Comprehensive Guide to DBT (Data Build Tool)

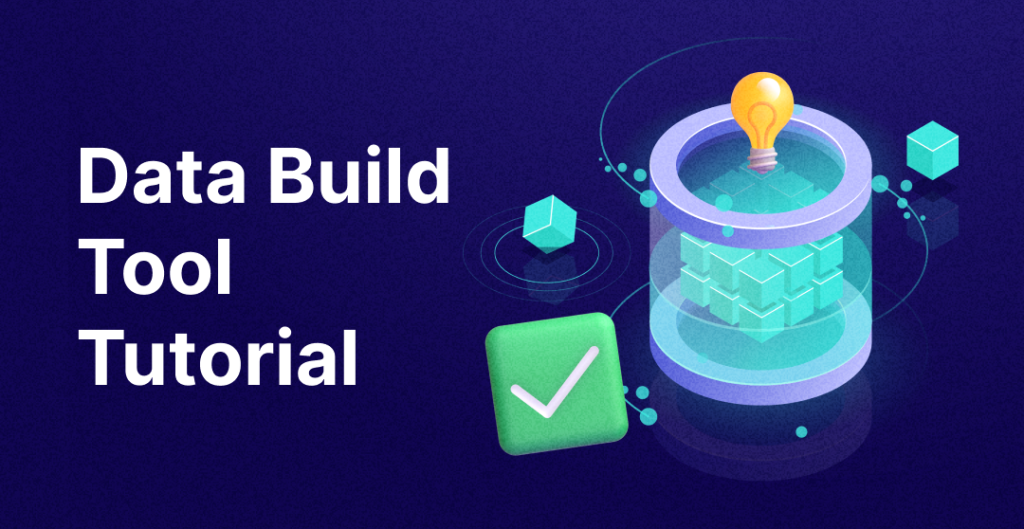
What is dbt?
dbt (Data Build Tool) is an open-source command-line tool that helps analysts and engineers transform data in their data warehouse. It allows you to manage your data transformations with SQL in a version-controlled and collaborative environment. dbt works by writing SQL queries, which are then run against your data warehouse, transforming raw data into the clean, structured formats needed for analysis.
Key Features of dbt
Modularity: dbt encourages the use of modular SQL code, making it easy to reuse and maintain your transformations.
Version Control: By integrating with Git, dbt allows you to track changes to your SQL code over time.
Testing: dbt includes built-in testing features to ensure data quality and correctness.
Documentation: Automatically generate documentation for your data models and transformations.
Deployment: Easily deploy your transformations to your data warehouse with a single command.
How dbt Works
Models: In dbt, models are simply SQL files that define transformations. Each model is a SELECT statement, which transforms data from one or more source tables into a new table or view.
Sources: Sources in dbt are the raw tables in your data warehouse. They are the starting point for your transformations.
Seeds: Seed files are CSV files that dbt can load into your data warehouse. These can be used as reference tables in your transformations.
Snapshots: Snapshots capture the state of your data at a particular point in time. This is useful for slowly changing dimensions or historical analysis.
Macros: Macros in dbt are reusable snippets of SQL or Jinja (a templating language) that can be used to DRY (Don't Repeat Yourself) up your code.
Setting Up dbt
Installation: dbt can be installed using pip:
pip install dbt
Initializing a Project: Create a new dbt project with:
dbt init my_project
Configuring dbt: Configure your dbt project by editing the
profiles.yml
file. This file contains information about your data warehouse connection.
Building Your First dbt Model
Create a Model: In the
models
directory, create a new SQL file, e.g.,my_model.sql
:-- my_model.sql select id, name, date, revenue from raw_data.sales
Run the Model: Run your dbt model with:
dbt run
Advanced dbt Features
Testing: Create tests to ensure data quality. For example, in
tests
directory:version: 2 models: - name: my_model tests: - unique: column_name: id - not_null: column_name: name
Documentation: Generate and view documentation with:
dbt docs generate dbt docs serve
Snapshots: Create a snapshot with a YAML configuration:
snapshots: - name: sales_snapshot target_schema: snapshots strategy: timestamp unique_key: id updated_at: updated_at check_cols: [revenue, date]
Macros and Variables: Use macros and variables for reusable code:
-- macros/get_columns.sql {% macro get_columns(table_name) %} select column_name from information_schema.columns where table_name = '{{ table_name }}' {% endmacro %}
Deployment: Deploy your models to different environments using different profiles in
profiles.yml
.
dbt Use Cases and Implementations
Data Warehousing: Transforming raw data into structured, analytics-ready data. For example, transforming raw sales data into a dimensional model with fact and dimension tables.
ETL (Extract, Transform, Load) Processes: Automating ETL processes using dbt to transform data after it has been loaded into a data warehouse.
Data Quality Assurance: Implementing tests in dbt to ensure data quality. For example, checking for null values, unique constraints, and foreign key relationships.
Data Documentation and Lineage: Using dbt’s documentation features to create a comprehensive data catalog, which includes data lineage. This helps in understanding how data flows through the system and how different models are related.
Analytics and Reporting: Preparing data for BI tools and reporting. For example, creating aggregate tables for faster query performance in dashboards.
Implementation Example: Transforming Sales Data
Scenario
Suppose you have raw sales data in your data warehouse, and you want to transform it into a model that can be used for reporting and analysis.
Step-by-Step Implementation
Define Sources: Create a source configuration file to define your raw data tables.
version: 2 sources: - name: raw_data tables: - name: sales - name: customers - name: products
Create Models: Define models to transform the raw data into the desired format.
Sales Model:
-- models/staging/stg_sales.sql with sales as ( select id, customer_id, product_id, sale_date, amount from {{ source('raw_data', 'sales') }} ) select * from sales
Customer Model:
-- models/staging/stg_customers.sql with customers as ( select id, name, email from {{ source('raw_data', 'customers') }} ) select * from customers
Product Model:
-- models/staging/stg_products.sql with products as ( select id, name, category from {{ source('raw_data', 'products') }} ) select * from products
Join Models: Create a final model that joins the staging models to create a comprehensive view.
Final Sales Model:
-- models/marts/sales_summary.sql with sales as ( select * from {{ ref('stg_sales') }} ), customers as ( select * from {{ ref('stg_customers') }} ), products as ( select * from {{ ref('stg_products') }} ) select sales.id as sale_id, customers.name as customer_name, products.name as product_name, sales.sale_date, sales.amount from sales join customers on sales.customer_id = customers.id join products on sales.product_id = products.id
Run Models: Execute the models to transform the data.
dbt run
Test Models: Implement tests to ensure the quality of the transformed data.
Tests for Sales Summary:
version: 2 models: - name: sales_summary columns: - name: sale_id tests: - not_null - unique - name: customer_name tests: - not_null - name: product_name tests: - not_null - name: sale_date tests: - not_null
Run the tests:
dbt test
Document Models: Generate documentation for the project.
dbt docs generate dbt docs serve
Conclusion
dbt is a powerful tool that bridges the gap between data engineering and analytics, enabling teams to transform data more efficiently and accurately. By leveraging software engineering best practices, dbt ensures that your data transformations are maintainable, testable, and version-controlled. As data continues to be a critical asset for businesses, tools like dbt will play an increasingly important role in the modern data stack.
Subscribe to my newsletter
Read articles from Abhishek Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Jaiswal
Abhishek Jaiswal
As a dynamic and motivated B.Tech student specializing in Computer Science and Engineering, I am deeply driven by my unwavering passion for harnessing the transformative potential of data engineering, devops, and cloud technologies to tackle multifaceted problems. Armed with a solid foundation in the Python programming language, I possess an extensive skill set and proficiency in utilizing a comprehensive stack of technical tools, including Apache Airflow, Apache Spark, SQL, MongoDB, and data warehousing solutions like Snowflake. Throughout my academic journey, I have diligently honed my abilities in problem-solving, software development methodologies, and fundamental computer science principles. My adeptness in data structures and algorithms empowers me to approach challenges with efficiency and creativity, enabling me to break down complex problems into manageable tasks and craft elegant solutions. In addition to my technical prowess, I bring exceptional communication and collaboration skills to the table, allowing me to thrive in team settings and make meaningful contributions to collaborative projects. I am highly adaptable and excel in dynamic environments that foster continuous learning and growth, as they provide me with the opportunity to expand my knowledge and refine my skills further.