Chapter 3: Functions and Scope
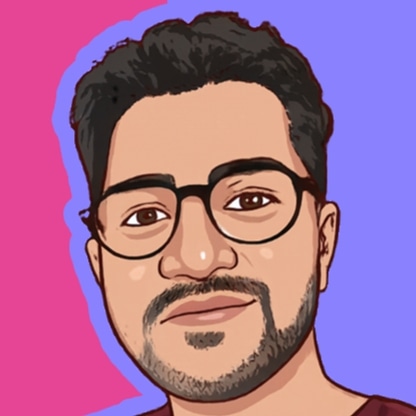
Welcome back to "Mastering JavaScript"! In Chapter 2, we explored the basics of JavaScript, including variables, data types, operators, and control flow. Now, let's dive into functions and scope, which are essential concepts for writing efficient and modular code in JavaScript.
3.1 Definition and Usage
Functions are blocks of code designed to perform a particular task. They help you break your code into smaller, reusable pieces. To define a function in JavaScript, you use the function
keyword followed by a name, parentheses ()
, and curly braces {}
.
// Function definition
function greet() {
console.log("Hello, World!");
}
// Function invocation
greet(); // Output: Hello, World!
3.2 Parameters and Arguments
Functions can take parameters, which are variables listed inside the parentheses in the function definition. When you call a function, you can pass arguments to it, which are the actual values you provide.
// Function with parameters
function greet(name) {
console.log("Hello, " + name + "!");
}
// Function call with arguments
greet("Alice"); // Output: Hello, Alice!
greet("Bob"); // Output: Hello, Bob!
3.3 Return Values
Functions can return values using the return
statement. This allows you to get a result from a function and use it elsewhere in your code.
// Function that returns a value
function add(a, b) {
return a + b;
}
let sum = add(5, 3);
console.log(sum); // Output: 8
3.4 Function Expressions and Arrow Functions
Function expressions store a function in a variable. This can be handy for passing functions as arguments to other functions or when you want to define a function conditionally.
// Function expression
const multiply = function(a, b) {
return a * b;
};
console.log(multiply(4, 5)); // Output: 20
Arrow functions provide a shorter syntax for writing function expressions. They are especially useful for inline functions.
// Arrow function
const divide = (a, b) => {
return a / b;
};
console.log(divide(10, 2)); // Output: 5
// Arrow function with implicit return
const subtract = (a, b) => a - b;
console.log(subtract(9, 3)); // Output: 6
3.5 Scope and Hoisting
Scope determines the accessibility of variables and functions in different parts of your code. JavaScript has two main types of scope: global scope and local scope.
Global scope: Variables declared outside any function are in the global scope and can be accessed from anywhere in the code.
Local scope: Variables declared inside a function are in the local scope and can only be accessed within that function.
let globalVar = "I'm global!";
function showScope() {
let localVar = "I'm local!";
console.log(globalVar); // Output: I'm global!
console.log(localVar); // Output: I'm local!
}
showScope();
console.log(globalVar); // Output: I'm global!
// console.log(localVar); // Error: localVar is not defined
Hoisting is JavaScript's behavior of moving declarations to the top of their scope before code execution. This means you can use variables and functions before they are declared in your code.
// Function hoisting
sayHello();
function sayHello() {
console.log("Hello!");
}
// Variable hoisting
console.log(hoistedVar); // Output: undefined
var hoistedVar = "This is hoisted";
console.log(hoistedVar); // Output: This is hoisted
It's important to note that let
and const
are not hoisted in the same way as var
. Using let
or const
before they are declared will result in a ReferenceError
.
console.log(letVar); // ReferenceError: Cannot access 'letVar' before initialization
let letVar = "This is let";
console.log(constVar); // ReferenceError: Cannot access 'constVar' before initialization
const constVar = "This is const";
In this chapter, we covered the basics of functions and scope in JavaScript. We learned how to define and use functions, pass parameters, return values, and the differences between function expressions and arrow functions. We also explored scope and hoisting, two crucial concepts for understanding how variables and functions behave in your code.
In the next chapter, we'll dive into objects and arrays, where you'll learn how to work with these essential data structures in JavaScript.
Feel free to try out the code examples and share your thoughts or questions in the comments.
Happy coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
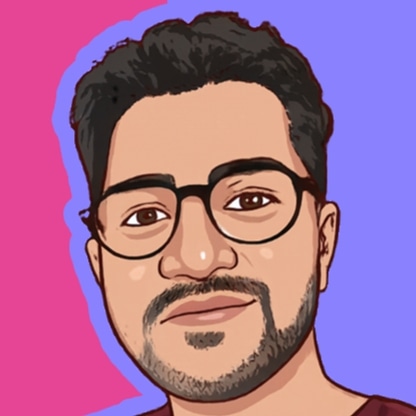
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code ๐ CS Grad at UTA | Full Stack Developer ๐ป | Problem Solver ๐ง