A Rookie's Guide for REST Architecture and APIs

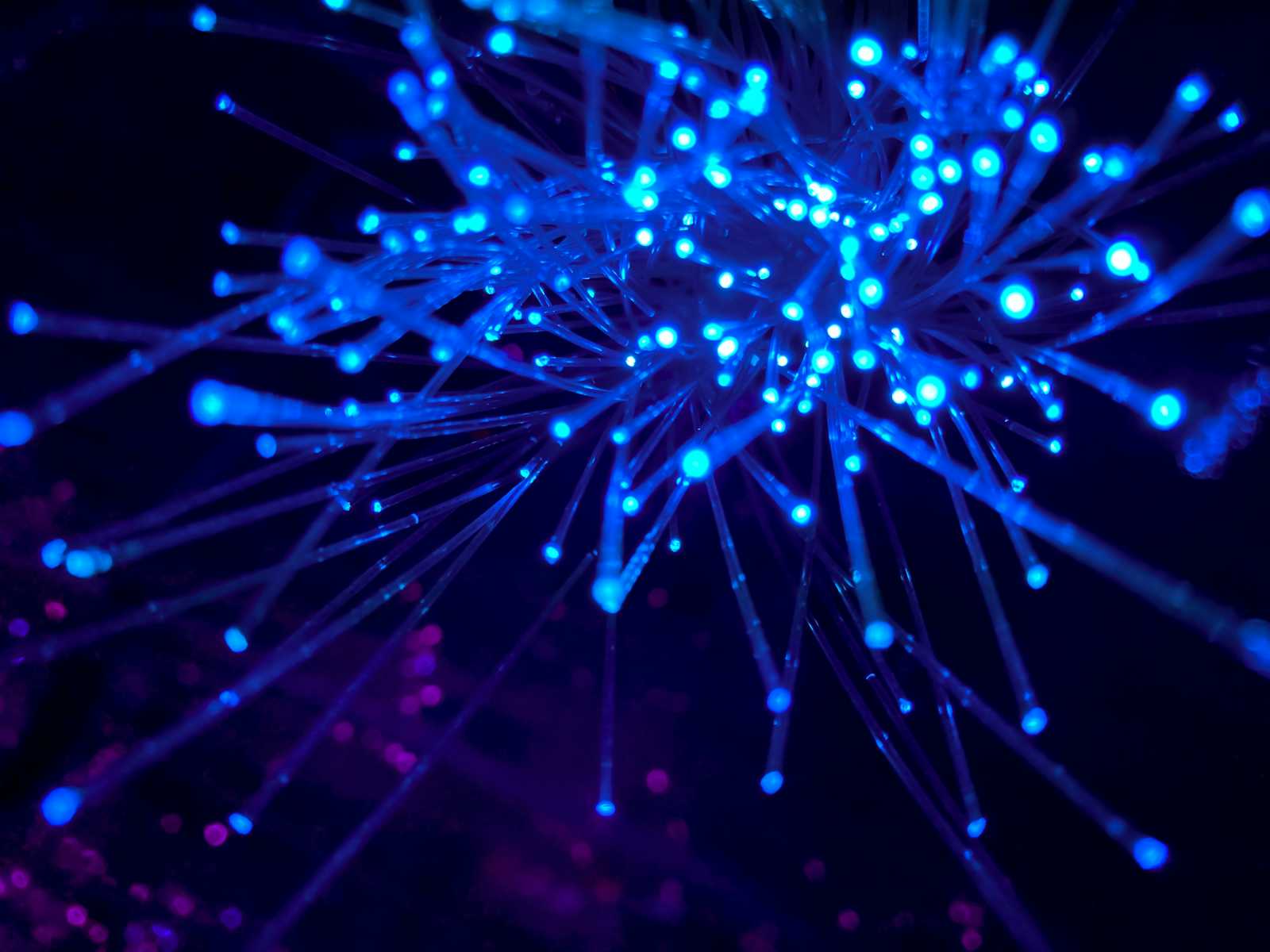
Firstly, REST stands for REpresentational State Transfer
Why should I be bothered about it at the first place?
According to a 2017 study,83 percent of APIs now use the REST architecture, while 15 percent use the older SOAP protocol.
It is of the most widely used approaches for building web-based APIs (Application Programming Interfaces).
Used in many popular APIs such as twilio, Stripe, Google Maps, etc.
Believe it or not, REST is not a protocol or a standard, it is an architectural style. During the development phase, API developers can implement REST in a variety of ways.
What is REST in one liner? Perhaps two!
REST is the web’s architecture. REST isn’t specifically about web services (i.e. machine-readable APIs that return JSON or XML).
It means when a RESTful API is called, the server will transfer to the client a representation of the state of the requested resource.
REST Constraints:
REST is based on some constraints and principles that promote simplicity, scalability, and statelessness in the design. The six guiding principles or constraints of the RESTful architecture are:
Uniform Interface
Simplifying system architecture and improving interaction clarity can be achieved through four constraints:
Resource Identification: Each resource has a unique identifier.
Manipulation via Representations: Resources are uniformly represented for state modification.
Self-Descriptive Messages: Messages contain processing information and next actions.
Hypermedia as Engine of Application State: Clients use the initial URI and hyperlinks for navigation and interactions.
Example: HTTP-based REST APIs use standard methods (GET, POST, PUT, DELETE) and URIs to consistently identify and interact with resources.
Client-Server Architecture:
The client application must entirely keep the session state.
The client-server design pattern enforces the separation of concerns, which helps the client and the server components evolve independently.
We improve the portability of the user interface across multiple platforms and improve scalability by simplifying the server components.
While the client and the server evolve, we have to make sure that the interface/contract between the client and the server does not break.
Statelessness:
The server doesn't remember previous interactions. Each request from the client includes all needed info. The server can store session data in a database for authentication.
When the client wants to change states, it sends a request. During this change, the client is "in transition." Each app state has links for future transitions.
Example: Think of a website where each page you visit is independent. Every time you visit a page, you provide your info. The site can store your data in a database for login purposes. Each page has links to other pages for navigation.
The server cannot take advantage of any previously stored context information on the server.
Cacheability:
On the web, both clients and intermediaries (like browsers or proxy servers) can store (cache) responses. Responses need to clearly indicate if they can be cached to avoid clients receiving outdated or wrong data. Proper caching can reduce the number of client-server interactions, enhancing performance and scalability.
Example: When you visit a website, your browser might save the page content . If you visit again soon, the browser can show the saved content instead of asking the server, making the site load faster.
Services for Cacheability: Services like Content Delivery Networks (CDNs), reverse proxies (e.g., Nginx, Varnish), and caching layers (e.g., Redis, Memcached) help manage caching. They store and serve cached content efficiently, reducing server load and improving response times
Layered System:
- In a layered system, a client doesn’t know if it's directly connected to the server or an intermediary like a proxy or load balancer.
This means adding or removing these intermediaries doesn’t require changes to client or server code. Intermediaries improve scalability through load balancing and shared caches.
Security can also be added as a separate layer, clearly separating business logic from security logic.
A server can call multiple other servers to generate a response for the client. Components in this system can only interact with the layer directly next to them.
Example: The MVC (Model-View-Controller) pattern is like a layered system. It separates different parts of an application, making it easier to develop, maintain, and scale.
Code on Demand (Optional):
In REST, there's an optional feature where servers can enhance a client's capabilities by sending executable code. This code could be in the form of Java applets or JavaScript scripts. By downloading and executing this code, clients can gain new functionalities without needing to implement them beforehand. This approach simplifies clients by offloading some processing tasks to the server, which dynamically provides and executes the necessary code as needed.
Example: Imagine a web application using REST. When you interact with certain features, the server may send JavaScript code to your browser. Your browser then runs this code, allowing you to perform specific tasks like form validation or interactive user interfaces, without these capabilities being built directly into the application beforehand.
A Quick Introduction to REST:
- Everything in REST is a resource -> an entitiy, allowing flexibility in how data is stored and served.
For e.g., If you are building a Cloth Merchandise Web Application, the potential resources are users, items to be sold, sellers, delievery partners, payement merchants, database for the transactions etc.
Representation of the entities is central to this idea.
It suggests how should entities communicate with each other, how can a client request and server response, maybe in a table format, or some string, some object. It defines a format.
Though storage representation does not matter*,* if you want to store data about users in one table, you definitely can, all the transcations can be stored in some documents (NoSQL) format.
The Resources can be composed of the following:
the data
the metadata describing the data
and the hypermedia links that can help the clients transition to the next desired state.
{ "id": 123, "title": "What is REST", "content": "REST is an architectural style for building web services...", "published_at": "2023-11-04T14:30:00Z", "author": { "id": 456, "name": "John Doe", "profile_url": "https://example.com/authors/456" }, "comments": { "count": 5, "comments_url": "https://example.com/posts/123/comments" }, "self": { "link": "https://example.com/posts/123" } }
Based on Client-Server Archirecture, i,e, the request-response model, the client ask (request) for some entity type and the server cater to that request by sending a dedicated response in a defined format/ represenation.
But what all about these representations? A REST implementation, a Client may demand for any of the multiple respresenation in any format that a server can support such as JSON, XML, CSV etc.
But in a more practical secenraio, you must have observed while working with any HTTP endpoints implemention in modern systems, we try to implement it in JSON format, but keep in mind REST does not restrict us.
Now, the external represenation for HTTP Server in the above diagram is JSON, but for internal represenation betwen Server and Database Storage, it can be in the form of tables, documents, etc.
Now, what can be do with this resource and representation?
- All the CRUD ( Create, Read, Update and Delete) operations can be performed on the resources with the help of HTTP verbs.
Though REST doesn't restrict us ( remember, REST is just a set of specification/rules) to a certain protocol, but the REST goes really well with HTTP, exisiting tooling, and infrastructure components enhances performance and simplifies development.
REST vs.HTTP
Many people prefer to compare HTTP with REST. REST and HTTP are not the same.
REST != HTTP
Though REST also intends to make the web (internet) more streamlined and standard. And that’s where people try to start comparing REST with the web, but REST does not favour any certain protocol.
HTTP is a contract, a communication protocol and REST is a concept. It is an architectural style which may use HTTP, FTP or other communication protocols but is widely used with HTTP.
How do we use HTTP in RESTful Apps?
We utilise the HTTP verbs and corresponding operations as the following:
HTTP verb | Operation |
POST | Create |
GET | Read |
PATCH | Update |
DELETE | Delete |
So, by looking at a partucular verb we can guess their well-defined meanings.
- So, for every endpoint, your action is identified by HTTP method i.e. a verb, and your resource is identified by URI ( Uniform Resource Identifier) i.e grouped by Nouns.
DELETE /item/100
-- We are deleting the resource of type "item" identified by 100
GET /user/1
-- To get details of user 1, instead of `/getStudent`
UPDATE /user/1
-- We can multiplex a particular endpoint without separate endpoint,
by just changing method
Why HTTP?
HTTP is most commonly used in REST API just because REST was inspired by WWW (world wide web) which largely used HTTP before REST was defined, so it’s easier to implement REST API style with HTTP.
We can leverage the existing HTTP Tooling and eco-system for RESTful Apps-
HTTP Clients: cURL, postman, Requests etc.
Web Cache: Ngix Cache, varnish, Ha Proxy
HTTP monitoring rate: tracing, packet snifling
Load Balancers: distribute load uniformly
Security Control: SSL
Compression
What are the downsides of using HTTP for RESTful specifications?
Consumption is not easy and repetitive.
HTTP payloads are huge e.g. JSON may not suit well for low-latency requirement.
You cannot switch protocols easily TCP -> UDP.
Some webservers may not support all the HTTP verbs.
Advantages of REST Architecture:
Advantages of REST APIs include:
Scalability: Easily scales with web traffic and diverse client needs.
Simplicity: Uses standard HTTP methods and URIs for straightforward interactions.
Flexibility: Supports various data formats and can be used across different platforms and devices.
Performance: Lightweight and efficient due to statelessness and caching support.
Interoperability: Allows integration with other systems and languages seamlessly.
Disadvantages of REST Architecture
- Lack of state: most web applications require stateful mechanisms. Suppose you purchase a website which has a mechanism to have a shopping cart. It is required to know the number of items in the shopping cart before the actual purchase is made. This burden of maintaining the state lies on the client, which makes the client application heavy and difficult to maintain.
- Last of security: REST doest impose security such as SOAP. That is the reason REST is appropriate for public URLs, but it is not good for confidential data passage between client and server.
Though most of the API in the main stream industry, use REST API, but for use cases we can refer to other alternatives to REST architecture:
GraphQL: Provides a flexible query language allowing clients to request specific data structures.
SOAP (Simple Object Access Protocol): Uses XML-based messaging for exchanging structured information between networked systems. It can useds in IOT devices.
gRPC: It is an open-source framework developed by Google for building RPC (Remote Procedural Calls) APIs. It allows developers to define service interfaces and generate client and server code in multiple programming languages. gRPC uses protocol buffers and a language-agnostic data serialization format for efficient data transfer, making it ideal for high-performance applications.
Websockets: The WebSocket protocol enables bi-directional, real-time communication between clients and servers. Unlike REST, which is request/response-based, WebSockets allow servers to push data to clients as soon as it becomes available, making it ideal for applications that require real-time updates, such as chat applications and online gaming.
Conclusion:
REST simplifies API development by reducing complexity and resource requirements. It efficiently manages resources with minimal operations. Today, REST dominates internet traffic, particularly in web development stacks like MERN, leveraging tools like Express and Node.js for building robust RESTful APIs.
Resources/Website Referenced for this Article:
https://en.wikipedia.org/wiki/REST#Architectural_constraints
https://www.visual-paradigm.com/guide/development/what-is-rest-api/
https://www.merge.dev/blog/understanding-the-difference-between-rpc-and-rest-for-web-apis
Book of AlexYu - System Design Interview
The images used in this article belong to their respective copyrighted companies. They have been utilized as they are available in the public domain. I do not claim ownership or rights over these images.
Subscribe to my newsletter
Read articles from Harsh Bansal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
