The Hitchhiker's Guide to Computer Science
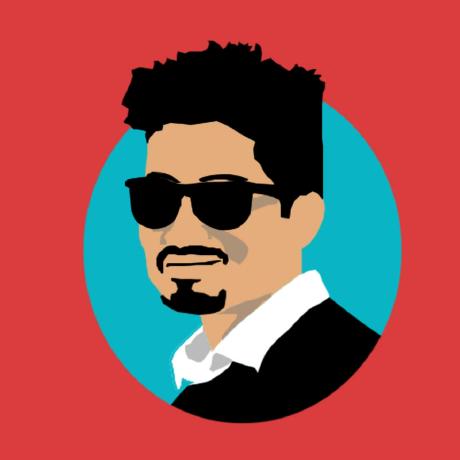
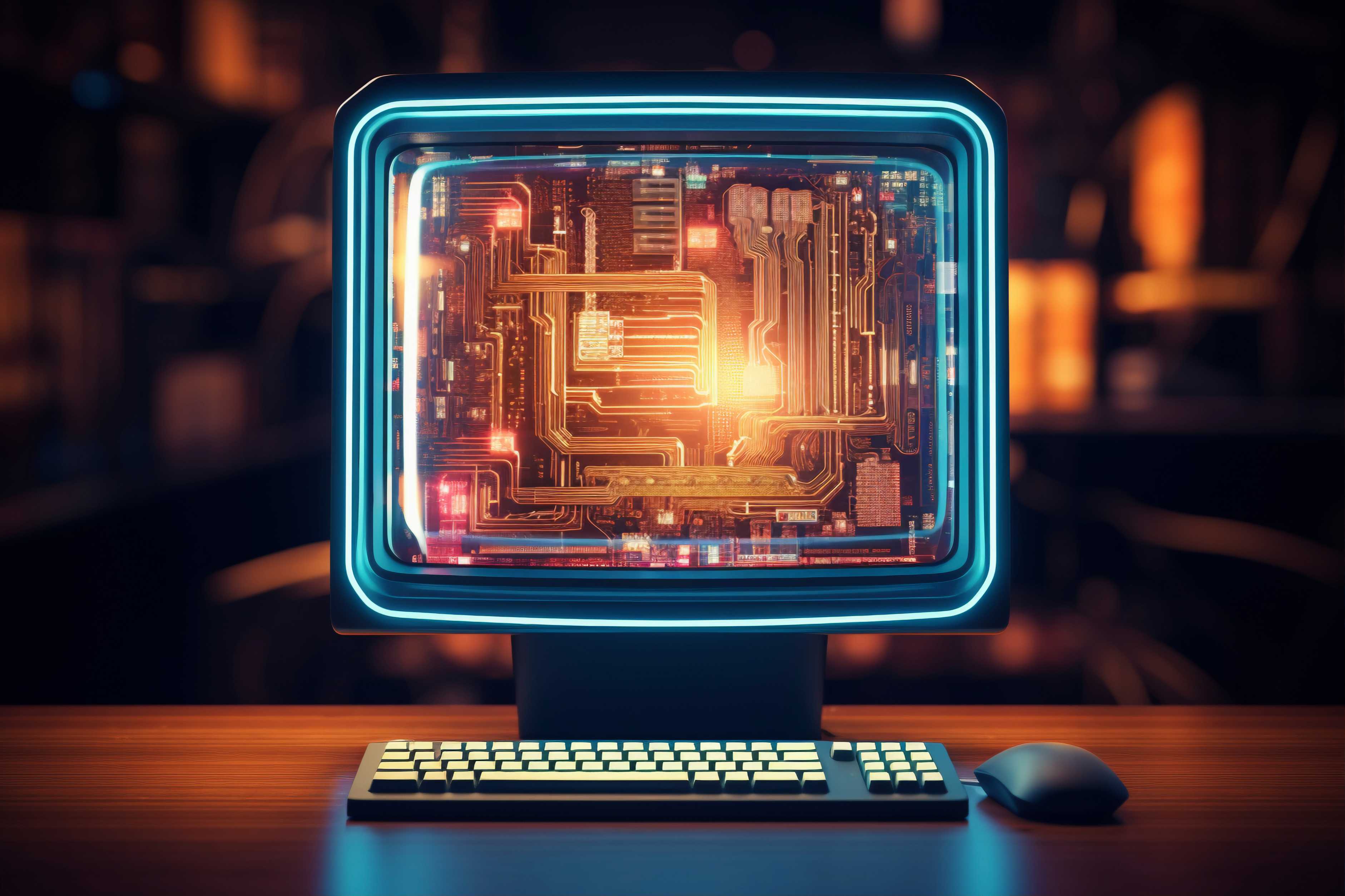
Welcome, fellow travelers, to a journey through the vast landscape of computer science. This guide aims to distill computer science into a single post, guiding you from the depths of hardware to the heights of artificial intelligence.
Hardware and Binary Basics
The Heart of the Machine: Understanding Hardware
Imagine opening your computer and peering inside. The first thing you'll notice is the motherboard, the backbone connecting all the essential parts. Key components include:
CPU (Central Processing Unit): Often referred to as the brain of the computer, the CPU performs calculations and executes instructions. It’s composed of billions of transistors that switch on and off to process data. Think of it as a maestro conducting an orchestra, ensuring every component plays in harmony. For example, when you press a key on your keyboard, the CPU processes this input and tells the appropriate software to display the character on the screen.
The CPU consists of several parts:
ALU (Arithmetic Logic Unit): Handles mathematical operations and logical comparisons. Imagine the ALU as the mathematician of the CPU, constantly crunching numbers and making decisions.
Control Unit: Directs the operation of the processor. The control unit is like the conductor of an orchestra, directing various components to work together seamlessly.
Registers: Small storage locations for immediate data manipulation. Registers are the CPU's scratchpad, where it temporarily stores data needed for computations.
The CPU executes instructions through a cycle known as the fetch-decode-execute cycle:
Fetch: Retrieve an instruction from memory.
Decode: Translate the instruction into signals.
Execute: Perform the action specified by the instruction.
RAM (Random Access Memory): Think of RAM as your computer’s short-term memory. It temporarily holds data that the CPU needs quick access to, speeding up processing times. Imagine trying to cook a complicated recipe; RAM is like having all your ingredients prepped and within arm's reach. When you open a program, it gets loaded from your hard drive into RAM to run smoothly.
RAM operates at much higher speeds compared to storage. For example, when you open a web browser, the program files and any web pages you visit are loaded into RAM, allowing for quick access and smooth browsing. There are different types of RAM, including:
DRAM (Dynamic RAM): Needs to be refreshed thousands of times per second.
SRAM (Static RAM): Faster and more expensive, does not need to be refreshed.
Storage (Hard Drives and SSDs): Unlike RAM, your storage devices retain information even when the power is off. Hard drives use magnetic storage, while SSDs (Solid-State Drives) use flash memory, providing faster data access. For instance, SSDs can dramatically reduce boot times for your operating system compared to traditional hard drives.
Hard drives consist of spinning platters with a magnetic coating, and data is read/written by a moving arm with read/write heads. SSDs, on the other hand, have no moving parts and use interconnected flash memory chips, making them faster and more durable. SSDs are particularly useful for tasks that require rapid data access, such as booting up an operating system or launching applications.
The Binary World: Bits and Bytes
At the core of all computing is the binary system. Everything your computer does, from displaying images to running programs, is ultimately represented by binary digits (bits). A bit can be either a 0 or a 1. Group eight bits together, and you get a byte.
Binary Arithmetic: Computers perform calculations using binary arithmetic. For example, adding two binary numbers involves carrying over just like in decimal addition but with base-2. If you add binary 1010 (10 in decimal) and 0101 (5 in decimal), you get 1111 (15 in decimal).
Binary multiplication and division follow similar principles but are simpler because they only involve 0s and 1s. For example, multiplying 101 (5 in decimal) by 10 (2 in decimal) results in 1010 (10 in decimal). These operations are fundamental to how CPUs process data, as they break down complex tasks into simple binary operations.
Data Representation: All data types, whether text, images, or audio, are encoded in binary. For instance, the letter 'A' is represented as 01000001 in ASCII code. Images are stored as a matrix of pixels, each pixel represented by a combination of bits that define its color.
Sound is digitized by sampling the audio signal at regular intervals and converting each sample into binary form. For instance, a CD audio track samples the sound at 44,100 times per second (44.1 kHz), and each sample is a 16-bit binary number. Videos are a series of frames (images) displayed in quick succession, with each frame being a grid of pixels encoded in binary.
Assembly and High-Level Languages
The Low-Level: Assembly Language
Before diving into high-level programming, it’s crucial to understand the bridge between human-readable code and machine code: assembly language.
Machine Code: Directly executed by the CPU, machine code is written in binary. A simple operation like adding two numbers might look like a long string of 0s and 1s.
Machine code is highly efficient but incredibly difficult for humans to read and write. Each instruction in machine code is a series of binary digits that directly correspond to specific operations the CPU can perform.
Assembly Language: A step above machine code, it uses mnemonic codes and labels instead of binary, making it slightly more readable (e.g.,
MOV A, B
). For example, an assembly instruction to add two numbers might look like this:MOV AL, 1 ; Load 1 into register AL ADD AL, 2 ; Add 2 to the value in AL
Assembly language is specific to each type of CPU architecture (e.g., x86, ARM) and provides a way to write programs that are closely tied to the hardware. Writing in assembly allows programmers to optimize performance-critical sections of code and manage hardware directly.
The High-Level: Programming Languages
High-level programming languages abstract away the complexities of machine code and assembly, making it easier for humans to write and understand code.
Python: Known for its readability and simplicity, Python is often used for web development, data analysis, and automation. For example, a simple Python program to add two numbers and print the result looks like this:
a = 1 b = 2 print(a + b) # Outputs: 3
Python's syntax is designed to be intuitive and mirrors natural language, making it an excellent choice for beginners and rapid development. Its extensive standard library and vibrant ecosystem make it suitable for a wide range of applications, from scripting to web development.
Java: Platform-independent and widely used in enterprise environments, Java’s "write once, run anywhere" capability makes it versatile. A basic Java program to accomplish the same task would be:
public class Main { public static void main(String[] args) { int a = 1; int b = 2; System.out.println(a + b); // Outputs: 3 } }
Java runs on the Java Virtual Machine (JVM), which allows it to execute on any platform that has the JVM installed. This makes Java highly portable and scalable. It's commonly used for building large-scale applications, including web applications, Android apps, and enterprise systems.
C++: Combining the efficiency of C with object-oriented features, C++ is used in system/software development and game programming. Here’s how you’d add two numbers in C++:
#include <iostream> using namespace std; int main() { int a = 1; int b = 2; cout << a + b << endl; // Outputs: 3 return 0; }
C++ provides low-level memory manipulation capabilities along with high-level abstractions, making it suitable for performance-critical applications. It is widely used in developing operating systems, game engines, and real-time simulation systems.
Data Structures and Algorithms
Data Structures: Organizing Information
Data structures are ways of organizing and storing data so it can be accessed and modified efficiently.
Arrays: Fixed-size lists that store elements of the same type. Example: an array of integers
[1, 2, 3, 4, 5]
.Arrays provide fast access to elements using an index but have a fixed size once created. They are useful for storing collections of data that won't change in size. Arrays are often used for tasks like storing pixel values in images or maintaining a fixed list of items.
Linked Lists: Collections of nodes that together form a sequence, where each node points to the next. Unlike arrays, linked lists allow for efficient insertion and deletion of elements. Example:
struct Node { int data; Node* next; };
Linked lists come in various forms, such as singly linked lists (each node points to the next) and doubly linked lists (each node points to both the next and the previous nodes). They are useful for dynamic data structures where elements need to be frequently added or removed.
Trees: Hierarchical structures with a root node and child nodes, used in databases and file systems. A common type of tree is the binary tree, where each node has at most two children. Example:
struct TreeNode { int data; TreeNode* left; TreeNode* right; };
Trees are used to represent hierarchical relationships. Binary search trees (BSTs) are a type of tree that allows for fast lookup, insertion, and deletion of elements. They are commonly used in databases to index data and in file systems to organize files and directories.
Graphs: Collections of nodes and edges representing relationships, used in network routing and social networks. Example:
struct GraphNode { int data; vector<GraphNode*> neighbors; };
Graphs can be directed (edges have a direction) or undirected (edges have no direction) and are used to model complex relationships between entities. Applications of graphs include social networks, where nodes represent users and edges represent connections, and network routing, where nodes represent routers and edges represent communication links.
Algorithms: The Recipes for Problem Solving
Algorithms are step-by-step procedures for solving problems and performing tasks.
Sorting Algorithms: Methods like Quick Sort and Merge Sort organize data in a specific order. Quick Sort, for example, works by selecting a 'pivot' element and partitioning the array around the pivot.
Quick Sort:
def quicksort(arr): if len(arr) <= 1: return arr pivot = arr[len(arr) // 2] left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot] right = [x for x in arr if x > pivot] return quicksort(left) + middle + quicksort(right)
Merge Sort:
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = merge_sort(arr[:mid]) right = merge_sort(arr[mid:]) return merge(left, right) def merge(left, right): result = [] i = j = 0 while i < len(left) and j < len(right): if left[i] < right[j]: result.append(left[i]) i += 1 else: result.append(right[j]) j += 1 result.extend(left[i:]) result.extend(right[j:]) return result
Sorting algorithms are fundamental for tasks like organizing lists, preparing data for binary search, and optimizing storage operations.
Searching Algorithms: Techniques like Binary Search efficiently locate items within sorted data. Binary Search divides the array into halves to reduce the search interval quickly.
Binary Search:
def binary_search(arr, target): left, right = 0, len(arr) - 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: left = mid + 1 else: right = mid - 1 return -1
Searching algorithms are crucial for tasks such as finding specific records in a database, looking up values in a sorted list, and optimizing search operations in large datasets.
Dynamic Programming: A method for solving complex problems by breaking them down into simpler subproblems, optimizing for performance. The Fibonacci sequence is a classic example, where each term is the sum of the two preceding ones.
Fibonacci Sequence:
def fibonacci(n): fib = [0, 1] for i in range(2, n+1): fib.append(fib[i-1] + fib[i-2]) return fib[n]
Dynamic programming is used in optimization problems such as shortest path algorithms (e.g., Dijkstra's algorithm), sequence alignment in bioinformatics, and resource allocation problems.
The Operating System: The Conductor of the Orchestra
An Operating System (OS) manages hardware resources and provides common services for computer programs.
Process Management: The OS handles the creation, scheduling, and termination of processes. Each running application is a process. For example, when you open a browser, the OS creates a process for it.
The OS uses process scheduling algorithms to manage CPU time. Common algorithms include First-Come, First-Served (FCFS), Shortest Job Next (SJN), and Round Robin (RR). These algorithms balance the load on the CPU and ensure that all processes get a fair share of processing time.
Memory Management: It keeps track of each byte in a computer’s memory and manages the allocation and deallocation of memory spaces. The OS ensures that each application has enough memory to function while maintaining system stability.
Virtual memory is a technique that allows the execution of processes that may not be completely in memory. It extends physical memory onto a disk, allowing larger programs to run on systems with limited RAM. The OS uses paging and segmentation to manage virtual memory, swapping data between RAM and disk as needed.
File Systems: The OS manages files on different storage devices, ensuring data is stored and retrieved efficiently. For example, NTFS on Windows and ext4 on Linux.
File systems organize data into files and directories, providing a hierarchical structure for storage. They also manage metadata, such as file permissions and timestamps. Different file systems have different features, such as journaling (to prevent data corruption) and support for large files.
For example, NTFS (New Technology File System) on Windows supports file compression, encryption, and large volume sizes. On the other hand, ext4 (Fourth Extended File System) on Linux provides robust performance, large file support, and journaling capabilities.
Networking and the Internet
Networking: Connecting Computers
Networking involves connecting multiple computers to share resources and information.
LAN (Local Area Network): A network that connects computers within a limited area, like a home or office. For example, your home Wi-Fi network.
LANs use technologies such as Ethernet and Wi-Fi to connect devices. Ethernet cables connect devices directly, while Wi-Fi uses radio waves for wireless communication. LANs enable resource sharing, such as printers and files, within a localized area.
WAN (Wide Area Network): A network that covers a broad area, such as a city, country, or even globally. The Internet is the largest WAN.
WANs use technologies such as fiber optics, satellite links, and microwave transmission to connect geographically dispersed networks. ISPs (Internet Service Providers) provide access to WANs, allowing users to connect to the Internet from virtually anywhere.
For instance, the Internet is a global network of networks, linking millions of private, public, academic, business, and government networks. It's a critical infrastructure for communication, commerce, and information sharing.
The Internet: A Network of Networks
The Internet is a global system of interconnected computer networks.
TCP/IP (Transmission Control Protocol/Internet Protocol): The fundamental protocol suite for Internet communication. TCP ensures reliable transmission, while IP handles addressing and routing.
TCP handles data packetization, error checking, and reassembly, ensuring data is transmitted accurately. IP addresses uniquely identify devices on the network, and routers use these addresses to forward packets to their destinations. For example, when you send an email, TCP/IP ensures it reaches the recipient's mail server.
TCP/IP operates at multiple layers:
Application Layer: Includes protocols like HTTP (for web browsing), FTP (for file transfer), and SMTP (for email).
Transport Layer: Provides end-to-end communication services (e.g., TCP, UDP).
Internet Layer: Manages logical addressing and routing (e.g., IP).
Link Layer: Handles physical addressing and media access (e.g., Ethernet).
DNS (Domain Name System): Translates human-readable domain names (like www.example.com) into IP addresses. For instance, DNS resolves
www.google.com
to its IP address so your browser can connect to Google's servers.DNS operates in a hierarchical manner, with root servers at the top level, followed by top-level domain (TLD) servers, and authoritative servers for specific domains. When you enter a URL in your browser, a DNS query is sent to resolve the domain name to an IP address, enabling the connection to the web server.
The DNS system is distributed and relies on a network of DNS servers worldwide to provide fast and reliable domain resolution. For example, Google's public DNS servers (8.8.8.8 and 8.8.4.4) offer a free and reliable DNS resolution service.
Databases
Relational Databases: Structured Data Storage
SQL (Structured Query Language) is used to manage and manipulate relational databases, which store data in tables.
Tables: Consist of rows and columns, where each row represents a record, and each column represents a field. For example, a table of students might have columns for ID, name, and age.
Tables are linked by primary and foreign keys, establishing relationships between data. This relational model allows for complex queries and data integrity. For example, a database for an online store might have tables for customers, orders, and products, with relationships linking orders to customers and products.
Queries: SQL commands used to retrieve and manipulate data. For example, the query
SELECT * FROM students WHERE age > 20;
retrieves all students older than 20.SQL supports various operations, including:
SELECT: Retrieve data from one or more tables.
INSERT: Add new records to a table.
UPDATE: Modify existing records.
DELETE: Remove records.
SQL also supports advanced features like joins (to combine data from multiple tables), transactions (to ensure data consistency), and indexing (to improve query performance). For example, a join query might combine data from the orders and customers tables to retrieve customer information for each order.
NoSQL Databases: Flexibility and Scalability
NoSQL databases provide a flexible schema design and are ideal for handling unstructured data.
Document Stores: Store data in JSON-like documents. For example, MongoDB stores records as documents with fields and values:
{ "name": "John Doe", "age": 25, "courses": ["Math", "Science"] }
Document stores allow for dynamic schemas, meaning fields can vary between documents. This flexibility is useful for applications with evolving data models. For example, a content management system might store articles with varying structures and metadata.
Key-Value Stores: Simple databases where each item is a pair consisting of a key and a value. For example, Redis stores key-value pairs like
"user:1000": "John Doe"
.Key-value stores provide fast access to data and are suitable for caching and real-time applications. They are often used for session management, shopping carts, and user profiles. For example, an e-commerce site might use a key-value store to quickly retrieve product details based on product IDs.
Column-Family Stores: Organize data into columns rather than rows. Each column family contains rows with a unique key. Example: Apache Cassandra.
Column-family stores are optimized for read and write performance on large datasets. They are used in applications requiring high availability and scalability, such as recommendation engines and real-time analytics.
Graph Databases: Designed to store and query relationships between entities. Example: Neo4j.
Graph databases use nodes, edges, and properties to represent and store data, making them ideal for applications like social networks, fraud detection, and network management.
Cybersecurity
Encryption: Securing Data
Encryption transforms data into a coded format that can only be read by someone with the right decryption key.
Symmetric Encryption: Uses the same key for both encryption and decryption. Example: AES (Advanced Encryption Standard).
Symmetric encryption is efficient but requires secure key distribution. If the key is compromised, the data can be decrypted by unauthorized parties. AES is widely used for encrypting sensitive data, such as files and communication channels.
Asymmetric Encryption: Uses a pair of keys – a public key for encryption and a private key for decryption. Example: RSA (Rivest-Shamir-Adleman).
Asymmetric encryption allows for secure communication without sharing a secret key. The public key is widely distributed, while the private key is kept secure. RSA is commonly used for secure data transmission, digital signatures, and key exchange.
Defenses: Firewalls and Ethical Hacking
Firewalls: Act as barriers between a trusted network and an untrusted network, controlling incoming and outgoing traffic. Firewalls can be hardware-based or software-based.
Firewalls use rules to filter traffic, allowing or blocking packets based on criteria such as IP addresses, port numbers, and protocols. For example, a firewall might block traffic from known malicious IP addresses or restrict access to certain ports.
There are different types of firewalls, including:
Packet-Filtering Firewalls: Examine individual packets and make decisions based on header information.
Stateful Inspection Firewalls: Track the state of active connections and make decisions based on the context of the traffic.
Application-Layer Firewalls: Inspect the payload of packets and make decisions based on the application data.
Ethical Hacking: Security experts test systems for vulnerabilities to ensure they are protected against malicious attacks. Ethical hackers use the same tools and techniques as malicious hackers but in a legal and authorized manner.
Ethical hacking involves penetration testing, vulnerability assessments, and security audits to identify and mitigate potential security risks. Ethical hackers follow a structured process:
Reconnaissance: Gathering information about the target.
Scanning: Identifying potential entry points.
Exploitation: Attempting to exploit vulnerabilities.
Reporting: Documenting findings and providing recommendations.
Organizations often conduct regular penetration tests to ensure their defenses are up to date and effective. For example, an ethical hacker might test a web application for SQL injection vulnerabilities, cross-site scripting (XSS), and insecure authentication mechanisms.
Cloud Computing, Blockchain, and AI
Cloud Computing: Beyond the Physical
Cloud computing delivers computing services over the Internet, allowing for scalable and flexible resources.
IaaS (Infrastructure as a Service): Provides virtualized computing resources over the Internet. Example: Amazon Web Services (AWS) EC2.
IaaS offers virtual machines, storage, and networking infrastructure on demand. Users can scale resources up or down based on their needs. For example, a startup might use IaaS to quickly deploy servers for a new application without investing in physical hardware.
PaaS (Platform as a Service): Offers hardware and software tools over the Internet. Example: Google App Engine.
PaaS provides a platform for developing, testing, and deploying applications. It abstracts the underlying infrastructure, allowing developers to focus on coding. For example, a development team might use PaaS to streamline the deployment of a web application, with built-in support for databases, authentication, and monitoring.
SaaS (Software as a Service): Delivers software applications over the Internet. Example: Google Workspace (formerly G Suite).
SaaS applications are accessible via web browsers and typically follow a subscription model. Users benefit from automatic updates and maintenance. For example, businesses use SaaS applications for email, collaboration, customer relationship management (CRM), and enterprise resource planning (ERP).
Cloud computing offers several benefits:
Scalability: Easily adjust resources to meet demand.
Cost Efficiency: Pay for what you use, reducing upfront capital expenses.
Accessibility: Access services from anywhere with an Internet connection.
Reliability: Cloud providers offer high availability and disaster recovery options.
Blockchain: The Immutable Ledger
Blockchain is a decentralized digital ledger that records transactions across many computers in a way that ensures security and transparency.
Cryptocurrencies: Digital or virtual currencies using cryptography for security. Example: Bitcoin, which uses blockchain to secure transactions.
Cryptocurrencies operate on decentralized networks, eliminating the need for intermediaries like banks. Transactions are recorded in blocks, which are linked to form a chain. Each block contains a cryptographic hash of the previous block, a timestamp, and transaction data. This structure ensures the integrity and immutability of the blockchain.
Smart Contracts: Self-executing contracts with the terms directly written into code. Example: Ethereum, which allows for complex smart contracts.
Smart contracts automate the execution of agreements, ensuring that conditions are met before actions are taken. They enable trustless transactions and reduce the need for intermediaries. For example, a smart contract could be used in a crowdfunding campaign to release funds to a project only if a certain funding goal is reached by a specific date.
Blockchain technology has applications beyond cryptocurrencies:
Supply Chain Management: Track and verify the origin and journey of goods.
Voting Systems: Ensure secure and transparent elections.
Healthcare: Manage patient records securely and enable interoperability.
Intellectual Property: Protect and manage digital assets and rights.
Artificial Intelligence and Machine Learning
AI and ML are transforming how we interact with technology.
Supervised Learning: Machines learn from labeled data to make predictions or decisions. Example: Training a model to recognize cats in photos.
Supervised learning involves feeding the model input-output pairs and adjusting its parameters to minimize errors. Techniques include linear regression, decision trees, and neural networks. For example, a supervised learning model could predict house prices based on features like size, location, and age.
Unsupervised Learning: Machines find hidden patterns in unlabeled data. Example: Grouping customers based on purchasing behavior.
Unsupervised learning algorithms, such as clustering and association, identify patterns and relationships within the data without predefined labels. For example, an e-commerce site might use unsupervised learning to segment customers into different groups based on their browsing and purchasing history.
Deep Learning: A subset of ML using neural networks to model complex patterns in data. Example: AlphaGo, the AI that defeated human champions in the game of Go.
Deep learning models, such as convolutional neural networks (CNNs) and recurrent neural networks (RNNs), consist of multiple layers that learn hierarchical representations of data. CNNs are particularly effective for image recognition tasks, while RNNs are used for sequence data, such as natural language processing (NLP) and time series forecasting.
AI and ML have numerous applications:
Healthcare: Diagnose diseases, personalize treatment plans, and discover new drugs.
Finance: Detect fraud, automate trading, and provide personalized financial advice.
Transportation: Enable autonomous vehicles and optimize logistics.
Customer Service: Power chatbots and virtual assistants.
Conclusion
And there you have it – A quick tour of the vast and intricate world of computer science. From the foundational hardware to the cutting-edge frontiers of AI and blockchain, we've explored the essential components and principles that drive this ever-evolving field. As you continue your journey, remember that computer science is a landscape rich with opportunities for discovery and innovation. Happy coding!
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
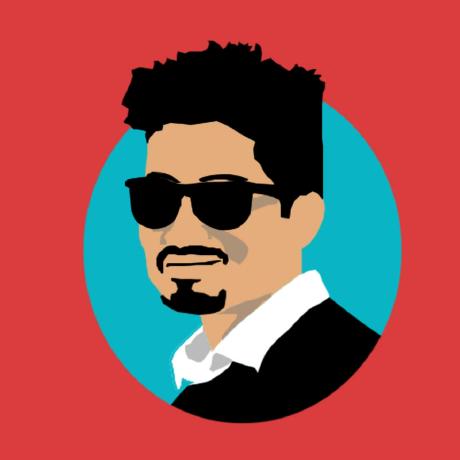