Handle GET and POST Request in Express
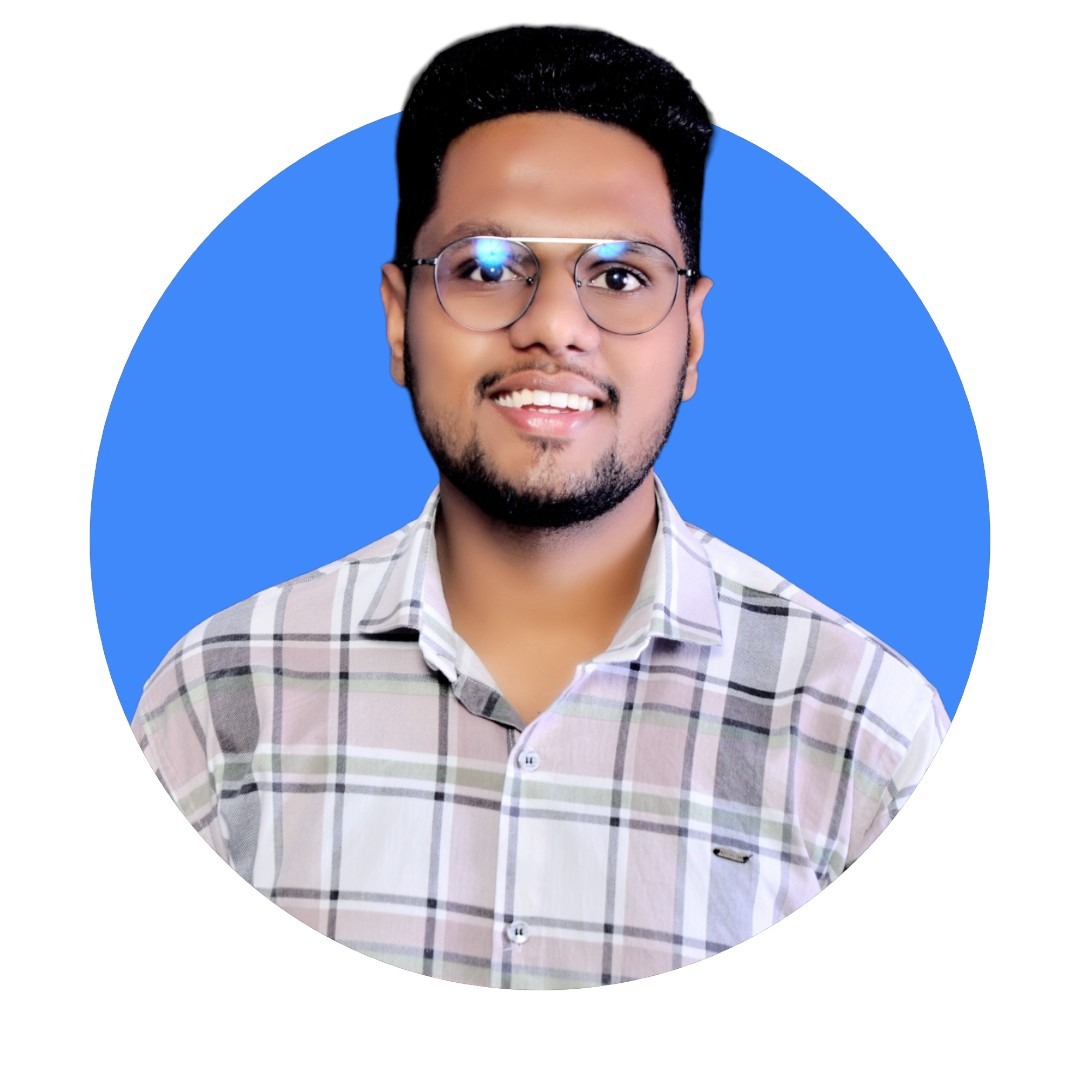
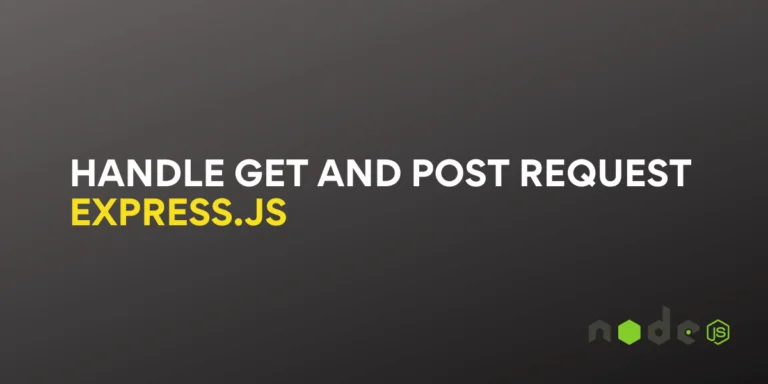
GET and POST are the two common HTTP Requests used for building REST APIs.
Both of these calls are meant for some special purpose.
As per the documentation GET requests are meant to fetch data from specified resources and POST requests are meant to submit data to a specified resource.
This tutorial will teach you the way to handle GET and POST requests in Express.
HTTP GET Methode :
he Hypertext Transfer Protocol(HTTP) Get method is mainly used on the client (Browser) side to send a request to a specified server to get certain data or resources.
Using this method the server should only let us receive the data and not change its state.
Hence it is only used to view something and not to change it.
The Get method is one of the most used HTTP methods.
The request parameter of the get method is appended to the URL.
Get request is better for the data which does not need to be secure (It means the data which does not contain images or Word documents).
Handling GET requests :
Handling GET requests in Express is pretty straightforward.
You have to use the instance of Express to call the method Get.
The Get method is used to handle the get requests in Express.
It takes two arguments, the first one is the route(i.e. path), and the second is the callback.
Inside the callback, we send the response when a user requests that path.
Below is the code snippet where we used the Get method to send a string “Hello World!” to respond to the “/” route.
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Output:
We Send The Request From Hoppscotch Platorm
GET requests can be cached and remains in the browser history.
This is why the GET method is not recommended to use for sensitive data (passwords, ATM pins, etc).
You should GET requests to retrieve data from the server only.
HTTP POST Methode:
The Hypertext Transfer Protocol(HTTP) Post method is mainly used at the client (Browser) side to send data to a Specified server in order to create or rewrite a particular resource/data.
This data sent to the server is stored in the request body of the HTTP request.
Post method eventually leads to the creation of a new resource or updating an existing one.
Due to this dynamic use, it is one of the most used HTTP methods.
Whether Http post is secure or not it is totally depends on its security or its implementation, its security depends upon some factors like encryption(HTTPS), authentication, proper data validation, authorization etc.
Post request is better for the data which needs to be secure (It means the data which contains images or word documents).
Handling POST requests :
The POST request is handled in Express using the Post express method.
This is used when there is a requirement of fetching data from the client such as form data.
The data sent through the POST request is stored in the request object
Express requires an additional middleware module to extract incoming data of a POST request.
This middleware is called the “body-parser”. We need to install it and configure it with Express instance.
You can install body-parser using the following command.
|
You need to import this package into your project and tell Express to use this as middleware.
You need to import this package into your project and tell Express to use this as middleware.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.post('/', (req, res) => {
let data = req.body;
res.send('Data Received: ' + JSON.stringify(data));
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
If you find the above code confusing we have a separate article on Getting Form Data in Node.js, consider reading it.
Output:
Demo App: Login System :
To demonstrate this, let’s code one simple login system that uses a GET request to send a login form to the client and a POST request to fetch the username and password to the server from the client.
Let’s create a new Node.js project.
|
Let’s install the dependencies.
|
Next, let’s create an Express web server.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Now use the Get method to send the HTML which contains a login form.
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html')
})
When the user clicks on the Login button on the HTML page we will POST the request to the Server and get the response.
app.post('/login', (req, res) => {
let username = req.body.username;
let password = req.body.password;
if (username === 'admin' && password === 'admin') {
res.send('Login successful');
}
else {
res.send('Login failed');
}
})
Here is the complete server.js file code.
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: false }))
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html')
})
app.post('/login', (req, res) => {
let username = req.body.username;
let password = req.body.password;
if (username === 'admin' && password === 'admin') {
res.send('Login successful');
}
else {
res.send('Login failed');
}
})
app.listen(3000, () => {
console.log('Example app listening on port 3000!')
})
Here is the code of the index.html page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="/login" method="post">
<input type="text" name="username" placeholder="username">
<input type="password" name="password" placeholder="password">
<input type="submit" value="Login">
</form>
</body>
</html>
Run the Server using the command: node server.js
Output:
Difference between HTTP GET and HTTP POST
HTTP GET | HTTP POST |
In GET method we can not send large amount of data rather limited data of some number of characters is sent because the request parameter is appended into the URL. | In POST method large amount of data can be sent because the request parameter is appended into the body. |
GET request is comparatively better than Post so it is used more than the Post request. | POST request is comparatively less better than Get method, so it is used less than the Get request. |
GET requests are only used to request data (not modify) | POST requests can be used to create and modify data. |
GET request is comparatively less secure because the data is exposed in the URL bar. | POST request is comparatively more secure because the data is not exposed in the URL bar. |
Request made through GET method are stored in Browser history. | Request made through POST method is not stored in Browser history. |
GET method request can be saved as bookmark in browser. | POST method request can not be saved as bookmark in browser. |
Request made through GET method are stored in cache memory of Browser. | Request made through POST method are not stored in cache memory of Browser. |
Data passed through GET method can be easily stolen by attackers as the data is visible to everyone.GET requests should never be used when dealing with sensitive data | Data passed through POST method can not be easily stolen by attackers as the URL Data is not displayed in the URL |
In GET method only ASCII characters are allowed. | In POST method all types of data is allowed. |
In GET method, the Encoding type is application/x-www-form-urlencoded | In POSTmethod, the encoding type is application/x-www-form-urlencoded or multipart/form-data. Use multipart encoding for binary data |
Summary
Express is the most popular framework for creating web applications in Node.js, it requires less time and fewer lines of code. It can able to handle GET and POST requests without installing any third-party module. The GET request can be handled using the Get method from the Express instance, and the POST request is used using the Post method. Hope this tutorial helps you understand how to handle GET and POST requests in Express.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
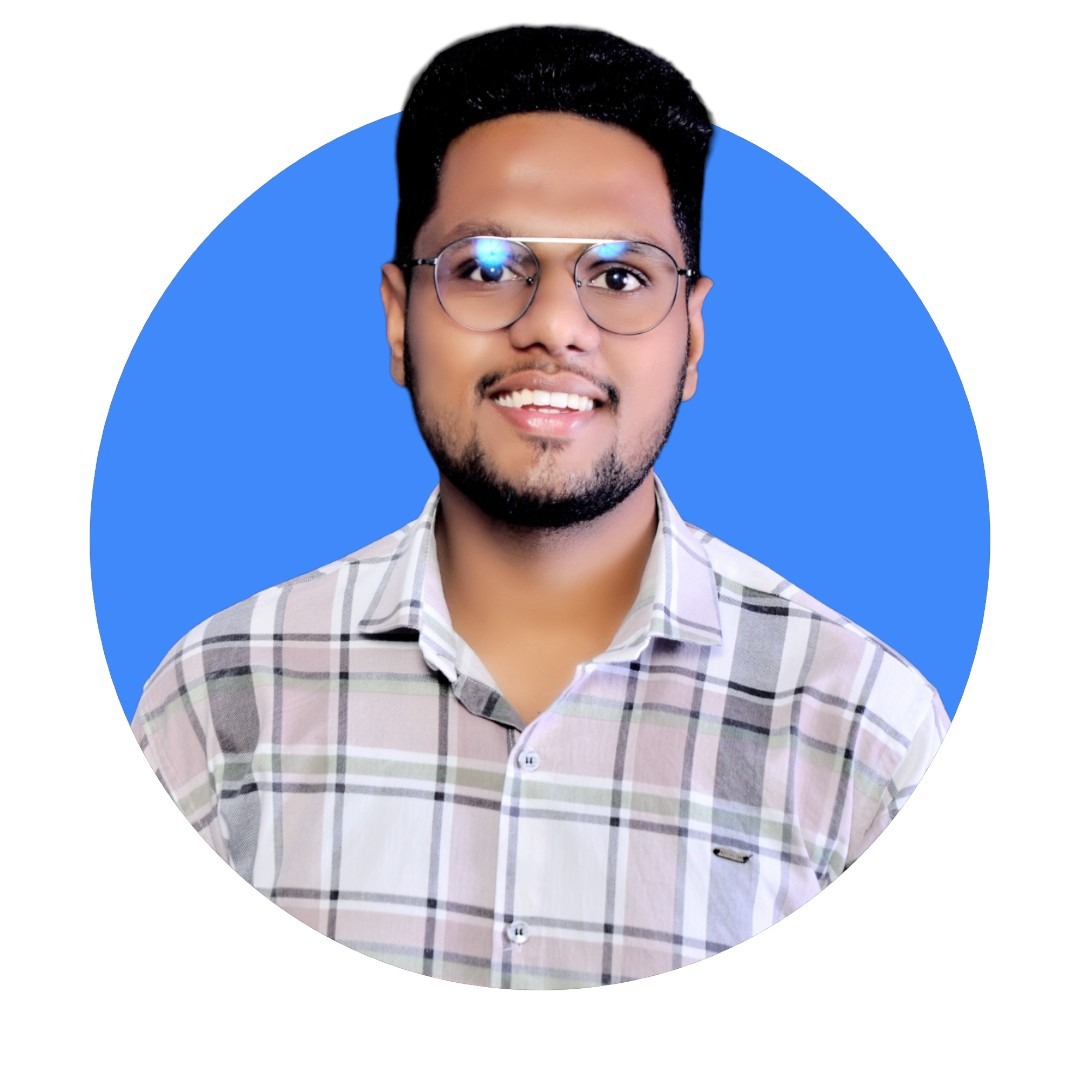
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.