Boost App Quality: A Deep Dive into Models, Entities, DTOs, and View Models
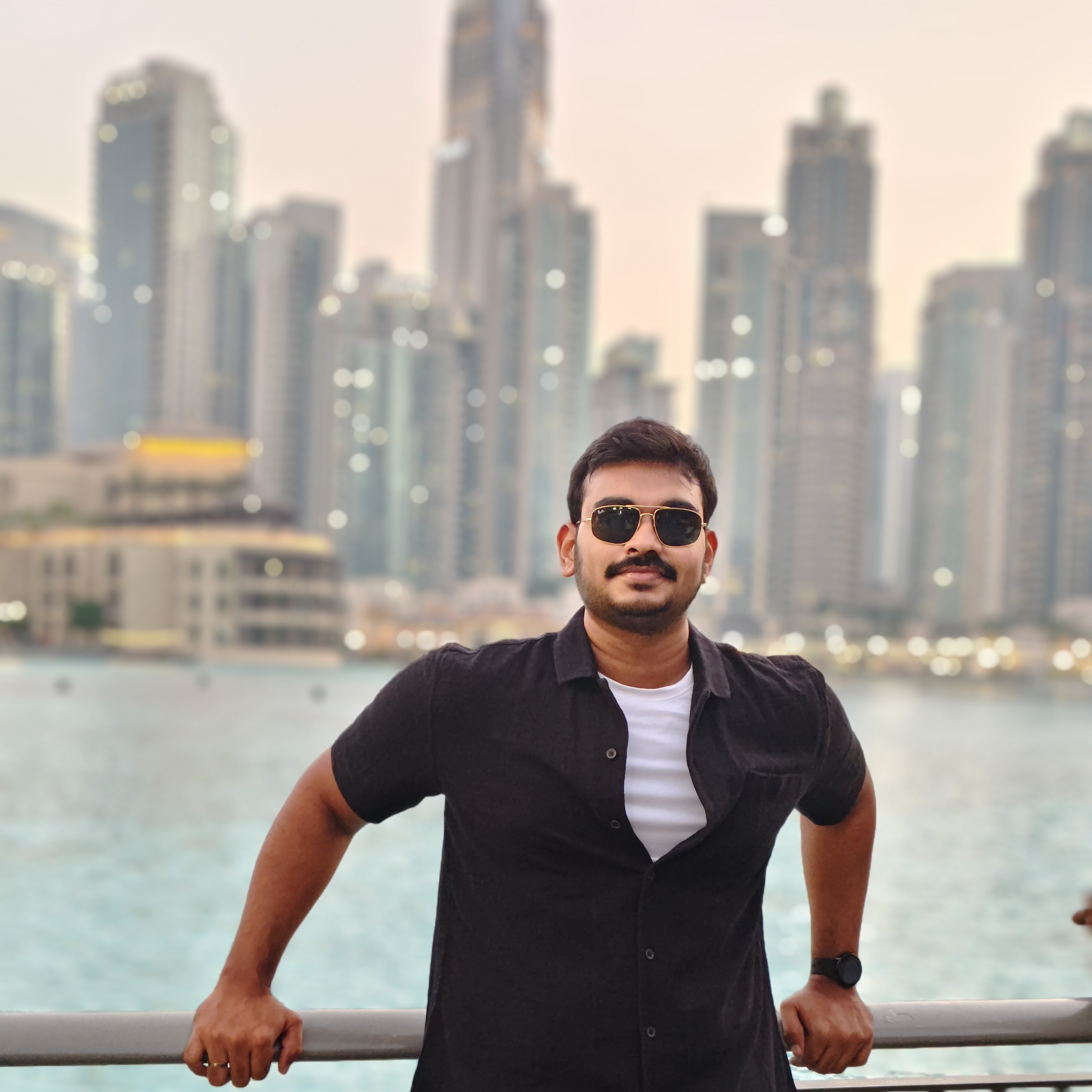
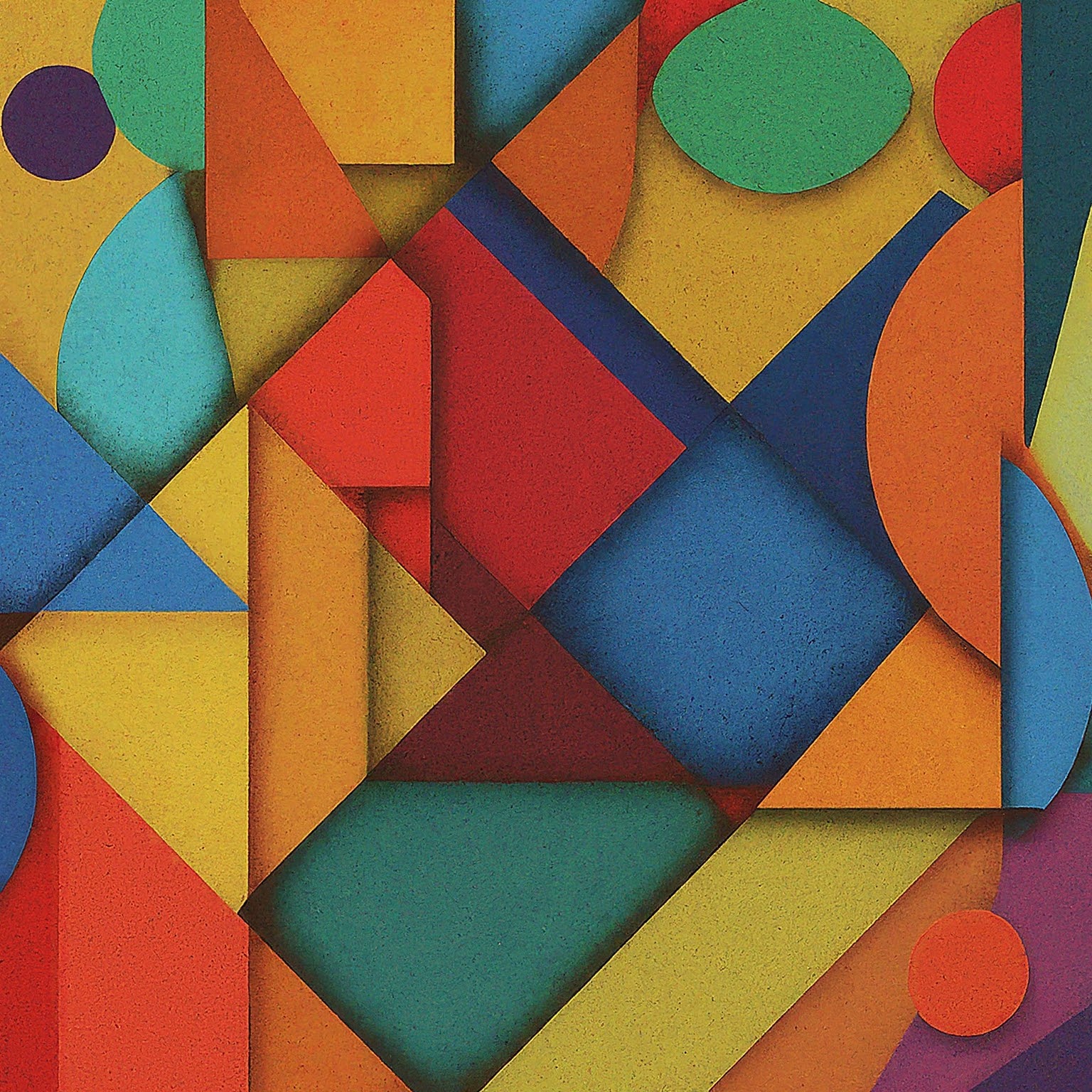
In the world of software development, especially when dealing with data-intensive applications, understanding the concepts of Models, Entities, Data Transfer Objects (DTOs), and View Models is crucial for building clean, maintainable, and efficient systems. These terms are often used interchangeably, but they serve distinct purposes and have specific roles within an application's architecture.
Models, Entities, DTOs, and View Models: A Breakdown
Entities
Core representation of real-world objects.
Persistent: Typically mapped to database tables.
Contain business logic: Encapsulate the behavior and rules related to the object.
Example:
Customer
entity with properties likeId
,Name
,Address
, and methods likeCalculateLoyaltyPoints
.
Data Transfer Objects (DTOs)
Lightweight objects for data transfer.
No behavior: Primarily used for transporting data between systems or layers.
Optimized for performance: Contains only the necessary data for the specific operation.
Example:
CustomerDto
with properties likeCustomerId
,CustomerName
, andAddress
(without any methods).
View Models
Data structures tailored for specific views.
May contain additional properties: Beyond the data from Entities or DTOs, View Models can include properties for view-specific formatting, calculations, or UI logic.
Example:
CustomerViewModel
with properties likeCustomerName
,FormattedAddress
, andOrderCount
.
Models (General Term)
Can refer to any of the above: The term "Model" is often used generically to represent any data structure used within an application.
Contextual: The specific meaning depends on the context in which it's used.
Why Use Different Constructs?
Separation of Concerns: Distinguishing between Entities, DTOs, and View Models helps maintain a clear separation of concerns between different parts of the application.
Performance: DTOs and View Models can be optimized for data transfer and presentation, respectively, improving performance.
Flexibility: DTOs and View Models allow for different data shapes to be used in different parts of the application without affecting the underlying Entities.
Data Protection: By using DTOs and View Models, you can expose only the necessary data to external systems or UI layers, protecting sensitive information.
Common Use Cases
REST APIs: DTOs are commonly used to represent data exchanged between clients and servers.
Data Mapping: AutoMapper or similar libraries can be used to map between Entities, DTOs, and View Models.
UI Binding: View Models are often used as data sources for UI components.
// Entity
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Address { get; set; }
public int CalculateLoyaltyPoints() { ... }
}
// DTO
public class CustomerDto
{
public int CustomerId { get; set; }
public string CustomerName { get; set; }
public string Address { get; set; }
}
// View Model
public class CustomerViewModel
{
public string CustomerName { get; set; }
public string FormattedAddress { get; set; }
public int OrderCount { get; set; }
}
Best Practices
Keep Mappings Clean: Avoid overly complex mapping configurations. Break down complex mappings into smaller steps.
Use Conventions: Leverage naming conventions and conventions provided by mapping tools to reduce configuration effort.
Test Thoroughly: Ensure correct mapping behavior through unit tests.
Consider Performance: For performance-critical scenarios, profile mapping operations and optimize as needed.
Models, Entities, DTOs, and View Models in Different Architectural Patterns
The usage and interactions of these constructs vary based on the architectural pattern employed. Let's examine a few common patterns:
Three-Tier Architecture
Entities: Reside in the data access layer, representing database tables.
DTOs: Used for data transfer between tiers, often employed in the service layer.
View Models: Created in the presentation layer for UI binding.
Domain-Driven Design (DDD)
Entities: Core domain objects with identity and lifecycle.
DTOs: Used for exposing domain data to external systems or for data transfer.
View Models: Created for specific UI needs, possibly enriched with presentation logic.
Clean Architecture
Entities: Pure domain objects, independent of infrastructure and UI.
DTOs: Used for data transfer between layers, especially in the use case layer.
View Models: Created in the presentation layer for UI binding.
Microservices Architecture
Entities: Typically owned by individual microservices.
DTOs: Used for communication between microservices and for exposing data to external systems.
View Models: Created by client applications consuming microservices APIs.
Additional Considerations
Performance Optimization: For large datasets or frequent mapping operations, consider techniques like lazy loading, batching, or caching.
Data Validation: Implement data validation at appropriate layers (e.g., DTOs, service layer) to ensure data integrity.
Security: Protect sensitive data by carefully controlling which properties are included in DTOs and View Models.
Conclusion
By understanding the distinctions between Entities, DTOs, and View Models, you can create more structured, maintainable, and scalable applications. Using these constructs effectively can lead to improved code quality, better performance, and enhanced developer productivity.
Subscribe to my newsletter
Read articles from Phani Veludurthi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
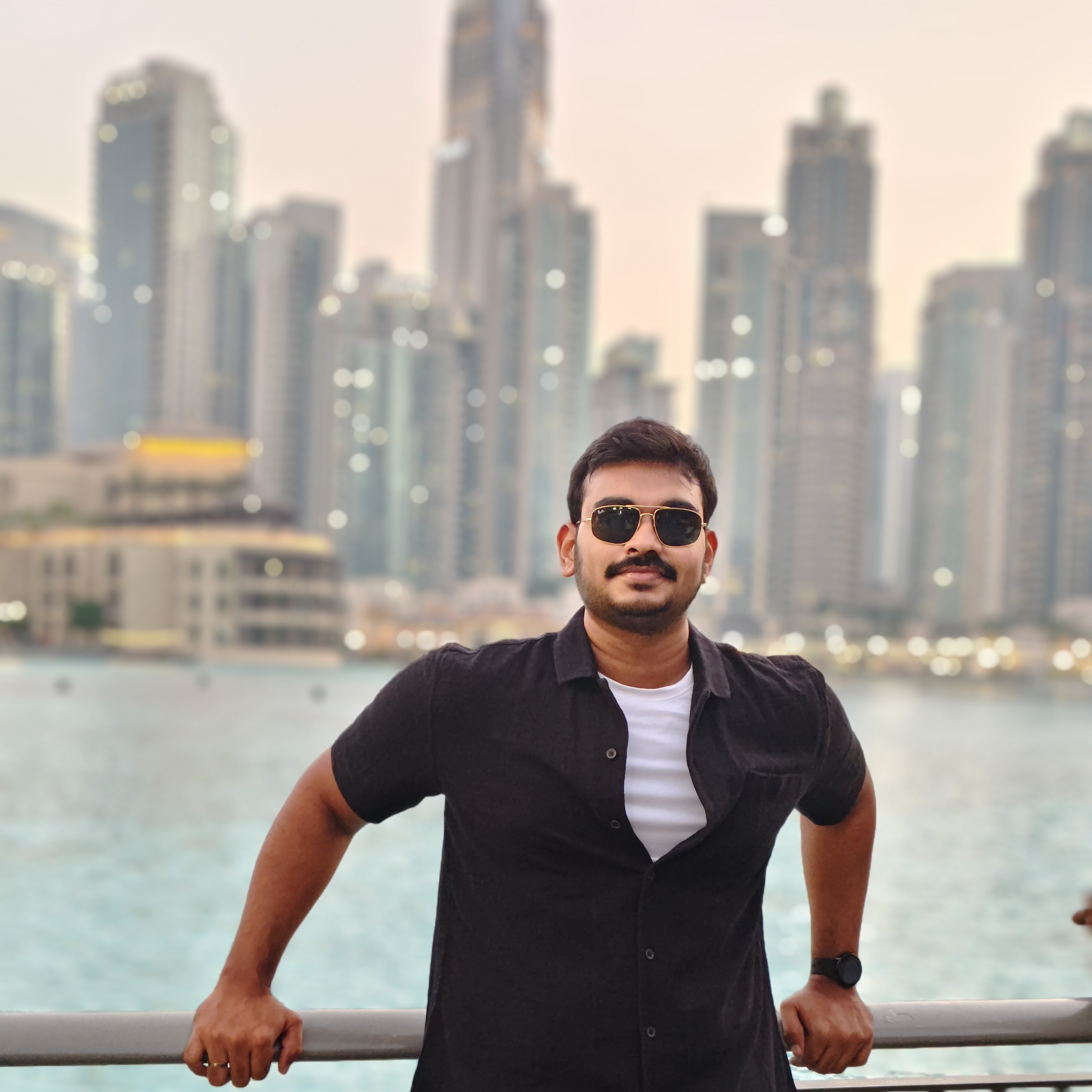