Data Structures for Beginners: Essential Concepts Simplified
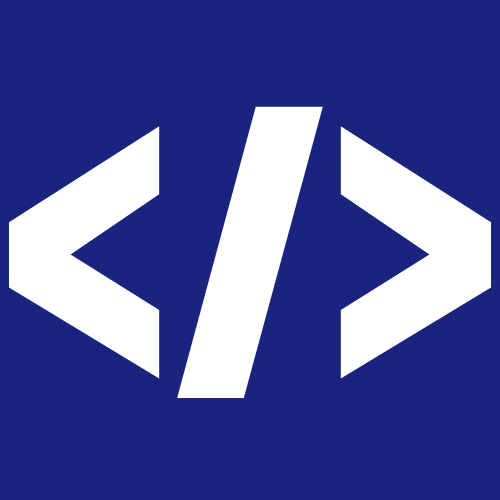
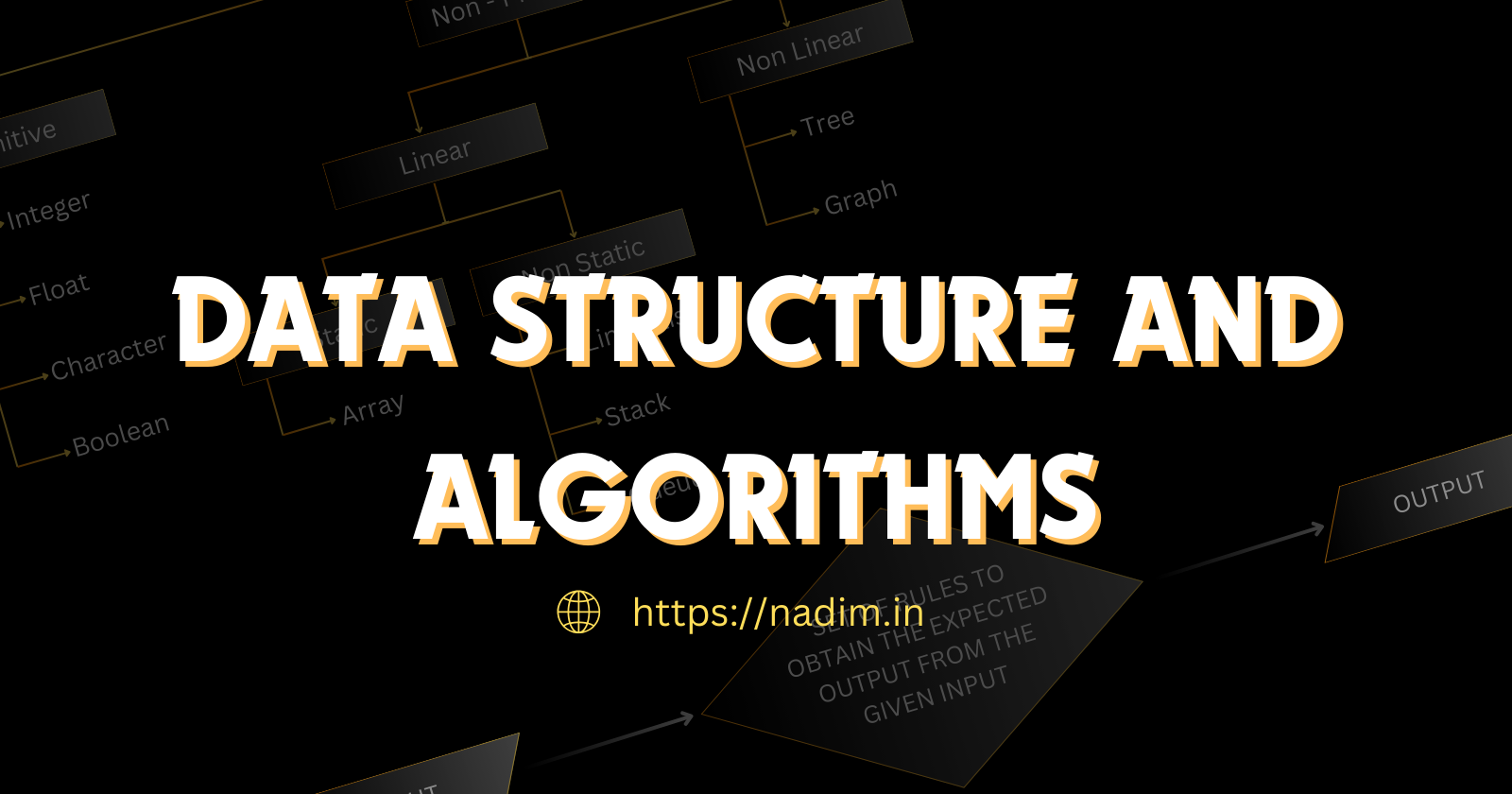
What is Data Structure?
A data structure is a way of organizing data so that it can be used effectively and efficiently. From a code design perspective, we need to pay particular attention to the way data is structured. If data isn’t stored properly, efficiently, or correctly structured, then the overall performance of the code will be reduced.
What is an Algorithm?
It is a set of instructions to perform a task.
Let's consider a scenario to understand the importance of data structure and algorithms.
Imagine we have a disorganized bunch of wood, and we want to choose a specific color, such as black. Since the wood is not organized, finding the desired color would be time-consuming as we would need to check each piece one by one.
Now, if we organize the wood based on color, finding any color of wood becomes easy. This is similar to what data structure does in applications. All applications deal with data and perform operations on that data. Before processing the data, it's organized in a way that makes the process efficient.
So, if we want to use organized wooden pieces for flooring, certain tasks need to be accomplished.
Types of data structure
Primitive: Data structures built into the programming language itself, available for the programmer.
Non-Primitive: Also known as user-defined data structures. They are created by combining two or more primitive data structures.
Linear: Items are arranged in memory in a linear, sequential manner. They can be either static or dynamic.
Static: Sizes and structures associated with memory location are fixed at compile time.
Dynamic: Also known as non-static, the memory location changes every compile time.
Non-Linear: Data is connected to several other items and is not organized sequentially.
Types of algorithms
Algorithms can be classified based on the problem they are trying to solve based on the working principle, there are eight different types of algorithms
Simple recursive algorithms
Backtracking Algorithms
Divide and Conquer Algorithms
Dynamic Programming Algorithms
Greedy Algorithms
Brach & Bound Algorithms
Brute Force Algorithms
Randomized Algorithms
What makes a good algorithm?
A good algorithm typically has the following characteristics:
Correctness: Make sure the algorithm solves the problem correctly. Test it with different inputs to see if it always produces the right output.
Efficiency: Try to write an algorithm that runs quickly and doesn't use too much memory. This means understanding basic concepts like time and space complexity, even at a high level.
Simplicity: Keep your algorithm simple. Avoid over-complicating your solution. Clear and simple code is easier to understand and debug.
Robustness: Your algorithm should be able to handle unexpected inputs without crashing. Think about edge cases and test them.
Scalability: As you get more comfortable, start thinking about how your algorithm performs with larger inputs. Does it slow down significantly? If so, you might need to find a more efficient approach.
Readability: Write your code in a way that others (and you in the future) can easily read and understand it. Use meaningful variable names, and add comments if needed.
Example: Find the Largest Among Three Numbers
Start
Declare variables
a
,b
, andc
.Read values for variables
a
,b
, andc
.Compare the values:
If
a
is greater thanb
:If
a
is also greater thanc
:- Display
a
is the largest number.
- Display
Else:
- Display
c
is the largest number.
- Display
Else:
If
b
is greater thanc
:- Display
b
is the largest number.
- Display
Else:
- Display
c
is the largest number.
- Display
Stop
Subscribe to my newsletter
Read articles from Nadim Anwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
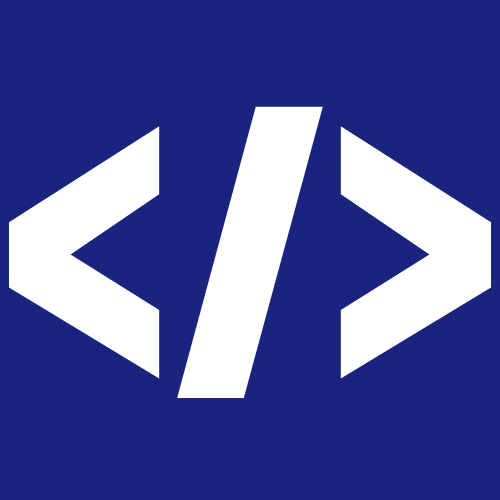
Nadim Anwar
Nadim Anwar
As an experienced front-end developer, I have expertise in building scalable and responsive web applications using modern technologies like ReactJS, Next.js, and TailwindCSS. With a solid understanding of JavaScript and a strong design sense, I excel at turning concepts into visually appealing and practical user interfaces. I am committed to continuously enhancing my skills and staying abreast of the latest industry trends to provide cutting-edge solutions.