Chapter 12: Advanced Concepts
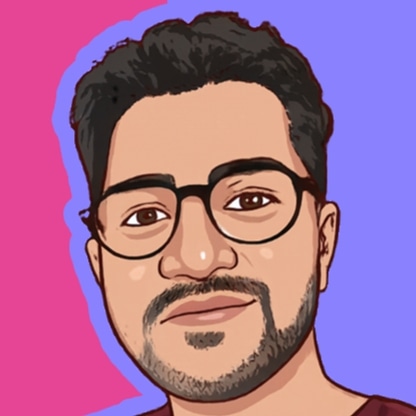
Welcome to the final chapter of our "Mastering JavaScript" series! In this chapter, we'll explore some advanced JavaScript concepts that will elevate your coding skills and deepen your understanding of this powerful language.
12.1 Closures
A closure is a function that retains access to its outer scope, even after the outer function has finished executing. This allows the inner function to access variables and parameters of the outer function.
Example:
function outerFunction() {
let outerVariable = 'I am from outer scope';
function innerFunction() {
console.log(outerVariable); // Can access outerVariable
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Output: I am from outer scope
Closures are useful for creating private variables and functions.
12.2 Promises and Async/Await
Promises are used to handle asynchronous operations in JavaScript. They represent a value that may be available now, in the future, or never.
Example:
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Promise fulfilled!');
}, 2000);
});
myPromise.then((message) => {
console.log(message); // Output after 2 seconds: Promise fulfilled!
}).catch((error) => {
console.log(error);
});
Async/Await is a syntactic sugar built on top of Promises, making asynchronous code look more like synchronous code.
Example:
async function fetchData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
12.3 The Event Loop
The Event Loop is a mechanism that allows JavaScript to perform non-blocking operations by offloading operations to the browser or Node.js APIs. It checks the call stack and the task queue, processing events and executing queued tasks.
Example:
console.log('Start');
setTimeout(() => {
console.log('Callback');
}, 0);
console.log('End');
// Output: Start, End, Callback
12.4 Higher-Order Functions
A higher-order function is a function that can take another function as an argument, or return a function as a result.
Example:
function higherOrderFunction(callback) {
return function (name) {
callback(name);
};
}
const greet = higherOrderFunction((name) => {
console.log(`Hello, ${name}!`);
});
greet('Alice'); // Output: Hello, Alice!
12.5 Currying
Currying is the process of transforming a function with multiple arguments into a sequence of functions, each taking a single argument.
Example:
function curryFunction(a) {
return function (b) {
return function (c) {
return a + b + c;
};
};
}
const result = curryFunction(1)(2)(3);
console.log(result); // Output: 6
12.6 Memoization
Memoization is an optimization technique used to speed up function calls by caching the results of expensive function calls.
Example:
function memoize(fn) {
const cache = {};
return function (...args) {
const key = JSON.stringify(args);
if (cache[key]) {
return cache[key];
}
const result = fn(...args);
cache[key] = result;
return result;
};
}
const factorial = memoize((n) => {
if (n === 0) {
return 1;
}
return n * factorial(n - 1);
});
console.log(factorial(5)); // Output: 120
Congratulations on completing the "Mastering JavaScript: From Fundamentals to Advanced Concepts" series! By now, you've covered a wide range of topics, from the basics to advanced concepts. Remember, mastering JavaScript is a journey, and continuous learning and practice are key.
In this chapter, we explored:
Closures
Promises and Async/Await
The Event Loop
Higher-Order Functions
Currying
Memoization
These advanced concepts are powerful tools that will help you write more efficient and sophisticated JavaScript code. As always, feel free to reach out with any questions or share your thoughts in the comments below.
Happy coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
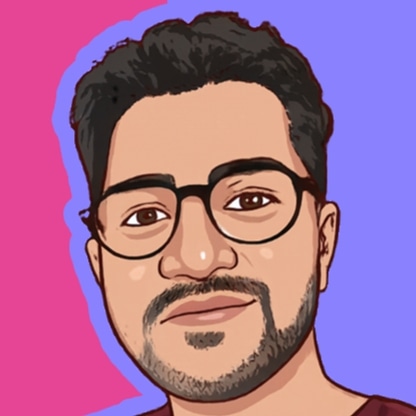
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code ๐ CS Grad at UTA | Full Stack Developer ๐ป | Problem Solver ๐ง