Simplify Your Git Management: Automate Merged Branch Deletion in GitHub with Python
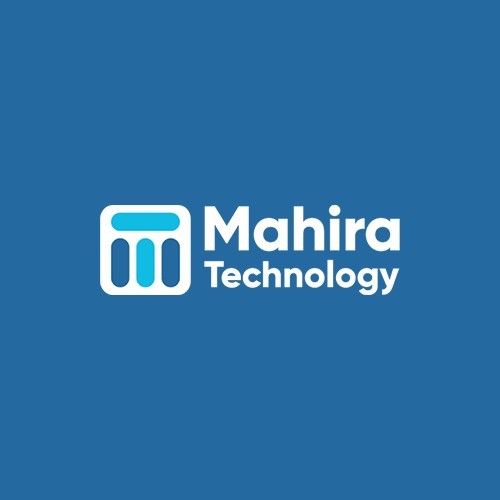
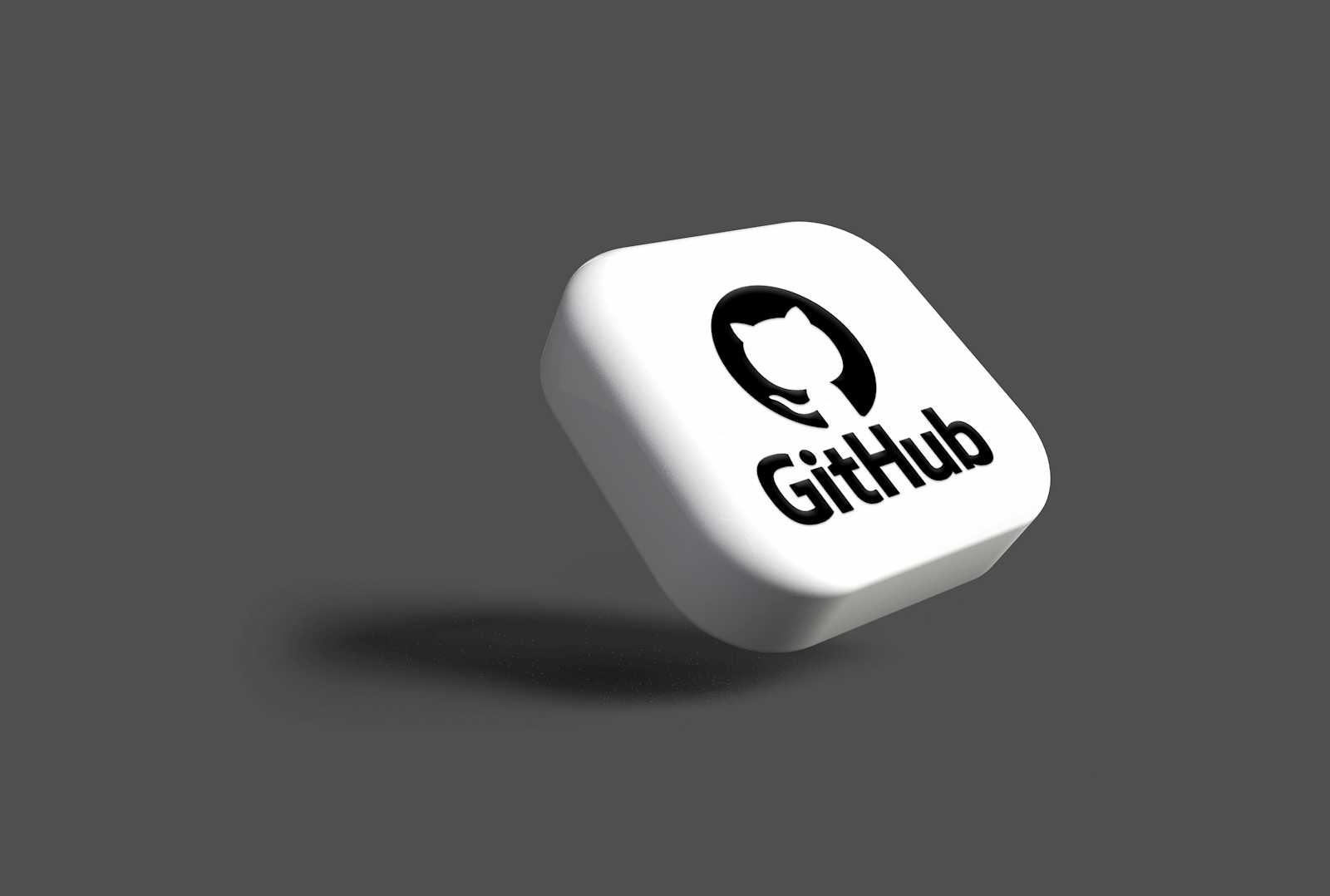
Overview :-
Managing branches in Git can quickly become overwhelming, especially in active projects with multiple contributors. After merging branches, cleaning up the old ones can be tedious and often forgotten. Automating this process not only keeps your repository clean but also saves time and reduces the risk of human error. In this post, we’ll explore how to automate the deletion of merged branches in GitHub using Python, making your Git management process smoother and more efficient.
Pre-requisites :-
Before diving into the automation script, ensure you have the following set up:
GitHub Account: You need a GitHub account with a repository where you have administrative privileges.
Python Environment: A local Python environment (Python 3.x) where scripts can be executed.
Git Installed: Your machine should have Git installed to interact with GitHub repositories.
Access Tokens: A GitHub personal access token with the appropriate permissions to manage branches. You can generate this token in your GitHub account settings under ‘Developer settings’.
Procedure :-
Step 1: Setting Up Your Python Environment
First, ensure your Python environment is ready. If you haven’t already, install Python and pip. Then, install the necessary libraries:
pip install python3
Step 2: Writing the Python Script
Create a new Python file, delete_merged_
branches.py
, and open it in your favorite code editor. Here’s a simple script to get you started:
import argparse
from github import Github
import requests
def find_merged_branches_not_deleted(username, token, repo_owner, repo_name):
# Initialize GitHub client
g = Github(username, token)
try:
# Get the repository
repo = g.get_repo(f"{repo_owner}/{repo_name}")
# Get the default branch
default_branch = repo.default_branch
# Get all branches
branches = [branch.name for branch in repo.get_branches()]
# Get merged pull requests
merged_branches = []
for pr in repo.get_pulls(state='closed'):
if pr.merged and pr.base.ref == default_branch:
merged_branches.append(pr.head.ref)
# Find branches that are merged but not deleted
merged_not_deleted = list(set([branch for branch in merged_branches if branch in branches]))
return merged_not_deleted
except Exception as e:
print(f"Error: {e}")
return []
def delete_branch(username, token, repo_owner, repo_name, branch_name):
url = f"https://api.github.com/repos/{repo_owner}/{repo_name}/git/refs/heads/{branch_name}"
response = requests.delete(url, auth=(username, token))
if response.status_code == 204:
print(f"Deleted branch: {branch_name}")
else:
print(f"Failed to delete branch: {branch_name}. Status code: {response.status_code}. Response: {response.text}")
def main():
# Parse command-line arguments
parser = argparse.ArgumentParser(description="Delete merged branches in a GitHub repository")
parser.add_argument("username", help="GitHub username")
parser.add_argument("token", help="GitHub personal access token")
parser.add_argument("repo_owner", help="Repository owner")
parser.add_argument("repo_name", help="Repository name")
args = parser.parse_args()
username = args.username
token = args.token
repo_owner = args.repo_owner
repo_name = args.repo_name
merged_not_deleted = find_merged_branches_not_deleted(username, token, repo_owner, repo_name)
if merged_not_deleted:
print("Merged branches not deleted:")
for branch in merged_not_deleted:
print(branch)
delete_branch(username, token, repo_owner, repo_name, branch)
else:
print("No merged branches found that are not deleted.")
if __name__ == "__main__":
main()
Step 3: Running the Script
*Install the Required Library:
pip install requests
*Run the Script:
python delete_merged_branches.py YOUR_GITHUB_USERNAME YOUR_GITHUB_TOKEN REPO_OWNER REPO_NAME
- Replace “YOUR_GITHUB_USERNAME” “GITHUB_TOKEN”, “REPO_OWNER” and “REPO_NAME” with your github repo values.
Conclusion :-
Automating the deletion of merged branches in your GitHub repository using Python not only simplifies your Git management but also enhances productivity. By integrating such scripts into your workflow, you can maintain a cleaner and more organized repository without manual intervention. Feel free to modify the script to suit your specific needs or integrate it into larger automation workflows. Happy coding!
Subscribe to my newsletter
Read articles from Mahira Technology Private Limited directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
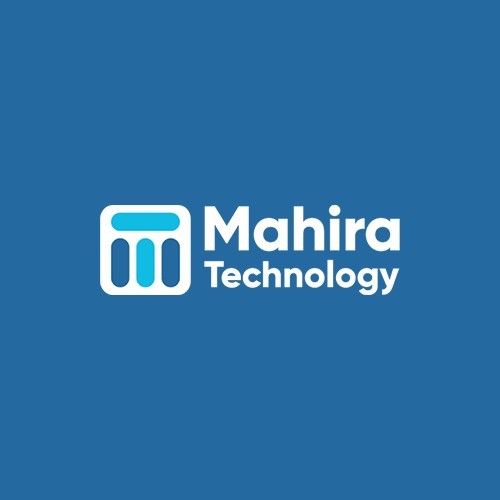
Mahira Technology Private Limited
Mahira Technology Private Limited
A leading tech consulting firm specializing in innovative solutions. Experts in cloud, DevOps, automation, data analytics & more. Trusted technology partner.