Understanding Scoping in JavaScript : A Developer's Guide
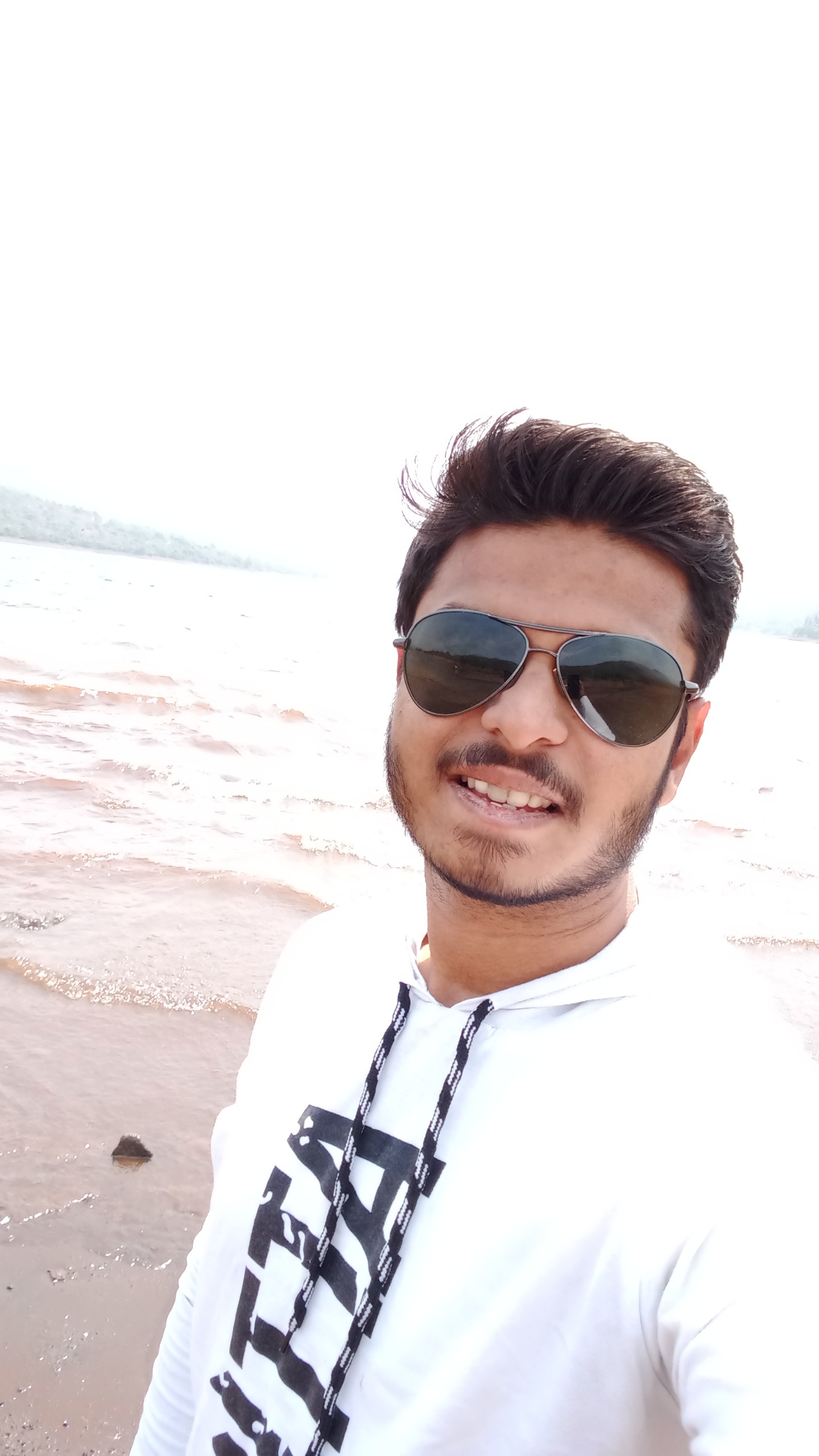
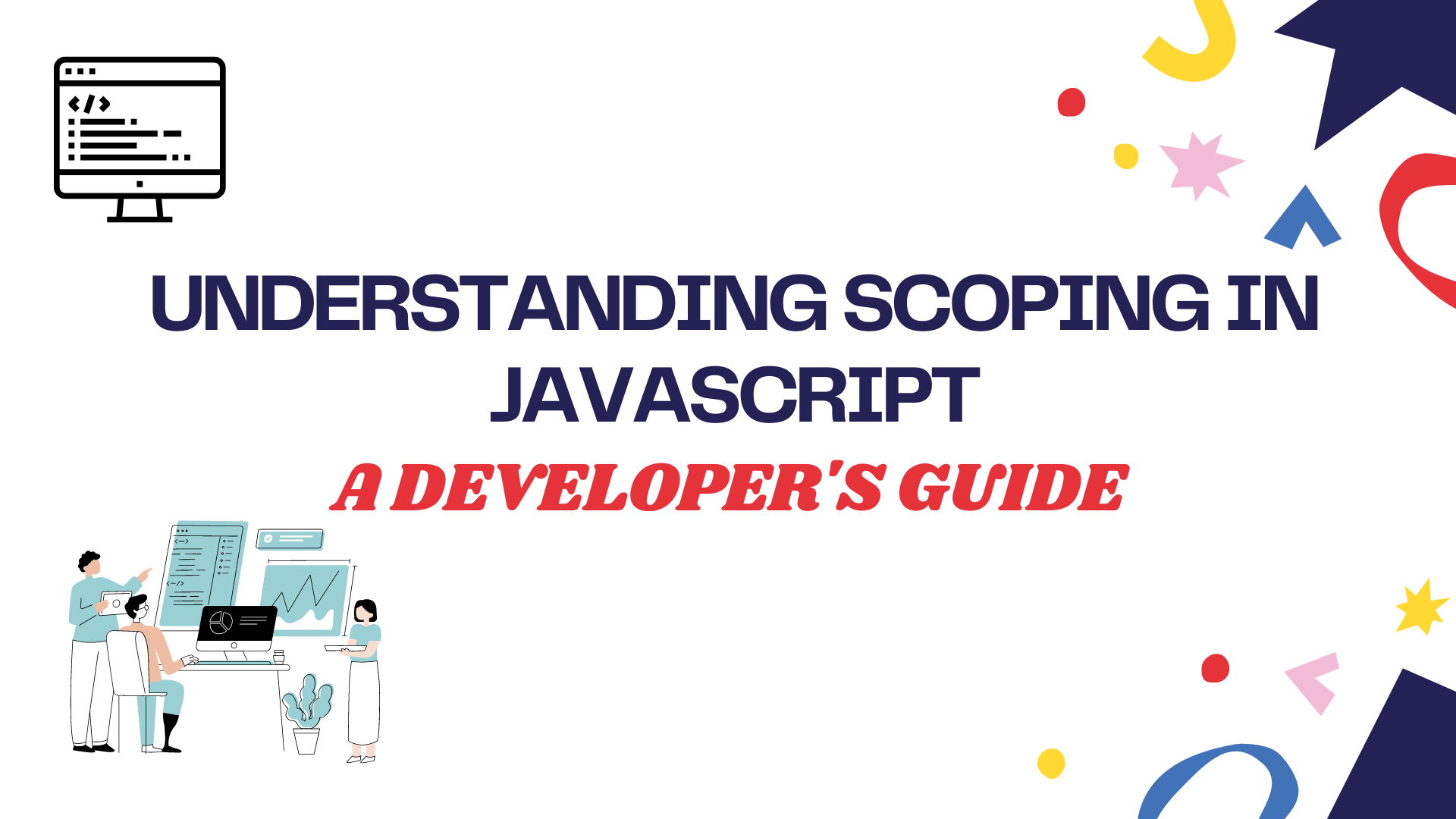
JavaScript is a powerful and versatile language that relies heavily on the concepts of scoping. Understanding these concepts is essential for writing efficient and bug-free code. In this blog post, we'll explore what scoping mean in JavaScript, how it works, and why it is crucial for developers.
What is Scope?
In JavaScript, scope refers to the current context of execution, which determines the accessibility of variables and functions. There are two main types of scope in JavaScript:
Global Scope
Local Scope
Global Scope
Variables declared outside any function or block are in the global scope. They can be accessed from anywhere in your code.
let globalVar = 'I am a global variable';
function globalScopeExample() {
console.log(globalVar); // I am a global variable
}
globalScopeExample();
console.log(globalVar); // I am a global variable
Local Scope
Variables declared within a function are in the local scope. They can only be accessed within that function.
function localScopeExample() {
let localVar = 'I am a local variable';
console.log(localVar); // I am a local variable
}
localScopeExample();
console.log(localVar); // ReferenceError: localVar is not defined
Lexical Scope
Lexical scope, also known as static scope, refers to the scope defined at the time of writing the code. In JavaScript, functions are lexically scoped, meaning they remember the scope in which they were defined, not where they are called.
To understand lexical scope better, let's consider a nested function example:
function outerFunction() {
let outerVar = 'I am from outer function';
function innerFunction() {
console.log(outerVar); // I am from outer function
}
innerFunction();
}
outerFunction();
In the example above, innerFunction can access outerVar because of lexical scope. It remembers the environment where it was defined.
Closures and Lexical Scope
Closures are a fundamental concept that leverage lexical scope. A closure is created when a function is defined within another function, and the inner function continues to have access to the outer function's variables even after the outer function has executed.
function closureExample() {
let closureVar = 'I am a closure variable';
return function() {
console.log(closureVar);
};
}
const myClosure = closureExample();
myClosure(); // I am a closure variable
In this example, myClosure retains access to closureVar even after closureExample has finished executing, demonstrating the power of closures and lexical scope.
Block Scope
With the introduction of let and const in ES6, JavaScript now supports block-level scope. Variables declared with let and const are only accessible within the block they are defined in.
{
let blockVar = 'I am a block-scoped variable';
const blockConst = 'I am also block-scoped';
console.log(blockVar); // I am a block-scoped variable
console.log(blockConst); // I am also block-scoped
}
console.log(blockVar); // ReferenceError: blockVar is not defined
console.log(blockConst); // ReferenceError: blockConst is not defined
Block scope is especially useful in loops and conditionals to avoid variable hoisting and potential bugs.
Conclusion
Understanding scoping and lexical scope is crucial for mastering JavaScript. They define how variables and functions are accessed and managed, ensuring code clarity and avoiding unexpected behaviors. By leveraging these concepts, you can write more efficient and maintainable code.
Remember to consider the scope of your variables carefully, use let and const for block scoping, and embrace the power of closures to create robust and encapsulated functions.
Subscribe to my newsletter
Read articles from Shreeyog Gaikwad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
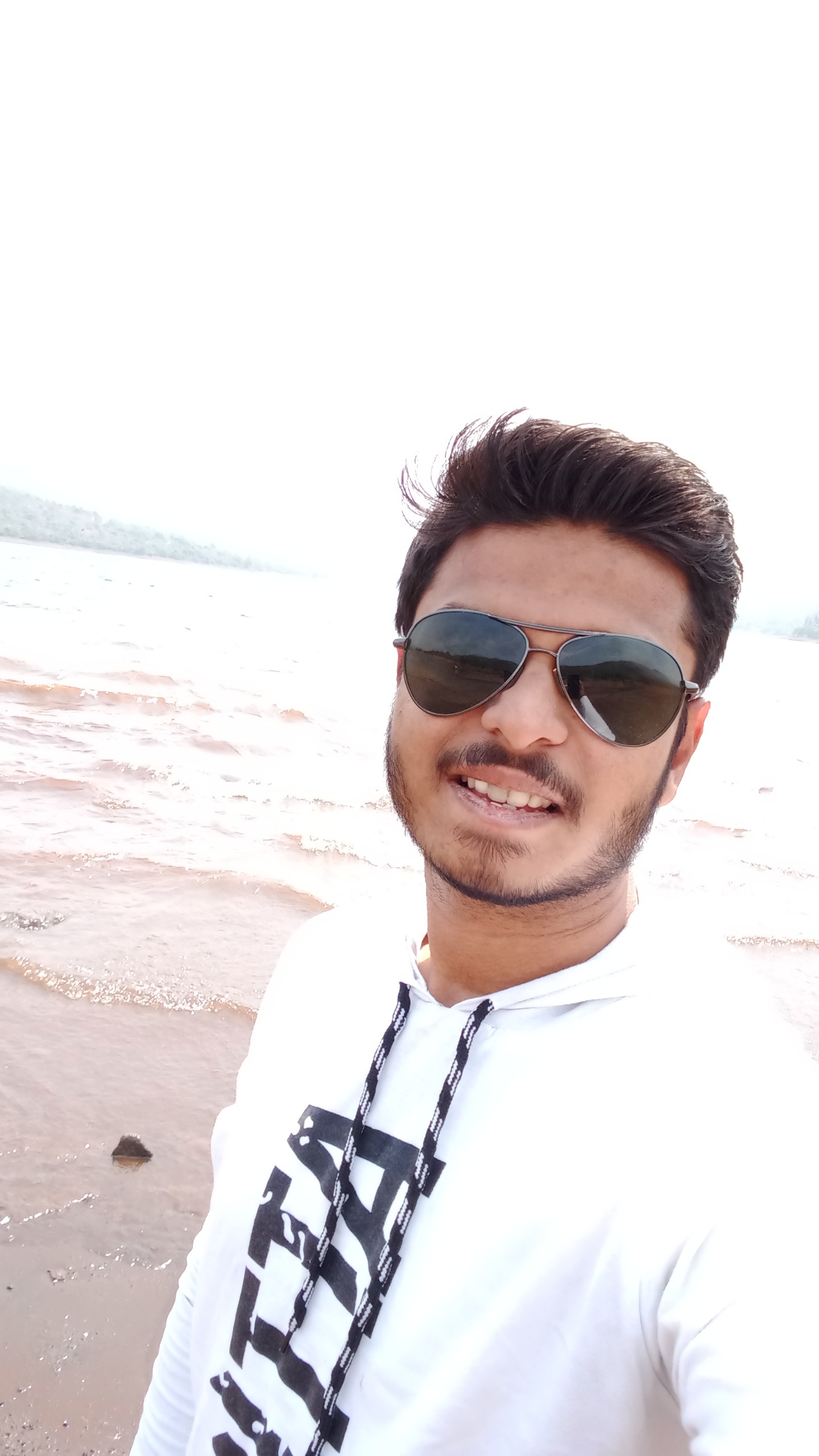
Shreeyog Gaikwad
Shreeyog Gaikwad
Passionate web developer with expertise in React, JavaScript and Node.js. Always eager to learn and share knowledge about modern web technologies. Join me on my journey of coding adventures and innovative web solutions!