A Comprehensive Guide to Material-UI for React Developers
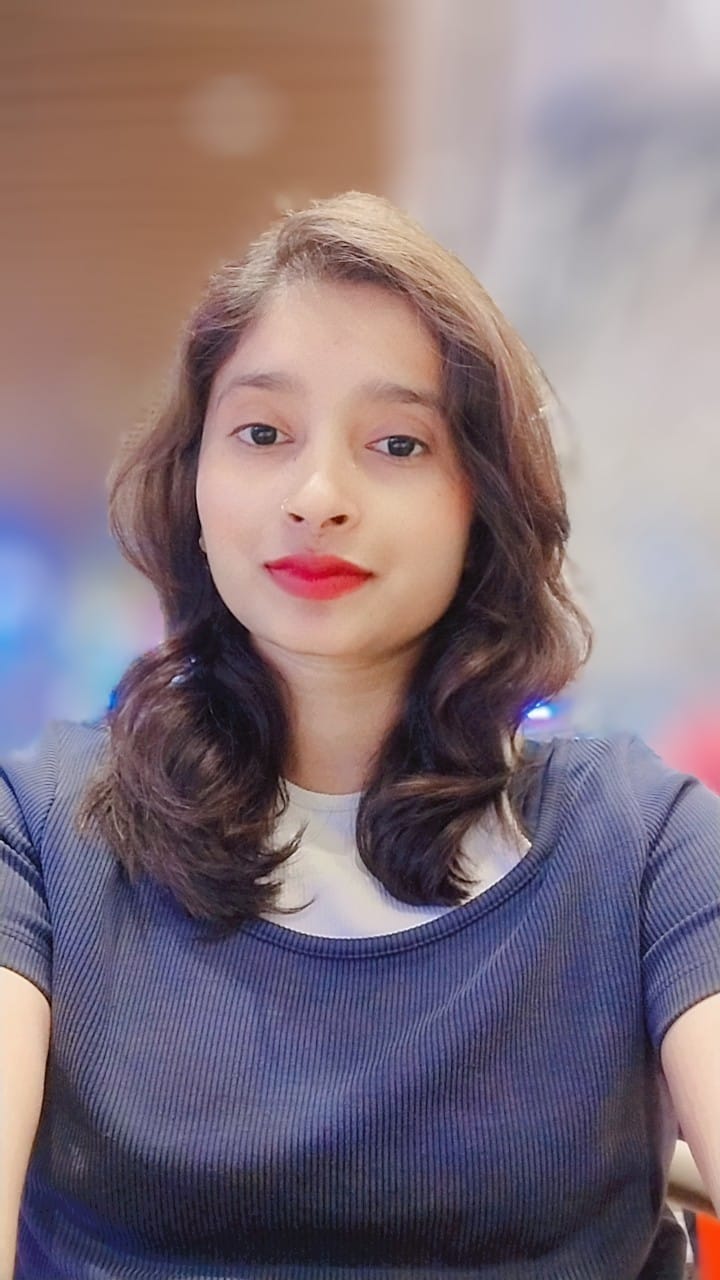
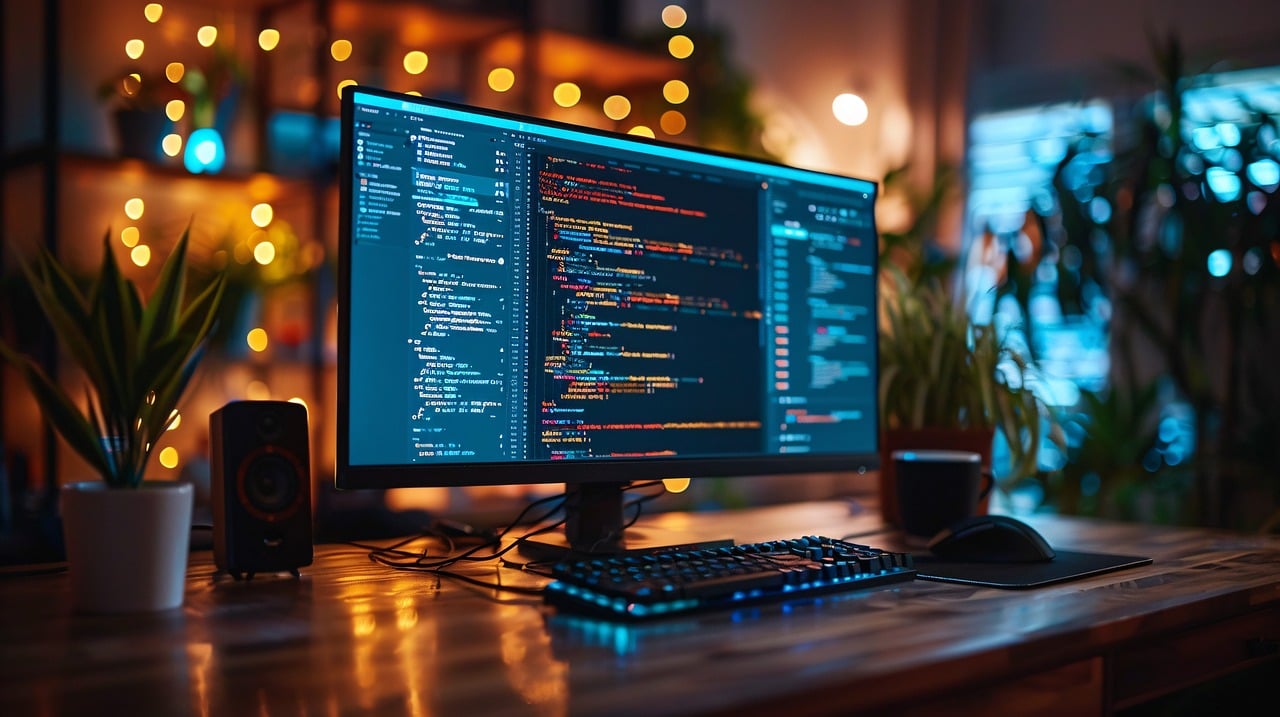
Introduction
As a React developer, building a visually appealing and user-friendly interface can sometimes be challenging. Thankfully, Material-UI provides a comprehensive suite of tools to make your life easier. Based on Google's Material Design guidelines, Material-UI offers a set of React components that are easy to integrate and customize. In this guide, we'll explore the basics of Material-UI, its core components, and how to effectively use it in your projects.
Getting Started with Material-UI
Before diving into the features and components of Material-UI, you need to set it up in your React project. Follow these steps to get started:
- Install Material-UI:
To add Material-UI to your project, you can use npm or yarn:
npm install @mui/material @emotion/react @emotion/styled
or
yarn add @mui/material @emotion/react @emotion/styled
Install Material-UI Icons:
If you want to use Material-UI icons, you need to install the
@mui/icons-material
package:bashCopy codenpm install @mui/icons-material
or
bashCopy codeyarn add @mui/icons-material
Setting Up the Theme:
Material-UI uses a theme to define the appearance of your application. You can customize the theme to match your design requirements. Here's a basic setup:
jsxCopy codeimport React from 'react'; import ReactDOM from 'react-dom'; import { ThemeProvider, createTheme } from '@mui/material/styles'; import App from './App'; const theme = createTheme({ palette: { primary: { main: '#1976d2', }, secondary: { main: '#dc004e', }, }, }); ReactDOM.render( <ThemeProvider theme={theme}> <App /> </ThemeProvider>, document.getElementById('root') );
Core Components
Material-UI provides a wide range of components that you can use to build your UI. Here are some of the core components you’ll frequently use:
Buttons:
Buttons are essential in any web application. Material-UI offers different types of buttons such as text, contained, and outlined buttons.
jsxCopy codeimport Button from '@mui/material/Button'; function MyButton() { return ( <div> <Button variant="text">Text</Button> <Button variant="contained">Contained</Button> <Button variant="outlined">Outlined</Button> </div> ); }
App Bar:
The App Bar component is used to create a navigation bar for your application.
jsxCopy codeimport AppBar from '@mui/material/AppBar'; import Toolbar from '@mui/material/Toolbar'; import Typography from '@mui/material/Typography'; function MyAppBar() { return ( <AppBar position="static"> <Toolbar> <Typography variant="h6">My Application</Typography> </Toolbar> </AppBar> ); }
Cards:
Cards are used to display content in a structured manner. They are versatile and can include images, text, and actions.
jsxCopy codeimport Card from '@mui/material/Card'; import CardContent from '@mui/material/CardContent'; import Typography from '@mui/material/Typography'; function MyCard() { return ( <Card> <CardContent> <Typography variant="h5">Card Title</Typography> <Typography variant="body2">Card content goes here.</Typography> </CardContent> </Card> ); }
Grid:
The Grid component helps in creating responsive layouts. It uses a 12-column grid layout, similar to Bootstrap.
jsxCopy codeimport Grid from '@mui/material/Grid'; function MyGrid() { return ( <Grid container spacing={2}> <Grid item xs={12} sm={6} md={4}> <div>Grid Item 1</div> </Grid> <Grid item xs={12} sm={6} md={4}> <div>Grid Item 2</div> </Grid> <Grid item xs={12} sm={6} md={4}> <div>Grid Item 3</div> </Grid> </Grid> ); }
Customization
One of the best features of Material-UI is its flexibility. You can easily customize components to match your design needs.
Overriding Styles:
You can override default styles using the
sx
prop or thestyled
API.jsxCopy codeimport Button from '@mui/material/Button'; function CustomButton() { return ( <Button sx={{ backgroundColor: 'green', color: 'white' }}> Custom Button </Button> ); }
Theming:
Customizing the theme allows you to change the appearance of your entire application.
jsxCopy codeconst theme = createTheme({ palette: { primary: { main: '#ff5722', }, secondary: { main: '#795548', }, }, typography: { fontFamily: 'Arial', }, });
Conclusion
Material-UI is a powerful library that can significantly speed up your development process while ensuring your application looks professional and fulfil to best design practices. By leveraging its wide range of components and customization options, you can build responsive and visually appealing user interfaces with easily.
Give Material-UI a try in your next project and experience the benefits firsthand. Happy coding!
Subscribe to my newsletter
Read articles from Pooja Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
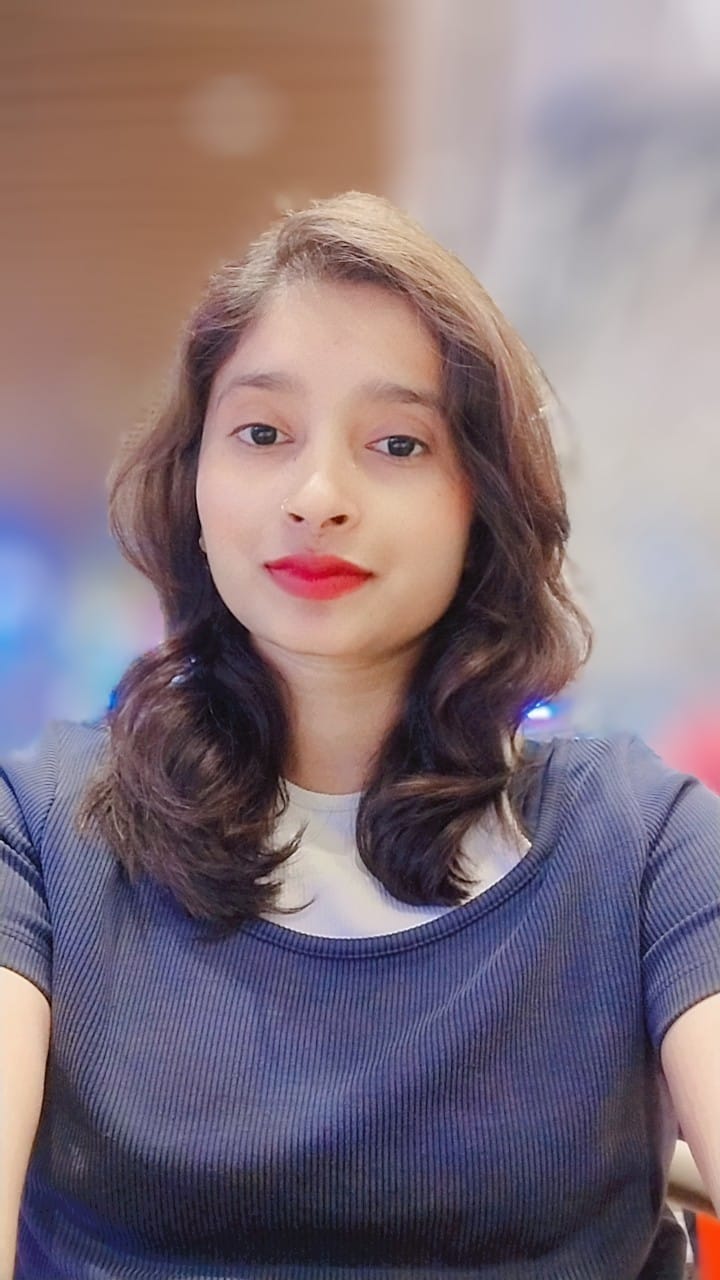
Pooja Verma
Pooja Verma
Full stack JavaScript developer