Mastering Quick Sort: A Kid-Friendly Guide to This Powerful Sorting Algorithm
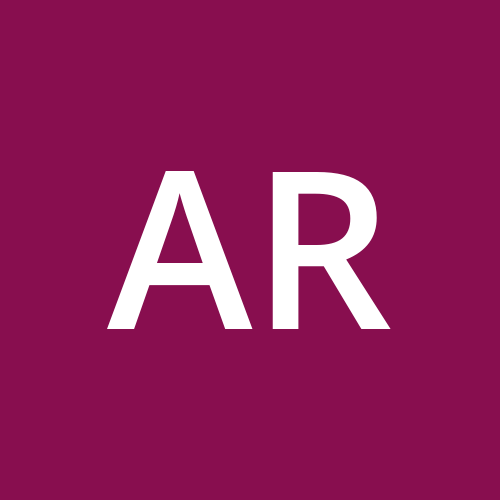
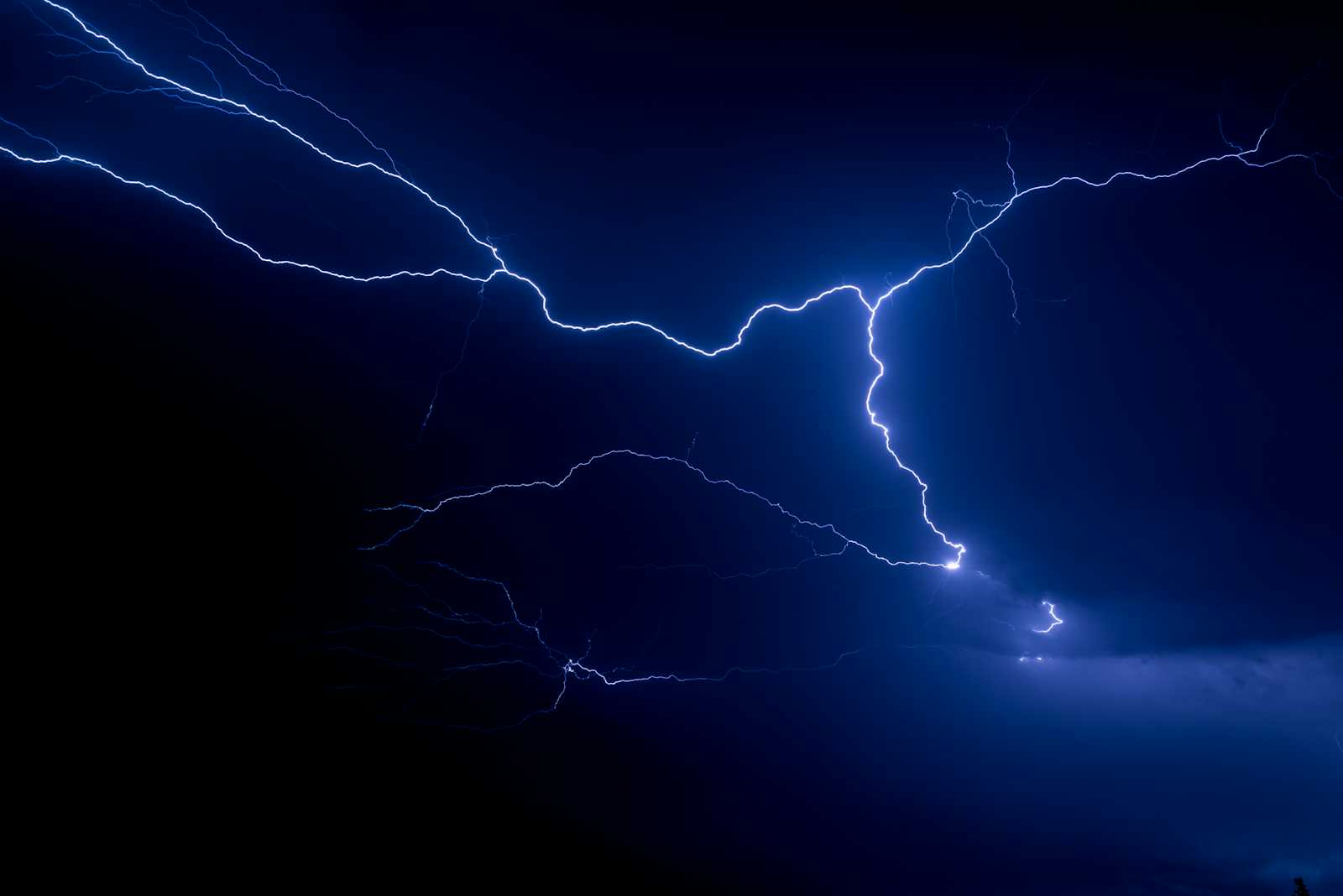
Introduction
Hey there, fellow coders! Today, we're diving into the world of sorting algorithms with a focus on one of the most powerful and efficient ones out there: Quick Sort. Sorting is a common task, whether you're organizing your books, your games, or even your data. Quick Sort uses a smart strategy to get things in order quickly. Let's break it down in simple terms and learn how it works!
What is Quick Sort?
Quick Sort is a super-efficient way to sort a list of items. It uses a clever method called "divide-and-conquer" to get the job done. Here's a simple way to think about it:
Divide: Pick one item from the list as a "pivot".
Conquer: Rearrange the other items into two groups: those less than the pivot and those greater than the pivot.
Combine: Sort each group and then put everything back together.
How It Works
Letβs go through the steps of Quick Sort with an easy example:
Choose a Pivot:
- Select an item from the list. This can be the first, last, middle, or any random item. For example, let's pick the last item.
Partition the Array:
- Move items smaller than the pivot to its left and items larger than the pivot to its right.
Recursively Apply Quick Sort:
- Apply the same steps to the left and right groups (sub-arrays) of items.
Combine:
- Repeat until everything is sorted.
Pseudocode example
Letβs say we have an array: [23, 1, 10, 5, 2]
.
Choose 5 as the pivot:
- Pivot:
5
- Pivot:
Partition into:
Left group:
[1, 2]
(less than 5)Right group:
[10, 23]
(greater than 5)
Recursively apply Quick Sort to each group:
Left group:
[1, 2]
(already sorted)Right group:
[10, 23]
(already sorted)
Combine to get the sorted array:
[1, 2, 5, 10, 23]
Time Complexity
Best Case: π(π log π) - When the pivot divides the array into two equal halves.
Average Case: π(π log π) - When the pivots create balanced partitions.
Worst Case: π(πΒ²) - When the pivot results in unbalanced partitions (like always picking the smallest or largest item as the pivot).
Space Complexity
Best Case: π(log π)
Worst Case: π(π) - for the recursive stack, but generally π(log π) for average-case scenarios.
Implementation in JavaScript
Here's how you can implement Quick Sort in JavaScript:
function quickSort(array) {
if (array.length <= 1) {
return array;
}
let pivot = array[array.length - 1];
let left = [];
let right = [];
for (let i = 0; i < array.length - 1; i++) {
if (array[i] < pivot) {
left.push(array[i]);
} else {
right.push(array[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
Implementation in Python
And here's how you can do it in Python:
function quickSort(array) {
if (array.length <= 1) {
return array;
}
let pivot = array[array.length - 1];
let left = [];
let right = [];
for (let i = 0; i < array.length - 1; i++) {
if (array[i] < pivot) {
left.push(array[i]);
} else {
right.push(array[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
Use Cases
Large Data Sets: very efficient for sorting large arrays and is often faster than other π(π log π) algorithms.
Systems with Limited Memory: can be implemented in-place, meaning it uses only a small amount of additional memory.
Commonly Used in Libraries: many standard libraries use Quick Sort (or its variants) as the default sorting algorithm because of its average-case efficiency.
Real-Time Applications: Quick Sortβs performance makes it suitable for real-time applications where quick sorting is required.
Parallel Processing: can be efficiently parallelized, making it suitable for multi-threaded or distributed computing environments.
More About Algorithms
If you enjoyed learning about Binary Search, you might also find these articles interesting:
Understanding Merge Sort: A Simple Guide for Aspiring Coders
Bubble Sort Demystified: A Beginner's Guide to Sorting Algorithms
These posts will help you further explore different sorting algorithms and improve your coding skills!
Conclusion
Quick Sort is a powerful and efficient sorting algorithm that uses the divide-and-conquer strategy to sort elements quickly. By understanding how it works and implementing it in your code, you can sort data efficiently, even with large datasets.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
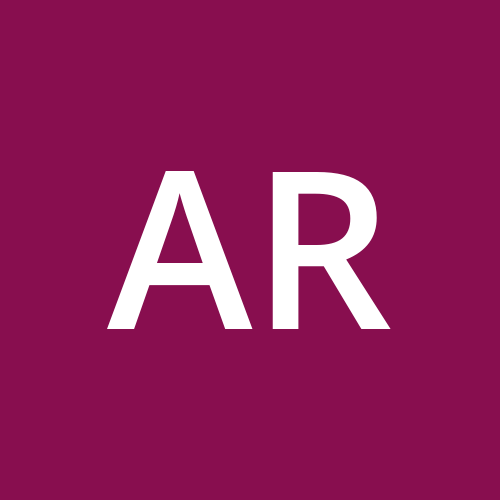