Day 2 ReactJS : Quick and Simple Learning
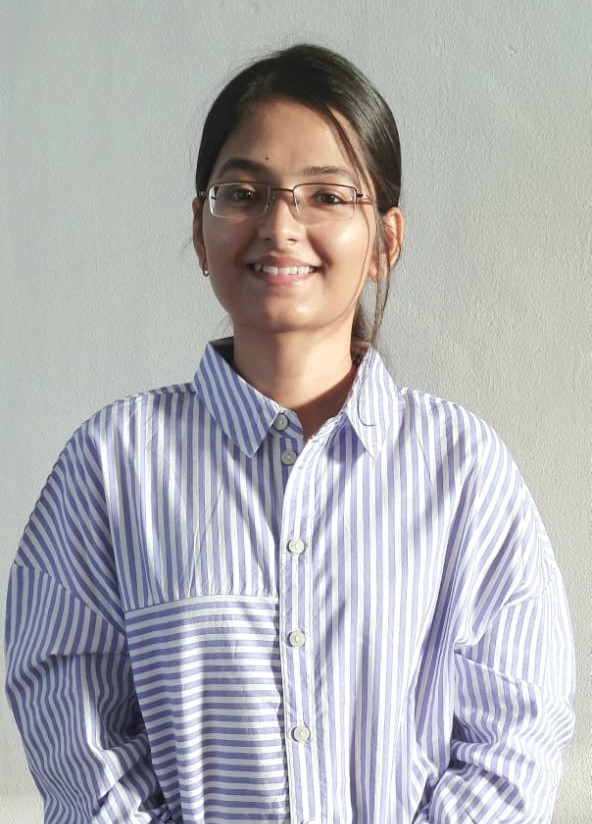
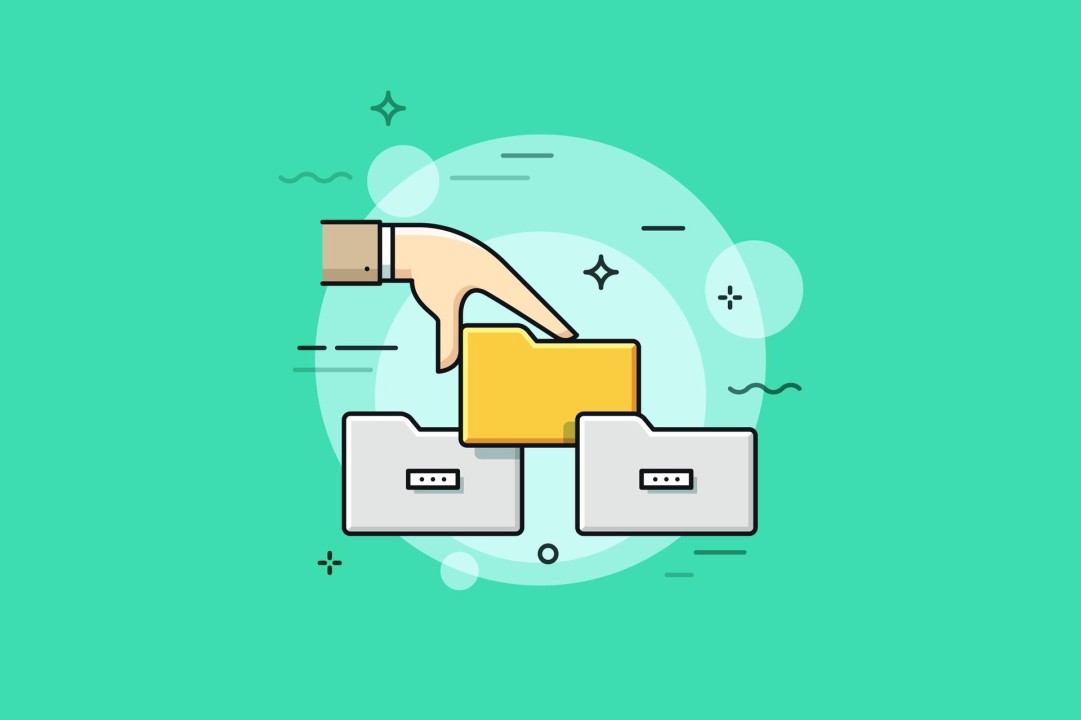
React is a powerful JavaScript library for building user interfaces (UIs) that are efficient, reusable, and maintainable. This Quick guide will equip you with the knowledge to create dynamic and interactive web applications using React.
Including React in Your Project
There are two primary ways to include React in your project:
- Using a CDN (Content Delivery Network):
This method is ideal for quick experimentation or small projects.
Include the React library directly from a CDN like unpkg:
<script src="https://unpkg.com/react@18/umd/react.production.min.js"></script> <script src="https://unpkg.com/react-dom@18/umd/react-dom.production.
- Using npm or yarn (Node Package Manager):
This method offers greater control over dependencies and version management for larger projects.
Install React and React DOM using npm or yarn: Bash
npm install react react-dom # or yarn add react react-dom # Run commands on terminal / CLI
Import React and ReactDOM in your JavaScript files:
// After previous commands add code to your file import React from 'react'; import ReactDOM from 'react-dom';
Creating a React Project with create-react-app (CRA)
For a streamlined development experience, consider using CRA:
Install CRA globally:
npm install -g create-react-app # or yarn global add create-react-app
Create a new React project:
npx create-react-app my-react-app # Run the commands on terminal
This command creates a new project directory with a pre-configured structure and essential dependencies.
Understanding ReactJS File and Directory Structure
CRA provides a well-organized file structure for your project. Here's a breakdown of key folders and files:
src
: This folder contains your application's source code.App.js
: The main component of your application.A functional component declared usingfunction.
App()
is typically exported usingexport default App;
so it can be imported and rendered fromindex.js
index.js
: The entry point for your React application. Renders the root component (you can see in above pic id="root"), react library, ReactDOM .App.css
(or other stylesheets): Styles for your components (can be organized further).Additional folders (optional): Organize components, API interactions, utilities, etc., as your project grows.
public
: This folder contains static assets that are directly accessible by the browser, likeindex.html
(the base HTML template for your app) andfavicon.ico
.package.json
: This file manages project dependencies and scripts.Dependancies: refer to external libraries or code modules that your project relies on to function properly.
TYPE & Examples -
a] Production Dependencies:
react
: The core React library for building components.react-dom
: For rendering React components into the browser DOM.react-router-dom
: To handle navigation between different pages in your single-page application.axios
(or a similar library): To make HTTP requests to your backend API for fetching product data, managing user accounts, or handling checkout processes.
b] Development Dependencies:
eslint
: For linting your code to catch potential errors and enforce code style.prettier
: To automatically format your code for consistency.jest
: A popular testing framework for unit testing your React components.react-dev-tools
: Browser extension for debugging your React application during development.
Other files (optional):
README.md
,.gitignore
, etc.
Writing Effective React Code
JSX (JavaScript XML): Learn JSX syntax for creating declarative templates that define your UI structure.
Component Lifecycle Methods: Understand how components mount (componentDidMount), update (componentDidUpdate), and unmount (componentWillUnmount) to manage their behavior.
State and Props: Utilize state to manage internal data and use props to receive data from parent components.
Event Handling: Handle user interactions with event listeners attached to DOM elements.
Beyond the Basics
Data Fetching: Consider libraries like
axios
orfetch
to make HTTP requests and manage API interactions.State Management: Explore options like Redux or Context API for managing complex application state.
Routing: For single-page applications, use libraries like React Router to handle navigation between views seamlessly.
Testing: Write unit and integration tests to ensure your components and application work as expected.
Learning Resources
Official React Documentation: https://react.dev/
React Community Resources: https://react.dev/community
Folder Structure: https://scrimba.com/articles/react-project-structure/
Conclusion
By understanding how to include React in your project, creating a React project with create-react-app, and familiarizing yourself with the file and directory structure, you lay a solid foundation for developing efficient and maintainable web applications.
Stay tuned for the next part, where we will delve into JSX and its rules!
Subscribe to my newsletter
Read articles from Sonal Diwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
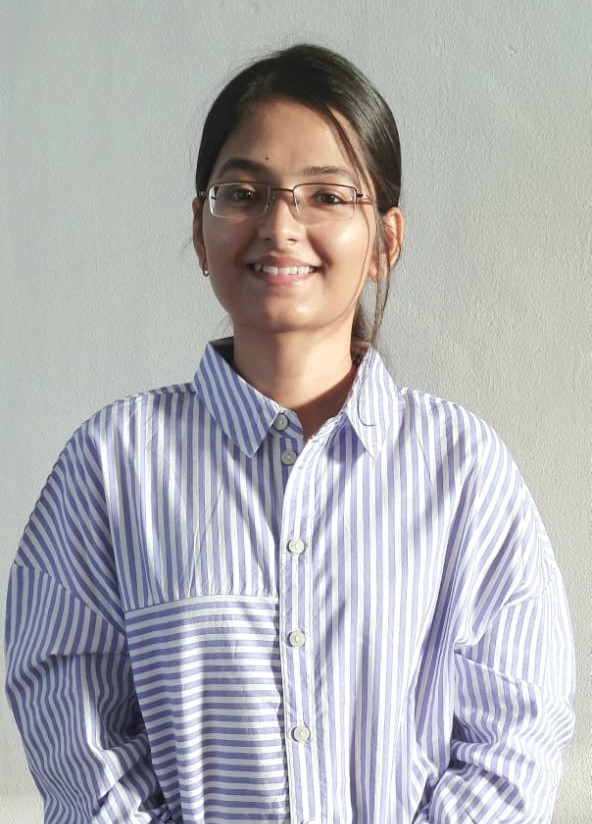
Sonal Diwan
Sonal Diwan
Data-Driven Fresher | Leveraging full stack technologies | Exploring tech-trends