Why lazy load not working in React ?

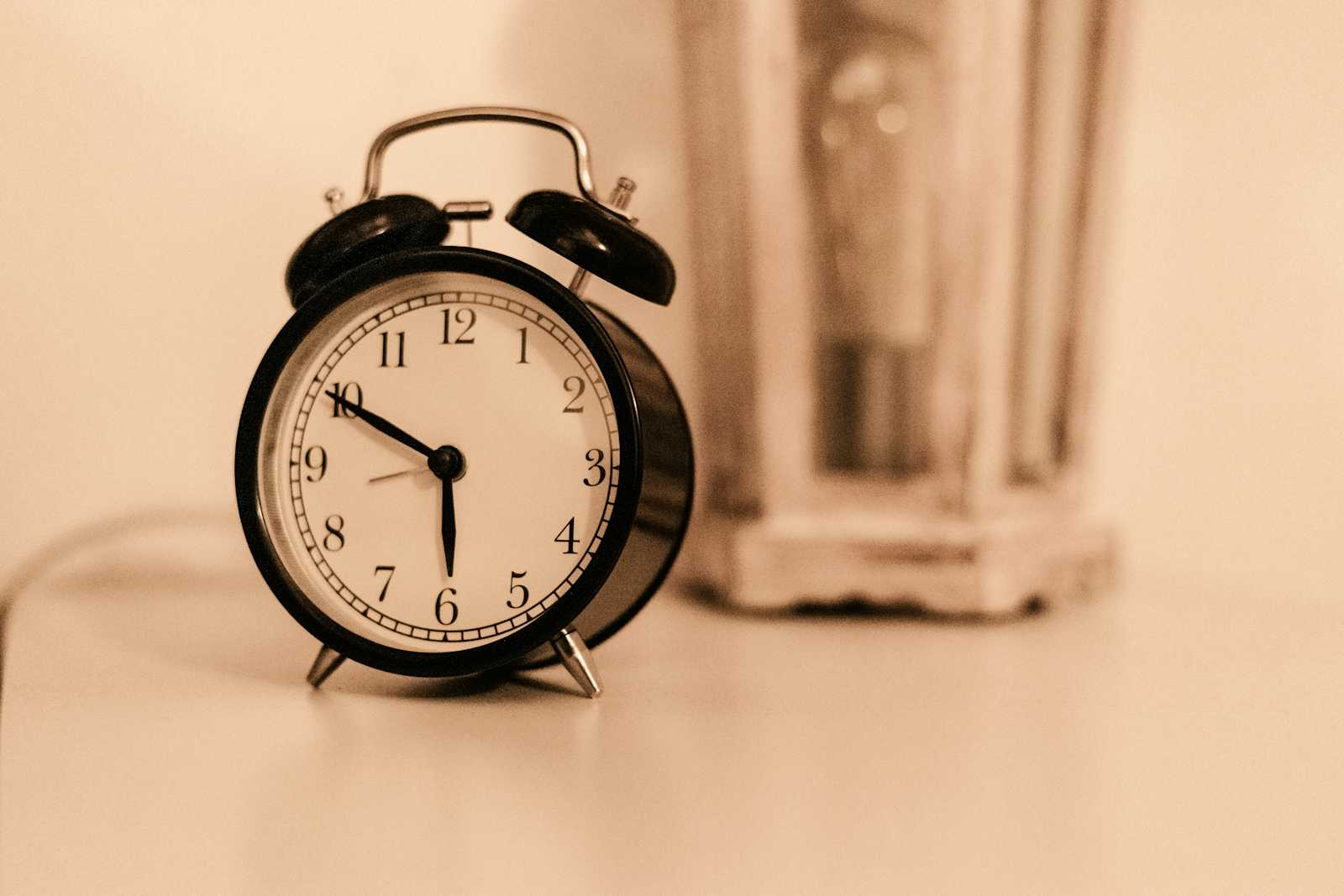
You implemented lazy load in your React application, but during testing, it doesn't seem to work. Why is that?
Lazy loading is a technique in React where you load components only when they are needed.
There are two benefits of using it
It will not block the loading of other components, and you can show a loader icon/message until the lazy component is loaded, which is good for UX/User experience.
It decreases the main bundle size. Code wrapped in lazy loading will be removed from the main bundle. Lazy component will be loaded based on a chunk bundle
src_About_js.bundle.js
(see below) that you can see in the browser console.
Let's understand this with a practical example.
App.js
import React, { lazy, Suspense } from 'react';
import Home from "./Home"
const About = lazy(() => import('./About'));
import { Routes, Route } from "react-router-dom"
function App() {
return (
<div className="App">
<Routes>
<Route path="/" element={ <Home/> } />
<Route path="about" element={
<Suspense fallback={<div>Loading</div>}>
<About/>
</Suspense>
} />
</Routes>
</div>
);
}
export default App;
Here, we are wrapping the /about route in lazy loading, so it will not load in advance and not counted in the main bundle size on page load.
As seen below, initially bundle.js will be loaded on first page load. When on /about route, src_About_js.bundle.js
will be loaded as a part of chunk.
Sometimes, src_About_js.bundle.js
is not generated even if you added lazy loading code for the about /route. You need to analyze your Webpack configuration for your React application. Ensure that the LimitChunkCountPlugin
is configured correctly. For example, if maxChunks is set to 1, increase it to a higher number based on your application's requirements, such as 5 or 10.
plugins: [
new webpack.optimize.LimitChunkCountPlugin({
maxChunks: 1
})
]
In conclusion, implementing lazy loading in your React application can significantly enhance user experience and optimize performance by reducing the initial bundle size and loading components only when needed. However, if lazy loading doesn't seem to work, it's crucial to review your Webpack configuration, particularly the LimitChunkCountPlugin
settings, to ensure that chunk generation is not restricted. By properly configuring lazy loading, you can achieve faster page load times and a more efficient application.
Subscribe to my newsletter
Read articles from Parveen Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Parveen Kumar
Parveen Kumar
I am a Full-stack MERN engineer with a product centric approach, specializing in the design and development of scalable, reliable, production ready web application, I bring expertise in JavaScript, React, and Node Js. Get in touch! paulparveen01@gmail.com