Mastering JavaScript Asynchronous Operations (Part-1)
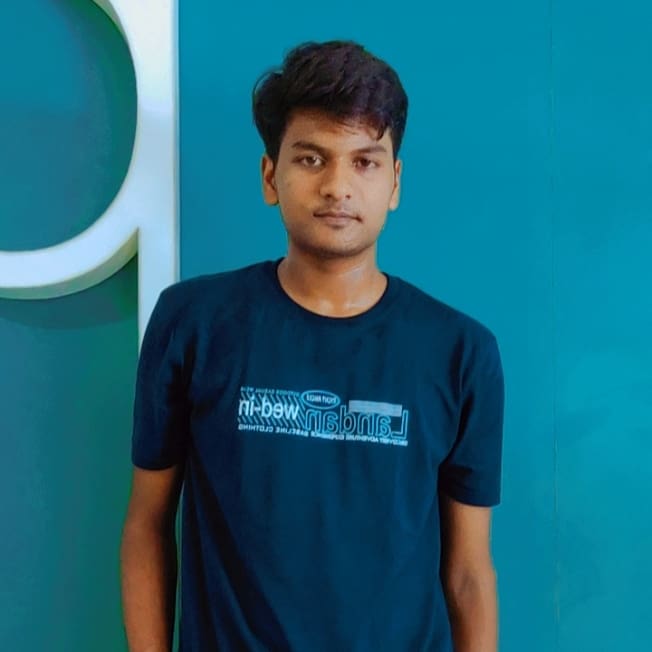
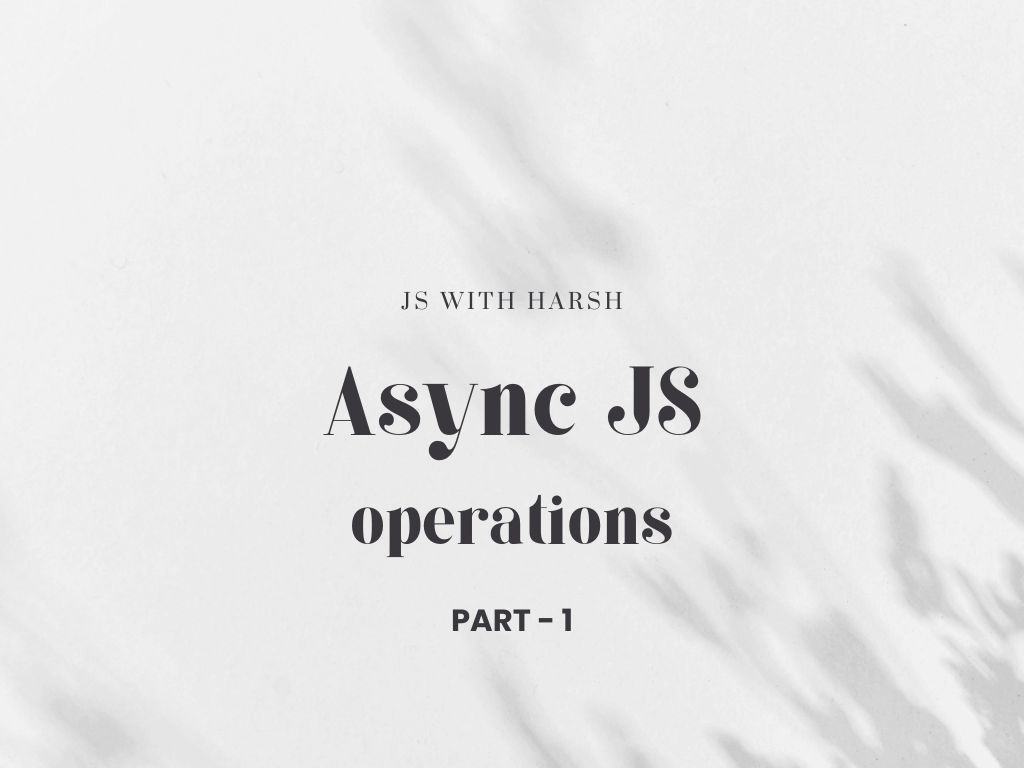
In this post, we will delve into the nuances of asynchronous JavaScript by exploring the setTimeout
and clearTimeout
functions. Understanding these concepts will enhance your ability to write efficient and non-blocking JavaScript code.
Setting Up Our HTML
First, let's set up a simple HTML structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Understanding async JS</h1>
<button id="stop">Stop</button>
</body>
<script src="script.js">
</script>
</html>
Introducing setTimeout
The setTimeout
function allows us to delay the execution of a function by a specified number of milliseconds. It is a method and this method also calls an API.
Also setTimeout() function/method sets a timer that executes a function or specified piece of code once the timer expires.
It takes 2 parameters, one is handler/callback function / or reference to a function and the other is time in milliseconds.
Here’s a basic example:
setTimeout(function(){
console.log("javascript");
}, 6000);
This code logs "javascript" to the console after a delay of 6 seconds.
Passing function references to setTimeout():
Instead of passing an anonymous function directly into the setTimeout
, we can pass a function reference. This makes our code cleaner and more maintainable.
const sayLangName = function() {
console.log("JS made easy");
};
setTimeout(sayHarsh, 7000);
In this example, the sayLangName
function will be executed after a delay of 7 seconds.
Changing Text Content with setTimeout
Let's change the text content of our h1
element after a delay:
document.querySelector('h1').innerHTML = "best js series";
//this will immediately change h1 text from "Unserstanding async JS" to "best js series"
const changeText = function() {
document.querySelector('h1').innerHTML = "best js series";
};
setTimeout(changeText, 4000);
This code changes the text of the h1
element to "best js series" after 4 seconds.
Stopping the Timeout with clearTimeout
What if we want to cancel the setTimeout
before it executes? This is where clearTimeout
comes into play.
const changeText = function() {
document.querySelector('h1').innerHTML = "best js series";
};
//passing the reference of the "changeText" function
const changeMe = setTimeout(changeText, 4000);
// Clear the timeout before it executes
clearTimeout(changeMe); //not a good way as clearTimeout() should be set on a particular event
//we can write clearTimeout() like this:
document.querySelector('#stop').addEventListener('click', function() {
clearTimeout(changeMe);
console.log("stopped");
});
n this example, the setTimeout
is set to change the h1
text after 4 seconds. However, the clearTimeout(changeMe)
call cancels it before it gets a chance to execute. Additionally, clicking the "Stop" button also stops the timeout.
Wrapping Up
Understanding setTimeout
and clearTimeout
is fundamental for managing asynchronous operations in JavaScript. These functions help you control the timing of your code execution, making your applications more responsive and efficient.
Subscribe to my newsletter
Read articles from Harsh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
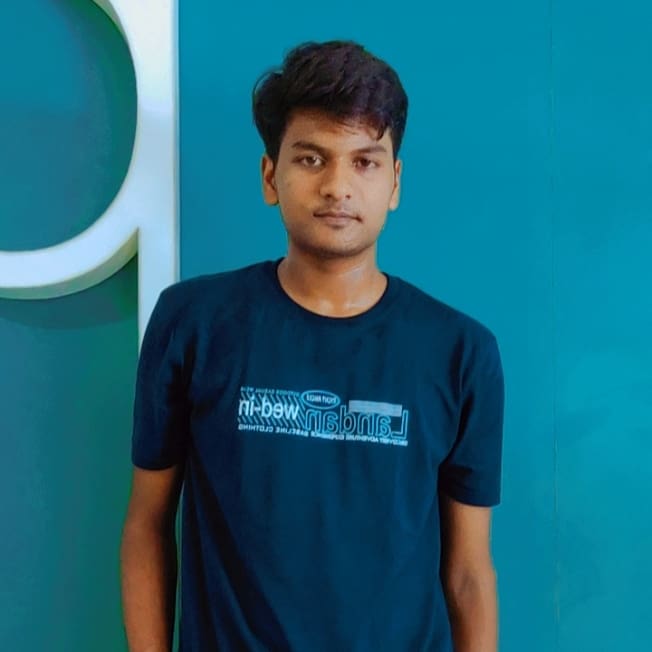
Harsh Gupta
Harsh Gupta
I am a CSE undergrad learning DevOps and sharing what I learn.