DSA Week 1: Master Data Types, Loops, and Conditional Operators to Kickstart Your Coding Journey!
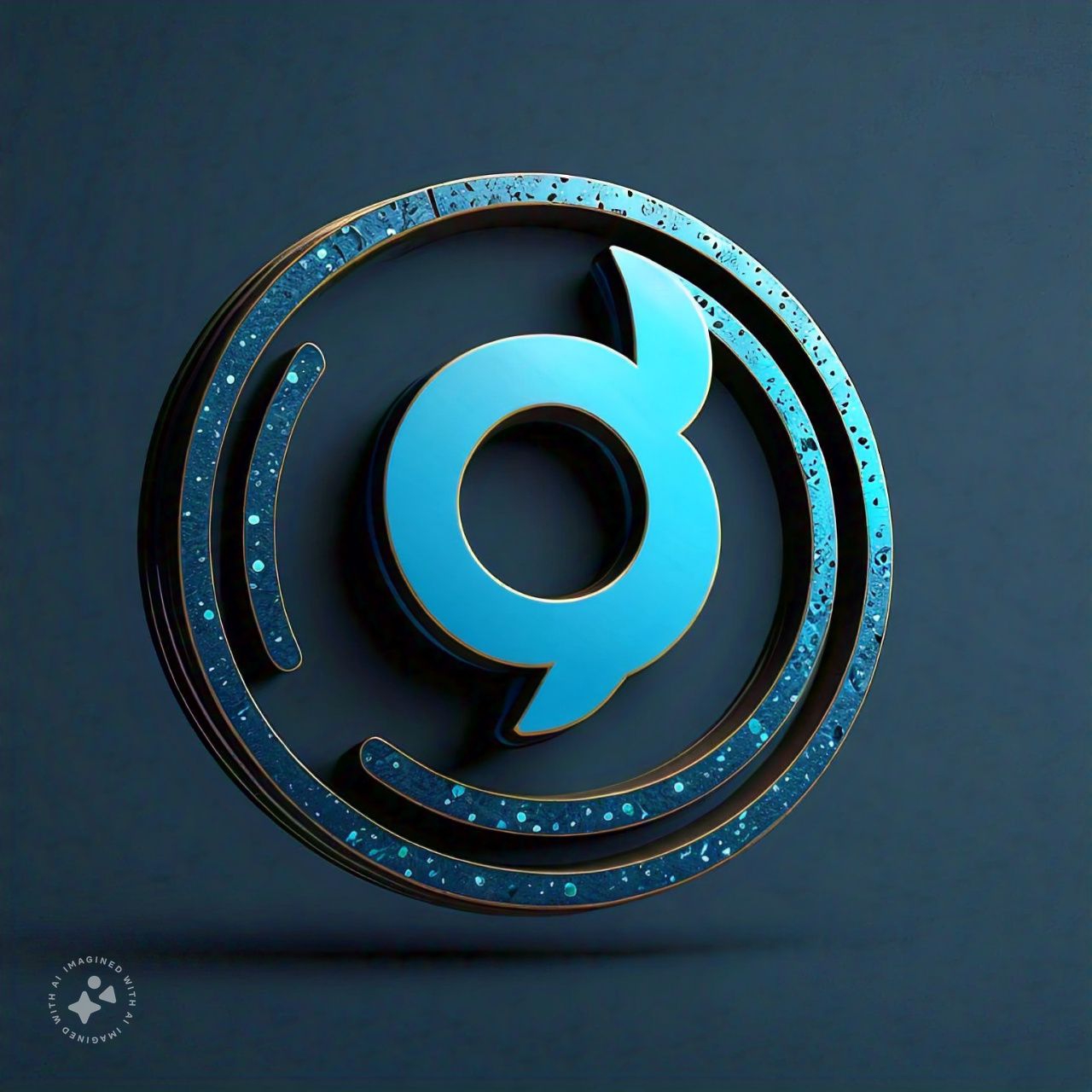
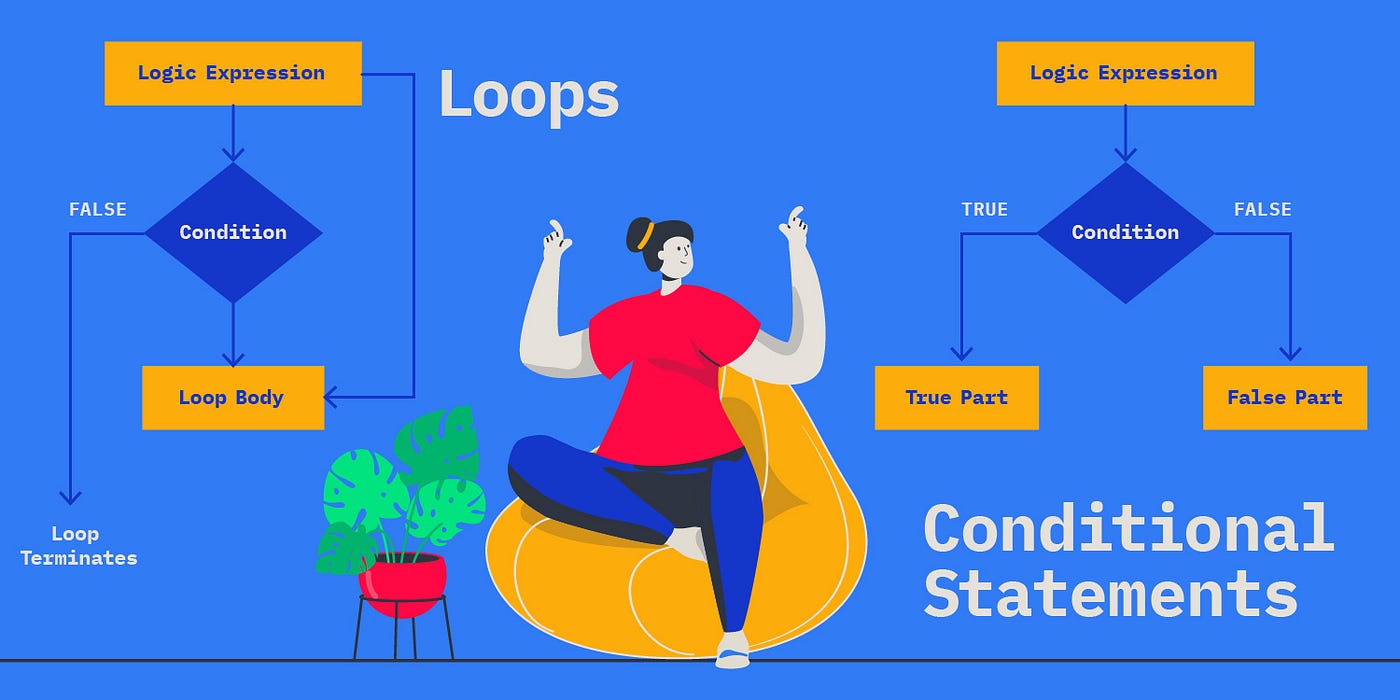
Week 1-2: Fundamentals
Day 1-2: Data Types
Learning: int, float, boolean, string, char, long, double, bigint
Practice: Exercises on each data type
Day 3-4: Condition Operators
Learning: if-else, switch statement, ternary operator
Practice: Conditional problems
Day 5-7: Loops
Learning: for, while, do-while
Practice: Loop-based problems
Data type
1 . Integer (int)
integer is a fundamental data type in programming that represents whole numbers without any fractional or decimal parts. Integers can be either positive, negative, or zero.
integer store in memory using fixed amount of space typically 4 byte or 8 byte or , 32bits , 64bit based on the system
A 32-bit or 4byte integer can hold values from approximately -2 billion to +2 billion.
A 64-bit or 4byte integer can hold values from approximately -9 quintillion to +9 quintillion.
Integers are commonly used for tasks involving counting, indexing arrays, looping, and any other situation where whole numbers are sufficient or required.
example
# Declare an integer variable
x = 42
# Perform arithmetic operations
y = x + 8 # y now holds the value 50
# Print the result
print(y)
2 . Float
float is also a type of number with decimal or in expontial form
float reprent real number with decimal or output value of fraction .
# declare an flot variable
x = 4.012
# performing arthimatic operator
y = 3.02 + x # now y is hold the value 7.032
# print the result
print(y)
3 . boolean
boolean is nothing but true or false
Control Flow: Used in conditional statements like
if
,else
,while
, andfor
loops to control the flow of a program.Logical Operations: Used in logical operations such as AND, OR, and NOT.
is_active = True
is_valid = False
if is_active:
print("The system is active.")
else:
print("The system is not active.")
4 . String
- The
string
is a sequence of characters, typically used to represent text. Different programming languages implement strings in various ways, but the core concept remains the same.
Characteristics of the string
Data Structure:
Immutable vs. Mutable:
Immutable Strings: In languages like Python and Java, strings are immutable, meaning once a string is created, it cannot be changed. Any modification results in a new string.
Mutable Strings: In some languages like C++ (with
std::string
) or Python (withbytearray
), strings can be mutable, meaning they can be changed in place.
String Literals:
- Represented by enclosing characters in quotes. Single (
'
), double ("
), or triple quotes ('''
or"""
) can be used depending on the language.
- Represented by enclosing characters in quotes. Single (
Common Operations:
Concatenation: Combining two or more strings.
Substring: Extracting a part of a string.
Length: Determining the number of characters in a string.
Indexing: Accessing individual characters.
Slicing: Extracting a part of a string using indices.
Searching: Finding a substring within a string.
Replacement: Replacing parts of a string with another string.
Splitting and Joining: Breaking a string into a list of substrings and vice versa.
Examples in Different Languages:
Python:
pythonCopy code # String creation name = "John Doe" greeting = 'Hello, world!' multiline = """This is a multi-line string.""" # Concatenation full_greeting = greeting + " " + name # Substring first_name = name[0:4] # Length length_of_greeting = len(greeting) # Indexing first_char = name[0] # Slicing last_name = name[5:] # Searching is_hello_present = "Hello" in greeting # Replacement new_greeting = greeting.replace("world", "Python") # Splitting and Joining words = greeting.split(" ") joined = " ".join(words)
5 . char
The char
data type is used to store single characters. It usually takes up 1 byte (8 bits) of memory.
Characteristics:
Stores a single character or ASCII value.
Enclosed in single quotes (e.g.,
'A'
,'b'
).
Examples:
javaCopy code
char letter = 'A';
System.out.println(letter); // Output: A
Python: Python does not have a distinct char
type; instead, it uses a string of length 1.
pythonCopy code
letter = 'A'
print(letter) # Output: A
6 . long
The long
data type is used to store large integer values. It typically takes up more memory than standard integer types (like int
).
Characteristics:
In many languages, it is at least 32 bits, often 64 bits.
Used when the range of the
int
type is insufficient.
Examples:
C++:
cppCopy code
long largeNumber = 1234567890L;
std::cout << largeNumber << std::endl; // Output: 1234567890
Python:
Python's int
type can handle arbitrary precision and acts like long
in other languages.
pythonCopy code
largeNumber = 12345678901234567890
print(largeNumber) // Output: 12345678901234567890
7 .double
The double
data type is used to store floating-point numbers (decimals). It provides double the precision of the float
type.
Characteristics:
Typically 64 bits in size.
Used for calculations requiring significant digits and precision.
Examples:
Java:
javaCopy code
double pi = 3.141592653589793;
System.out.println(pi); // Output: 3.14159
Python:
Python's float
type is equivalent to double
in other languages.
pythonCopy code
pi = 3.141592653589793
print(pi) # Output: 3.141592653589793
8 . BigInt
The BigInt
data type is used to store arbitrarily large integers, surpassing the limits of standard integer types.
Characteristics:
Provides arbitrary precision.
Useful for applications requiring very large numbers, like cryptography.
Examples:
JavaScript:
javascriptCopy code
let bigInt = BigInt("1234567890123456789012345678901234567890");
console.log(bigInt); // Output: 1234567890123456789012345678901234567890n
Python: Python's int
type can handle arbitrary precision and can be used similarly to BigInt
.
pythonCopy code
bigInt = 1234567890123456789012345678901234567890
print(bigInt) // Output: 1234567890123456789012345678901234567890
Summary
char: Used to store single characters. Commonly found in languages like C, C++, and Java.
long: Used for large integers that exceed the range of standard
int
. Found in languages like C, C++, Java, and Python (as standardint
).double: Used for double-precision floating-point numbers, providing greater accuracy for decimal values.
BigInt: Used for storing very large integers with arbitrary precision, available in languages like JavaScript and Python, and as
BigInteger
in Java.
💡 ya we learn the basic data structure
Conditional operator
if-else, switch statement, ternary operator are the main conditional operator
ussages this operator work on the base of boolean datatype
Control flow statements like if-else
, switch
, and the ternary operator are essential for making decisions in code based on conditions. Here's an overview of each, along with examples in various programming languages.
if-else Statement
The if-else
statement allows you to execute certain parts of your code based on conditions.
Syntax:
plaintextCopy code
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Examples:
Python:
pythonCopy code
age = 20
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
switch Statement
The switch
statement allows you to execute one block of code out of many based on the value of a variab
let grade = 'B';
switch (grade) {
case 'A':
console.log("Excellent!");
break;
case 'B':
console.log("Good!");
break;
case 'C':
console.log("Fair!");
break;
default:
console.log("Invalid grade.");
}
Ternary Operator
The ternary operator is a shorthand for if-el
se
that assigns a value based on a condition. It is also known as the conditional operator.
Syntax:
plaintextCopy code
condition ? expression_if_true : expression_if_false
Examples:
Python:
pythonCopy code
age = 20
status = "adult" if age >= 18 else "minor"
print(f"You are an {status}.")
let age = 20;
let status = (age >= 18) ? "adult" : "minor";
console.log("You are an " + status + ".");
Practical Applications:
if-else: Used for simple, straightforward decision making with two or more branches.
switch: Best used when there are multiple potential values for a single variable and each value needs to be handled differently.
ternary operator: Ideal for concise conditional assignments.
Understanding and using these control flow statements effectively is key to implementing decision-making logic in your programs.
<aside> 💡 yaw we completed data type
</aside>
Loops
A loop is a fundamental concept in programming that allows you to execute a block of code multiple times. Loops are used to perform repetitive tasks, iterate over data structures, and handle repeated operations efficiently. Here’s a detailed explanation of what loops are, why they are used, and examples in different programming languages:
Why Use Loops?
Repetition: Perform a task repeatedly without writing redundant code.
Iteration: Traverse elements in data structures like arrays, lists, or collections.
Automation: Automate repetitive tasks like reading data, updating records, or processing information.
Conditional Execution: Continue executing code as long as certain conditions are met.
Types of Loops
While Loop: Used when the number of iterations is not known and depends on a condition.
Do-While Loop: Similar to a while loop but guarantees at least one execution of the loop body.
For-Each Loop: Simplified loop for traversing collections or arrays.
For Loop
Structure:
Initialization: Set the starting point.
Increment/Decrement: Update the loop control variable.
Example in JavaScript:
// Print numbers from 1 to 5
for (let i = 1; i <= 5; i++) {
console.log(i);
}
// Output: 1 2 3 4 5
Example in Python:
# Print numbers from 1 to 5
for i in range(1, 6):
print(i)
# Output: 1 2 3 4 5
While Loop
// Print numbers from 1 to 5
let i = 1;
while (i <= 5) {
console.log(i);
i++;
}
// Output: 1 2 3 4 5
Example in Python:
# Print numbers from 1 to 5
i = 1
while i <= 5:
print(i)
i += 1
# Output: 1 2 3 4 5
Do-While Loop
Structure:
- Execute the loop body at least once, then check the condition.
Example in JavaScript:
// Print numbers from 1 to 5
let i = 1;
do {
console.log(i);
i++;
} while (i <= 5);
// Output: 1 2 3 4 5
Example in Python: Python does not have a native do-while
loop but you can simulate it:
# Print numbers from 1 to 5
i = 1
while True:
print(i)
i += 1
if i > 5:
break
# Output: 1 2 3 4 5
For-Each Loop
Structure:
- Iterate over elements in a collection or array.
Example in JavaScript:
// Print each element in an array
const arr = [1, 2, 3, 4, 5];
arr.forEach(element => {
console.log(element);
});
// Output: 1 2 3 4 5
Example in Python:
# Print each element in a list
arr = [1, 2, 3, 4, 5]
for element in arr:
print(element)
# Output: 1 2 3 4 5
Practical Applications
Data Processing: Read and process data from files or databases.
Algorithms: Implement algorithms like searching, sorting, and iterating through data structures.
User Input: Continuously prompt user input until a valid response is received.
Summary
For Loop: Best for known iteration counts.
Do-While Loop: Ensures the loop body is executed at least once.
For-Each Loop: Simplifies iteration over collections.
Loops are powerful tools in programming that help automate repetitive tasks, process data efficiently, and implement complex algorithms. Understanding how and when to use different types of loops is crucial for writing effective and efficient code.
Subscribe to my newsletter
Read articles from ritiksharmaaa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
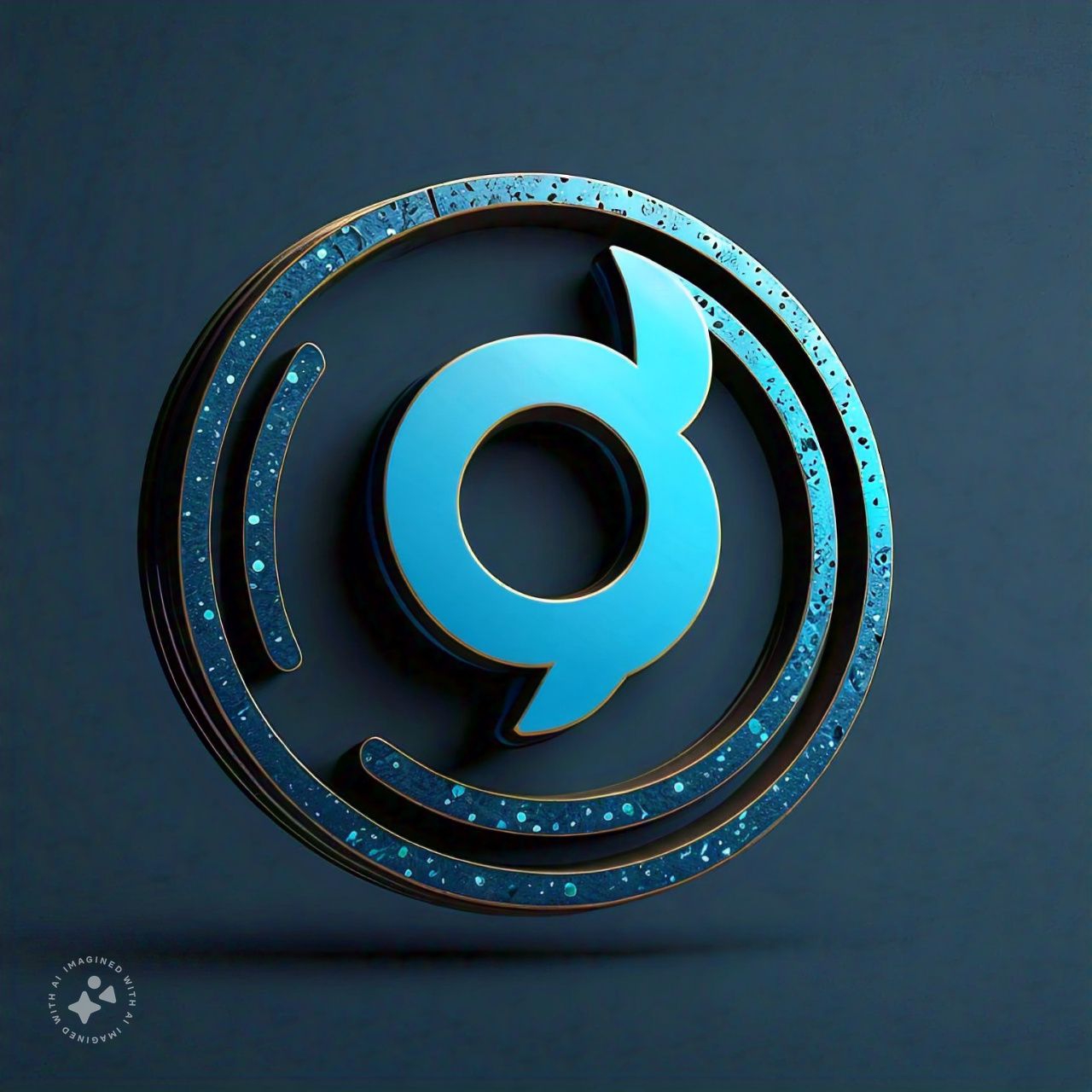
ritiksharmaaa
ritiksharmaaa
Hy this is me Ritik sharma . i am software developer