Chapter 8: Error Handling
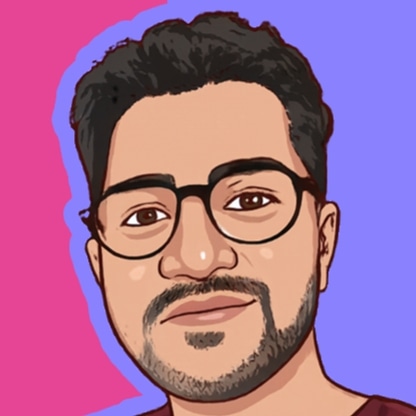
Welcome back to "Mastering JavaScript"! In the previous chapter, we explored JavaScript modules and how they can help you write cleaner, more organized code.
8.1 The Importance of Error Handling
Errors are inevitable in programming. They can occur due to various reasons, such as invalid user input, network failures, or logical mistakes in your code. Proper error handling ensures that your program can deal with these unexpected situations gracefully, without crashing or producing confusing results for users.
8.2 Types of Errors
JavaScript errors can be categorized into three main types:
Syntax Errors: Mistakes in the code syntax, such as missing parentheses or typos. These are usually caught by the JavaScript engine during compilation.
Runtime Errors: Errors that occur while the script is running, such as trying to access a property of
undefined
.Logical Errors: Errors in the logic of your code that cause it to behave incorrectly, such as incorrect calculations.
8.3 The try...catch
Statement
The try...catch
statement is used to handle runtime errors. Code that might throw an error is placed inside the try
block, and if an error occurs, it’s caught and handled in the catch
block.
Here's a basic example:
try {
let result = 10 / 0; // This will not cause an error, but let's assume something can go wrong here
console.log(result);
} catch (error) {
console.error("An error occurred: ", error.message);
}
In this example, if an error occurs inside the try
block, the catch
block executes, allowing you to handle the error and provide feedback to the user.
8.4 Throwing Errors
You can also create custom errors using the throw
statement. This is useful when you want to enforce certain conditions in your code and handle them explicitly.
function divide(a, b) {
if (b === 0) {
throw new Error("Division by zero is not allowed");
}
return a / b;
}
try {
console.log(divide(10, 2)); // Output: 5
console.log(divide(10, 0)); // This will throw an error
} catch (error) {
console.error("An error occurred: ", error.message);
}
In this example, if the divisor is zero, an error is thrown, and the catch
block handles it, preventing the application from crashing.
8.5 Practical Examples
Let’s see some practical examples of error handling in JavaScript.
Example 1: Handling Network Errors
async function fetchData(url) {
try {
let response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Failed to fetch data: ", error.message);
}
}
fetchData('https://jsonplaceholder.typicode.com/posts/1');
fetchData('https://invalidurl.com'); // This will throw an error
In this example, we handle potential network errors that can occur during a fetch request.
Example 2: Validating User Input
function getUserAge() {
let age = prompt("Enter your age:");
try {
if (isNaN(age)) {
throw new Error("Invalid input: age must be a number");
}
if (age < 0) {
throw new Error("Invalid input: age cannot be negative");
}
console.log(`Your age is ${age}`);
} catch (error) {
console.error("Error: ", error.message);
}
}
getUserAge();
In this example, we validate user input to ensure it is a positive number and handle any errors that arise.
In this chapter, we explored error handling in JavaScript, an essential skill for building robust applications. We covered:
The importance of error handling
Different types of errors
Using the
try...catch
statementThrowing custom errors
Practical examples of error handling
By incorporating proper error handling in your code, you can create applications that are more resilient and user-friendly.
In the next chapter, we'll dive into JavaScript's event-driven architecture, exploring how to handle events and build interactive web applications.
Happy coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
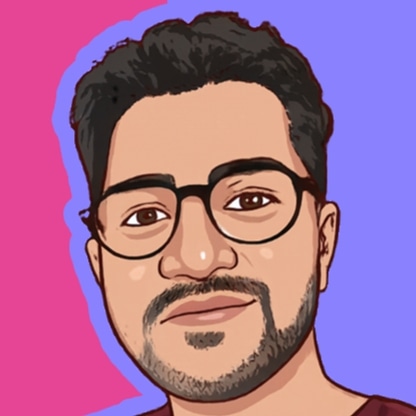
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠