Improve Content Loading: The Benefits of Infinite Scrolling
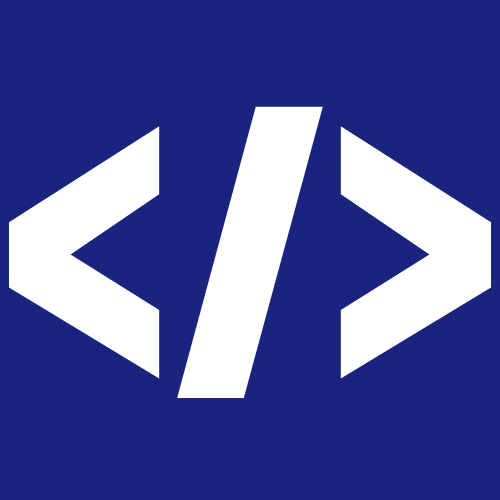
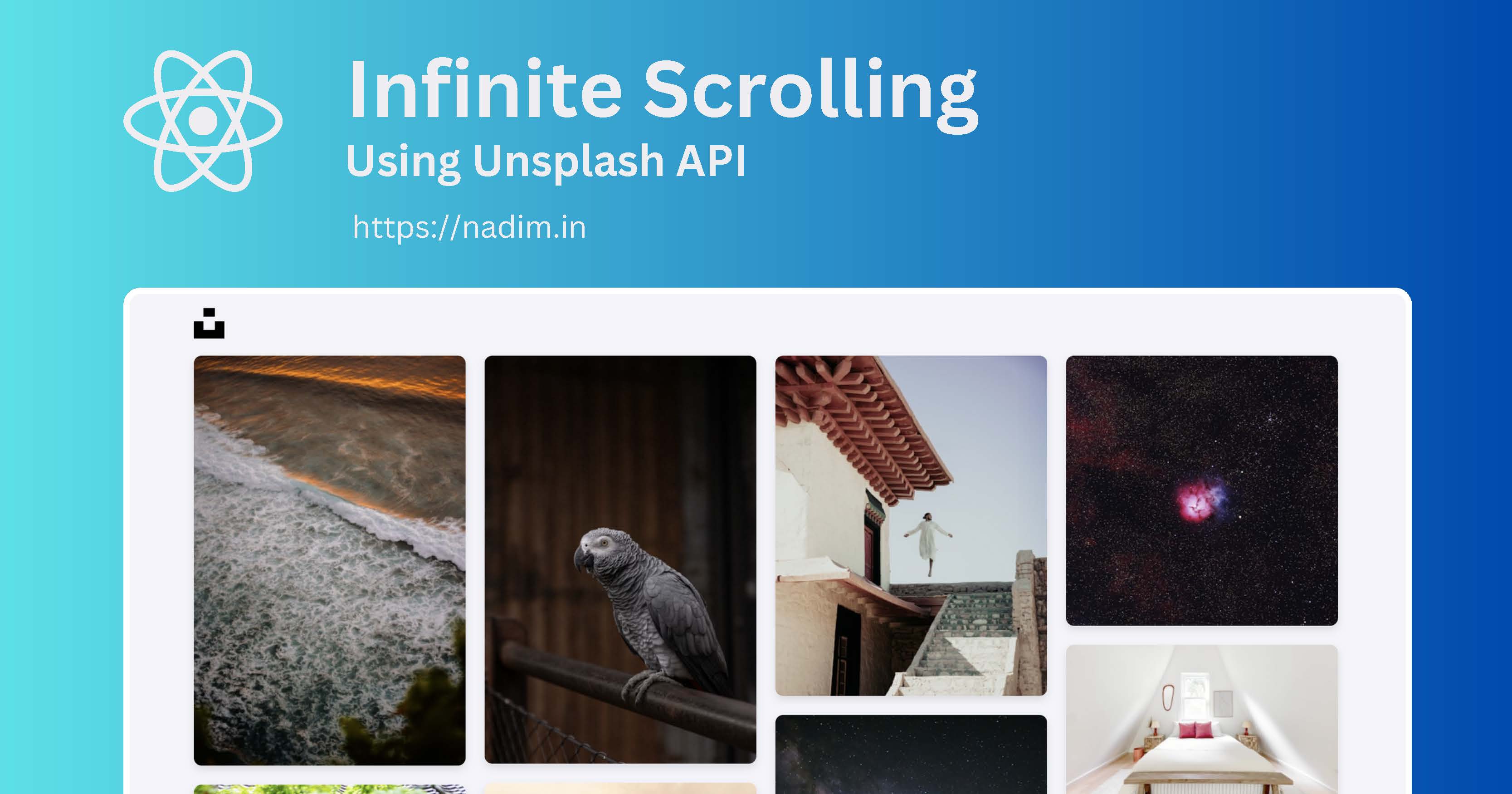
Introduction
In today's fast-paced digital world, users expect seamless and uninterrupted browsing experiences. Infinite scrolling is a web design technique that meets this expectation by continuously loading content as users scroll down a page. This approach eliminates the need for pagination, creating a more engaging and intuitive user experience. In this blog post, we'll explore what infinite scrolling is, its benefits and drawbacks, and how to implement it in a React application using an API.
What is Infinite Scrolling?
Infinite scrolling, also known as endless scrolling, is a web design pattern where additional content is loaded dynamically as the user scrolls down a page. This method is widely used on social media platforms like Facebook, Twitter, and Instagram, as well as news websites and e-commerce stores. Instead of clicking through pagination links, users can continue scrolling to access more content.
Benefits of Infinite Scrolling
Improved User Experience: Users can consume content seamlessly without interruption, making the browsing experience more enjoyable.
Increased Engagement: Infinite scrolling can keep users engaged on a site longer, as they are more likely to continue scrolling rather than clicking through pages.
Modern Design: It gives websites a modern, sleek look and feel, aligning with contemporary web design trends.
Mobile Friendliness: Infinite scrolling is particularly useful on mobile devices where tapping on small pagination links can be cumbersome.
Drawbacks of Infinite Scrolling
Performance Issues: Loading large amounts of data can slow down a website and increase load times.
Navigation Difficulties: Users may find it challenging to return to specific content or track their browsing progress.
SEO Challenges: Infinite scrolling can make it harder for search engines to index all content, potentially impacting SEO.
Accessibility Concerns: It may not be suitable for users with disabilities who rely on screen readers or keyboard navigation.
Implementing Infinite Scrolling in React
To demonstrate how to implement infinite scrolling, we'll create a simple image gallery using React and the Unsplash API. The gallery will load more images as the user scrolls down.
Step 1: Set Up the React Project
First, set up a new React project using Create React App:
npx create-react-app infinite-scroll
cd infinite-scroll
Step 2: Install Dependencies
We'll use the Fetch API to request data from the Unsplash API. No additional dependencies are needed.
Step 3: Create the InfiniteScroll Component
Create a new file InfiniteScroll.js
and add the following code:
import React, { useCallback, useState, useEffect } from 'react';
import '../App.css'; // Make sure to update the path if your CSS file is located elsewhere
function InfiniteScroll() {
const [items, setItems] = useState([]);
const [hasMore, setHasMore] = useState(true);
const [page, setPage] = useState(1);
const fetchItems = useCallback(async () => {
try {
const response = await fetch(`https://api.unsplash.com/photos?page=${page}&client_id=YOUR ACCESS KEY`);
const data = await response.json();
setItems(prevItems => [...prevItems, ...data]);
if (data.length === 0) {
setHasMore(false);
}
} catch (error) {
console.error('Error fetching items:', error);
}
}, [page]);
useEffect(() => {
fetchItems();
}, [fetchItems]);
const handleScroll = () => {
if (window.innerHeight + document.documentElement.scrollTop >= document.documentElement.offsetHeight - 500 && hasMore) {
setPage(prevPage => prevPage + 1);
}
};
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => window.removeEventListener('scroll', handleScroll);
}, [hasMore]);
return (
<div className="container">
{items.map((item, index) => (
<figure key={index}>
<img src={item.urls.small} alt={item.alt_description} className="image card" />
</figure>
))}
{hasMore && <p className="loading">Loading more items...</p>}
</div>
);
}
export default InfiniteScroll;
Replace YOUR_ACCESS_KEY
with your Unsplash API access key.
Step 4: Style the Component
Create or update the App.css
file with the following styles to make the gallery visually appealing:
body {
background-color: #f4f4f9;
font-family: 'Roboto', Arial, Helvetica, sans-serif;
color: #333;
margin: 0;
padding: 20px;
display: flex;
justify-content: center;
}
.logo{
height: 50px;
width: 50px ;
border-radius: 8px;
}
.card{
max-width: 100%;
display: block;
border-radius: 8px;
transition: transform 0.3s ease-in-out;
}
img:hover {
transform: scale(1.05);
}
figure {
margin: 0;
display: grid;
grid-template-rows: 1fr auto;
margin-bottom: 20px;
break-inside: avoid;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
overflow: hidden;
transition: box-shadow 0.3s ease-in-out;
}
figure:hover {
box-shadow: 0 8px 16px rgba(0, 0, 0, 0.2);
}
figure > img {
grid-row: 1 / -1;
grid-column: 1;
}
figure a {
color: #333;
text-decoration: none;
}
figcaption {
grid-row: 2;
grid-column: 1;
background-color: rgba(255, 255, 255, 0.9);
padding: 0.5em 1em;
justify-self: start;
font-size: 0.9em;
}
.container {
column-count: 4;
column-gap: 20px;
max-width: 1200px;
width: 100%;
}
@media (max-width: 1200px) {
.container {
column-count: 3;
}
}
@media (max-width: 900px) {
.container {
column-count: 2;
}
}
@media (max-width: 600px) {
.container {
column-count: 1;
}
}
Step 5: Use the Component
In your App.js
file, import and use the InfiniteScroll
component:
import React from 'react';
import InfiniteScroll from './InfiniteScroll';
import './App.css';
function App() {
return (
<div className="App">
<InfiniteScroll />
</div>
);
}
export default App;
Conclusion
Infinite scrolling is a powerful technique to enhance user experience by providing a seamless and engaging way to browse content. While it has its drawbacks, careful implementation can mitigate many of these issues. By following the steps outlined above, you can add infinite scrolling to your React applications, creating a modern and user-friendly interface. Experiment with the design and functionality to best suit your project's needs and always keep user experience at the forefront of your design decisions.
Subscribe to my newsletter
Read articles from Nadim Anwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
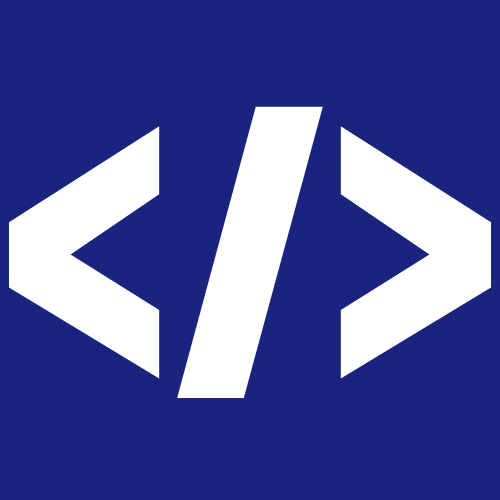
Nadim Anwar
Nadim Anwar
As an experienced front-end developer, I have expertise in building scalable and responsive web applications using modern technologies like ReactJS, Next.js, and TailwindCSS. With a solid understanding of JavaScript and a strong design sense, I excel at turning concepts into visually appealing and practical user interfaces. I am committed to continuously enhancing my skills and staying abreast of the latest industry trends to provide cutting-edge solutions.