Operator Precedence
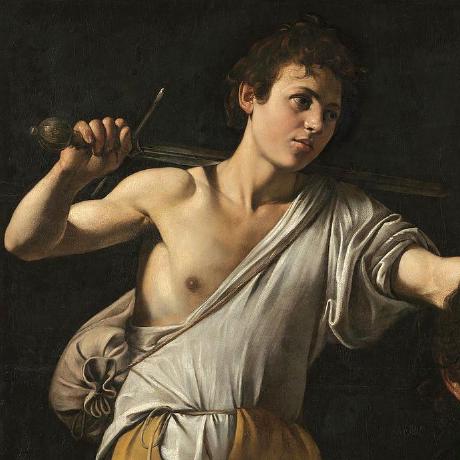
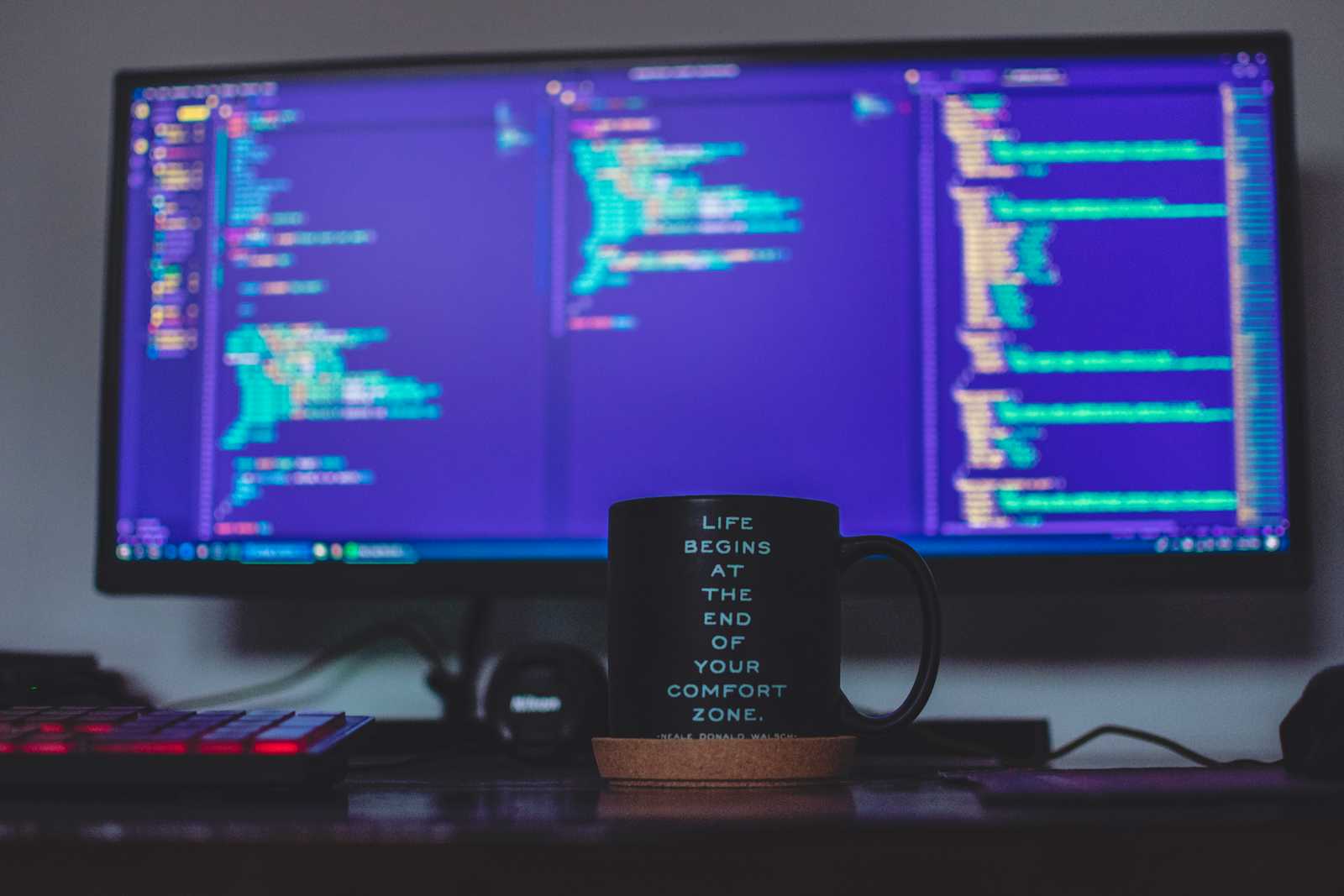
When you're coding in JavaScript, you often use various operators to perform operations on data. These can be arithmetic operators like +
, -
, *
, /
, or logical operators like &&
and ||
. But did you know that the order in which these operations are performed is governed by a set of rules known as operator precedence?
In this blog post, we'll explore what operator precedence is, why it's important, and how it affects your code. Let's dive in!
What is Operator Precedence?
Operator precedence determines the order in which operators are evaluated in an expression. In other words, it decides which part of your code gets executed first when multiple operators are involved. For more information about how every operator ranked according to their precedence, I'd suggest you check out the MDN's operator precedence guide: Link
For example, in the expression 3 + 4 * 2
, the multiplication happens before the addition because multiplication has higher precedence than addition. So, the expression is evaluated as 3 + (4 * 2)
, resulting in 11
.
Why is Operator Precedence Important?
Understanding operator precedence is crucial because it helps you predict the outcome of complex expressions. If you're not aware of the precedence rules, your code might produce unexpected results, leading to bugs that are hard to track down.
Operator Precedence Table
Here's a simplified table of some common JavaScript operators and their precedence levels (from highest to lowest) from MDN's web docs:
Precedence Level | Operator(s) | Description |
20 | () | Grouping |
19 | ++ , -- | Increment and Decrement |
18 | ! , typeof | Logical NOT, Typeof |
17 | * , / , % | Multiplication, Division, Modulus |
16 | + , - | Addition, Subtraction |
15 | < , <= , > , >= | Relational Operators |
14 | == , != , === , !== | Equality Operators |
13 | && | Logical AND |
12 | ` | |
11 | ?: | Ternary |
10 | = | Assignment |
Examples:
Let's look at some examples to see how operator precedence works in practice.
- Arithmetic Operations:
let result = 3 + 4 * 2;
console.log(result); // Output: 11
In this example, the multiplication is performed first, then the addition.
- Using Parentheses:
let result = (3 + 4) * 2;
console.log(result); // Output: 14
By using parentheses, you change the order of operations. Now, the addition happens before the multiplication.
- Combining Logical and Comparison Operators:
let a = true;
let b = false;
let result = a || (b && !a);
console.log(result); // Output: true
Here, the &&
(Logical AND) operator has higher precedence than ||
(Logical OR). So the expression is evaluated as a || (b && !a)
.
Tips:
Use Parentheses: When in doubt, use parentheses to make your code's intentions clear. This not only helps avoid unexpected results but also makes your code easier to read.
Keep It Simple: Try to write expressions that are easy to understand. Complex expressions can be broken down into smaller, more manageable parts.
Practice: The best way to get comfortable with operator precedence is to practice writing and evaluating different expressions.
Using MDN Web Docs: Bookmark the MDN's Operator precedence page and revisit it every time you want to be ensure about the ranking of the operator. You don't have to remember every rank, but keeping the important information regarding the most used operator would make you more confident about what you write!
Conclusion
Operator precedence in JavaScript can seem daunting at first, but with a little practice, you'll get the hang of it. By understanding these rules, you can write more predictable and bug-free code.
Subscribe to my newsletter
Read articles from Arpit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
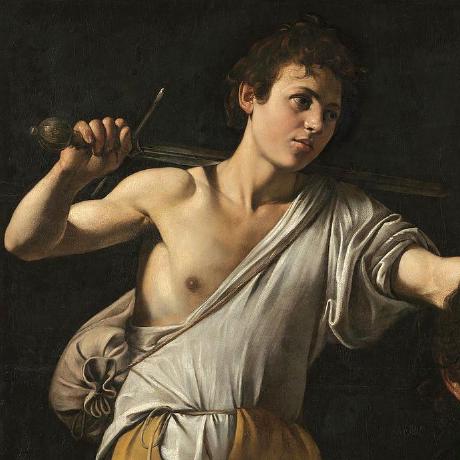
Arpit
Arpit
Greetings! I'm Magnifiques 📿, a web developer and UI/UX designer passionate about creating amazing digital experiences. I specialize in ReactJS and JavaScript, and my projects highlight my expertise with the ReactJS library. I also use Node.js and Express.js for backend development and Figma for designing user-friendly interfaces. I'm committed to delivering top-quality solutions that exceed user expectations.