A Comprehensive Overview of Template Engines
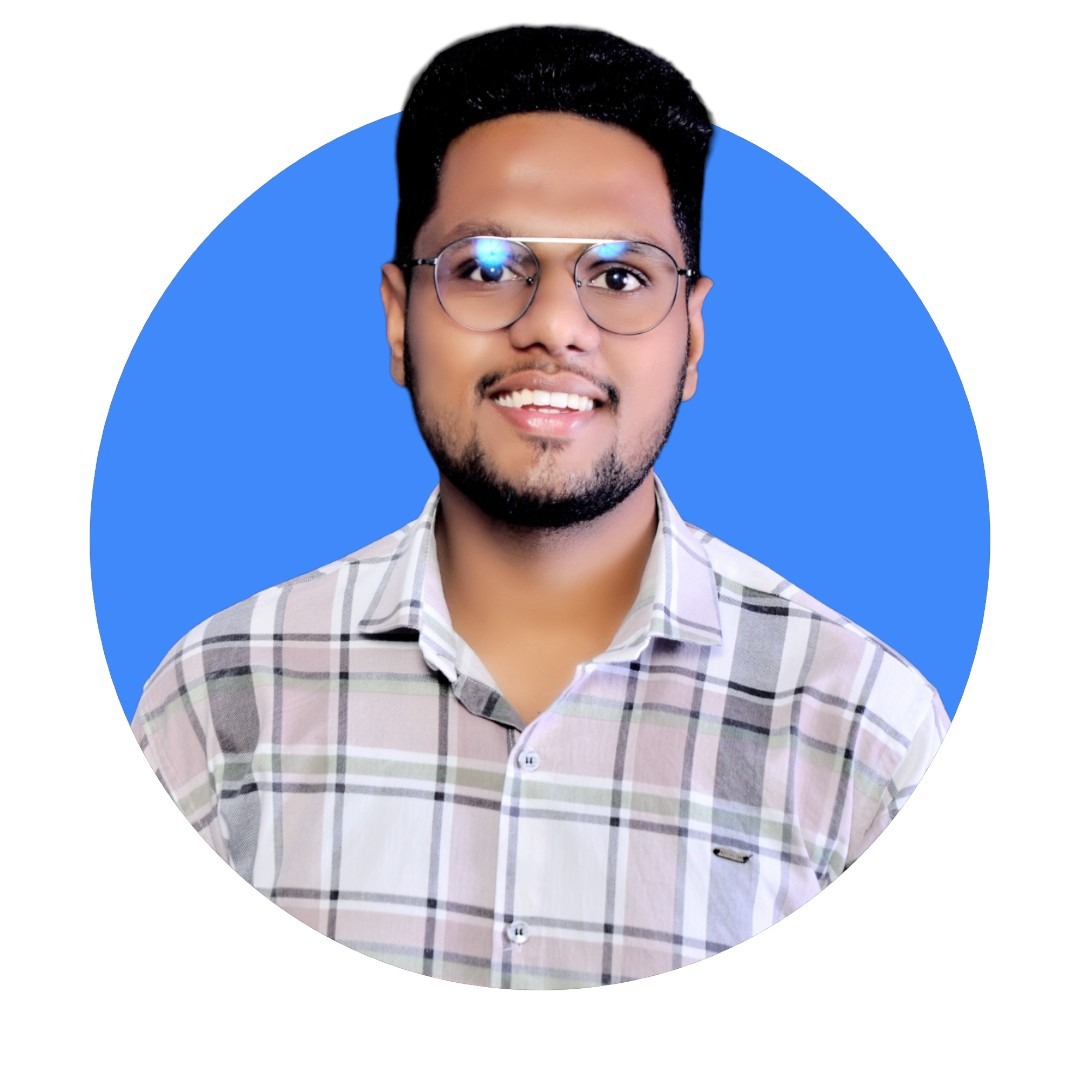
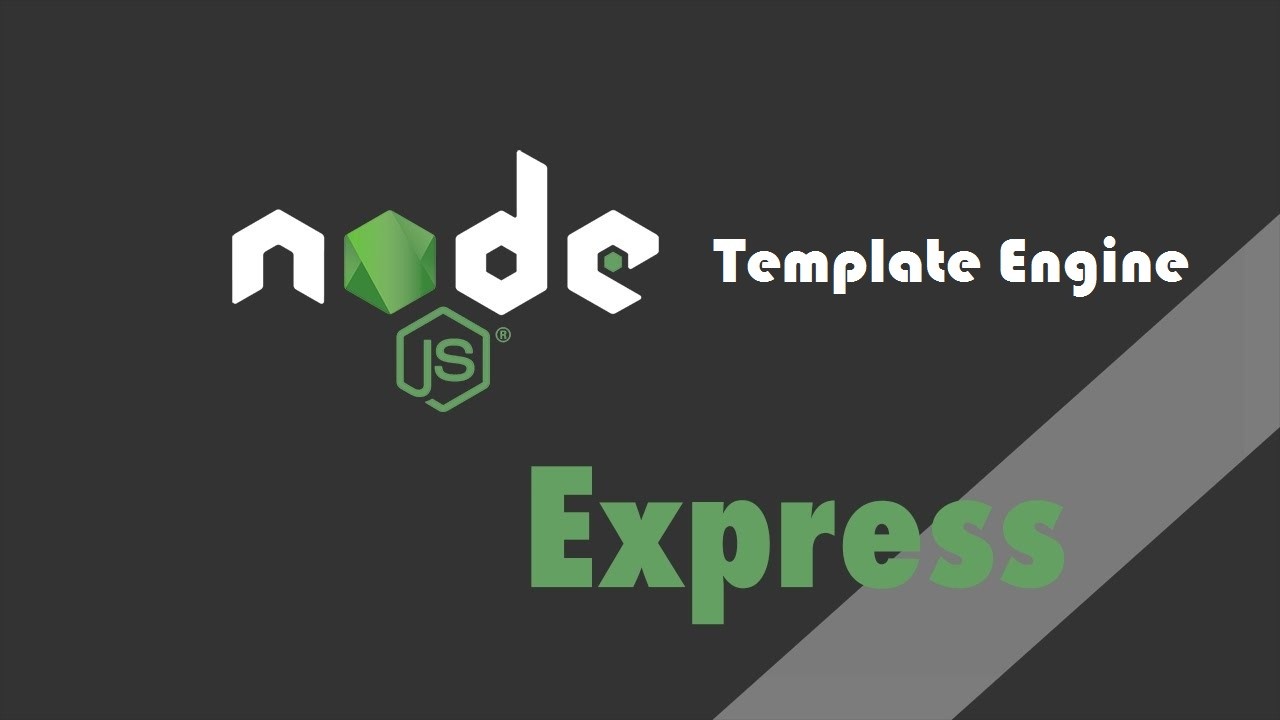
What is a template engine?
A template engine facilitates you to use static template files in your applications.
At runtime, it replaces variables in a template file with actual values, and transforms the template into an HTML file sent to the client.
So this approach is preferred to design HTML pages easily.
Template engine helps us to create an HTML template with minimal code.
Also, it can inject data into HTML template at client side and produce the final HTML.
Following figure shown how template engine works in Node.js.
In above figure, client-side browser loads the HTML template, JSON data and template engine library loads from the server side. Template engine produces the final HTML using template and render the server side data in client's browser. Also some HTML templates process data and generate final HTML page at server side.
Following is a list of some popular template engines that work with Express.js:
Pug (formerly known as jade)
mustache
dust
atpl
eco
ect
ejs
We have see basic info about template engine, So we can learn how to use template engine in Node.js application using Express.js.In this blog, We will learn how to use "EJS" template engine in Node.js application using Express.js.
What is EJS?
EJS or Embedded Javascript Templating is a templating engine used by Node.js.
Template engine helps to create an HTML template with minimal code.
Also, it can inject data into an HTML template on the client side and produce the final HTML.
EJS is a simple templating language that is used to generate HTML markup with plain JavaScript.
It also helps to embed JavaScript into HTML pages
Embedded JavaScript Templates (EJS) use for lightweight solution towards creating HTML markup with simple JavaScript code.
Fast code execution, ease of debugging makes this the perfect templating engine for those who want to do HTML work with their favourite language, probably JavaScript.
Install and configure EJS template:
Go inside the project & then Go inside the terminal and install the EJS (Embedded Javascript) template using the following command.
npm install ejs --save
Now, import the ejs template as well as configure the ejs template.
Write the final code inside the app.js file.
const express = require('express');
const path = require('path');
const ejs = require('ejs');
var app = express();
const port = 3000;
//set view engine & view folder path
app.set('view engine', 'ejs');
app.set('views',path.join(__dirname, 'views'));
app.get('/', (req, res) => {
// render welcome ejs file from views folder.
res.render('welcome.ejs', {data: 'Welcome to NodeJS Application with EJS Template'});
});
app.listen(port, () => console.log(`Create app listening on port ${port}!`));
`Create app listening on port ${port}!`));
As you can see in the above code, first we have import express module, ejs template engine and then set the view engine using app.set() method.
The set() method sets the "view engine", which is one of the application setting property in Express.js.
In the HTTP Get request for home page, it renders welcome.ejs from the views folder using res.render() method.
So, here we have set the template engine to ejs. Now create a file called the welcome.ejs inside the views folder.
Let's see welcome.ejs file look like;
<!-- welcome.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>EJS Template</title>
</head>
<body>
<!-- here we render server side data -->
<%= data %>
</body>
</html>
As you can see above welcome.ejs file code we bind the server side data using ejs expression tag <%= %> like <%= data %>.
Finally we have setting up all code in app.js file and also bind the server side data into the template file.
Finally, we need to go to project folder directory and open command prompt there and run below command.
nodemon app.js
After execution of above command the Node.js application running 3000 port.
So go to browser and hit the "http://localhost:3000" to show node.js application base URL response as 'Welcome to NodeJS Application with EJS template' on the browser.
Output look like:
Now, we have successfully integrated the EJS template.
Finally, How To Use Template Engines With Express blog with Example is over.
Features OF EJS :
Fast compilation and rendering
Simple template tags: <% %>
Custom delimiters (e.g., use [? ?] instead of <% %>)
Sub-template includes
Ships with CLI
Both server JS and browser support
Static caching of intermediate JavaScript
Static caching of templates
Complies with the Express view system
Tags Of EJS :
<%
'Scriptlet' tag, for control-flow, no output<%_
‘Whitespace Slurping’ Scriptlet tag, strips all whitespace before it<%=
Outputs the value into the template (HTML escaped)<%-
Outputs the unescaped value into the template<%#
Comment tag, no execution, no output<%%
Outputs a literal '<%'%>
Plain ending tag-%>
Trim-mode ('newline slurp') tag, trims following newline_%>
‘Whitespace Slurping’ ending tag, removes all whitespace after it
Example:
You'll likely want to use the raw output tag (<%-
) with your include to avoid double-escaping the HTML output.
<ul>
<% users.forEach(function(user){ %>
<%- include('user/show', {user: user}); %>
<% }); %>
</ul>
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
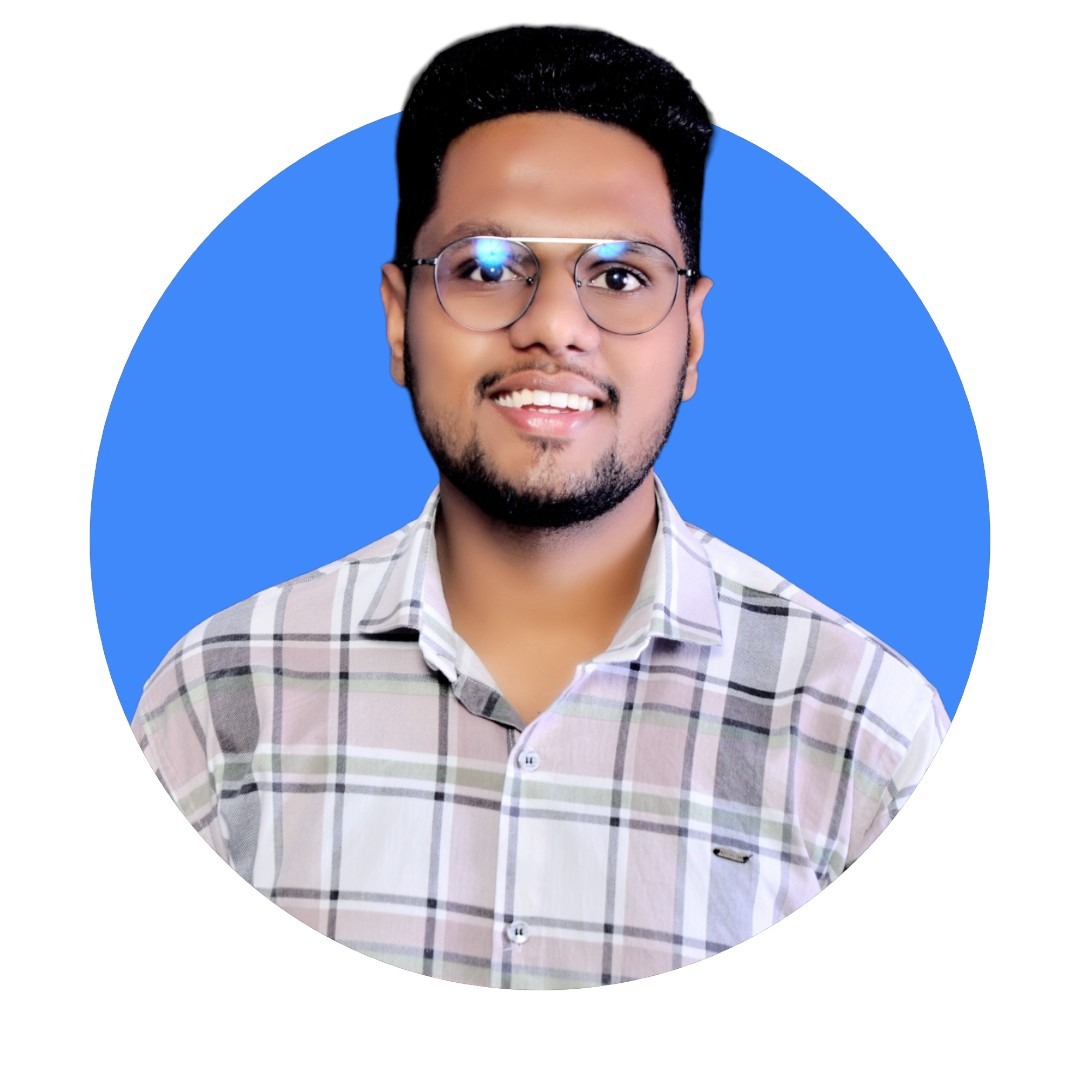
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.