Advanced TypeScript Optimization: Balancing Performance and Maintainability
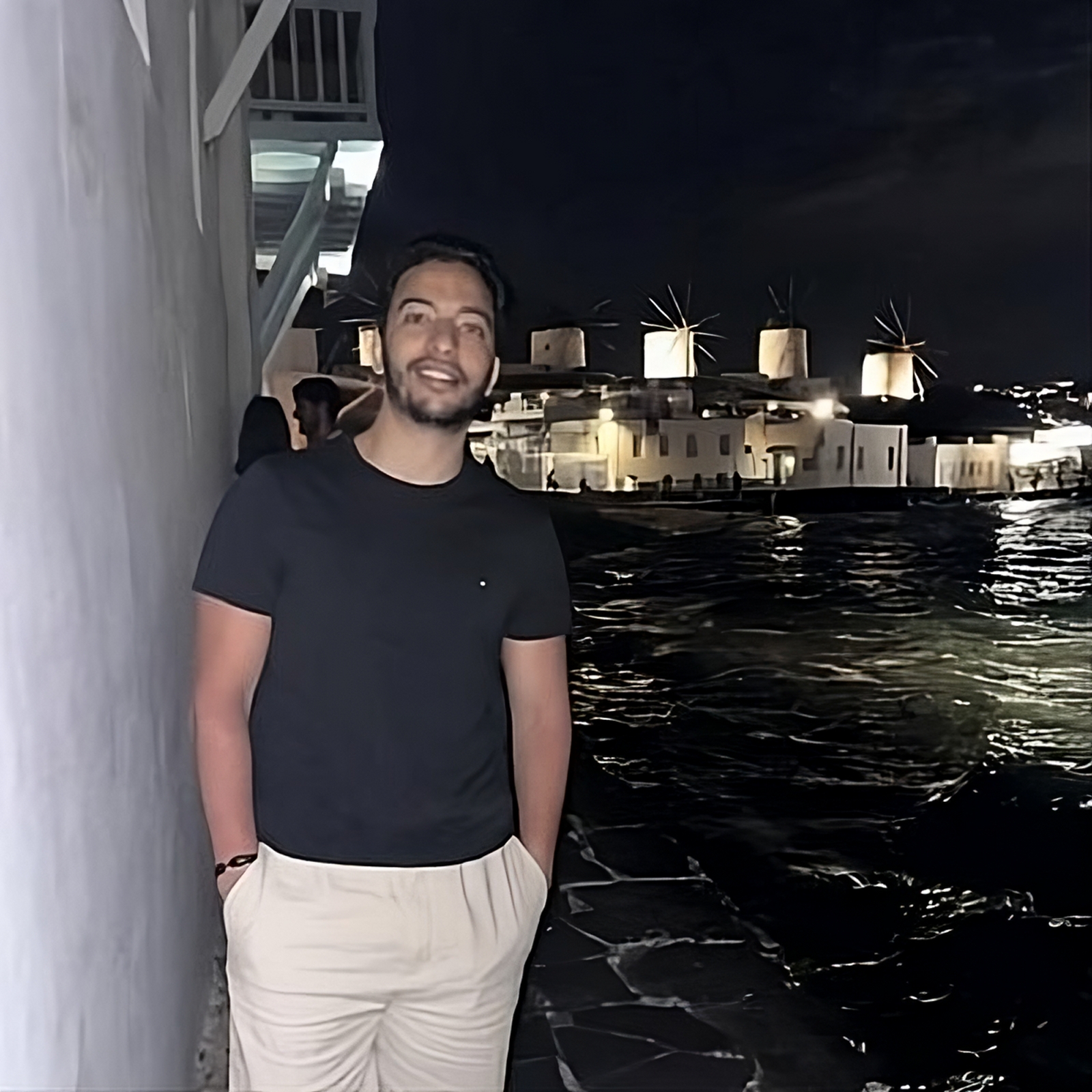
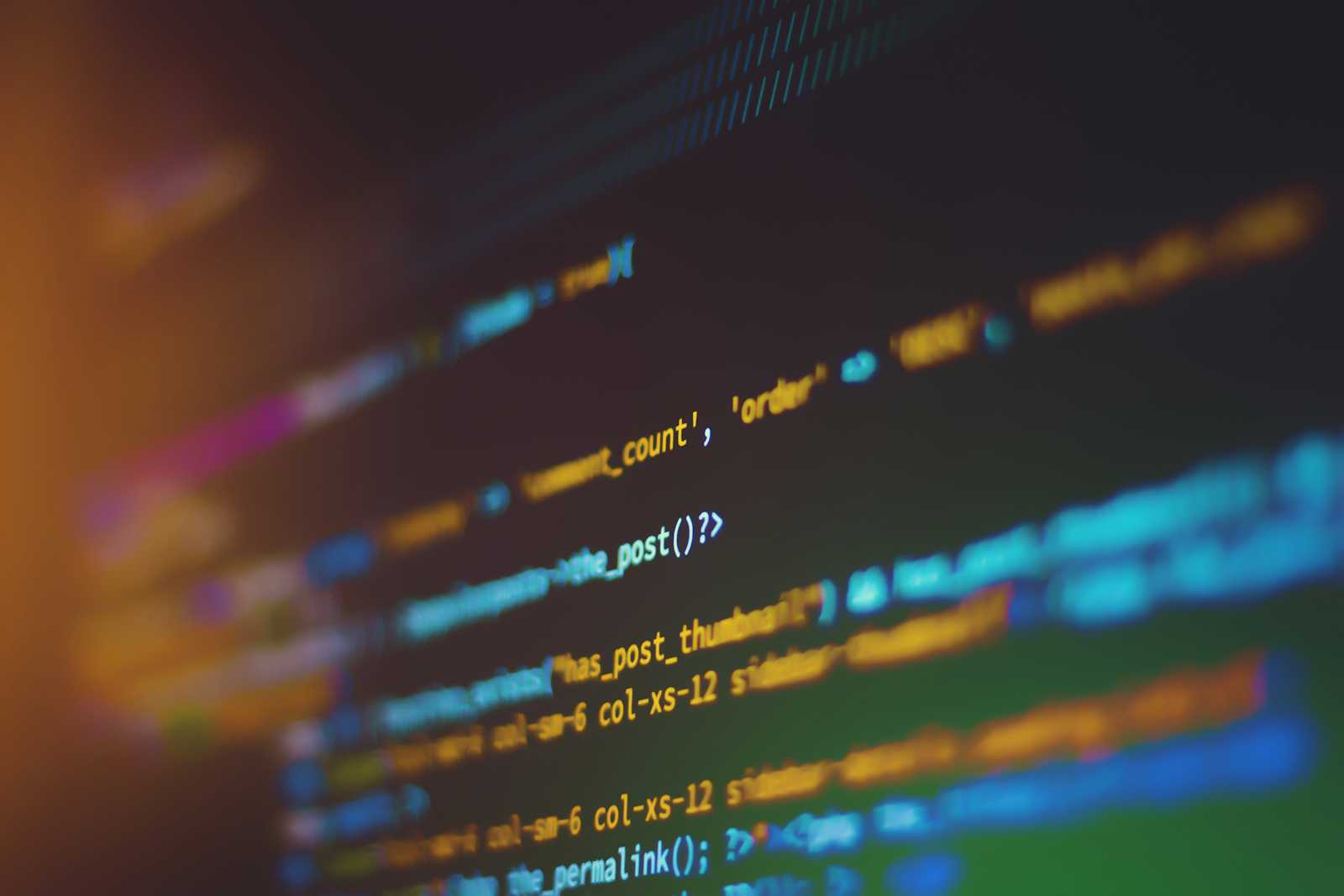
In our previous discussion on code optimization in TypeScript, we explored various techniques to improve performance while maintaining readability. Building on those foundations, this article delves into more advanced optimization patterns and their implications for code maintainability. We'll examine how to push the boundaries of TypeScript's capabilities to achieve peak performance without sacrificing the clarity and robustness of your codebase.
1. Leveraging Conditional Types for Performance
Conditional types in TypeScript can be used not just for type safety, but also for performance optimization. By using conditional types, we can create more specific and efficient code paths:
type IsArray<T> = T extends any[] ? true : false;
function processData<T>(data: T): IsArray<T> extends true ? number[] : number {
if (Array.isArray(data)) {
return data.map(item => item * 2) as any;
} else {
return (data as number) * 2;
}
}
const result1 = processData([1, 2, 3]); // Type: number[]
const result2 = processData(4); // Type: number
This approach allows the compiler to optimize the code based on the input type, potentially leading to better performance. However, it's crucial to balance this with readability, as complex conditional types can be challenging to understand and maintain.
2. Using TypeScript Decorators for Memoization
Decorators can be a powerful tool for adding memoization to methods without cluttering the main code:
function Memoize() {
return function (target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
const cache = new Map();
descriptor.value = function (...args: any[]) {
const key = JSON.stringify(args);
if (cache.has(key)) {
return cache.get(key);
}
const result = originalMethod.apply(this, args);
cache.set(key, result);
return result;
};
return descriptor;
};
}
class ExpensiveCalculations {
@Memoize()
fibonacci(n: number): number {
if (n <= 1) return n;
return this.fibonacci(n - 1) + this.fibonacci(n - 2);
}
}
This approach separates the memoization logic from the business logic, improving both performance and maintainability.
3. Optimizing Object Creation with TypeScript's Utility Types
TypeScript's utility types can be leveraged to create optimized object structures:
type User = {
id: number;
name: string;
email: string;
preferences: {
theme: 'light' | 'dark';
notifications: boolean;
};
};
type UserUpdateParams = Partial<Omit<User, 'id'>>;
function updateUser(id: number, params: UserUpdateParams) {
// Implementation here
}
updateUser(1, { name: 'John Doe', preferences: { theme: 'dark' } });
This pattern allows for flexible and type-safe object updates while preventing unnecessary property assignments, potentially improving performance in large-scale applications.
4. Using TypeScript Generics for Compile-Time Optimizations
Generics can be used to create highly optimized functions that the TypeScript compiler can inline:
function swap<T, U>(tuple: [T, U]): [U, T] {
return [tuple[1], tuple[0]];
}
const result = swap([1, 'two']); // Type: [string, number]
While this might seem simple, for complex operations, such compile-time optimizations can lead to significant performance improvements, especially when used extensively throughout a large codebase.
5. Optimizing Asynchronous Operations with Custom Promises
Creating custom Promise implementations can lead to more efficient asynchronous operations:
class FastPromise<T> implements PromiseLike<T> {
private result?: T;
private error?: any;
private resolve?: (value: T | PromiseLike<T>) => void;
private reject?: (reason?: any) => void;
constructor(executor: (resolve: (value: T | PromiseLike<T>) => void, reject: (reason?: any) => void) => void) {
try {
executor(
value => {
if (this.resolve) this.resolve(value);
else this.result = value;
},
reason => {
if (this.reject) this.reject(reason);
else this.error = reason;
}
);
} catch (e) {
if (this.reject) this.reject(e);
else this.error = e;
}
}
then<TResult1 = T, TResult2 = never>(
onfulfilled?: ((value: T) => TResult1 | PromiseLike<TResult1>) | undefined | null,
onrejected?: ((reason: any) => TResult2 | PromiseLike<TResult2>) | undefined | null
): PromiseLike<TResult1 | TResult2> {
return new FastPromise((resolve, reject) => {
const wrappedResolve = (value: T | PromiseLike<T>) => {
try {
resolve(onfulfilled ? onfulfilled(value as T) : value as any);
} catch (e) {
reject(e);
}
};
const wrappedReject = (reason: any) => {
if (onrejected) {
try {
resolve(onrejected(reason));
} catch (e) {
reject(e);
}
} else {
reject(reason);
}
};
if (this.result !== undefined) wrappedResolve(this.result);
else if (this.error !== undefined) wrappedReject(this.error);
else {
this.resolve = wrappedResolve;
this.reject = wrappedReject;
}
});
}
}
This custom Promise implementation can be more efficient for certain use cases, particularly when dealing with a high volume of rapidly resolving promises. However, it's important to note that such optimizations should be used judiciously, as they can increase code complexity and potentially introduce bugs if not implemented correctly.
Conclusion
While these advanced optimization techniques can significantly boost performance, they often come at the cost of increased complexity. It's crucial to apply them judiciously, always considering the trade-offs between performance gains and code maintainability.
Remember these key points when applying advanced optimizations:
Profile First: Always measure performance before and after optimization to ensure the complexity is justified.
Document Thoroughly: Complex optimizations should be well-documented, explaining both the how and the why.
Encapsulate Complexity: Where possible, encapsulate complex optimizations within well-named functions or classes to preserve the overall readability of your codebase.
Consider the Team: Think about how these optimizations will affect your team's ability to understand and maintain the code.
Stay Updated: TypeScript and JavaScript are evolving languages. Keep an eye out for new features that might offer simpler ways to achieve the same performance gains.
By carefully balancing these advanced optimization techniques with code maintainability, you can create TypeScript applications that are both high-performing and sustainable in the long term.
Subscribe to my newsletter
Read articles from Ayoub Touba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
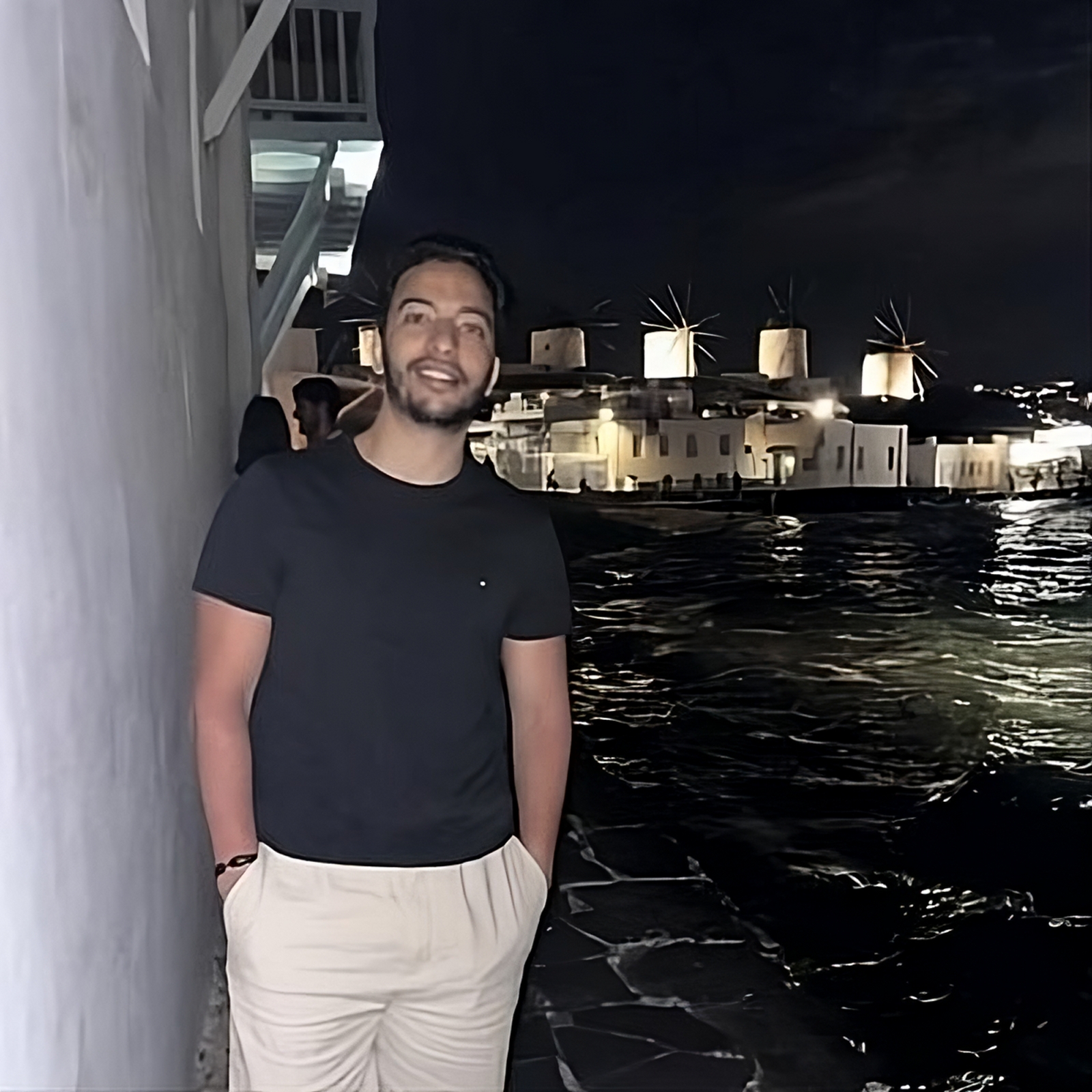
Ayoub Touba
Ayoub Touba
With over a decade of hands-on experience, I specialize in building robust web applications and scalable software solutions. My expertise spans across cutting-edge frameworks and technologies, including Node.js, React, Angular, Vue.js, and Laravel. I also delve into hardware integration with ESP32 and Arduino, creating IoT solutions.