Go Struct
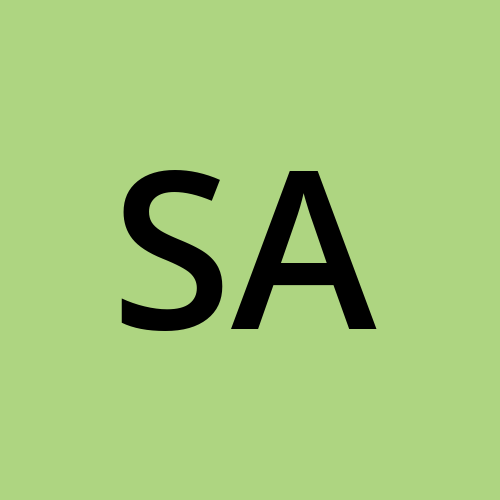
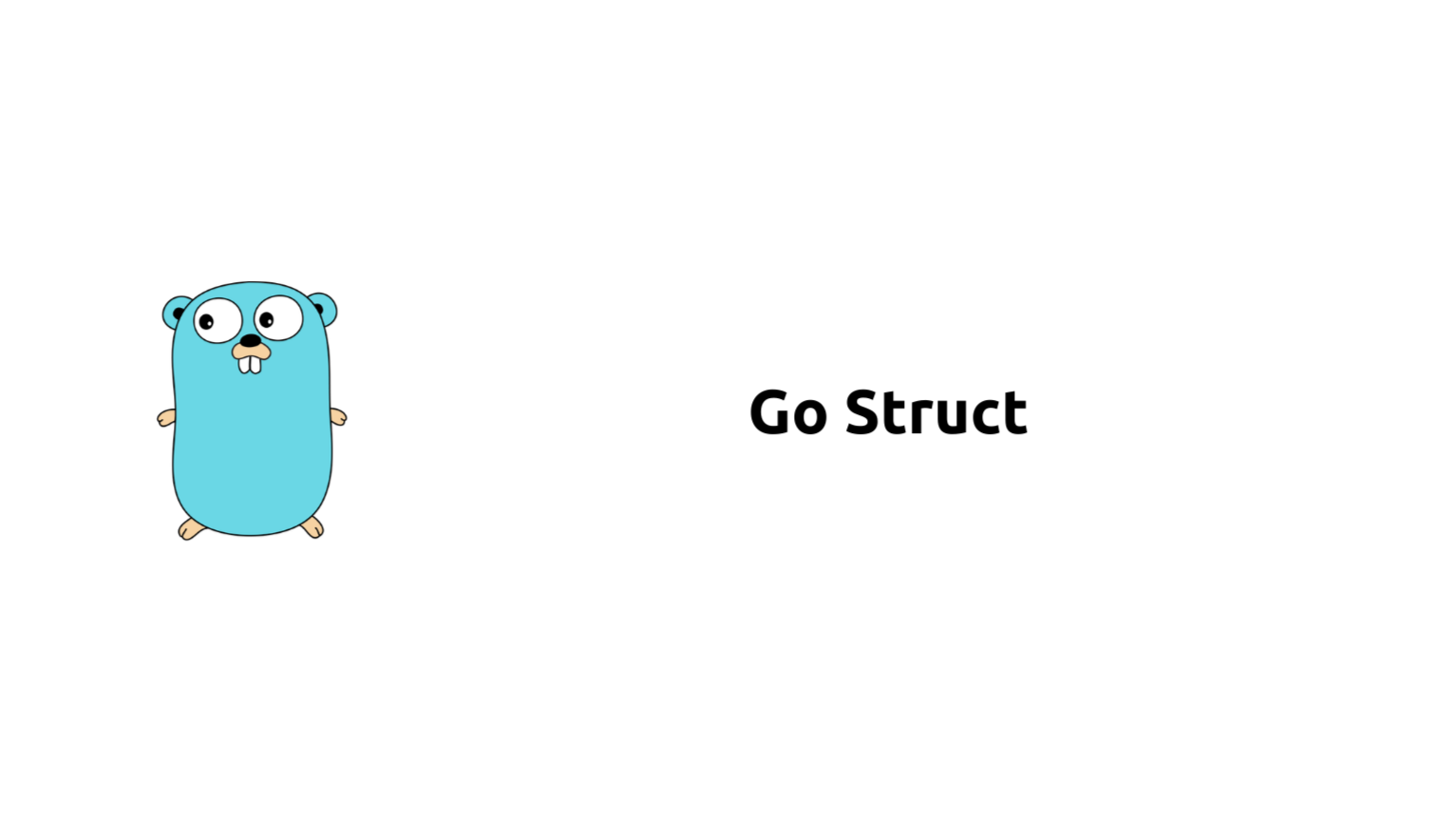
Introduction
Structures, often referred to as structs, are a composite data type in Go that allow you to group together variables of different types into a single entity. Unlike arrays, which store multiple values of the same data type, structs enable you to create custom data types with a mixture of different data types.
Declaring a Struct
To declare a struct in Go, you use the type
and struct
keywords followed by the struct's name and its member variables enclosed in curly braces.
type Car struct {
brand string
model string
year int
price float64
}
In this example, we define a struct named Car
with four member variables: brand
, model
, year
, and price
.
Accessing Struct Members
You can access individual members of a struct using the dot (.
) operator followed by the member name. Here's how you can declare a struct variable and access its members:
package main
import "fmt"
type Car struct {
brand string
model string
year int
price float64
}
func main() {
var myCar Car
myCar.brand = "Toyota"
myCar.model = "Camry"
myCar.year = 2020
myCar.price = 25000.50
fmt.Println("Brand:", myCar.brand)
fmt.Println("Model:", myCar.model)
fmt.Println("Year:", myCar.year)
fmt.Println("Price:", myCar.price)
}
Output:
Brand: Toyota
Model: Camry
Year: 2020
Price: 25000.5
Passing Structs as Function Arguments
You can pass structs as function arguments, allowing you to work with structured data within functions. Here's an example:
package main
import "fmt"
type Car struct {
brand string
model string
year int
price float64
}
func main() {
myCar := Car{"Honda", "Civic", 2018, 20000.75}
printCarDetails(myCar)
}
func printCarDetails(c Car) {
fmt.Println("Brand:", c.brand)
fmt.Println("Model:", c.model)
fmt.Println("Year:", c.year)
fmt.Println("Price:", c.price)
}
Output:
Brand: Honda
Model: Civic
Year: 2018
Price: 20000.75
Conclusion
Structs are a powerful feature in Go that allow you to create custom data types to represent complex entities. They provide a convenient way to encapsulate related data and operations into a single unit. By understanding how to declare and use structs, you can write more organized and maintainable code in your Go programs. Experiment with different struct designs to model your data effectively and efficiently.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
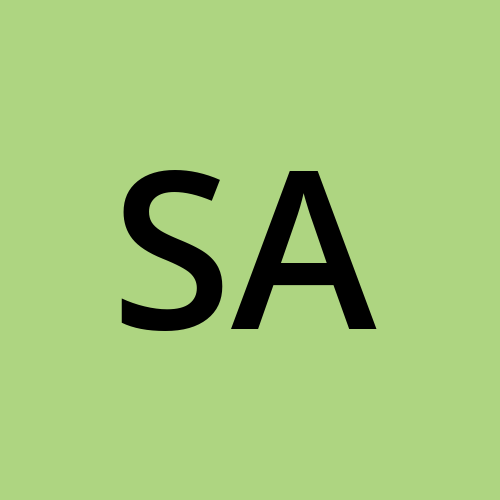
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.