Backpropagation in Deep Learning: The Key to Optimizing Neural Networks
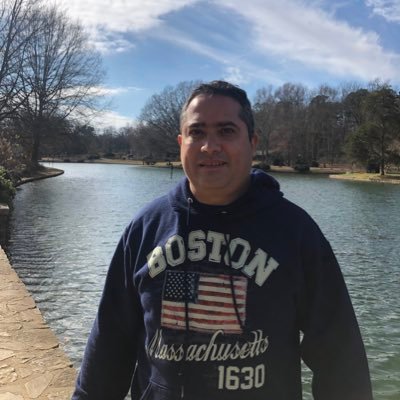
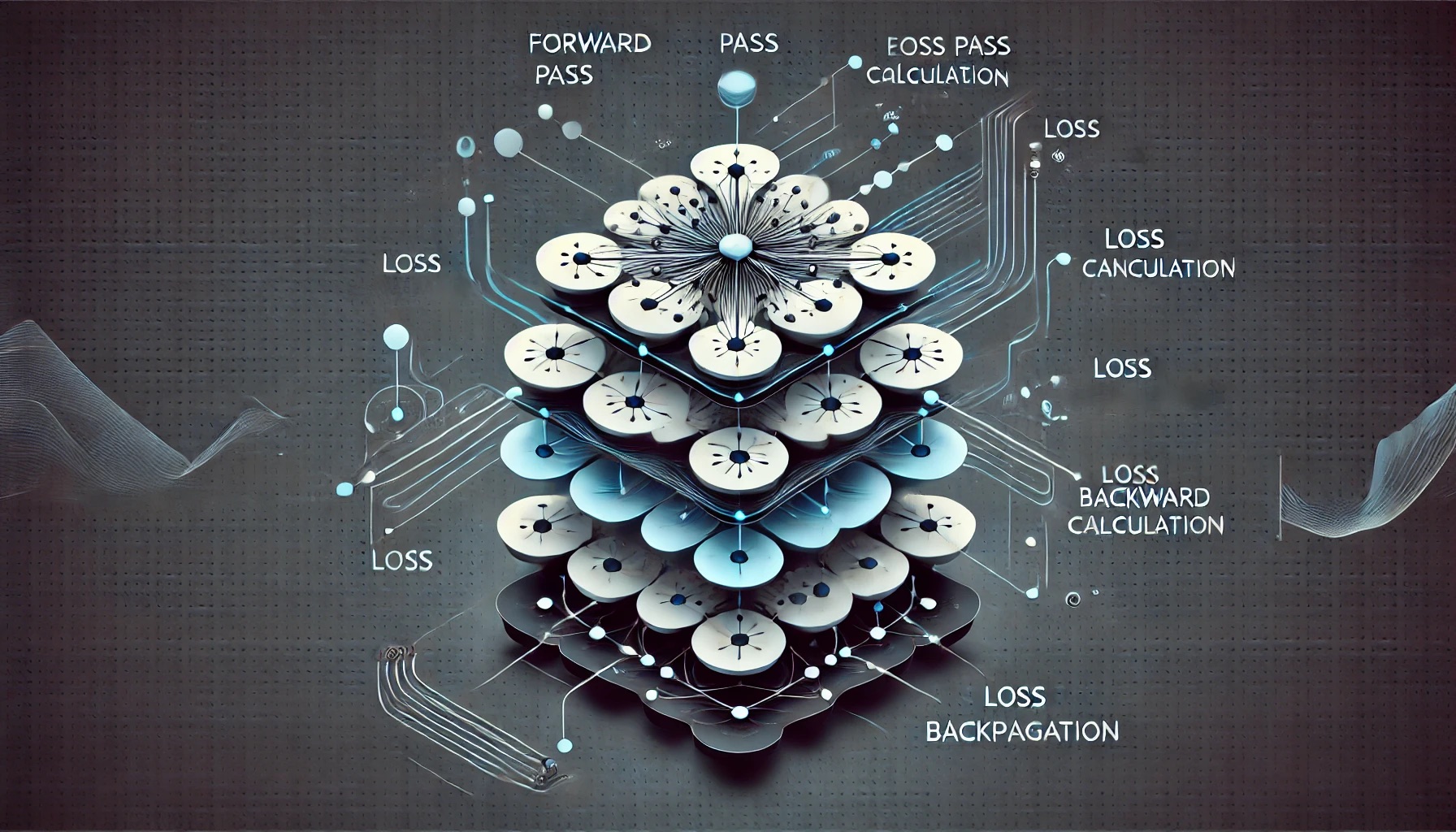
Have you ever wondered how neural networks learn?
Have you ever wondered how your smartphone recognizes your face or how virtual assistants understand your voice?
The secret lies in a powerful algorithm called backpropagation.
Imagine trying to teach a child to ride a bike without giving them any feedback. It would be frustrating and time-consuming. This is exactly what happens to a neural network without backpropagation—learning becomes inefficient and impractical.
This article delves into backpropagation, explaining how it revolutionized machine learning by enabling efficient training of deep neural networks.
By the end of this article, you will understand how backpropagation works, why it's crucial, and how it powers advancements in AI technologies like image recognition and natural language processing.
Understanding Backpropagation
What is Backpropagation?
Backpropagation is a supervised learning algorithm used for training artificial neural networks.
It computes the gradient of the loss function with respect to each weight by the chain rule.
This process allows the network to update its weights and biases, minimizing the error in predictions.
Without backpropagation, training deep neural networks would be inefficient and impractical.
The Importance of Backpropagation
Backpropagation revolutionized the field of machine learning by enabling the training of deep neural networks.
Before its introduction, training multi-layered networks was extremely challenging due to the vanishing gradient problem.
By efficiently calculating gradients, backpropagation allows for the effective training of complex models.
This capability has led to breakthroughs in image recognition, natural language processing, and many other AI applications.
The Mechanics of Backpropagation
The Forward Pass
In the forward pass, the neural network processes the input data to generate predictions.
Each layer of the network applies a set of weights and an activation function to the input from the previous layer.
The output of the final layer is the network's prediction.
x (input) -> [Layer 1] -> [Layer 2] -> ... -> [Layer n] -> ŷ (output)
The Loss Function
The loss function measures the difference between the predicted output and the actual target.
Common loss functions include mean squared error for regression tasks and cross-entropy loss for classification tasks.
The goal of training is to minimize this loss function, thereby improving the accuracy of the network's predictions.
L(ŷ, y) = measure of difference between prediction ŷ and true value y
Now that we have a way to quantify error, how do we use this information to improve our network?
Enter backpropagation to the game.
Backpropagation: The Learning Engine
Backpropagation is all about efficiently computing how each parameter in the network contributes to the overall error.
It does this by cleverly applying the chain rule of calculus, propagating the error gradient backwards through the network.
Chain Rule
The chain rule is a fundamental concept in calculus that is crucial for backpropagation.
It allows the computation of the derivative of a composite function by breaking it down into simpler parts.
In the context of neural networks, the chain rule helps in computing the gradient of the loss function with respect to each weight.
∂L/∂θ = (∂L/∂ŷ) * (∂ŷ/∂z) * (∂z/∂θ)
Where:
L is the loss
ŷ is the network output
z is the input to the activation function
θ is any weight or bias in the network
The Backward Pass: Propagating Gradients
The backward pass is where the magic happens.
We start at the output layer and work our way backwards, computing gradients for each parameter along the way.
1- Compute the gradient of the loss with respect to the output: ∂L/∂ŷ 2- For each layer, from output to input: - Compute the gradient of the layer output with respect to its input: ∂z/∂x - Compute the gradient of the layer output with respect to its weights and biases: ∂z/∂w, ∂z/∂b - Propagate the gradient to the previous layer: ∂L/∂x = (∂L/∂z) * (∂z/∂x)
Gradient Descent: Optimizing the Network
Once we have our gradients, we use them to update the network's parameters.
This is typically done using an optimization algorithm like gradient descent.
θ_new = θ_old - η * ∂L/∂θ
Where η (eta) is the learning rate, controlling how big of a step we take in the direction of the negative gradient.
By repeating this process many times over large datasets, the network gradually improves its performance, learning to make increasingly accurate predictions.
Computational Efficiency
Backpropagation's elegance lies in its efficiency.
It allows us to compute gradients for networks with millions or even billions of parameters in a computationally feasible way.
This scalability is what enables the training of deep neural networks, unlocking their impressive capabilities in areas like computer vision, natural language processing, and reinforcement learning.
Vanishing and Exploding Gradients: Challenges in Deep Networks
As powerful as backpropagation is, it's not without its challenges.
In very deep networks, gradients can sometimes become vanishingly small (vanishing gradient problem) or explosively large (exploding gradient problem) as they're propagated backwards.
This can make training deep networks difficult or unstable.
Techniques like careful weight initialization, normalized activation functions (e.g., ReLU), and architectural innovations like skip connections (as in ResNets) help mitigate these issues.
These advancements have enabled the training of ever-deeper networks, pushing the boundaries of what's possible in AI.
Implementing Backpropagation: A Practical Example
To truly understand backpropagation, let's walk through a simple implementation.
We'll create a basic neural network with one hidden layer and train it to approximate a simple function.
import numpy as np
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(x):
return x * (1 - x)
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1], 4)
self.weights2 = np.random.rand(4, 1)
self.y = y
self.output = np.zeros(y.shape)
def feedforward(self):
self.layer1 = sigmoid(np.dot(self.input, self.weights1))
self.output = sigmoid(np.dot(self.layer1, self.weights2))
def backprop(self):
d_weights2 = np.dot(self.layer1.T, 2*(self.y - self.output) \
* sigmoid_derivative(self.output))
d_weights1 = np.dot(self.input.T, np.dot(2*(self.y - \
self.output) * sigmoid_derivative(self.output), \
self.weights2.T) * sigmoid_derivative(self.layer1))
self.weights1 += d_weights1
self.weights2 += d_weights2
def train(self, iterations):
for _ in range(iterations):
self.feedforward()
self.backprop()
# Example usage
X = np.array([[0,0,1], [0,1,1], [1,0,1], [1,1,1]])
y = np.array([[0], [1], [1], [0]])
nn = NeuralNetwork(X, y)
nn.train(1500)
Beyond Basic Backpropagation: Advanced Techniques
As neural networks have evolved, so too have the techniques for training them.
While standard backpropagation remains the core algorithm, several advanced methods have been developed to enhance its effectiveness and efficiency.
Stochastic Gradient Descent (SGD) and Mini-batch Training
Instead of computing gradients over the entire dataset (which can be computationally expensive), SGD computes gradients on small subsets (mini-batches) of the data.
This approach introduces some noise into the optimization process, which can actually help the network escape local minima and potentially find better solutions.
Momentum and Adaptive Learning Rates
Techniques like momentum and adaptive learning rate algorithms (e.g., Adam, RMSprop) help accelerate training and navigate the loss landscape more effectively.
These methods adjust the learning rate dynamically based on the recent history of gradients, allowing for faster convergence and better handling of different scales of gradients across parameters.
Batch Normalization
Batch normalization normalizes the inputs to each layer, which can dramatically speed up training and allow for higher learning rates.
It helps mitigate the internal covariate shift problem, where the distribution of each layer's inputs changes during training, making it harder for subsequent layers to adapt.
Regularization Techniques
Methods like L1/L2 regularization and dropout help prevent overfitting by adding constraints to the network's parameters or randomly deactivating neurons during training.
Conclusion
Backpropagation is the backbone of deep learning, enabling neural networks to learn from data and improve their performance.
Its ability to efficiently compute gradients and update network parameters has driven significant advancements in AI.
As we continue to innovate and address its challenges, backpropagation will remain a fundamental tool in the quest for intelligent machines.
Whether you're a seasoned practitioner or a newcomer to deep learning, understanding backpropagation is crucial.
By mastering this powerful algorithm, you can harness the full potential of neural networks and contribute to the ongoing revolution in artificial intelligence.
PR: If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
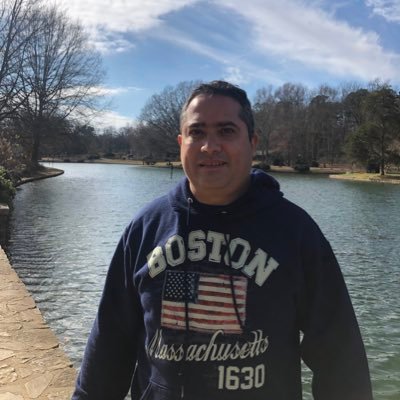
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io