(lt.49) Master React Development: Introduction to Core Concepts (SPA, JSX, Components & More

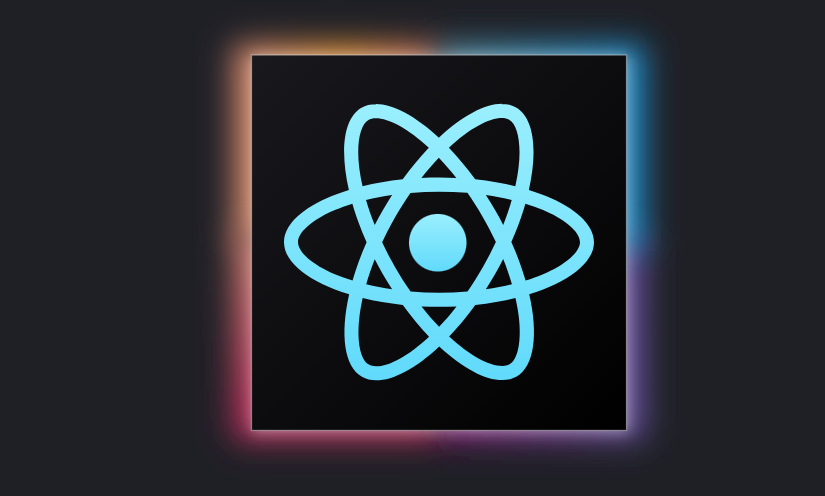
Introduction
React is a popular JavaScript library for building user interfaces, particularly for single-page applications where you want a fast and interactive user experience.
React is widely used in web development for creating dynamic and responsive web applications. It is also the basis for React Native, which allows developers to build mobile applications using the same principles and components as React for web.
Single Page Application (SPA)
It is a web application that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from the server. This approach provides a more fluid and responsive user experience, similar to a desktop application.
Key features
Single-page experience: Loads once, updates content dynamically.
JavaScript-powered interactions: No full page reloads, feels faster and smoother.
Client-side routing: Manages navigation within the app using JavaScript.
Asynchronous data fetching: Retrieves data without blocking the UI.
Enhanced UX: Faster transitions, more interactive and responsive.
Creating react elements manually
Goto react cdn and copy the code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>react</title>
</head>
<body>
<!-- html elements make a dom node actually -->
<div id="root">
</div>
<script crossorigin src="https://unpkg.com/react@18/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@18/umd/react-dom.development.js"></script>
<script>
// react dosent create a dom node
// react element is json object which defines the property of elements
// react element
/*
{type: 'button',
props:
{
children :'submit',
classname :''
}
}
*/
const button= ()=>
React.createElement("button" ,{id :'btnn'}, React.createElement("spann" ,{} ,"submit"))
// for react to render on a web page we have to create a html tag with id
const rootcont = document.getElementById('root')
const root1 = ReactDOM.createRoot(rootcont)
root1.render(button())
</script>
</body>
</html>
Setting up react using vite and create react app
run command
npx create-react-app my-app
sometimes this command may show error then to solve it:
update to latest version of node.js : npm install -g npm
Clear npm Cache: npm cache clean
then run the above command again
then npm start
using vite
run the command : npm create vite@latest
coding demonstration to understand it more easily
npm create vite@latest
Need to install the following packages:
create-vite@5.3.0
Ok to proceed? (y)
> npx
> create-vite
√ Project name: ... vite-react
? Select a framework: » - Use arrow-keys. Return to submit.
> Vanilla
? Select a framework: » - Use arrow-keys. Return to submit.
Vanilla
? Select a framework: » - Use arrow-keys. Return to submit.
Vanilla
√ Select a framework: » React
√ Select a variant: » JavaScript
Now run:
cd vite-react
npm install
npm run dev
Local: http://localhost:513/
What is jsx
(JavaScript XML) is a syntax extension for JavaScript that is commonly used with React to describe what the UI should look like. It allows developers to write HTML-like code within JavaScript, which makes it easier to visualize and write the structure of the user interface.
JSX allows developers to create dynamic, interactive user interfaces with ease. While it requires a build step to transpile into standard JavaScript, the benefits it brings to the development process make it an essential part of React development.
What is a transpile
Transpiling, short for "transformation" and "compiling," refers to the process of converting source code written in one programming language into another language that has a similar level of abstraction. This is different from compiling, which typically converts high-level code to a lower-level language
Examples of Transpilers
Babel: Babel is a popular JavaScript transpiler that converts ES6/ES7 code into ES5. It allows developers to use modern JavaScript features while ensuring compatibility with older environments.
TypeScript Compiler (tsc): TypeScript is a statically typed superset of JavaScript. The TypeScript compiler converts TypeScript code into JavaScript.
SCSS to CSS: SCSS is a preprocessor scripting language that is interpreted or compiled into CSS. Tools like Sass convert SCSS to standard CSS.
Benefits of Transpiling
Backward Compatibility: Transpiling allows developers to use modern language features and ensure that the code runs in older environments that do not support these features natively.
Enhanced Productivity: By using modern syntax and language features, developers can write cleaner, more maintainable code more efficiently.
Cross-Language Interoperability: Transpilers enable code written in one language to run in an environment designed for another language, broadening the scope of where and how the code can be used.
understanding components in jsx
A component is a fundamental building block used to define the structure and behavior of a user interface in React applications. Components make it easier to break down complex UIs into smaller, reusable pieces.
{html + css + js} = component
Types of Components
Functional Components: These are simple JavaScript functions that return JSX. They are ideal for presenting static content or UI that does not require state management.
Class Components: These are ES6 classes that extend React.Component and can manage their own state and lifecycle methods. They are more powerful than functional components but are being gradually replaced by functional components .
Since html elements and react components have same structure , so to distinguish between an html element and the react component is that: the name of react component should start from capital letter.
We can't return two elements together so if we have to return more then one element then we have to make a parent element and then return it because react except elements to be wrapped inside single parent .function
function DogCard()
{
return (
// if we cant return in single line then we have to use the parenthesis
<div>
<h3>Shadow</h3>
<img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
</div>
);
}
Import and export components
Importing and exporting components is a fundamental part of working with React and JSX. It allows you to organize your code into modular, reusable pieces
Named Export
Named exports allow you to export multiple components or variables from a single file. You need to import them using the same names.
import React from 'react';
export function Header() {
return <h1>This is the header</h1>;
}
export function Footer() {
return <footer>This is the footer</footer>;
}
Default Export
Default export is used to export a single component or value from a file. It allows you to import the component with any name.
import React from 'react';
function Header() {
return <h1>This is the header</h1>;
}
export default Header;
Named Import
When using named exports, you must import the components with their exact names.
mport React from 'react';
import { Header, Footer } from './components';
function App() {
return (
<div>
<Header />
<Footer />
</div>
);
}
export default App;
Coding demonstration
The below coding demonstration shows how we can use different permutation and combination using these import and export
Create a file name dogcard.js
// function DogCard()
// {
// return (
// <div>
// <h3>Shadow</h3>
// <img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
// </div>
// );
// }
/*+++++++++++++++++++++ Method 2 alternate of the above method+++++++++++++++++++++++++++++++++++++++++++++++++*/
// function Image ()
// {
// return <img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
// }
// function DogCard()
// {
// return (
// <div>
// <h3>Shadoww</h3>
// <Image/>
// </div>
// );
// }
// export default DogCard;
/*+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ */
// // using named export method
// export function Image ()
// {
// return <img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
// }
// export function DogCard()
// {
// return (
// <div>
// <h3>Shadoww</h3>
// <Image/>
// </div>
// );
// }
// // named and default export both are used together
export function Image ()
{
return <img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
}
function DogCard()
{
return (
<div>
<h3>Shadoww</h3>
<Image/>
</div>
);
}
export default DogCard;
another file name app.js
// import DogCard from './DogCard';
// // the below is jsx not an html tag
// function App() {
// return (
// <DogCard/>
// );
// }
// export default App;
/*++++++++++++++++++++++++++++++++++++++++++++++++++++++++ */
// code when named export is used
// import { Image, DogCard } from "./DogCard";
// // the below is jsx not an html tag
// function App() {
// return <DogCard />;
// }
// export default App;
/*+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ */
// code when both named and default methods are used
import DogCard, { Image } from "./DogCard";
function App()
{
return(
<div>
<DogCard />;
{/* <Image /> */}
</div>
);
}
export default App;
The problem is that if we have to return more then one element then we have to make a parent element mostly <div> but the problem is that <div> tag takes extra space in dom and sometimes can overwrite the css applied .
Therefore to counter it we can use empty closing and opening tag which is called fragment.
function DogCard()
{
return (
<div>
<h3>Shadow</h3>
<img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
</div>
);
}
// using opening nd closing
function DogCard()
{
return (
<>
<h3>Shadow</h3>
<img src="https://t4.ftcdn.net/jpg/06/02/74/39/360_F_602743936_qbTuk7bb34cSYBgSDbsirlmJSbxRBUFM.jpg" />
</>
);
}
Props
In JSX, props (short for "properties") are a way to pass data from parent components to child components. Props allow components to be dynamic and reusable by providing them with different data. They are read-only and should not be modified by the child component.
function Image(prop)
{
return(
<div>
<img src={prop.src}/>
</div>
);
}
export default Image;
Important
cause: In JavaScript, if you write a return statement without anything on the same line, it automatically inserts a semicolon, causing the function to return undefined.
Code to demonstrate Props
Index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
{/* <App /> */}
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
DogCard.js
import Image from "./Image";
function DogCard(props)
{
return(
<>
<h3>{props.name}</h3>
<Image src ={props.Image} />
<Image src ={props.image} />
</>
)
}
export default DogCard
App.js
import DogCard, { Image } from "./DogCard";
function App()
{
return(
<div>
<DogCard name = "tiger" Image ="https://cdn.wallpapersafari.com/65/9/Owx3Du.jpg"/>;
<DogCard name = "yalk" Image="https://images.pexels.com/photos/26050467/pexels-photo-26050467/free-photo-of-a-black-and-white-photo-of-a-highland-cow.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=1"/>;
<DogCard name = "tiger" image="https://files.worldwildlife.org/wwfcmsprod/images/Tiger_resting_Bandhavgarh_National_Park_India/hero_small/6aofsvaglm_Medium_WW226365.jpg"/>;
</div>
);
}
export default App;
Image.js
function Image(props)
{
return(
<div>
<img src={props.src}/>
</div>
);
}
export default Image;
// second method to write above code
function Image({src =100}) // src =100 default
{
return(
<div >
<img src={src}/>
</div>
);
}
export default Image;
lt.49: https://hashnode.com/preview/668f8bb8ed2bf788ae4d1961
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
