Asynchronous Programming in Python: Enhancing Efficiency and Performance
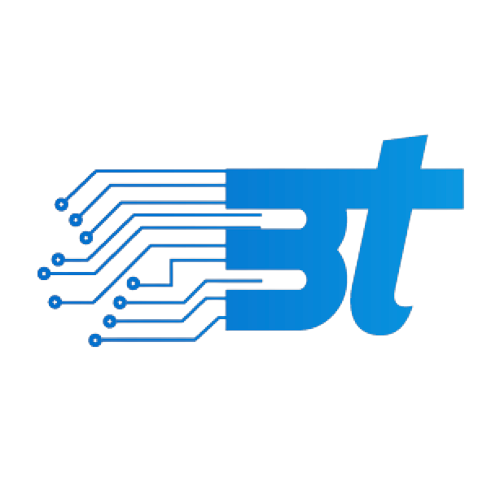
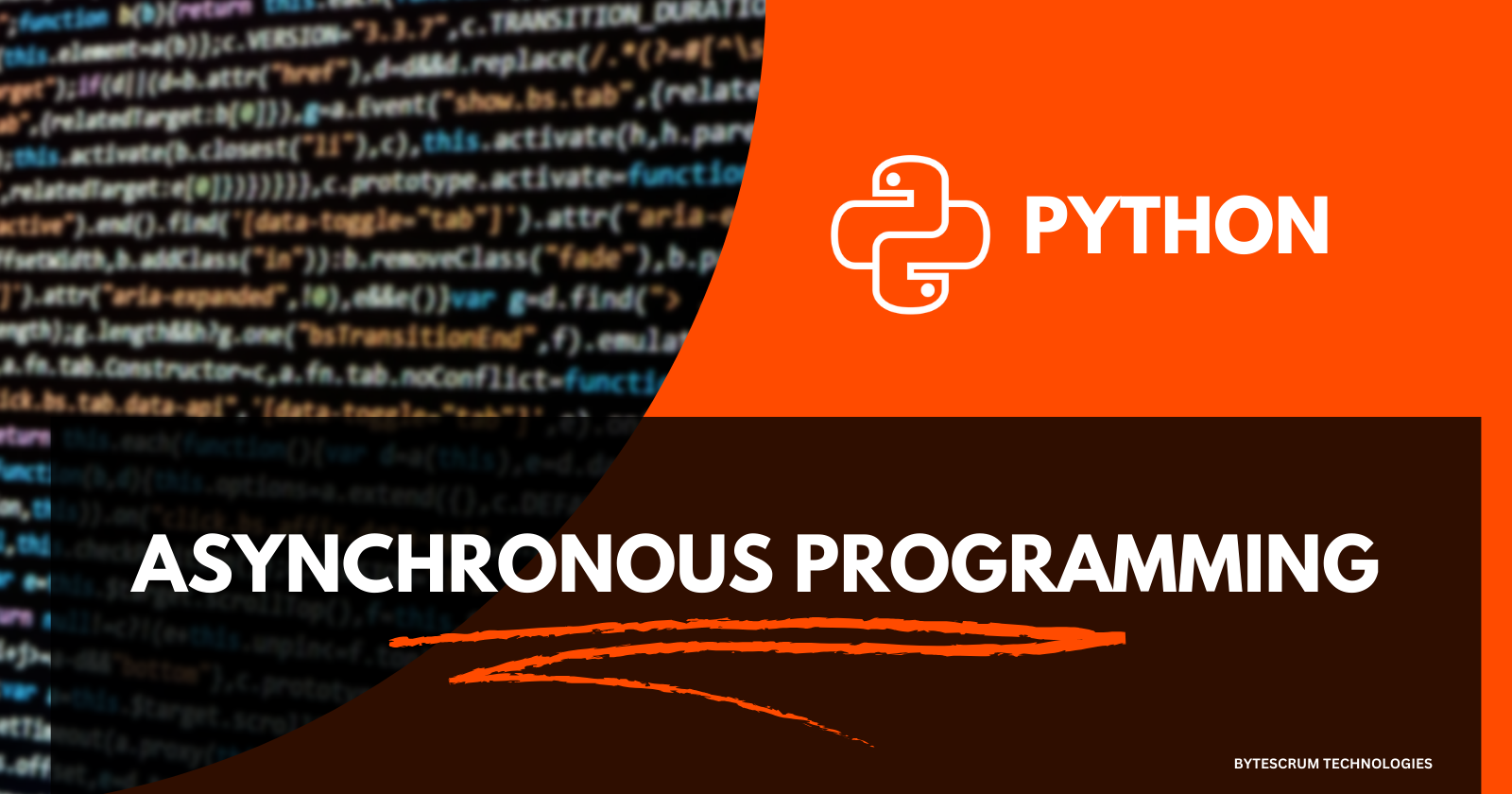
In modern software development, efficiently handling tasks that involve waiting for external resources, such as network responses or file I/O operations, is crucial. Asynchronous programming allows your program to continue executing other tasks while waiting for these operations to complete, thus improving the overall performance and responsiveness. In this blog, we'll explore the fundamentals of asynchronous programming in Python, including its setup, key concepts, and practical examples.
What is Asynchronous Programming?
Asynchronous programming is a paradigm that allows multiple tasks to run concurrently without waiting for each task to complete before starting the next one. This is particularly useful for I/O-bound tasks, such as network requests, file operations, or database queries, where the program can perform other operations while waiting for these tasks to complete.
Why Use Asynchronous Programming?
Improved Performance: Asynchronous programming can significantly improve the performance of I/O-bound applications by allowing them to handle multiple operations concurrently.
Enhanced Responsiveness: By not blocking the main thread, asynchronous programming can keep applications responsive, providing a better user experience.
Resource Efficiency: Asynchronous tasks can make better use of system resources by not idling while waiting for I/O operations to complete.
Setting Up Asynchronous Programming in Python
Python provides built-in support for asynchronous programming with the asyncio
module, which includes tools for managing asynchronous tasks, coroutines, and event loops.
Prerequisites
- Python 3.6 or later installed on your machine. You can download it from python.org.
Key Concepts in Asynchronous Programming
1. Coroutines
Coroutines are special functions that can pause and resume their execution. They are defined using the async def
syntax.
import asyncio
async def my_coroutine():
print("Hello")
await asyncio.sleep(1)
print("World")
# Running the coroutine
asyncio.run(my_coroutine())
2. The Event Loop
The event loop manages the execution of asynchronous tasks, scheduling them to run when they are ready.
async def main():
print("Start")
await asyncio.sleep(1)
print("End")
# Running the event loop
asyncio.run(main())
3. Tasks
Tasks are used to schedule coroutines concurrently.
async def my_coroutine():
print("Start")
await asyncio.sleep(1)
print("End")
async def main():
task1 = asyncio.create_task(my_coroutine())
task2 = asyncio.create_task(my_coroutine())
await task1
await task2
asyncio.run(main())
Practical Examples of Asynchronous Programming
Example 1: Asynchronous HTTP Requests
Using the aiohttp
library to make asynchronous HTTP requests.
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
url = 'https://example.com'
html = await fetch(url)
print(html)
asyncio.run(main())
Example 2: Asynchronous File I/O
Using the aiofiles
library to read and write files asynchronously.
import aiofiles
import asyncio
async def read_file(file_path):
async with aiofiles.open(file_path, mode='r') as file:
contents = await file.read()
print(contents)
async def write_file(file_path, content):
async with aiofiles.open(file_path, mode='w') as file:
await file.write(content)
async def main():
await write_file('example.txt', 'Hello, world!')
await read_file('example.txt')
asyncio.run(main())
Example 3: Asynchronous Database Operations
Using aiomysql
to perform asynchronous database operations.
import asyncio
import aiomysql
async def fetch_data():
conn = await aiomysql.connect(host='localhost', port=3306, user='root', password='password', db='test_db')
async with conn.cursor() as cur:
await cur.execute("SELECT * FROM my_table")
result = await cur.fetchall()
print(result)
conn.close()
asyncio.run(fetch_data())
Handling Exceptions in Asynchronous Code
Handling exceptions in asynchronous code is similar to synchronous code, but with the added complexity of tasks and coroutines.
async def my_coroutine():
try:
await asyncio.sleep(1)
raise ValueError("An error occurred")
except ValueError as e:
print(f"Caught an exception: {e}")
asyncio.run(my_coroutine())
Conclusion
asyncio
module and other asynchronous libraries, you can write efficient, non-blocking code that makes better use of system resources. Whether you're building web applications, performing data processing, or handling network operations, mastering asynchronous programming can significantly enhance your development workflow.Happy Coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
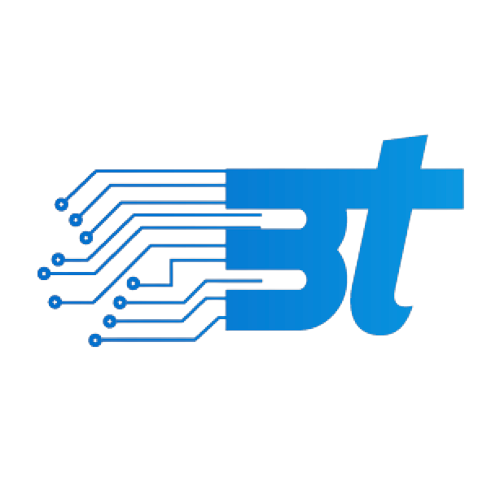
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.