Recursive Function Implementation in Backend

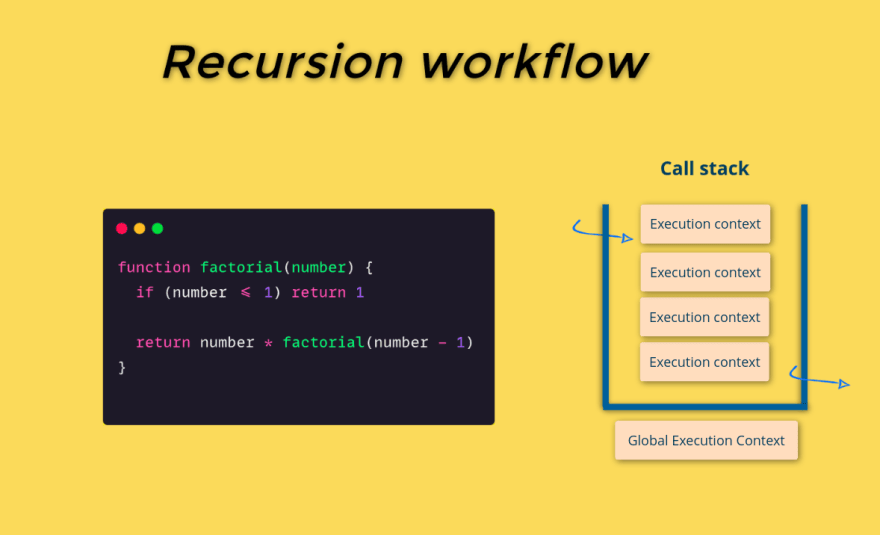
Example: File System Traversal
Imagine you are developing a backend application that needs to traverse a file system to find all files with a specific extension (e.g., .txt
, .jpg
) and compute the total size of these files.
const fs = require('fs');
const path = require('path');
// Function to recursively traverse a directory
function traverseDirectory(dirPath, extension, totalSize = 0) {
const files = fs.readdirSync(dirPath);
files.forEach(file => {
const filePath = path.join(dirPath, file);
const stats = fs.statSync(filePath);
if (stats.isDirectory()) {
// Recursively traverse subdirectories
totalSize = traverseDirectory(filePath, extension, totalSize);
} else if (stats.isFile() && path.extname(file) === extension) {
// Process files with the specified extension
console.log(`Found file: ${filePath} (${stats.size} bytes)`);
totalSize += stats.size;
}
});
return totalSize;
}
// Example usage: Traverse the current directory recursively for .txt files
const directoryPath = './';
const fileExtension = '.txt';
const totalFileSize = traverseDirectory(directoryPath, fileExtension);
console.log(`Total size of ${fileExtension} files: ${totalFileSize} bytes`);
Explanation
Function
traverseDirectory
: This function usesfs.readdirSync
to read the contents of a directory synchronously. It iterates through each file and directory within the specifieddirPath
.Recursive Case: If the current item (
file
) is a directory (stats.isDirectory()
), the function recursively calls itself with the directory path (filePath
) to explore its contents further.Base Case: If the current item (
file
) is a file (stats.isFile()
) and has the specified extension (path.extname(file) === extension
), it processes the file (in this case, printing its path and size) and adds its size (stats.size
) tototalSize
.Returning Results: The function returns
totalSize
recursively accumulated from all files matching the extension in the directory tree.
Usage
In this example, the recursive function traverseDirectory
is used to traverse a directory structure and find all .txt
files, printing their paths and sizes. The function could easily be adapted to handle different file operations, such as copying files, counting files, or analyzing file contents recursively.
This scenario demonstrates how recursive functions are essential for tasks that involve hierarchical or nested data structures, such as file systems, directory trees, nested comments, organizational charts, and more.
Subscribe to my newsletter
Read articles from Kumar Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
