Terraform - Provisioners
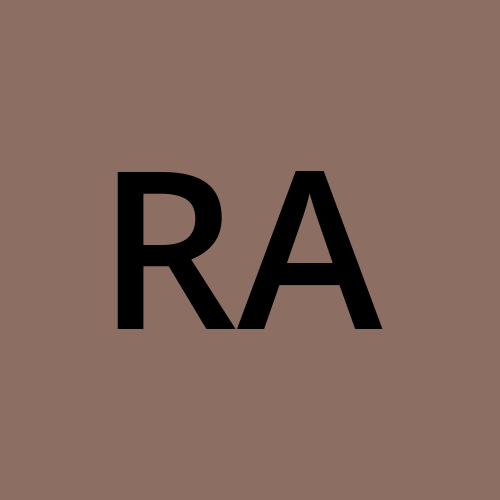
We all have played with the toys in Kinder Joy chocolate, right? So, remember this for terraform provisioners, Kinder Joy includes a toy and an instruction note that helps to set up the pieces of the toy. In the same way, Terraform provisioners act like the instruction note, guiding the setup and configuration of the infrastructure resources Terraform creates.
Provisioners are a special type of resource in Terraform. Provisioners in Terraform are used to execute scripts or commands on a local or remote machine as part of the resource creation or destruction process. They are used to perform tasks on the virtual machines or other resources after they have been created. Think of them as instructions for "what to do next" after your infrastructure is set up. For example:
Installing software on a virtual machine
Configuring settings
Running scripts
ssh-keygen
is a command-line utility used to generate, manage, and convert authentication keys for SSH (Secure Shell). It creates a pair of public and private keys that can be used for secure communication between a client and a server.
To generate a new SSH key pair, you can use the following command:
ssh-keygen
var.tf
create this terraform file or a similar one.
REGION: This variable sets the default AWS region to "us-east-2".
ZONE1: This variable sets the default availability zone to "us-east-2a".
AMIS: This variable is a map that associates AWS regions with specific Amazon Machine Images (AMIs). For example, "us-east-2" is associated with the AMI "ami-03657b56516ab7912".
USER: This variable sets the default user to "ec2-user".
variable "REGION" {
default = "us-east-2" # Default AWS region
}
variable "ZONE1" {
default = "us-east-2a" # Default availability zone
}
variable "AMIS" {
type = map # Type of the variable is a map
default = {
us-east-2 = "ami-03657b56516ab7912" # AMI for us-east-2 region
us-east-1 = "ami-0947d2ba12ee1ff75" # AMI for us-east-1 region
}
}
variable "USER" {
default = "ec2-user" # Default user
}
This setup allows you to reference these variables in your Terraform configuration, making it easier to manage and change values centrally.
provider.tf
defines an AWS provider in Terraform and sets the region using a variable.
provider "aws" {
region = var.REGION
}
provider "aws": This block configures the AWS provider, which is necessary for Terraform to interact with AWS services.
region = var.REGION: This line sets the AWS region for the provider using the value of the
REGION
variable defined earlier. This allows for flexible and centralized management of the region setting.
This setup ensures that the AWS provider will use the region specified in the REGION
variable, making it easier to change the region without modifying multiple configuration files.
web.sh
This is a shell script designed to set up a web server using Apache HTTP Server on a system that uses yum
for package management (typically a Red Hat-based distribution).
#!/bin/bash
yum install wget unzip httpd -y
systemctl start httpd
systemctl enable httpd
wget https://www.tooplate.com/zip-templates/2117_infinite_loop.zip
unzip -o 2117_infinite_loop.zip
cp -r 2117_infinite_loop/* /var/www/html/
systemctl restart httpd
Explanation:
#!/bin/bash
: This shebang line tells the system to use the Bash shell to execute the script.yum install wget unzip httpd -y
: Installswget
,unzip
, andhttpd
(Apache HTTP Server) packages without prompting for confirmation (-y
flag).systemctl start httpd
: Starts the Apache HTTP Server.systemctl enable httpd
: Enables the Apache HTTP Server to start on boot.wget
https://www.tooplate.com/zip-templates/2117_infinite_loop.zip
: Downloads a zip file containing a website template from the specified URL.unzip -o 2117_infinite_
loop.zip
: Unzips the downloaded file, overwriting any existing files (-o
flag).cp -r 2117_infinite_loop/* /var/www/html/
: Copies the contents of the unzipped template to the Apache web server's root directory (/var/www/html/
).systemctl restart httpd
: Restarts the Apache HTTP Server to apply any changes.
This script automates the process of setting up a web server, downloading a website template and deploying it to the server.
instance.tf
Defines an AWS key pair and an EC2 instance and it uses provisioners to set up the instance.
variable "REGION" {
default = "us-east-2"
}
variable "ZONE1" {
default = "us-east-2a"
}
variable "AMIS" {
type = map
default = {
us-east-2 = "ami-03657b56516ab7912"
us-east-1 = "ami-0947d2ba12ee1ff75"
}
}
variable "USER" {
default = "ec2-user"
}
provider "aws" {
region = var.REGION
}
resource "aws_key_pair" "terraform-key" {
key_name = "terraform-key"
public_key = file("terraform-key.pub")
}
resource "aws_instance" "exercise3-inst" {
ami = var.AMIS[var.REGION]
instance_type = "t2.micro"
availability_zone = var.ZONE1
key_name = aws_key_pair.terraform-key.key_name
vpc_security_group_ids = ["your security group"]
tags = {
Name = "exercise3-Instance"
Project = "exercise3"
}
provisioner "file" {
source = "web.sh"
destination = "/tmp/web.sh"
}
provisioner "remote-exec" {
inline = [
"chmod +x /tmp/web.sh",
"sudo /tmp/web.sh"
]
}
connection {
user = var.USER
private_key = file("terraform-key")
host = self.public_ip
}
}
Explanation:
Variables:
REGION
: Sets the default AWS region to "us-east-2".ZONE1
: Sets the default availability zone to "us-east-2a".AMIS
: A map associating AWS regions with specific AMIs.USER
: Sets the default user to "ec2-user".
Provider:
- Configures the AWS provider to use the region specified by the
REGION
variable.
- Configures the AWS provider to use the region specified by the
AWS Key Pair:
- Creates an SSH key pair named "terraform-key" using the public key file "terraform-key.pub".
AWS Instance:
Creates an EC2 instance with the following properties:
ami
: Uses the AMI specified in theAMIS
map for the selected region.instance_type
: Sets the instance type to "t2.micro".availability_zone
: Uses the availability zone specified by theZONE1
variable.key_name
: Associates the instance with the SSH key pair "terraform-key".vpc_security_group_ids
: Associates the instance with the specified security group.tags
: Adds tags to the instance for identification.
Provisioners:
Connection:
Defines the connection details for SSH:
user
: Uses the user specified by theUSER
variable.private_key
: Uses the private key file "terraform-key".host
: Connects to the instance's public IP address.
Run these Terraform Commands
terraform init
This command initializes a Terraform working directory. It prepares the directory by downloading and installing the necessary provider plugins and setting up the backend configuration. This is the first command that should be run after writing a new Terraform configuration or cloning an existing one from version control.
terraform init
terraform fmt
This command formats the Terraform configuration files in the current directory to a canonical format and style. It ensures that the code is properly indented and organized, making it easier to read and maintain.
terraform fmt
terraform validate
This command checks the syntax and validity of the Terraform configuration files in the current directory. It ensures that the configuration is syntactically correct and that all required arguments are specified. This is a useful step to catch errors before applying the configuration.
terraform validate
terraform plan
This command creates an execution plan, showing what actions Terraform will take to achieve the desired state defined in the configuration files. It compares the current state with the desired state and lists the changes that will be made such as creating, updating, or deleting resources. This allows you to review the proposed changes before applying them.
terraform plan
terraform apply
This command applies the changes required to reach the desired state of the configuration as defined by the Terraform files. It creates, updates or deletes infrastructure resources to match the configuration. Before making any changes, Terraform will show a plan of the actions it will take and ask for your confirmation.
terraform apply
Go to the AWS Management Console and navigate to the EC2 Dashboard.
Check the Created Instance:
In the EC2 Dashboard, find the instance you created. You can identify it by the tags you assigned, such as "Name: exercise3-Instance" and "Project: exercise3".
Ensure the instance is in the "running" state.
Copy the Public IP Address:
Select the instance to view its details.
Locate the "Public IPv4 address" field and copy the IP address.
Check the Website in a Web Browser:
Open a web browser.
Paste the copied public IP address into the address bar and press Enter.
You should see the website that was set up by the
web.sh
script.
This process verifies that the instance is running and that the web server has been correctly configured and deployed.
https://github.com/Ragavi04P/Terraform-Practice/tree/main/exercise3
Subscribe to my newsletter
Read articles from Ragavi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
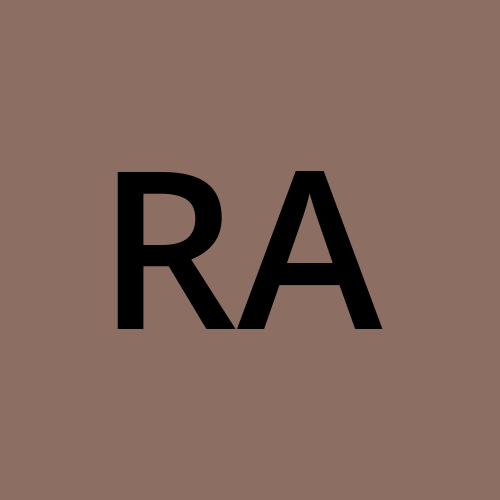
Ragavi
Ragavi
Hey there! I'm Ragavi, I share blogs on AWS & DevOps, books I read and business insights! I'm passionate about sharing my unique perspective through my blogs.