10 Steps to Build Your First Web Application Using Python Flask
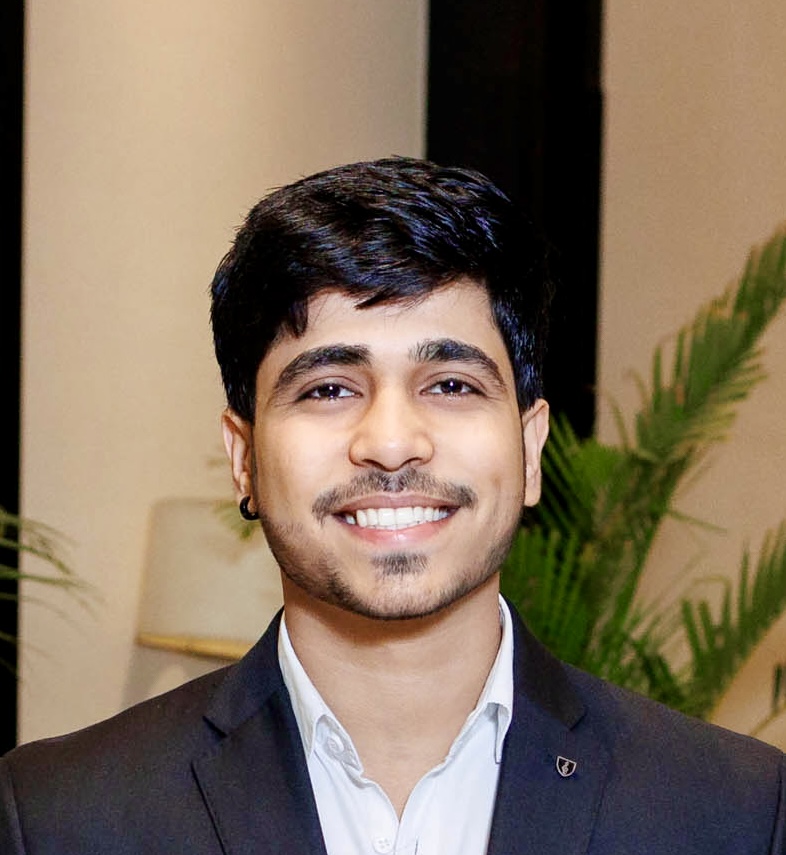
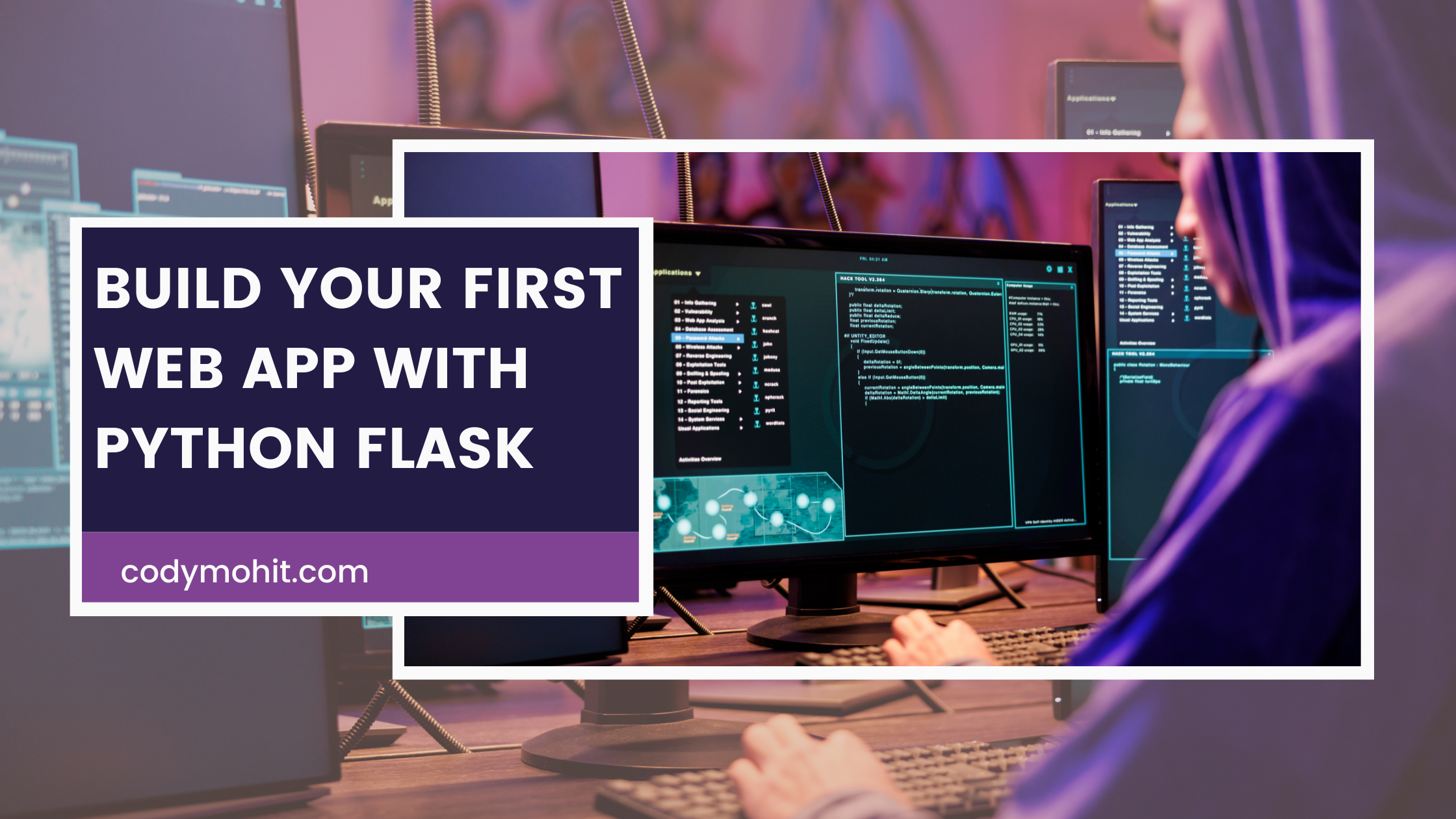
Hey there, tech enthusiasts! 🌟 Are you ready to dive into the world of web development with Python Flask? Building your first web application might seem like a daunting task, but trust me, it's easier than you think! Whether you're a seasoned coder or just starting out, this guide will walk you through 10 easy steps to get your first Flask web app up and running. From setting up your environment to deploying your masterpiece online, we've got you covered. So grab your favorite coding snack, and let's get started on this exciting journey together. Let's code some magic! 💻✨
What is Flask?
Flask is a popular web framework for Python. It’s simple and lightweight, designed to make it easy to get started with web development. Flask doesn’t come with many built-in features, instead, it gives you the tools to build a web app and lets you add only what you need. Think of Flask as a toolbox that gives you the essentials to create web applications, and you can add more tools as you need them.
Why choose Flask for web development?
There are several reasons why developers might choose Flask:
Simplicity and Flexibility: Flask is easy to understand and use, especially for beginners. It doesn’t force you into a specific project structure or require you to use certain tools or libraries. You have the freedom to structure your project the way you want.
Lightweight: Flask is lightweight, meaning it doesn’t come with many pre-built features. This is great because it means there is less bloat and you can keep your application lean and efficient.
Extensibility: Flask allows you to add extensions for additional functionality, like form validation, database interaction, and authentication. You only add what you need, which keeps your application simple.
Community and Documentation: Flask has a large and active community. This means you can find plenty of tutorials, documentation, and third-party libraries to help you with your project.
Benefits of using Flask for beginners
Easy to Learn: Flask's simplicity and straightforwardness make it an excellent choice for beginners. The framework itself is not too complicated, and you can start building web applications quickly.
Minimal Setup: Flask requires minimal setup. You can start a new project in just a few minutes, and there are no complicated configurations to worry about.
Encourages Best Practices: Flask’s design encourages best practices in web development. For example, it promotes the use of blueprints to organize your application and follows the principles of RESTful API design.
Clear Documentation: Flask has excellent documentation that is beginner-friendly. This helps new developers understand the framework and find answers to their questions easily.
Support for Testing: Flask supports testing and debugging out of the box, which is important for beginners learning how to develop robust and reliable applications.
In conclusion, Flask is a great choice for web development, especially for beginners. Its simplicity, flexibility, and strong community support make it a powerful tool for building web applications. Plus, you have the freedom to add only the features you need, keeping your project clean and efficient.
Step 1: Setting Up Your Development Environment
Installing Python
How to Check if Python is Already Installed
Before installing Python, you can check if it is already installed on your system:
On Windows:
Open the Command Prompt (press
Win + R
, typecmd
, and hit Enter).Type
python --version
orpython -V
and press Enter.If Python is installed, you will see the version number. If not, you'll see an error message.
On macOS:
Open the Terminal (press
Cmd + Space
, typeTerminal
, and hit Enter).Type
python --version
orpython3 --version
and press Enter.If Python is installed, you will see the version number. If not, you'll see an error message.
On Linux:
Open the Terminal.
Type
python --version
orpython3 --version
and press Enter.If Python is installed, you will see the version number. If not, you'll see an error message.
Steps to Install Python on Different Operating Systems
On Windows:
Go to the Python downloads page.
Download the latest version of Python.
Run the downloaded installer.
During installation, ensure you check the option "Add Python to PATH".
Click "Install Now" and follow the prompts to complete the installation.
On macOS:
Open the Terminal.
Install Homebrew if you haven't already, by pasting the following command and pressing Enter:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Once Homebrew is installed, install Python by typing:
codebrew install python
Follow the prompts to complete the installation.
On Linux:
Open the Terminal.
For Debian-based systems (like Ubuntu), type:
sudo apt update sudo apt install python3
For Red Hat-based systems (like Fedora), type:
sudo dnf install python3
Follow the prompts to complete the installation.
Setting Up a Virtual Environment
Importance of Virtual Environments
Virtual environments are crucial for several reasons:
Isolation: They isolate your project dependencies, ensuring that each project has its own set of dependencies, which prevents conflicts.
Version Management: You can use different versions of packages for different projects without interference.
Reproducibility: Virtual environments make it easier to reproduce the exact environment needed to run a project, aiding in collaboration and deployment.
Creating and Activating a Virtual Environment
Creating a Virtual Environment:
Open your terminal or command prompt.
Navigate to your project directory using
cd <project-directory>
.Type the following command to create a virtual environment:
python -m venv venv
Here,
venv
is the name of the virtual environment folder. You can name it anything you like.
Activating a Virtual Environment:
On Windows:
venv\Scripts\activate
On macOS and Linux:
source venv/bin/activate
Once activated, your terminal prompt will change to indicate that you are now working within the virtual environment.
Deactivating a Virtual Environment:
To deactivate the virtual environment, simply type:
deactivate
By following these steps, you can easily install Python and set up a virtual environment, ensuring your projects are well-organized and free from dependency conflicts.
Step 2: Installing Flask
Using pip to Install Flask
Basic pip Commands
pip
is a package installer for Python, and it's the preferred way to install packages from the Python Package Index (PyPI). Here are some basic pip
commands:
Install a package:
pip install package_name
Upgrade a package:
pip install --upgrade package_name
Uninstall a package:
pip uninstall package_name
List installed packages:
pip list
Installing Flask
To install Flask using pip
, open your terminal or command prompt and run the following command:
pip install Flask
Verifying the Installation of Flask
After installing Flask, you can verify the installation by running:
pip show Flask
This command displays information about the Flask package, including the version number, installation path, and dependencies.
You can also check the installed version of Flask by opening a Python shell and running:
import flask
print(flask.__version__)
Setting Up a Basic Flask Application
Creating Your First Flask App
Create a new directory for your project and navigate into it:
mkdir my_flask_app cd my_flask_app
Create a virtual environment (optional but recommended):
python -m venv venv
Activate the virtual environment:
On Windows:
venv\Scripts\activate
On macOS/Linux:
source venv/bin/activate
Install Flask within the virtual environment (if not already installed):
pip install Flask
Create a new Python file named
app.py
and add the following code:from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(debug=True)
Running the Flask Development Server
To run your Flask app, execute the following command in your terminal:
python app.py
You should see output similar to this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
* Restarting with stat
* Debugger is active!
* Debugger PIN: xxx-xxx-xxx
Open your web browser and navigate to http://127.0.0.1:5000/
to see your Flask app in action. You should see the text "Hello, World!" displayed in the browser.
That's it! You've successfully installed Flask, set up a basic Flask application, and run the Flask development server.
Step 3: Creating Your Project Structure
Organizing Your Flask Project
When developing a Flask application, organizing your project structure efficiently is crucial for maintainability and scalability. A well-structured project makes it easier to manage your code, collaborate with others, and scale your application as it grows. Here’s a recommended project structure for Flask applications, along with explanations of key directories and files.
Recommended Project Structure
my_flask_app/
│
├── app/
│ ├── __init__.py
│ ├── main/
│ │ ├── __init__.py
│ │ ├── routes.py
│ │ ├── forms.py
│ │ └── models.py
│ ├── static/
│ │ ├── css/
│ │ ├── js/
│ │ └── images/
│ ├── templates/
│ │ ├── base.html
│ │ └── index.html
│ └── extensions.py
│
├── tests/
│ ├── __init__.py
│ └── test_main.py
│
├── config.py
├── run.py
├── requirements.txt
└── README.md
Explanation of Key Directories and Files
app/
- init.py: This file initializes your Flask application and sets up the application factory pattern. It typically imports and registers Blueprints, extensions, and configurations.
from flask import Flask
def create_app():
app = Flask(__name__)
# Import and register Blueprints here
# Import and initialize extensions here
return app
main/: This subdirectory contains modules related to the main functionality of your application.
static/: This directory is for static files such as CSS, JavaScript, and images.
css/: Contains CSS files for styling.
js/: Contains JavaScript files.
images/: Contains image files.
templates/: This directory is for HTML templates.
base.html: A base template that other templates can extend.
index.html: An example of a specific page template.
extensions.py: This file is for initializing Flask extensions (e.g., database, login manager). It helps keep the
__init__.py
file clean.from flask_sqlalchemy import SQLAlchemy db = SQLAlchemy()
tests/
config.py: This file contains configuration settings for your application, such as database URIs, secret keys, and other settings. You can have different configurations for development, testing, and production.
class Config: SECRET_KEY = 'your_secret_key' SQLALCHEMY_DATABASE_URI = 'sqlite:///site.db'
run.py: The entry point for running your Flask application. It typically imports the application factory and runs the app.
from app import create_app app = create_app() if __name__ == '__main__': app.run(debug=True)
requirements.txt: This file lists the Python dependencies for your project. It allows you to recreate the environment easily.
Flask==2.0.1 Flask-SQLAlchemy==2.5.1
README.md: A markdown file that provides an overview of your project, including instructions for setting up and running the application. This is useful for other developers or contributors.
By organizing your Flask project in this manner, you create a modular and scalable structure that is easy to navigate and maintain. This approach follows best practices and helps ensure that your application can grow and evolve efficiently over time.
Step 4: Handling Routes and Views
Understanding Flask Routes
What are routes in Flask?
Routes in Flask are like directions for your web application. They tell your app how to respond when a user visits a specific URL. Think of routes as the addresses where your app "lives" online. Each route is linked to a function in your code that defines what should happen when someone visits that address.
For example, when someone goes to http://yourapp.com/home
, Flask looks for a route that matches /home
and then runs the function associated with it. This function can return a webpage, some data, or even a message.
Defining and handling routes in your application
To define a route in Flask, you use the @app.route
decorator. This decorator is placed above a function to specify which URL should trigger that function. Here’s a basic example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Welcome to the Home Page!'
@app.route('/about')
def about():
return 'About Us Page'
if __name__ == '__main__':
app.run(debug=True)
In this example, visiting http://127.0.0.1:5000/
will display "Welcome to the Home Page!", and visiting http://127.0.0.1:5000/about
will display "About Us Page".
Creating Views in Flask
Returning HTML content from routes
Sometimes, you want to return more than just plain text from your routes. You might want to return a full HTML page. Flask makes this easy. You can return HTML directly from your route functions.
@app.route('/contact')
def contact():
return '<h1>Contact Us</h1><p>This is the contact page.</p>'
When someone visits http://127.0.0.1:5000/contact
, they’ll see an HTML page with a heading and a paragraph.
Using templates to create dynamic content
Returning HTML directly from routes is okay for simple pages, but for more complex pages, it's better to use templates. Templates allow you to create dynamic content by separating your HTML structure from your Python code. Flask uses the Jinja2 templating engine to render these templates.
Here’s how you can use templates in Flask:
Create a folder named
templates
in your project directory.Create an HTML file inside the
templates
folder. Let's call itindex.html
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home</title>
</head>
<body>
<h1>{{ title }}</h1>
<p>{{ message }}</p>
</body>
</html>
- Modify your Flask route to render this template:
from flask import render_template
@app.route('/')
def home():
return render_template('index.html', title='Welcome', message='Hello, Flask!')
In this example, the placeholders {{ title }}
and {{ message }}
in index.html
will be replaced with the values passed from the home
function. When someone visits http://127.0.0.1:5000/
, they'll see a webpage with the title "Welcome" and the message "Hello, Flask!".
Summary
Understanding Flask routes and creating views is key to building web applications. Routes direct traffic to the appropriate functions, and views handle what the user sees. Using templates allows you to create dynamic and maintainable content for your users. Flask makes all this easy, so you can focus on creating amazing web applications.
Step 5: Using Templates with Jinja2
Introduction to Jinja2 Templating Engine
What is Jinja2?
Jinja2 is a powerful templating engine for Python. It allows you to create dynamic HTML pages by mixing Python-like expressions and HTML code. Think of it as a way to generate HTML code programmatically using data from your backend. It makes your web applications more interactive and easier to manage by separating the design (HTML) from the logic (Python).
Benefits of Using Templates in Web Applications
Using templates like Jinja2 in web applications comes with several benefits:
Separation of Concerns: Keeps your HTML separate from your Python code, making both easier to read and maintain.
Reusability: You can create reusable components, reducing redundancy and making updates simpler.
Efficiency: Templates can reduce the amount of code you write, making your development process faster and more efficient.
Dynamic Content: Easily generate dynamic content by passing data from your backend to your templates.
Creating and Using Templates
Writing Your First Template
Creating a template with Jinja2 is straightforward. Let's start with a simple HTML file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{ title }}</title>
</head>
<body>
<h1>Welcome, {{ user }}!</h1>
<p>Today is {{ date }}</p>
</body>
</html>
In this example, {{ title }}
, {{ user }}
, and {{ date }}
are placeholders. These will be replaced with actual values when the template is rendered.
Rendering Templates in Flask
To use Jinja2 templates in a Flask application, you need to render them in your routes. Here's how you can do it:
First, create a Flask app and save it as
app.py
:from flask import Flask, render_template from datetime import datetime app = Flask(__name__) @app.route('/') def home(): return render_template('index.html', title='Home Page', user='Alice', date=datetime.now().strftime("%Y-%m-%d")) if __name__ == '__main__': app.run(debug=True)
Place your
index.html
file inside a folder namedtemplates
in the same directory as yourapp.py
file. Flask looks for templates in thistemplates
folder by default.Run your Flask app:
python app.py
When you navigate to http://127.0.0.1:5000/
, you'll see your dynamic web page with the title "Home Page", the user "Alice", and the current date.
By using Jinja2 with Flask, you can make your web applications more dynamic and easier to maintain. Happy coding!
Step 6: Working with Forms and User Input
Handling Forms in Flask
Creating HTML forms
When you're building a web application with Flask, one of the fundamental tasks is handling forms. Forms allow users to input data, like their name, email, or preferences, which your application can then process. To create a form in HTML, you need to define it within your template using <form>
tags. Here’s a simple example:
<form action="/submit" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<br>
<input type="submit" value="Submit">
</form>
In this snippet, we've created a form that sends data to /submit
using the HTTP POST method. Each input field (<input>
) has a unique id
and name
attribute, which Flask uses to identify and process the data.
Processing form data in Flask
Once a user submits a form, Flask needs to handle the data. This is done in a route function using request.form
. Here’s how you might handle the form submission:
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route('/submit', methods=['POST'])
def submit_form():
username = request.form['username']
email = request.form['email']
# Process the data (e.g., store it in a database)
return f"Thank you, {username}, for submitting your email: {email}!"
if __name__ == '__main__':
app.run(debug=True)
In this Flask route, request.form
is used to access the submitted data. We retrieve values using keys that match the name
attributes in our HTML form (username
and email
in this case).
Validating User Input
Importance of validation
Validating user input is crucial for security and data integrity. It ensures that the data your application receives is correct and safe to process. Without validation, malicious users could exploit your application or cause unintended errors by submitting incorrect data.
Using Flask-WTF for form validation
Flask-WTF is a Flask extension that integrates with WTForms, a flexible form validation and rendering library for Python. It simplifies the process of validating forms in Flask applications by providing easy-to-use tools for defining and enforcing validation rules. Here's a basic example of how to use Flask-WTF:
from flask import Flask, render_template
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired, Email
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
class MyForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
submit = SubmitField('Submit')
@app.route('/form', methods=['GET', 'POST'])
def form():
form = MyForm()
if form.validate_on_submit():
# Process validated data
return f"Form submitted successfully: Username - {form.username.data}, Email - {form.email.data}"
return render_template('form.html', form=form)
if __name__ == '__main__':
app.run(debug=True)
In this example, MyForm
is a FlaskForm class using Flask-WTF. Each field in the form (username
and email
) has associated validators (DataRequired
and Email
) that ensure the data meets specific criteria before it's accepted.
This approach not only helps you understand how to handle forms and validate user input in Flask but also ensures your applications are robust and secure. By integrating these concepts, you can enhance the functionality and reliability of your Flask projects significantly.
Step 7: Connecting to a Database
Introduction to Databases
Databases are like organized filing systems for storing and managing data. They're crucial for applications to store and retrieve information efficiently. When building web applications with Flask, choosing the right type of database is key to ensuring your app runs smoothly and scales effectively.
Types of Databases commonly used with Flask
Flask supports various types of databases, each suited to different needs:
SQLite: A lightweight, serverless database ideal for small to medium-sized applications or prototypes. It's easy to set up and doesn't require a separate server process.
MySQL: A popular open-source relational database management system (RDBMS) known for its reliability and performance. It's suitable for larger applications requiring robust transaction support.
PostgreSQL: Another powerful open-source RDBMS known for its advanced features, scalability, and support for complex queries. It's suitable for applications needing strong ACID compliance.
MongoDB: A NoSQL database that stores data in flexible, JSON-like documents. It's great for handling large volumes of unstructured data and is highly scalable.
Choosing the right database for your application
When choosing a database for your Flask application, consider factors such as:
Data Structure: Does your data have a clear structure (like tables and rows) or is it more flexible (like JSON documents)?
Scalability: Will your application grow over time, requiring the database to handle more data and requests?
Complexity: Do you need advanced querying capabilities, transactions, or a schemaless design?
Community and Support: Consider the community size, available documentation, and support channels for the database you choose.
Setting Up SQLAlchemy
SQLAlchemy is a popular SQL toolkit and Object-Relational Mapping (ORM) library for Python. It simplifies database interactions by letting you work with Python objects instead of SQL queries directly.
Installing and configuring SQLAlchemy
To get started with SQLAlchemy:
Install SQLAlchemy: Use pip to install SQLAlchemy in your virtual environment:
pip install sqlalchemy
Configure SQLAlchemy: In your Flask application, configure SQLAlchemy to connect to your chosen database. For example:
from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' db = SQLAlchemy(app)
Creating models and interacting with the database
Define database models as Python classes. Each class represents a table in your database:
from datetime import datetime
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
created_at = db.Column(db.DateTime, default=datetime.utcnow)
def __repr__(self):
return '<User %r>' % self.username
Interact with the database using these models to query, insert, update, and delete data:
# Example usage
new_user = User(username='john_doe', email='john@example.com')
db.session.add(new_user)
db.session.commit()
users = User.query.all()
for user in users:
print(user.username, user.email)
SQLAlchemy handles the SQL queries behind the scenes, making database operations intuitive and Pythonic.
This structure helps you grasp the essentials of databases and SQLAlchemy for Flask applications. Choosing the right database and configuring SQLAlchemy correctly are crucial steps towards building a robust and scalable web application.
Step 8: User Authentication and Authorization
Implementing User Authentication
Basics of User Authentication
User authentication is the process of verifying the identity of a user attempting to access a system. It ensures that users are who they claim to be before granting access. In web applications, this typically involves collecting credentials (such as username and password) from users and comparing them against stored credentials in a database.
Using Flask-Login for User Management
Flask-Login is a widely used extension for managing user authentication in Flask applications. It simplifies the process of handling user sessions, login, logout, and user management tasks. By integrating Flask-Login, developers can easily authenticate users and manage user sessions in their Flask applications.
from flask import Flask, render_template, request, redirect, url_for
from flask_login import LoginManager, UserMixin, login_user, logout_user, login_required
app = Flask(__name__)
app.secret_key = 'your_secret_key'
login_manager = LoginManager()
login_manager.init_app(app)
# Mock user database (replace with actual database implementation)
users = {'user_id': {'username': 'user', 'password': 'password', 'role': 'user'}}
class User(UserMixin):
pass
@login_manager.user_loader
def load_user(user_id):
user = User()
user.id = user_id
return user
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
if username in users and users[username]['password'] == password:
user = User()
user.id = username
login_user(user)
return redirect(url_for('dashboard'))
else:
return 'Login Failed. Please check your username and password.'
return render_template('login.html')
@app.route('/dashboard')
@login_required
def dashboard():
return 'Welcome to the dashboard!'
@app.route('/logout')
@login_required
def logout():
logout_user()
return 'You are now logged out.'
if __name__ == '__main__':
app.run(debug=True)
Adding Authorization
Difference Between Authentication and Authorization
Authentication verifies the identity of a user, ensuring they are who they claim to be. On the other hand, authorization determines what authenticated users are allowed to do. It involves granting appropriate permissions and access rights based on the user's role or specific permissions.
Implementing Role-Based Access Control
Role-based access control (RBAC) is a method of managing system access based on user roles. Each role is assigned certain permissions, and users are granted access based on their role. This approach simplifies permission management and enhances security by restricting access to sensitive operations or data.
# Example continued from previous code snippet
@app.route('/admin')
@login_required
def admin():
if current_user.is_authenticated:
if users[current_user.id]['role'] == 'admin':
return 'Welcome Admin! You have access to admin functionalities.'
else:
return 'You do not have permission to access this page.'
else:
return redirect(url_for('login'))
# Sample decorator for admin-only access
def admin_required(func):
def wrapper(*args, **kwargs):
if current_user.is_authenticated and users[current_user.id]['role'] == 'admin':
return func(*args, **kwargs)
else:
return 'You do not have permission to access this page.'
return wrapper
@app.route('/admin/settings')
@admin_required
def admin_settings():
return 'Admin settings page.'
In these examples, we've demonstrated how to implement user authentication using Flask-Login and enhance it with role-based access control (RBAC) for authorization. These concepts are fundamental for securing web applications and ensuring that users have appropriate access to resources based on their roles and permissions.
Step 9: Building and Using APIs
What are APIs?
APIs, or Application Programming Interfaces, serve as intermediaries that allow different software applications to communicate with each other. Think of them as messengers facilitating requests and responses between applications. This interaction enables seamless data exchange and functionality integration, making APIs fundamental to modern software development.
Importance of APIs in Web Development
APIs play a pivotal role in web development by enabling developers to leverage existing functionalities to build new applications rapidly. They abstract the complexities of underlying systems, promoting reusability and scalability. For instance, APIs empower developers to integrate payment gateways, social media logins, or even complex algorithms into their applications with minimal effort. This not only accelerates development but also enhances the overall user experience.
Creating APIs with Flask
Setting up API Routes
In Flask, setting up API routes involves defining URL endpoints that clients can access to perform specific actions or retrieve data. These routes are mapped to Python functions that handle incoming requests and generate appropriate responses. Here’s a simple example:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
data = {'message': 'Hello, API!'}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
In this example, accessing /api/data
triggers the get_data()
function, which returns a JSON response containing a greeting message.
Handling JSON Data in Flask
JSON (JavaScript Object Notation) is a lightweight data interchange format commonly used in APIs for transmitting structured data. Flask provides built-in support for JSON manipulation, making it straightforward to process JSON data both from incoming requests and outgoing responses. Here’s how you can handle JSON data in Flask:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/api/post', methods=['POST'])
def create_post():
if request.is_json:
data = request.get_json()
# Process and store the incoming JSON data
return jsonify({'message': 'Post created successfully', 'data': data}), 201
else:
return jsonify({'error': 'Invalid JSON'}), 400
if __name__ == '__main__':
app.run(debug=True)
In this example, the create_post()
function handles POST requests containing JSON payloads. It checks if the request body is in JSON format (request.is
_json
) and retrieves the data using request.get_json()
.
Step 10: Deploying Your Flask Application
Preparing for Deployment
Before deploying your application, it's crucial to follow best practices to ensure a smooth transition to production. Here are some key steps to consider:
Testing: Thoroughly test your application in a production-like environment to catch bugs early. Test cases should cover all functionalities and edge cases.
Environment Configuration: Configure your application for production by optimizing performance and securing sensitive information like API keys and database credentials. Use environment variables to manage configuration settings.
Documentation: Document all necessary steps and configurations required for deployment. Include setup instructions for developers who will be deploying or maintaining the application.
Deploying to a Cloud Service
When it comes to deploying your application to the cloud, there are several popular services available. Here’s a brief overview and a step-by-step guide for deploying on Heroku:
Overview of Popular Cloud Services
AWS (Amazon Web Services): Known for its scalability and wide range of services including Lambda for serverless computing and EC2 for virtual servers.
Heroku: Offers an easy-to-use platform for deploying and managing applications without worrying about infrastructure. It supports various programming languages and frameworks.
Step-by-Step Guide to Deploying on Heroku
Deploying on Heroku is straightforward and can be done in a few steps:
Create a Heroku Account: Sign up for a Heroku account if you haven’t already.
Install Heroku CLI: Download and install the Heroku Command Line Interface (CLI) to interact with Heroku from your terminal.
Prepare Your Application: Ensure your application is ready for deployment. This includes configuring a
Procfile
to specify how Heroku should run your app.Initialize a Git Repository: If you haven’t already, initialize a Git repository in your project folder.
Login to Heroku CLI: Use the
heroku login
command to log into your Heroku account from the terminal.Create a Heroku App: Use
heroku create
to create a new Heroku app. This will generate a unique URL for your application.Deploy Your Application: Deploy your application by pushing your code to the Heroku remote repository using
git push heroku main
.View Your Application: Once deployed, use
heroku open
to open your application in the browser.
By following these steps, you can successfully deploy your application to Heroku and make it accessible on the web.
Frequently Asked Questions
What is the difference between Flask and Django?
Flask and Django are both popular Python web frameworks, but they serve different purposes. Flask is a lightweight and flexible micro-framework that is ideal for smaller applications or when you need more control over the components you use. It allows developers to choose and integrate different libraries and tools as per their project requirements. On the other hand, Django is a full-stack framework that follows the "batteries-included" philosophy, providing a robust set of features out of the box for rapid development of complex applications, including an ORM, admin panel, authentication, and more.
How do I install Flask on my local machine?
To install Flask on your local machine, you can use Python's package manager, pip. Open your terminal or command prompt and run:
Copy codepip install Flask
This command will download and install Flask and its dependencies. Once installed, you can start building your Flask applications.
Can I use Flask for large-scale applications?
Flask is well-suited for large-scale applications if properly structured and configured. While it's more lightweight compared to Django, Flask provides the flexibility to scale applications by leveraging extensions and implementing best practices like modular design, blueprint usage for organizing routes, and utilizing appropriate database solutions such as SQLAlchemy for complex data handling.
How do I manage multiple routes in a Flask application?
In Flask, you manage multiple routes by defining them using decorators. Each route corresponds to a different endpoint in your application. For example:
pythonCopy codefrom flask import Flask app = Flask(__name__) @app.route('/') def index(): return 'Hello, World!' @app.route('/about') def about(): return 'About page' if __name__ == '__main__': app.run(debug=True)
Here,
/
and/about
are different routes, each handling specific HTTP requests.What are the best practices for securing a Flask application?
To secure a Flask application, consider:
Using HTTPS to encrypt data transmitted over the network.
Implementing authentication and authorization mechanisms (e.g., Flask-Login, Flask-JWT).
Validating and sanitizing user inputs to prevent injection attacks.
Setting secure HTTP headers (e.g., Content Security Policy, XSS Protection).
Keeping dependencies updated to patch security vulnerabilities.
Using Flask extensions like Flask-Security for comprehensive security features.
How do I connect a Flask application to a MySQL database?
To connect Flask to a MySQL database, you typically use SQLAlchemy, a popular ORM for Python. First, install the necessary packages:
Copy codepip install Flask-SQLAlchemy pip install mysqlclient
Then, configure your Flask application:
pythonCopy codefrom flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'mysql://username:password@hostname/database_name' db = SQLAlchemy(app)
Replace
username
,password
,hostname
, anddatabase_name
with your MySQL credentials. Now, you can define models and interact with your MySQL database using SQLAlchemy.What are some common debugging techniques for Flask applications?
Debugging Flask applications involves:
Enabling debug mode in your Flask application (
app.run
(debug=True)
).Using logging to track errors and application flow.
Utilizing Flask's built-in debugger and interactive debugger (if enabled).
Checking Flask's server logs for detailed error messages.
Using Flask extensions like Flask Debug Toolbar for profiling and debugging.
Testing and debugging APIs with tools like Postman or curl for HTTP request/response inspection.
How do I handle file uploads in Flask?
To handle file uploads in Flask, you need to set up a route that accepts
POST
requests with file data. Userequest.files
to access uploaded files and save them to a designated directory:pythonCopy codefrom flask import Flask, request, redirect, url_for import os app = Flask(__name__) UPLOAD_FOLDER = '/path/to/uploads' app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER @app.route('/upload', methods=['POST']) def upload_file(): if 'file' not in request.files: return redirect(request.url) file = request.files['file'] if file.filename == '': return redirect(request.url) if file: filename = secure_filename(file.filename) file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename)) return 'File uploaded successfully' if __name__ == '__main__': app.run(debug=True)
Ensure to set
enctype="multipart/form-data"
in your HTML form for file uploads.What are the benefits of using Flask for web development?
Flask offers several benefits:
Lightweight and easy to learn, making it ideal for small to medium-sized applications.
Flexible and modular, allowing developers to choose components based on project requirements.
Extensive documentation and a large community for support and extensions.
Well-suited for prototyping and building RESTful APIs.
Integrates easily with other Python libraries and frameworks.
Provides control over application structure and components used.
How can I deploy a Flask application on AWS?
To deploy a Flask application on AWS, you can use services like Elastic Beanstalk, EC2, or Lambda depending on your application's architecture and scale. Here’s a brief outline:
Elastic Beanstalk: Package your Flask application into a ZIP file, create an Elastic Beanstalk environment, and upload your application.
EC2: Launch an EC2 instance, configure it with Nginx/Gunicorn for serving Flask, and deploy your application manually or using deployment tools.
Lambda: Package your Flask application as a ZIP with AWS Lambda's handler and deploy using AWS CLI or through AWS Management Console.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
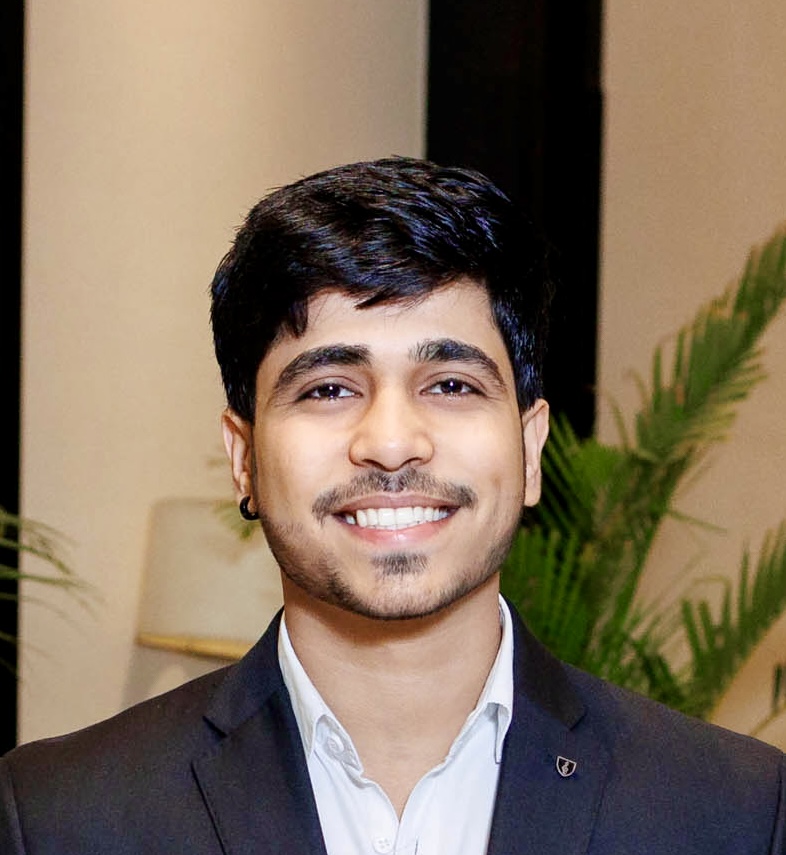
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.