Probability Distributions in Machine Learning
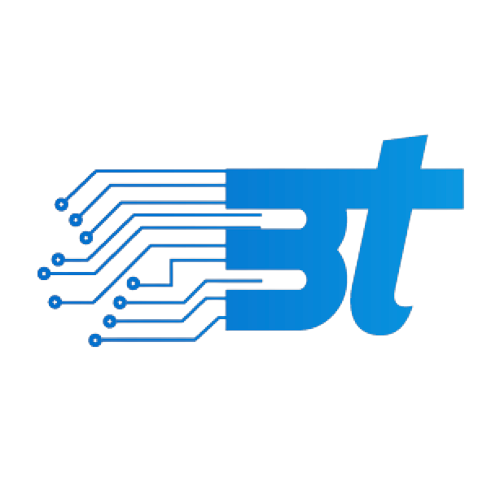
Table of contents
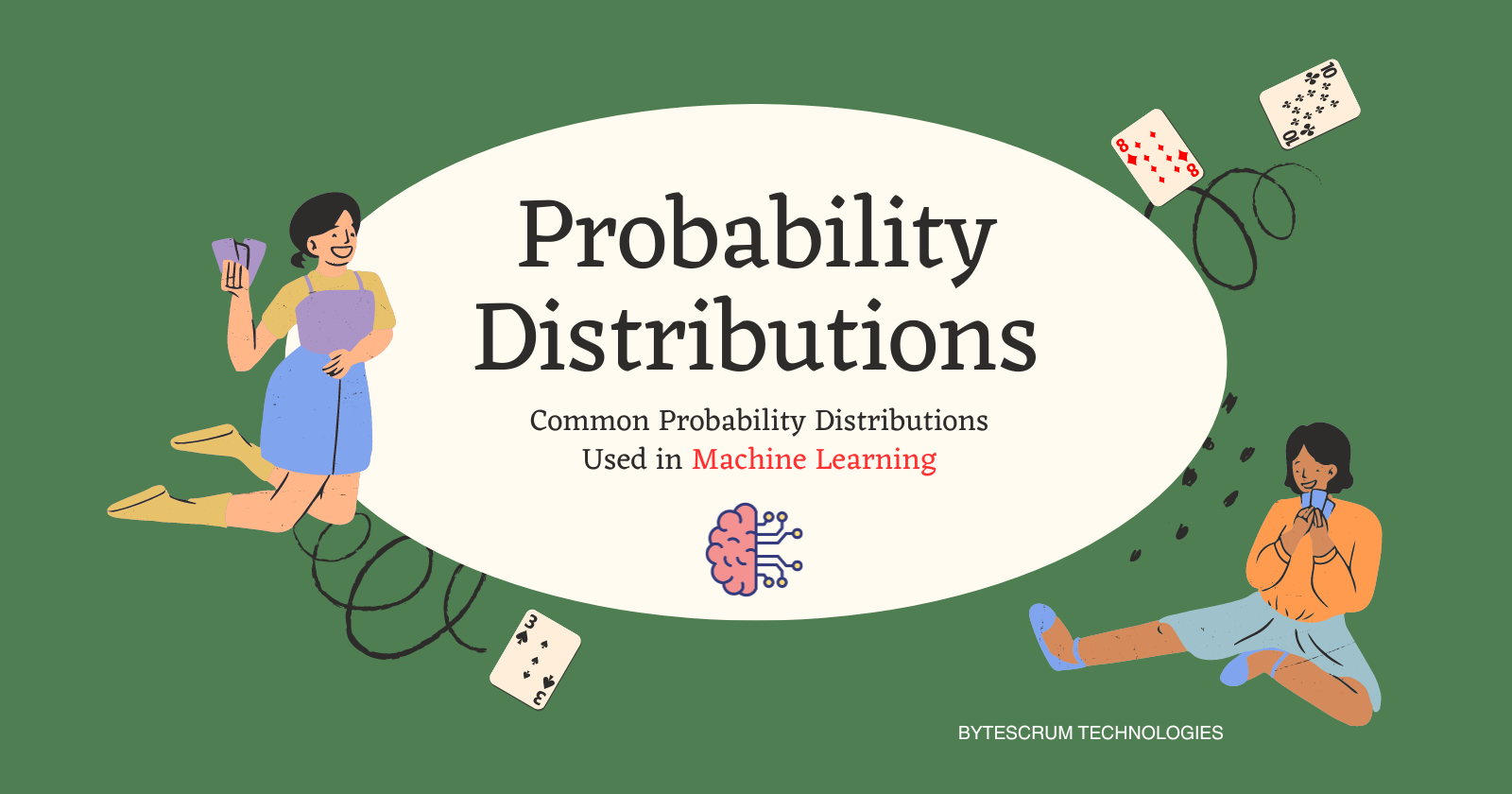
Probability distributions play a vital role in the field of machine learning, providing the mathematical foundation for many algorithms and models. They help in understanding data, making predictions, and estimating uncertainty. In this blog, we will explore the concept of probability distributions, their importance in machine learning, and some common types of distributions used in the field.
What is a Probability Distribution?
A probability distribution shows how the values of a random variable are spread out. It tells us the likelihood of different outcomes. There are two main types of probability distributions:
Discrete Probability Distributions: These are used for variables that can take on a countable number of values.
Continuous Probability Distributions: These are used for variables that can take on any value within a range.
Why are Probability Distributions Important in Machine Learning?
Data Understanding: By looking at the probability distribution of a dataset, insights about the data’s characteristics, such as central tendency, variability, and shape, can be gained. This helps in choosing suitable models and algorithms.
Modeling Uncertainty: Probability distributions help in modeling uncertainty and variability in the data, which is important for making reliable predictions and decisions.
Bayesian Inference: Many machine learning algorithms, especially those based on Bayesian inference, rely on probability distributions to update beliefs with new data.
Sampling and Simulation: Distributions are used to create synthetic data, useful for testing and validating models when real data is scarce.
Common Probability Distributions in Machine Learning
1. Normal Distribution (Gaussian Distribution)
The normal distribution is one of the most commonly used continuous distributions. It has a bell-shaped curve that is symmetric around the mean.
Key properties:
The mean, median, and mode are all equal.
The curve is symmetric about the mean.
About 68% of the data falls within one standard deviation of the mean, 95% within two, and 99.7% within three.
Example:
Imagine measuring the heights of a large group of people. Most people’s heights will be around the average, with fewer people being very short or very tall. This pattern follows a normal distribution.
Applications:
Used in algorithms like Gaussian Naive Bayes.
Assumed in many statistical methods and hypothesis tests.
Code Example:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import norm
# Parameters for the normal distribution
mean = 0
std_dev = 1
# Generate data
data = np.random.normal(mean, std_dev, 1000)
# Plot the data
plt.hist(data, bins=30, density=True, alpha=0.6, color='b')
# Plot the probability density function (PDF)
xmin, xmax = plt.xlim()
x = np.linspace(xmin, xmax, 100)
p = norm.pdf(x, mean, std_dev)
plt.plot(x, p, 'k', linewidth=2)
title = "Normal Distribution (mean = 0, std_dev = 1)"
plt.title(title)
plt.show()
2. Binomial Distribution
The binomial distribution is a discrete distribution that describes the number of successes in a fixed number of independent yes/no experiments.
Key properties:
Defined by the number of trials (n) and the probability of success (p).
The mean is npnpnp, and the variance is np(1−p)np(1-p)np(1−p).
Example:
Consider flipping a coin 10 times and counting how many times it lands on heads. The number of heads follows a binomial distribution.
Applications:
Used in binary classification problems.
Modeling binary outcomes like coin flips or predicting the number of successes.
Code Example:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import binom
# Parameters for the binomial distribution
n = 10 # number of trials
p = 0.5 # probability of success
# Generate data
data = np.random.binomial(n, p, 1000)
# Plot the data
plt.hist(data, bins=np.arange(0, n+1)-0.5, density=True, alpha=0.6, color='b')
# Plot the probability mass function (PMF)
x = np.arange(0, n+1)
pmf = binom.pmf(x, n, p)
plt.plot(x, pmf, 'ko', ms=8)
plt.vlines(x, 0, pmf, colors='k', lw=2)
title = "Binomial Distribution (n = 10, p = 0.5)"
plt.title(title)
plt.show()
3. Poisson Distribution
The Poisson distribution is a discrete distribution that describes the probability of a given number of events occurring in a fixed interval of time or space.
Key properties:
Defined by a single parameter λ (lambda), the average rate of occurrence.
The mean and variance are both equal to λ.
Example:
Imagine counting the number of emails received in an hour. If, on average, 5 emails are received per hour, the number of emails in any given hour follows a Poisson distribution.
Applications:
Modeling count data and rare events.
Used in anomaly detection, queueing theory, and natural language processing.
Code Example:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import poisson
# Parameter for the Poisson distribution
lambda_ = 5 # average rate of occurrence
# Generate data
data = np.random.poisson(lambda_, 1000)
# Plot the data
plt.hist(data, bins=30, density=True, alpha=0.6, color='b')
# Plot the probability mass function (PMF)
x = np.arange(0, np.max(data))
pmf = poisson.pmf(x, lambda_)
plt.plot(x, pmf, 'ko', ms=8)
plt.vlines(x, 0, pmf, colors='k', lw=2)
title = "Poisson Distribution (lambda = 5)"
plt.title(title)
plt.show()
4. Exponential Distribution
The exponential distribution is a continuous distribution used to model the time between events in a Poisson process.
Key properties:
Defined by a single parameter λ (lambda), the rate parameter.
Memoryless property: The probability of an event occurring in the next interval is independent of the time since the last event.
Example:
Think about the time between arrivals of buses at a bus stop. If buses arrive every 10 minutes on average, the time between arrivals follows an exponential distribution.
Applications:
- Modeling survival analysis, reliability engineering, and time-to-failure data.
Code Example:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import expon
# Parameter for the exponential distribution
lambda_ = 1 # rate parameter
# Generate data
data = np.random.exponential(1/lambda_, 1000)
# Plot the data
plt.hist(data, bins=30, density=True, alpha=0.6, color='b')
# Plot the probability density function (PDF)
xmin, xmax = plt.xlim()
x = np.linspace(xmin, xmax, 100)
pdf = expon.pdf(x, scale=1/lambda_)
plt.plot(x, pdf, 'k', linewidth=2)
title = "Exponential Distribution (lambda = 1)"
plt.title(title)
plt.show()
5. Multinomial Distribution
The multinomial distribution is a generalization of the binomial distribution for more than two outcomes. It describes the probabilities of counts for each possible outcome.
Key properties:
Defined by the number of trials (n) and the probabilities for each outcome (p).
Each trial results in exactly one of the possible outcomes.
Example:
Consider rolling a six-sided die 10 times and counting the number of times each face appears. The counts follow a multinomial distribution.
Applications:
Used in natural language processing for modeling word frequencies.
Applied in multi-class classification problems.
Code Example:
import numpy as np
import matplotlib.pyplot as plt
# Parameters for the multinomial distribution
n = 10 # number of trials
p = [1/6]*6 # probabilities for each outcome (fair die)
# Generate data
data = np.random.multinomial(n, p, 1000)
# Sum over all trials
counts = np.sum(data, axis=0)
# Plot the data
plt.bar(np.arange(1, 7), counts/np.sum(counts), alpha=0.6, color='b')
title = "Multinomial Distribution (n = 10, p = [1/6]*6)"
plt.title(title)
plt.xlabel("Outcome")
plt.ylabel("Probability")
plt.show()
Conclusion
Understanding these distributions allows better decisions on which models to use and how to interpret the results. Happy coding!
Follow us on Whatsapp and other social media!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
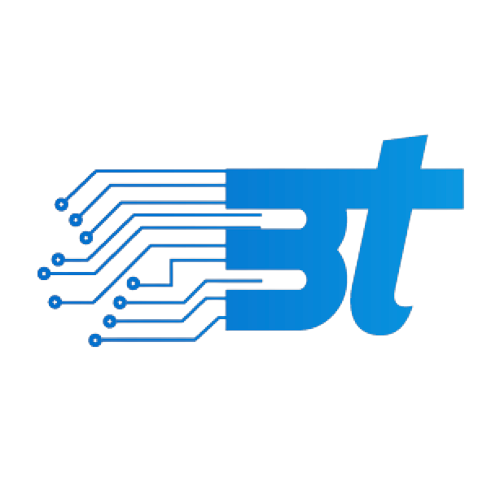
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.