AWS Amplify
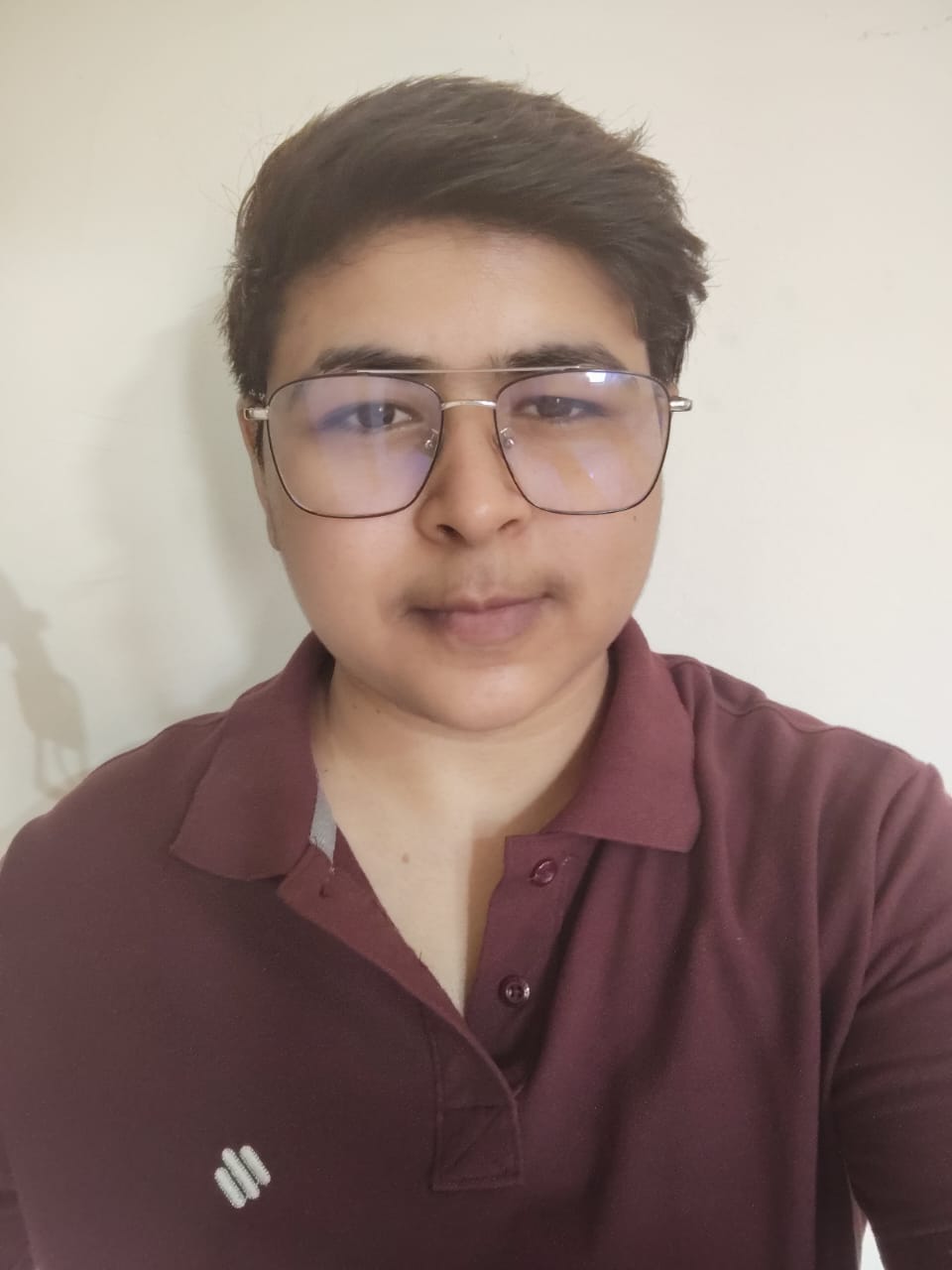
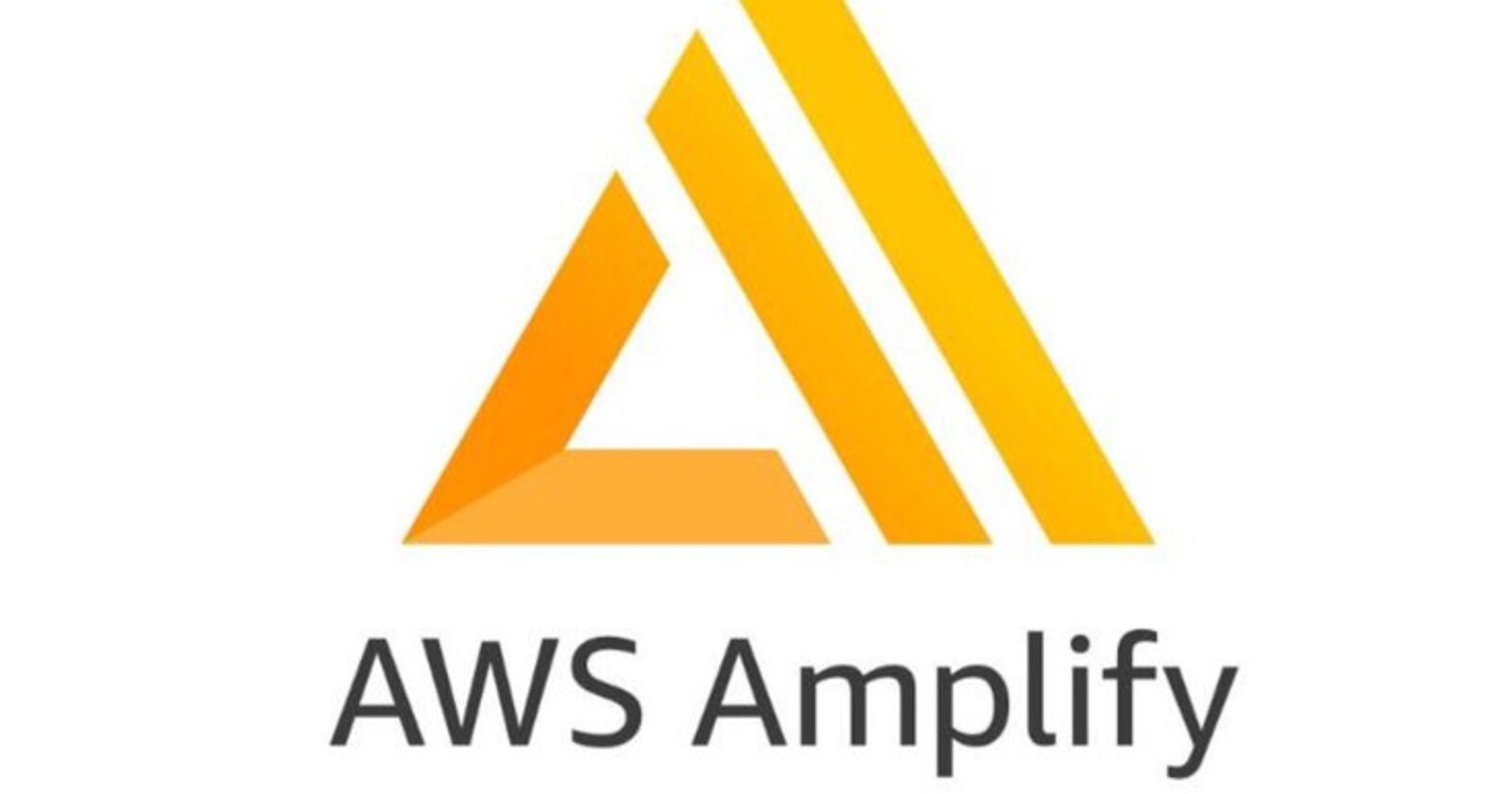
Introduction
In today's fast-paced digital world developers need tools that allow them to build and deploy full-stack applications rapidly and efficiently. AWS Amplify is a comprehensive suite of tools and services designed to make this possible. It provides a powerful framework for developing scalable and secure cloud-powered applications seamlessly integrating front-end frameworks with backend services.
This blog will provide an in-depth guide on AWS Amplify including setting up a front-end framework, integrating backend services and deploying your application. By the end of this tutorial you will be equipped to leverage AWS Amplify to build full-stack applications.
Overview of AWS Amplify
AWS Amplify is a set of services and tools designed to help developers build and deploy full-stack applications faster. It simplifies the integration of various AWS services with your front-end and backend providing features such as :-
Authentication : Easily add user sign-up, sign-in and access control to your app.
Data Storage : Integrate scalable data storage with Amazon DynamoDB or Amazon S3.
APIs : Build GraphQL and REST APIs with AWS AppSync and Amazon API Gateway.
Hosting : Deploy and host static web applications with continuous integration and delivery.
Analytics : Integrate analytics to track user behavior and application performance.
Setting Up AWS Amplify
Before you start ensure you have the following :-
An AWS account.
Node.js and npm installed.
A code editor (e.g. Visual Studio Code).
Basic knowledge of JavaScript and front-end frameworks (e.g. React, Angular, or Vue.js).
Step 1 :- Install the Amplify CLI
The Amplify CLI is a command-line tool that helps you set up and manage AWS resources for your application.
npm install -g @aws-amplify/cli
Step 2 :- Configure the Amplify CLI
Configure the Amplify CLI with your AWS credentials.
amplify configure
Follow the prompts to create a new IAM user and configure the CLI with the user credentials.
Step 3 :- Initialize a New Amplify Project
Create a new directory for your project and initialize Amplify.
mkdir my-amplify-app
cd my-amplify-app
amplify init
Follow the prompts to configure your project. For example :-
Enter a name for the project : myamplifyapp
Enter a name for the environment : dev
Choose your default editor : Visual Studio Code
Choose the type of app that you’re building : JavaScript
What JavaScript framework are you using : React
Source Directory Path : src
Distribution Directory Path : build
Build Command : npm run build
Start Command : npm start
Setting Up a Front-End Framework
Step 1 :- Create a React App
If you chose React as your front-end framework create a new React app.
npx create-react-app my-amplify-app
cd my-amplify-app
Step 2 :- Add Amplify to Your React App
Install the AWS Amplify and AWS Amplify React libraries.
npm install aws-amplify @aws-amplify/ui-react
Step 3 :- Configure Amplify in Your React App
Add the Amplify configuration to your React app. Create a new file named aws-exports.js in the src directory and add the following code :-
import Amplify from 'aws-amplify';
import awsconfig from './aws-exports';
Amplify.configure(awsconfig);
Include the Amplify configuration in your index.js file :-
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
import Amplify from 'aws-amplify';
import awsconfig from './aws-exports';
Amplify.configure(awsconfig);
ReactDOM.render(<App />, document.getElementById('root'));
serviceWorker.unregister();
Integrating Backend Services
Adding Authentication
Amplify makes it easy to add authentication to your application.
amplify add auth
Follow the prompts to configure authentication. For example :-
Do you want to use the default authentication and security configuration? Default configuration
How do you want users to be able to sign in? Username
Do you want to configure advanced settings? No
Push the changes to the cloud :-
amplify push
Using Authentication in Your App
Use the Amplify Auth component in your app to provide authentication.
import React from 'react';
import { withAuthenticator, AmplifySignOut } from '@aws-amplify/ui-react';
function App() {
return (
<div className="App">
<header className="App-header">
<h1>Welcome to My Amplify App</h1>
<AmplifySignOut />
</header>
</div>
);
}
export default withAuthenticator(App);
Adding a GraphQL API
Add a GraphQL API to your app using AWS AppSync.
amplify add api
Follow the prompts to configure the API. For example :-
Please select from one of the below mentioned services : GraphQL
Provide API name : myapi
Choose the default authorization type for the API : API key
Do you have an annotated GraphQL schema? No
Choose a schema template : Single object with fields (e.g. “Todo” with ID, name, description)
Push the changes to the cloud :-
amplify push
Using the GraphQL API in Your App
Use the Amplify API component to interact with the GraphQL API.
import React, { useState, useEffect } from 'react';
import { API, graphqlOperation } from 'aws-amplify';
import { listTodos } from './graphql/queries';
import { createTodo } from './graphql/mutations';
function App() {
const [todos, setTodos] = useState([]);
const [newTodo, setNewTodo] = useState('');
useEffect(() => {
fetchTodos();
}, []);
const fetchTodos = async () => {
const result = await API.graphql(graphqlOperation(listTodos));
setTodos(result.data.listTodos.items);
};
const addTodo = async () => {
if (newTodo.trim() !== '') {
const todo = { name: newTodo };
await API.graphql(graphqlOperation(createTodo, { input: todo }));
setNewTodo('');
fetchTodos();
}
};
return (
<div className="App">
<header className="App-header">
<h1>Todo List</h1>
<input
type="text"
value={newTodo}
onChange={(e) => setNewTodo(e.target.value)}
/>
<button onClick={addTodo}>Add Todo</button>
<ul>
{todos.map((todo) => (
<li key={todo.id}>{todo.name}</li>
))}
</ul>
<AmplifySignOut />
</header>
</div>
);
}
export default withAuthenticator(App);
Adding Storage with Amazon S3
Add file storage capabilities to your app using Amazon S3.
amplify add storage
Follow the prompts to configure the storage. For example :-
Please select from one of the below mentioned services : Content (Images, audio, video, etc.)
Provide a friendly name for your resource : myS3Bucket
Provide bucket name : my-s3-bucket
Who should have access? Auth users only
What kind of access do you want for Authenticated users? create/update, read, delete
Do you want to add a Lambda Trigger for your S3 Bucket? No
Push the changes to the cloud :-
amplify push
Using Storage in Your App
Use the Amplify Storage component to upload and retrieve files.
import React, { useState } from 'react';
import { Storage } from 'aws-amplify';
function App() {
const [file, setFile] = useState(null);
const [fileUrl, setFileUrl] = useState('');
const uploadFile = async () => {
if (file) {
await Storage.put(file.name, file);
const url = await Storage.get(file.name);
setFileUrl(url);
}
};
return (
<div className="App">
<header className="App-header">
<h1>Upload a File</h1>
<input type="file" onChange={(e) => setFile(e.target.files[0])} />
<button onClick={uploadFile}>Upload</button>
{fileUrl && <a href={fileUrl}>Download File</a>}
<AmplifySignOut />
</header>
</div>
);
}
export default withAuthenticator(App);
Deploying Your Application
Hosting with AWS Amplify
AWS Amplify provides a fully managed hosting service for your web applications with support for continuous integration and delivery.
amplify add hosting
Follow the prompts to configure hosting. For example :-
Select the plugin module to execute : Hosting with Amplify Console
Choose a type : Continuous deployment (Git-based deployments)
Push the changes to the cloud :-
amplify push
Connect Your Repository
Follow the prompts to connect your GitHub repository and set up the build settings. Once completed Amplify will automatically deploy your application whenever you push changes to the specified branch.
Conclusion
AWS Amplify simplifies the process of building and deploying full-stack applications by providing a powerful framework and a suite of integrated tools and services. By following this guide you have learned how to set up AWS Amplify, integrate front-end and backend services and deploy your application. With Amplify you can focus on building features and functionality while AWS handles the heavy lifting of infrastructure management.
Stay tuned for more insights in our upcoming blog posts.
Let's connect and grow on LinkedIn :Click Here
Let's connect and grow on Twitter :Click Here
Happy Cloud Computing!!!
Happy Reading!!!
Sudha Yadav
Subscribe to my newsletter
Read articles from Sudha Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
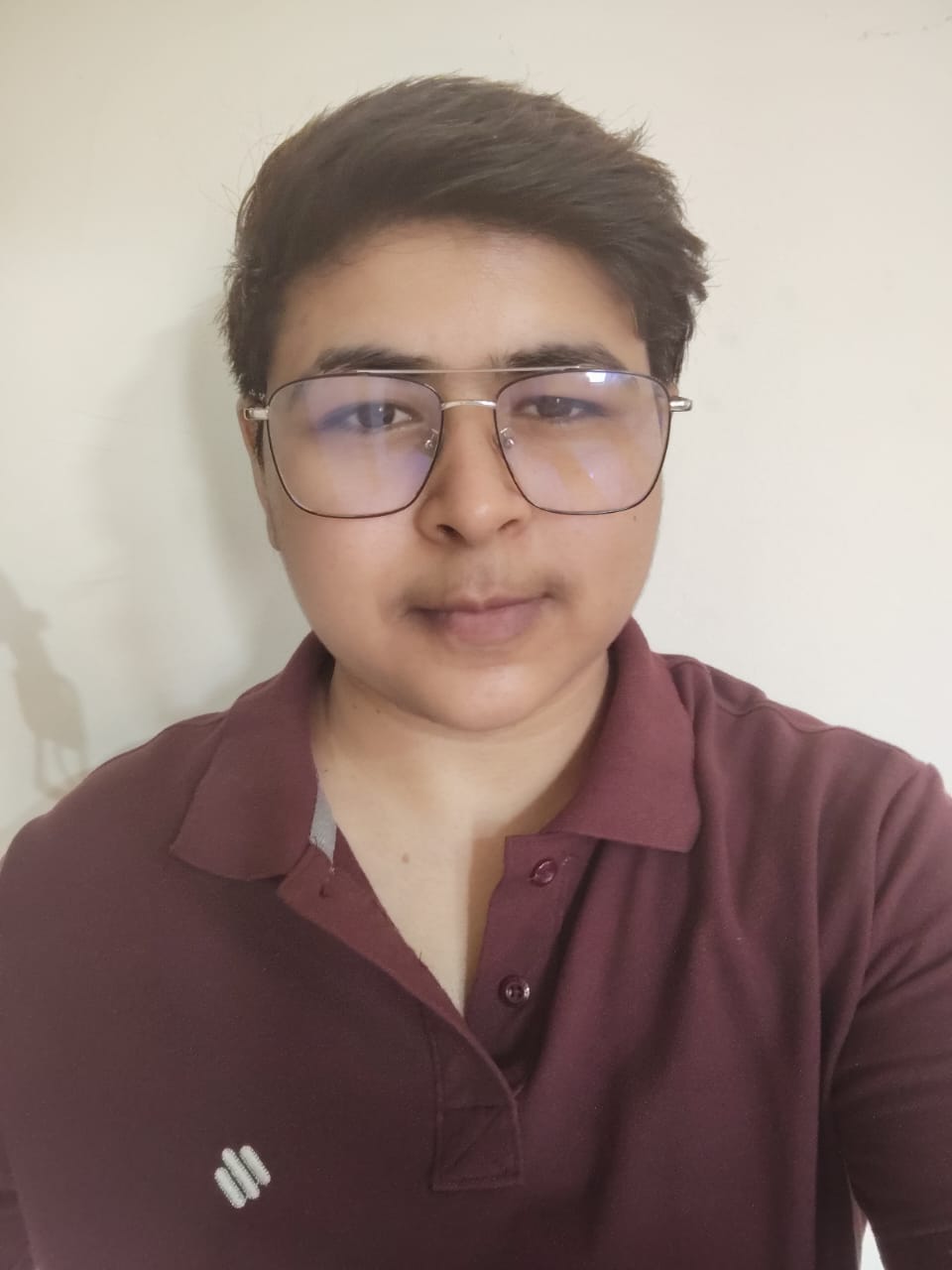
Sudha Yadav
Sudha Yadav
🚀 Hi everyone! I'm Sudha Yadav, a DevOps engineer with a big passion for all things DevOps. I've knowledge and worked on some cool projects and I can't wait to share what I'm learning with you all! 🛠️ Here's what's in my toolbox: Linux Github Docker Kubernetes Jenkins AWS Python Prometheus Grafana Ansible Terraform Join me as we explore AWS DevOps together. Let's learn and grow together in this ever-changing field! 🤝 Feel free to connect with me for: Sharing experiences Friendly chats Learning together Follow my journey on Twitter and LinkedIn for daily updates. Let's dive into the world of DevOps together! 🚀 #DevOps #AWS #DevOpsJourney #90DaysOfDevOps