } String getFullName({required String firstName, required String lastName}) { return '$firstName $lastName'; }
Document Your Functions: Use comments to explain what your functions do, their parameters, and return types. Think of it as writing instructions for assembling furniture. ๐ ๏ธ
/// Adds two integers and returns the result. /// /// [a]: The first integer. /// [b]: The second integer. /// Returns the sum of [a] and [b]. int add(int a, int b) { return a + b; }
Handle Errors Gracefully: Ensure your functions handle errors and edge cases appropriately. Like making sure you have enough ingredients before starting to bake! ๐ฐ
int divide(int a, int b) { if (b == 0) { throw ArgumentError('Divider cannot be zero'); } return a ~/ b; }
Test Your Functions: Write unit tests to ensure your functions work as expected. It's like taste-testing your food before serving it. ๐จโ๐ณ
void main() { test('add function test', () { expect(add(2, 3), 5); }); }
Conclusion
Understanding and utilizing functions effectively is essential for any Dart developer. By following best practices and leveraging the flexibility of Dart functions, you can write cleaner, more maintainable code. Whether you're defining simple functions, working with asynchronous operations, using advanced features like named and positional parameters, incorporating callbacks, or extending existing classes, Dart provides the tools you need to build robust applications.
With this guide, you should have a solid foundation to explore the vast possibilities with Dart functions. Happy coding! ๐
References
DartPad - Interactive code examples
Subscribe to my newsletter
Read articles from Shankar Kakumani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
