Spread Operator & Rest Operator
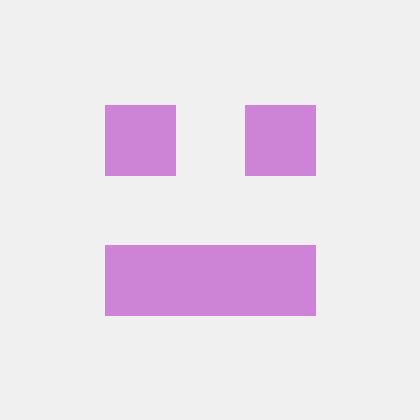
1 min read
Spread Operator
we spread the original array or object without changing it
// example 1 : object const animal = { status: 'healthy' } const dog = { ...animal, bark: true } console.log(animal) // {status: 'healthy'} console.log (dog) // {status: 'healthy', bark: true} // example 2 : array const animals = ['dog', 'cat'] const moreAnimals = [...animals, 'bird']; const useMoreThanOneSpread = [...animals, 'bird', ...animals]; console.log(animals) // ['dog', 'cat'] console.log(moreAnimals) // ['dog', 'cat', 'bird'] console.log(useMoreThanOneSpread ) // ['dog', 'cat', 'bird', 'dog', 'cat',]
Rest Operator
Use it with array, object, and parameter
// example 1 : object const dog = { status: 'healthy', bark: true, attribute: 'cute' } const {status, ...rest} = dog; console.log(status) // healthy console.log(rest) // {bark: true, attribute: 'cute'} // example 2 : array const numbers = [0, 1, 2, 3, 4, 5]; const [one, ...rest] = numbers; console.log(one); // 0 console.log(rest); // [1, 2, 3, 4, 5] // example 3 : parameter function returnRest(...rest) { return rest; } const result1 = returnRest(1, 2, 3, 4, 5); console.log(result1); // [1, 2, 3, 4, 5]
0
Subscribe to my newsletter
Read articles from Jae Yeon Jung directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
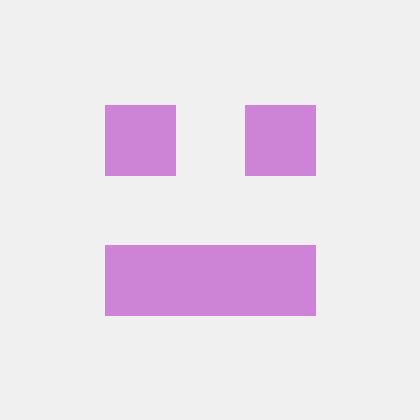