Beginner's Guide to Docker: Master Containerization Easily
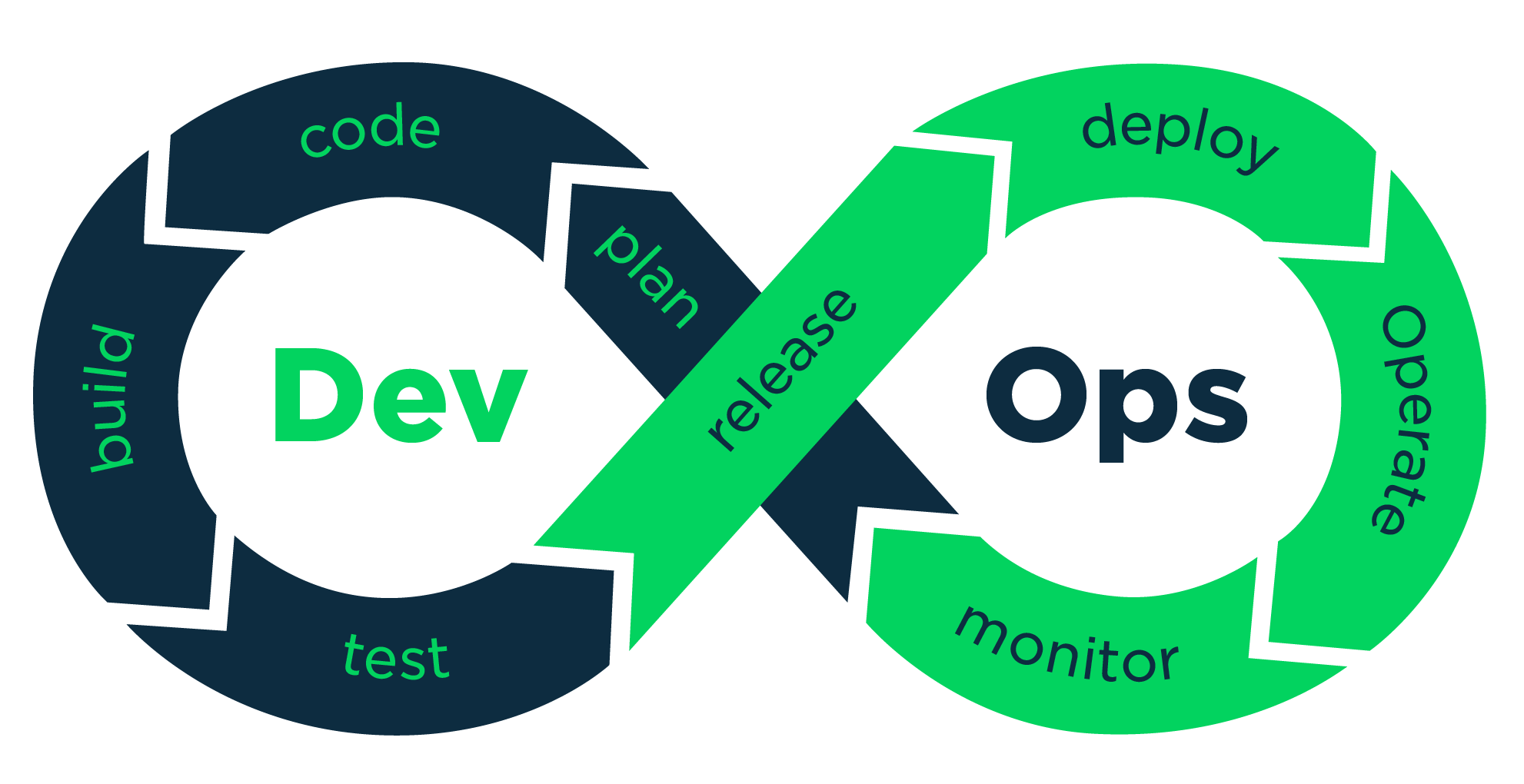
Table of contents
- What's Docker All About?
- Docker Architecture: The Building Blocks
- Installing Docker: Your Gateway to Container Land
- Docker Images: The Blueprint of Your Application
- Dockerfile: Your Recipe for Success
- Docker Containers: Where the Magic Happens
- Docker Networking: Connecting Your Containers
- Docker Volumes: Persistent Data Storage
- Docker Best Practices: Tips for Smooth Sailing
- Debugging Docker: When Things Go Wrong
- Real-World Example: Dockerizing a Python Web Application
- Wrapping Up
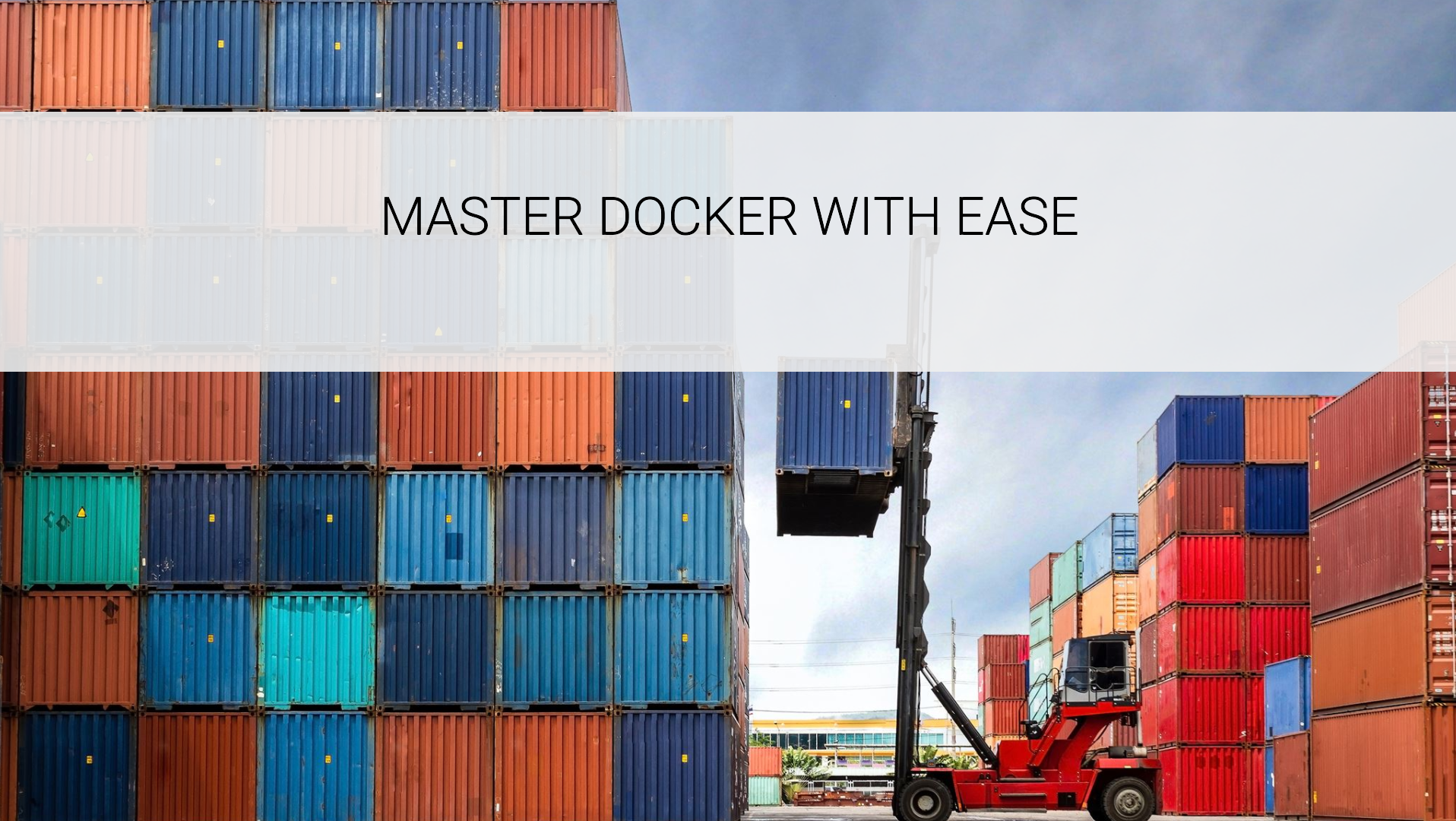
Hey there, tech enthusiasts! Ready to start a journey into the world of Docker? Buckle up, because we're about to dive deep into the world of containerization, exploring every aspect of this game-changing technology.
What's Docker All About?
At its core, Docker is a platform for developing, shipping, and running applications in containers. But what does that really mean?
Imagine you're a chef (stay with me here, even if your culinary skills are limited to microwaving leftovers). You've created the perfect recipe, but when you try to cook it in different kitchens, something always goes wrong. The stove is different, the ingredients aren't quite the same, or the pans are a different size.
That's the problem Docker solves but for software. It creates a standard "kitchen" (container) where your "recipe" (application) always works perfectly, no matter where you're "cooking" (running) it.
Docker Architecture: The Building Blocks
Before we dive into the how-to, let's understand the key components of Docker:
Docker Daemon: This is the background service running on the host that manages building, running, and distributing Docker containers.
Docker Client: This is the command line tool that allows the user to interact with the Docker daemon.
Docker Images: These are read-only templates used to create containers. Think of them as a snapshot of a container.
Docker Containers: These are runnable instances of Docker images. You can create, start, stop, move, or delete a container using the Docker API or CLI.
Docker Registry: This is where Docker images are stored. Docker Hub is a public registry that anyone can use.
Now that we've got the basics down, let's roll up our sleeves and get our hands dirty with some Docker magic!
Installing Docker: Your Gateway to Container Land
First things first, let's get Docker installed on your machine. The process varies depending on your operating system, but here's a detailed guide:
For Windows and Mac:
Download Docker Desktop from the official Docker website.
Run the installer and follow the prompts.
Once installed, start Docker Desktop.
For Linux (Ubuntu):
Update your package index:
sudo apt-get update
Install packages to allow apt to use a repository over HTTPS:
sudo apt-get install \ apt-transport-https \ ca-certificates \ curl \ gnupg \ lsb-release
Add Docker's official GPG key:
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
Set up the stable repository:
echo \ "deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu \ $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
Update the package index again:
sudo apt-get update
Install Docker Engine:
sudo apt-get install docker-ce docker-ce-cli containerd.io
After installation, verify that Docker is running correctly by opening a terminal and typing:
docker --version
docker run hello-world
If you see the Docker version and a welcome message, congratulations! You've successfully installed Docker.
Docker Images: The Blueprint of Your Application
Docker images are the foundation of containers. They're read-only templates that contain a set of instructions for creating a container that can run on the Docker platform.
Pulling Images
You can pull existing images from Docker Hub using the docker pull
command:
docker pull ubuntu:latest
This command pulls the latest Ubuntu image from Docker Hub.
Listing Images
To see the images you have locally:
docker images
Removing Images
To remove an image:
docker rmi image_name
Replace image_name
with the name or ID of the image you want to remove.
Dockerfile: Your Recipe for Success
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. It's like a recipe for your Docker image. Let's break down a more detailed Dockerfile:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Let's break down each command:
FROM
: Specifies the base image to use. Here, we're using Python 3.9 with a slim version of Debian.WORKDIR
: Sets the working directory for any subsequent ADD, COPY, CMD, ENTRYPOINT, or RUN instructions.COPY
: Copies files from your Docker client's current directory.RUN
: Executes commands in a new layer on top of the current image and commits the results.EXPOSE
: Informs Docker that the container listens on the specified network port at runtime.ENV
: Sets an environment variable.CMD
: Provides defaults for an executing container. There can only be one CMD instruction in a Dockerfile.
Building an Image from a Dockerfile
To build an image from a Dockerfile:
docker build -t my-python-app .
This command builds an image from the Dockerfile in the current directory (.
) and tags it (-t
) as "my-python-app".
Docker Containers: Where the Magic Happens
Now that we have our image, let's dive into containers - the runnable instances of Docker images.
Running a Container
To run a container from an image:
docker run -d -p 80:5000 my-python-app
Let's break this down:
-d
: Run the container in detached mode (in the background)-p 80:5000
: Map port 80 of the host to port 5000 in the containermy-python-app
: The name of the image to run
Listing Running Containers
To see what containers are currently running:
docker ps
To see all containers (including stopped ones):
docker ps -a
Stopping and Removing Containers
To stop a running container:
docker stop container_id
To remove a container:
docker rm container_id
Docker Networking: Connecting Your Containers
Docker networking allows containers to communicate with each other and with the outside world.
Default Bridge Network
By default, Docker creates a bridge network for each container. You can see your networks with:
docker network ls
Creating a Custom Network
To create your own network:
docker network create my-net
Connecting Containers to a Network
When you run a container, you can specify which network it should connect to:
docker run --network=my-net my-python-app
Docker Volumes: Persistent Data Storage
Volumes are the preferred mechanism for persisting data generated by and used by Docker containers.
Creating a Volume
To create a volume:
docker volume create my-vol
Using a Volume
To use a volume when running a container:
docker run -v my-vol:/app/data my-python-app
This mounts the volume my-vol
to the /app/data
directory in the container.
Docker Best Practices: Tips for Smooth Sailing
Use Official Images: Start with official images from Docker Hub when possible.
Minimize Layers: Each instruction in a Dockerfile creates a new layer. Try to combine commands to reduce the number of layers.
Use .dockerignore: Similar to .gitignore, this file lets you specify which files shouldn't be copied into the container.
Don't Run as Root: For security reasons, it's best to run your application as a non-root user inside the container.
Use Multi-Stage Builds: This can significantly reduce the size of your final image by leaving build dependencies behind.
Keep Images Small: Use alpine versions of images when possible, and remove unnecessary files.
Tag Your Images: Use meaningful tags for your images, not just
latest
.Use Environment Variables: This makes your containers more flexible and easier to configure.
Debugging Docker: When Things Go Wrong
Even with the best practices, sometimes things don't go as planned. Here are some tools to help you debug:
Viewing Container Logs
To see the logs from a container:
docker logs container_id
Executing Commands in a Running Container
To run a command in a running container:
docker exec -it container_id /bin/bash
This gives you a bash shell inside the container.
Inspecting a Container
To get detailed information about a container:
docker inspect container_id
Real-World Example: Dockerizing a Python Web Application
Let's put all this knowledge into practice by Dockerizing a simple Python web application using Flask.
- First, create a new directory for your project and navigate into it:
mkdir flask-docker-app && cd flask-docker-app
- Create a file named
app.py
with the following content:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, Docker!'
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0')
- Create a
requirements.txt
file:
Flask==2.0.1
- Create a Dockerfile:
FROM python:3.9-slim
WORKDIR /app
COPY . /app
RUN pip install --no-cache-dir -r requirements.txt
EXPOSE 5000
CMD ["python", "app.py"]
- Build the Docker image:
docker build -t flask-docker-app .
- Run the container:
docker run -d -p 5000:5000 flask-docker-app
Now, if you navigate to http://localhost:5000
in your web browser, you should see "Hello, Docker!".
Wrapping Up
Whew! We've covered a lot of ground, from the basics of Docker to some more advanced concepts and best practices. Docker is a powerful tool that can dramatically simplify your development and deployment processes, but like any powerful tool, it takes time and practice to master.
Remember, the best way to learn Docker is by doing. Start small, perhaps by containerizing a simple application like our Flask example, and gradually work your way up to more complex setups. Experiment, break things, and learn from your mistakes. Before you know it, you'll be orchestrating containers like a pro!
For more in-depth information and advanced topics, check out the official Docker documentation.
Happy Dockerizing, folks! May your containers be light, your images clean, and your deployments smooth as silk.
Keywords: Docker tutorial, container technology, DevOps tools, application deployment, Docker for beginners
Long-tail Keywords: How to use Docker for web development, Docker container management best practices, Step-by-step Docker installation guide
Subscribe to my newsletter
Read articles from vikash kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
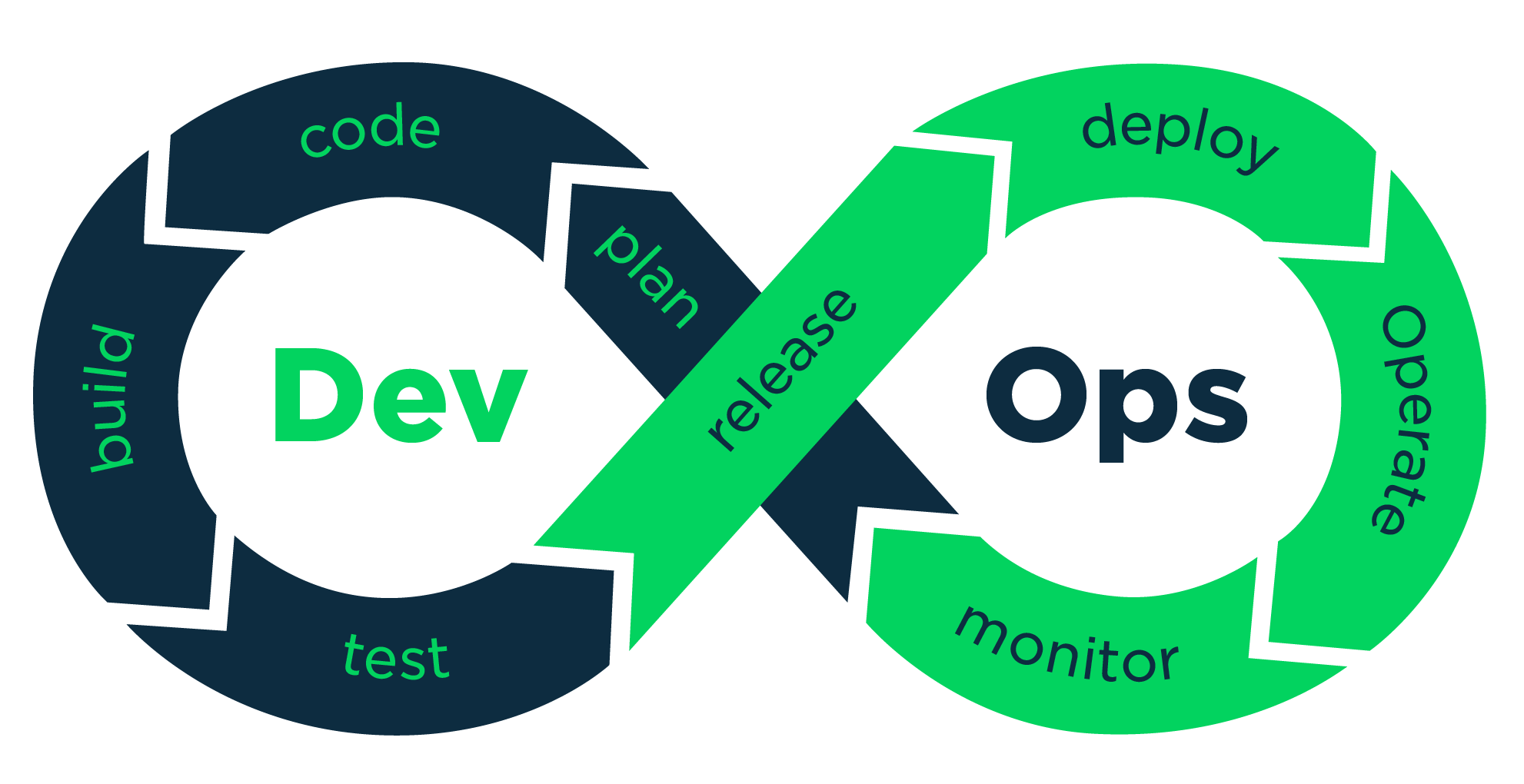
vikash kumar
vikash kumar
Hey folks! ๐ I'm Vikash Kumar, a seasoned DevOps Engineer navigating the thrilling landscapes of DevOps and Cloud โ๏ธ. My passion? Simplifying and automating processes to enhance our tech experiences. By day, I'm a Terraform wizard; by night, a Kubernetes aficionado crafting ingenious solutions with the latest DevOps methodologies ๐. From troubleshooting deployment snags to orchestrating seamless CI/CD pipelines, I've got your back. Fluent in scripts and infrastructure as code. With AWS โ๏ธ expertise, I'm your go-to guide in the cloud. And when it comes to monitoring and observability ๐, Prometheus and Grafana are my trusty allies. In the realm of source code management, I'm at ease with GitLab, Bitbucket, and Git. Eager to stay ahead of the curve ๐, I'm committed to exploring the ever-evolving domains of DevOps and Cloud. Let's connect and embark on this journey together! Drop me a line at thenameisvikash@gmail.com.