π Understanding the Difference Between if-else and the Ternary Operator in JavaScript
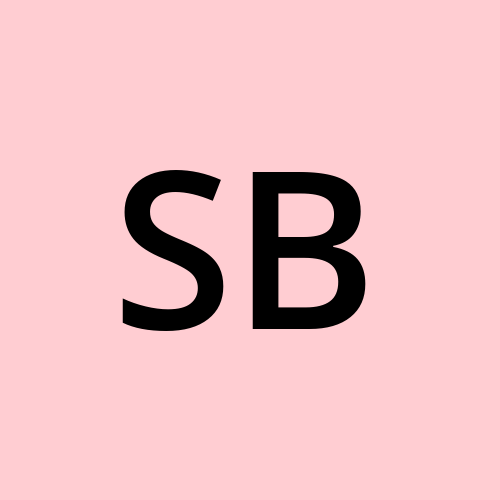
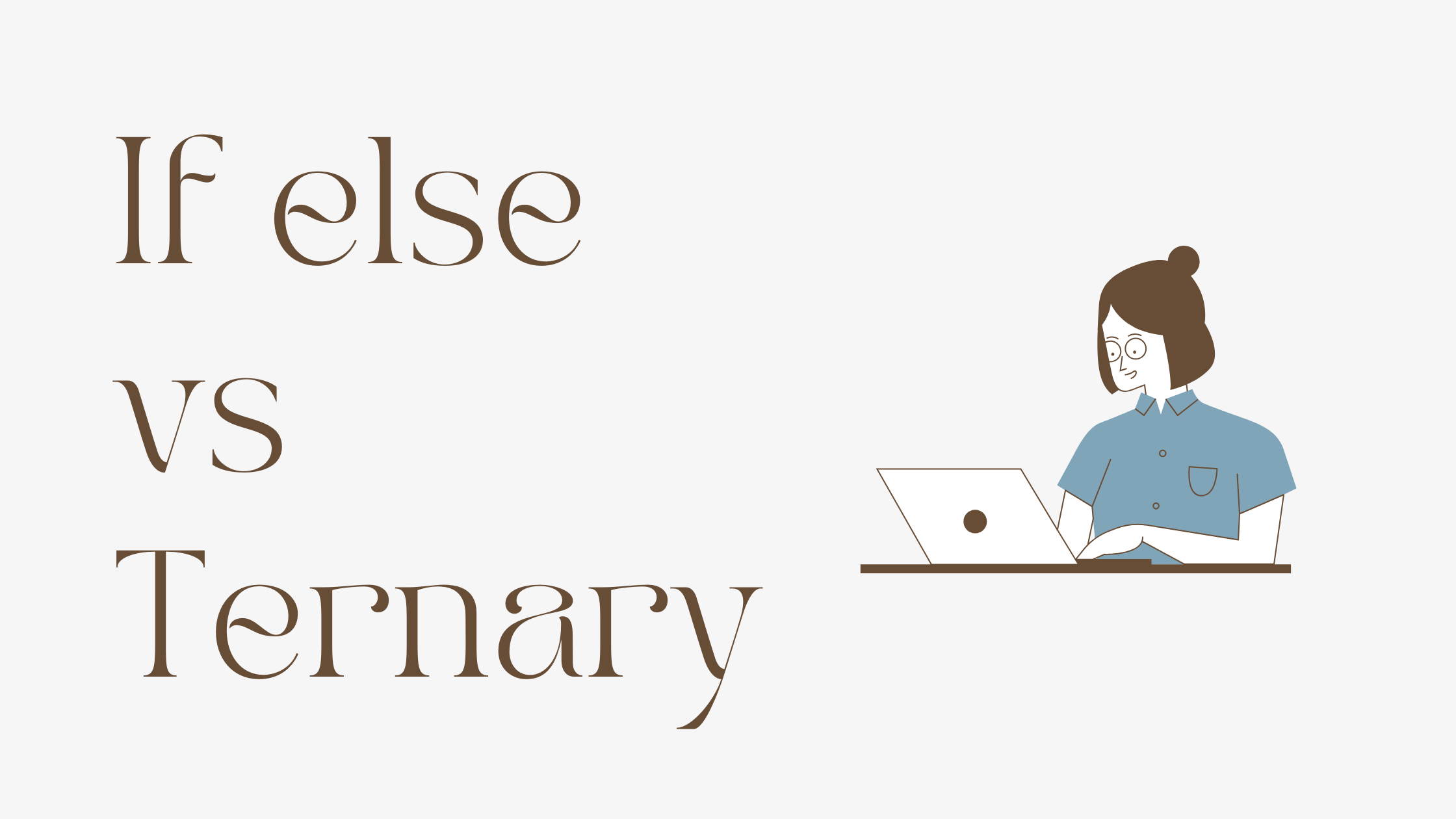
JavaScript is a versatile and widely-used programming language. Among its many features are control flow statements like if-else
and the ternary operator. Both are used to execute code based on conditions, but they have different use cases and syntax. This also makes it an important question in an interview's point of view. Letβs dive into the details! πββοΈ
π if-else
Statement
The if-else
statement is a fundamental control flow statement that allows you to execute code blocks based on certain conditions.
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
Pros:
Readability: Clear and easy to understand, especially for beginners.
Flexibility: Can be extended with multiple
else if
statements.
Cons:
- Verbosity: Can be more verbose for simple conditions.
Not suitable for expressions: Cannot be used directly in assignments or return statements.
π§© Ternary Operator
The ternary operator, also known as the conditional operator, is a shorthand way to perform conditional operations. It is called "ternary" because it involves three operands.
condition ? expressionIfTrue : expressionIfFalse
Pros:
Conciseness: Short and can be used directly within expressions.
Inline usage: Great for simple conditional assignments and return statements.
Cons:
Readability: Can become hard to read with complex conditions or nested ternary operators.
Limited flexibility: Not suitable for multiple conditional branches.
π Comparing if-else
and Ternary Operator
Hereβs a comparison to help you decide when to use each:
1. Readability:
if-else
: Better for readability, especially with complex logic.Ternary: Best for simple conditions and single-line expressions.
2. Use Case:
if-else
: Use when you need multiple branches or more complex logic.Ternary: Use for simple conditional assignments and expressions.
3. Flexibility:
if-else
: Can handle multiple conditions usingelse if
.Ternary: Limited to two outcomes (true or false).
π Conclusion
Both if-else
statements and the ternary operator have their place in JavaScript programming. By understanding their differences you can choose the right tool for the job, making your code more readable, maintainable, and efficient. Happy coding! π¨βπ»π©βπ»
Subscribe to my newsletter
Read articles from Sanjana Balaji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
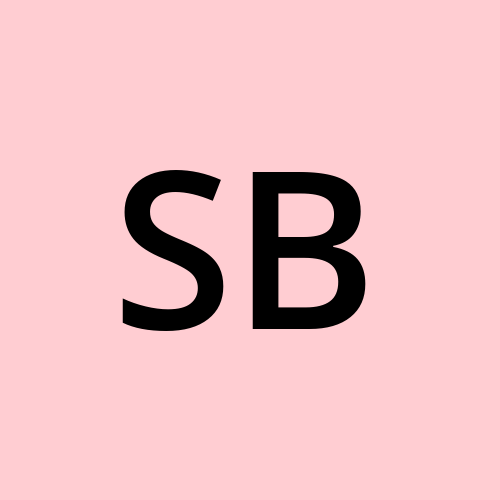