APIs in Action

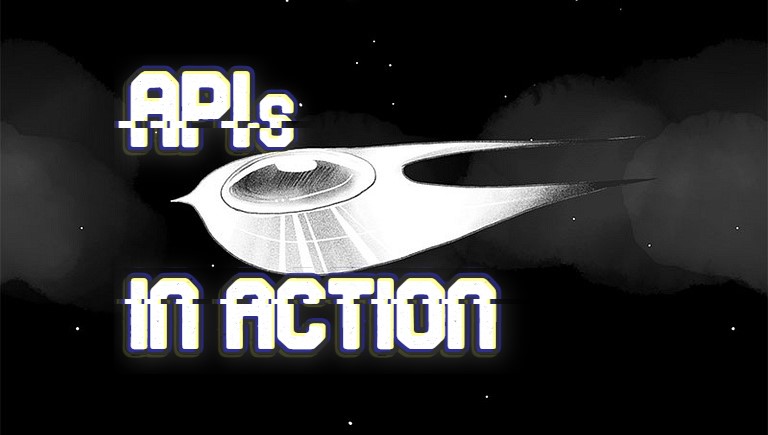
Introduction >
APIs simplify and accelerate application and software development by allowing developers to integrate data, services and capabilities from other applications, instead of developing them from scratch.
APIs allow for the sharing of only the information necessary, keeping other internal system details hidden, which helps with system security. Servers or devices do not have to fully expose data—APIs enable the sharing of small packets of data, relevant to the specific request.
API documentation is like a technical instruction manual that provides details about an API and information for developers on how to work with an API and its services. Well-designed documentation promotes a better API experience for users and generally makes for more successful APIs.
An API is like a waiter in a restaurant. Imagine we go to a restaurant and want to order food. We don't go into the kitchen ourselves to make the food. Instead, we tell the waiter what we want, and the waiter goes to the kitchen, tells the chefs, and then brings our food back to us.
In the same way, an API (Application Programming Interface) is a waiter for software. It takes your request, tells another software what we need, and then brings the result back to us.
Real-World Example: Ordering Pizza with an API >
Let's say we want to order pizza using a pizza delivery app. Here's how it works:
Opening the App:
- We start the pizza delivery app on our phone.
Search for Pizzas:
We tell the app we want to see the available pizzas (like telling the waiter what’s on the menu).
The app uses an API to ask the pizza restaurant for the list of pizzas.
Example Request:
httpCopy codeGET https://api.pizzashop.com/menu
Get the Menu:
The pizza restaurant’s API responds with a list of pizzas.
Example Response:
jsonCopy code{ "pizzas": [ {"name": "Margherita", "price": 10}, {"name": "Pepperoni", "price": 12}, {"name": "Veggie", "price": 11} ] }
Choosing a Pizza:
We look at the list and decide we want a Pepperoni pizza.
We tell the app to order a Pepperoni pizza.
Place the Order:
The app uses the API to send our order to the pizza restaurant.
Example Request:
httpCopy codePOST https://api.pizzashop.com/order Content-Type: application/json { "pizza": "Pepperoni", "address": "123 Main Street", "payment": {"cardNumber": "4111111111111111", "expiryDate": "12/24"} }
Confirm the Order:
The pizza restaurant’s API confirms the order and tells the app that our pizza is on its way.
Example Response:
jsonCopy code{
"orderId": "12345",
"status": "confirmed",
"deliveryTime": "30 minutes"
}
Types of APIs >
By Release Policies
By Use Cases
Web APIs:
These are like online waiters. They help websites talk to each other.
Example: When a weather website shows the current weather by asking another website for data.
Library-Based APIs:
These are like a toolkit you get with a toy. They help you build something specific.
Example: A drawing program giving you tools to draw lines and shapes.
Operating System APIs:
These are like the instructions that tell your computer how to open a file or run a program.
Example: When you open a game on your computer, and it knows how to use your graphics card to show images.
Hardware APIs:
These are like the remote control for your TV. They help software talk to hardware.
Example: A music app using your phone’s speakers to play a song.
Today, most APIs are web APIs. Web APIs are a type of remote API (meaning that the API uses protocols to manipulate external resources) that expose an application's data and functionality over the internet.
Types of web APIs
Open APIs
Open APIs are open-source application programming interfaces you can access with the HTTP protocol. Also known as public APIs, they have defined API endpoints and request and response formats.
Partner APIs
Partner APIs connect strategic business partners. Typically, developers access these APIs in self-service mode through a public API developer portal. Still, they need to complete an onboarding process and get login credentials to access partner APIs.
Internal APIs
Internal, or private, APIs remain hidden from external users. These private APIs aren't available for users outside of the company. Instead, organizations use them to improve productivity and communication across different internal development teams.
Composite APIs
Composite APIs combine multiple data or service APIs. They allow programmers to access several endpoints in a single call. Composite APIs are useful in microservices architecture where running a single task might require information from several sources.
Why APIs are Cool >
Integration:
They help different apps work together.
Example: The Fitness app showing how many steps we’ve taken by talking to our smartwatch.
Automation:
They can do things automatically without we doing them manually.
Example: A social media app posting our pictures at a scheduled time.
Data Access:
They let apps get information from other places.
Example: A travel app showing the weather at our destination by asking a weather website.
Adding Features:
They add new abilities to apps.
Example: A translation app using a language API to translate text for us
More Examples >
Universal logins
A popular API example is the function that enables people to log in to websites by using their Facebook, X, or Google profile login details. This convenient feature allows any website to use an API from one of the more popular services for quick authentication.
Internet of Things (IoT)
These “smart devices” offer added functionality, such as internet-enabled touchscreens and data collection, through APIs. For example, a smart fridge can connect to recipe applications or take and send notes to mobile phones through text message.
Travel booking comparisons
Travel booking sites aggregate thousands of flights, showcasing the cheapest options for every date and destination. APIs enable this service by providing application users access to the latest information about availability from hotels and airlines.
Navigation apps
Navigation apps use core APIs that display static or interactive maps. These apps also use other APIs and features to provide users with directions, speed limits, points of interest, traffic warnings and more. Users communicate with an API when plotting travel routes or tracking items on the move, such as a delivery vehicle.
Social media
Social media companies use APIs to allow other entities to share and embed content featured on social media apps to their own sites. For example, the Instagram API enables businesses to embed their Instagram grid on their website and for the grid to update automatically as users add new posts.
SaaS applications
APIs are an integral part of the growth in software as a service (SaaS) products. Platforms like CRMs (customer relationship management tools) often include several built-in APIs that let companies integrate with applications they already use, such as messaging, social media and email apps.
API Protocols >
API protocols define the rules and conventions for communication between software applications. Here are some common ones:
HTTP/HTTPS:
Hypertext Transfer Protocol: The foundation of data communication for the web. HTTPS is the secure version.
Example: Most web APIs use HTTP/HTTPS for communication.
SOAP (Simple Object Access Protocol):
A protocol for exchanging structured information in web services. Uses XML for message format.
Example: Used in enterprise-level applications like banking systems.
REST (Representational State Transfer):
An architectural style that uses HTTP requests to access and manipulate resources. Typically uses JSON for data format.
Example: Many modern web services, such as Twitter API, use REST.
GraphQL:
A query language for APIs that allows clients to request exactly the data they need.
Example: Used by companies like Facebook and GitHub for their APIs.
gRPC (gRPC Remote Procedure Call):
A high-performance RPC framework that uses HTTP/2 for transport, Protocol Buffers as the interface description language, and supports multiple programming languages.
Example: Used in microservices architectures for efficient communication.
API Languages >
JSON (JavaScript Object Notation):
A lightweight data-interchange format that's easy for humans to read and write and easy for machines to parse and generate.
Example: Widely used in RESTful APIs.
XML (eXtensible Markup Language):
A markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable.
Example: Common in SOAP APIs.
Protocol Buffers (protobuf):
A language-neutral, platform-neutral extensible mechanism for serializing structured data.
Example: Used in gRPC for defining data structures and services.
API Architectural Styles >
Determine the structure and behavior of the API
REST (Representational State Transfer):
Based on stateless communication and standard HTTP methods (GET, POST, PUT, DELETE).
Key Principles: Stateless, cacheable, client-server architecture, uniform interface.
Example: Google Maps API.
SOAP (Simple Object Access Protocol):
A protocol that uses XML-based messages and can operate over different lower-level protocols like HTTP, SMTP.
Key Features: Strict standards, built-in error handling, security features like WS-Security.
Example: PayPal API.
GraphQL:
Allows clients to specify the structure of the response data.
Key Features: Single endpoint, flexible queries, real-time updates.
Example: GitHub API.
RPC (Remote Procedure Call):
Enables executing a procedure (subroutine) on a remote server.
Types: gRPC (Google), XML-RPC (uses XML to encode its calls), JSON-RPC (uses JSON).
Example: gRPC in microservices.
API components: calls, keys, endpoints >
An API call is a request a client app forwards to a server. It contains
operations to be executed (for example, GET to retrieve a resource or POST to send data),
authentication details — for example, an API key that identifies the client,
additional parameters, and
a destination address — the URL of the API endpoint.
An API endpoint is like an entrance to a place where a sought-after resource (data or feature) lives. The endpoint checks the API key and, if approved, sends back a response with the information on the operation status (error or success) and requested resources.
Integrating API >
Understand the API Documentation: First, familiarize yourself with the API documentation provided by the service you want to integrate. This documentation typically includes endpoints, request methods, parameters, authentication methods, and response formats.
Choose Your Programming Language/Platform: Decide which programming language or platform you'll use to make API requests. Common choices include Python, JavaScript (Node.js), Java, and others.
Set Up Authentication: If the API requires authentication (which many do), set up the necessary credentials or tokens. This might involve obtaining an API key, OAuth tokens, or other forms of authentication.
Make API Requests: Use your chosen programming language's HTTP library (like
requests
in Python oraxios
in JavaScript) to make HTTP requests to the API endpoints specified in the documentation. Include required headers, parameters, and the request body as specified.Handle Responses: Once you receive a response from the API, handle it appropriately based on your application's needs. This may involve parsing JSON or XML responses, error handling, and logging.
Implement Error Handling: APIs can return errors for various reasons (e.g., invalid parameters, rate limiting). Implement error handling to gracefully manage these scenarios and provide feedback to users if necessary.
Testing and Debugging: Test your integration thoroughly in a development environment. Use tools like Postman or curl to manually test API requests. Debug any issues encountered during testing.
Monitor and Maintain: After deployment, monitor your API usage and performance. Keep an eye on rate limits, API changes, and updates from the service provider.
Security Considerations: Ensure secure handling of API keys and tokens. Avoid hardcoding sensitive information in your application's source code.
Ending >
This project, built by me, utilizes the Weather API to provide real-time weather information. Developed using React.js, this application serves as a practical example of integrating external data sources seamlessly into web applications. Users can easily access current weather conditions and forecasts directly through the intuitive interface, demonstrating the power of API-driven development in enhancing user experiences.
So in this post we explored how APIs make apps talk to each other easily. They're like the glue that connects different software, making our digital world more connected and powerful.
I hope this post has been helpful. If you have any questions, please feel free to leave a comment below.
Happy Coding !
Thank You
Cover Image Credits: Rachel Nabors ( Dribbble )
Subscribe to my newsletter
Read articles from Shrutik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrutik
Shrutik
Student of code, seeker of solutions. I write short, helpful articles to inspire and guide tech enthusiasts.