Understanding PHP Loops: For, While, and Do-While Explained

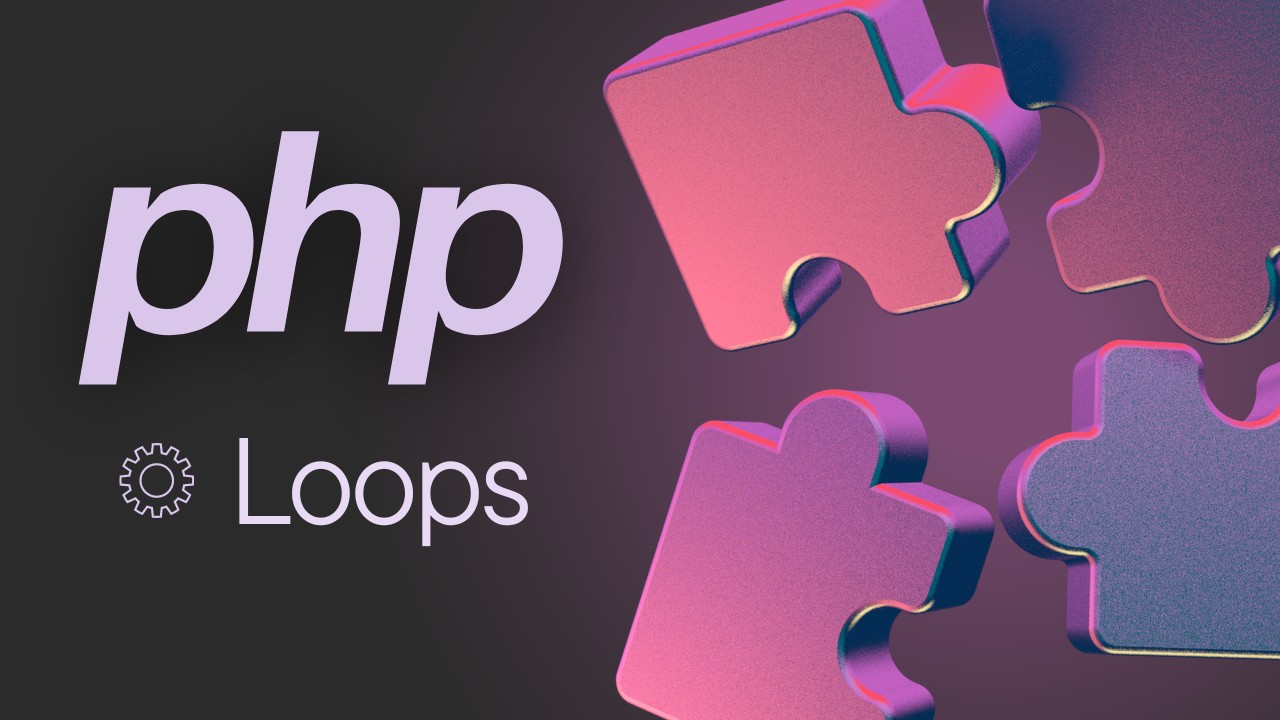
Today, we will explore loops in PHP, which allow you to execute a block of code multiple times based on certain conditions. Loops are essential for tasks that require repetitive actions, such as iterating through arrays or processing data in batches. We will cover three types of loops in PHP: for
, while
, and do-while
.
Types of Loops in PHP
for Loop
while Loop
do-while Loop
1. for Loop
The for
loop is used when you know in advance how many times you want to execute a statement or a block of statements. It consists of three parts: initialization, condition, and increment/decrement.
Syntax:
for (initialization; condition; increment/decrement) {
// Code to be executed
}
Example:
<?php
for ($i = 0; $i < 5; $i++) { // Initialize $i to 0; Run loop while $i is less than 5; Increment $i by 1 after each iteration
echo "The number is: $i <br>"; // Output the current value of $i
}
// Outputs: The number is: 0, The number is: 1, The number is: 2, The number is: 3, The number is: 4
?>
2. while Loop
The while
loop is used when you want to repeat a block of code as long as a condition is true. The condition is evaluated before the execution of the loop's body.
Syntax:
while (condition) {
// Code to be executed
}
Example:
<?php
$i = 0; // Initialize $i to 0
while ($i < 5) { // Run loop while $i is less than 5
echo "The number is: $i <br>"; // Output the current value of $i
$i++; // Increment $i by 1 after each iteration
}
// Outputs: The number is: 0, The number is: 1, The number is: 2, The number is: 3, The number is: 4
?>
3. do-while Loop
The do-while
loop is similar to the while
loop, but it ensures that the block of code is executed at least once. The condition is evaluated after the execution of the loop's body.
Syntax:
do {
// Code to be executed
} while (condition);
Example:
<?php
$i = 0; // Initialize $i to 0
do {
echo "The number is: $i <br>"; // Output the current value of $i
$i++; // Increment $i by 1 after each iteration
} while ($i < 5); // Run loop while $i is less than 5
// Outputs: The number is: 0, The number is: 1, The number is: 2, The number is: 3, The number is: 4
?>
Best Practices for Using Loops
Avoid Infinite Loops:
- Ensure that the loop condition will eventually become false to avoid infinite loops that can crash your application.
// Example of a potential infinite loop
$i = 0;
while ($i >= 0) {
echo $i;
$i++; // This will never stop as $i will always be greater than or equal to 0
}
Use Break and Continue Wisely:
Use
break
to exit a loop prematurely when a certain condition is met.Use
continue
to skip the rest of the current iteration and proceed to the next iteration.Note: We will cover break and continue in the next blog.
for ($i = 0; $i < 10; $i++) {
if ($i == 5) {
break; // Exit the loop when $i is 5
}
if ($i % 2 == 0) {
continue; // Skip even numbers
}
echo $i; // Output only odd numbers less than 5
}
Optimize Loop Performance:
- Avoid unnecessary calculations or function calls within the loop. Move them outside the loop if possible to improve performance.
$count = count($array);
for ($i = 0; $i < $count; $i++) {
// Process each element
}
Conclusion
Today, we explored the three main types of loops in PHP: for
, while
, and do-while
. Each loop type serves different purposes and can be used to execute a block of code multiple times based on specific conditions. Understanding how to use loops effectively is crucial for writing efficient and maintainable code.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.