Unlocking the Potential of Comment Tags in Code

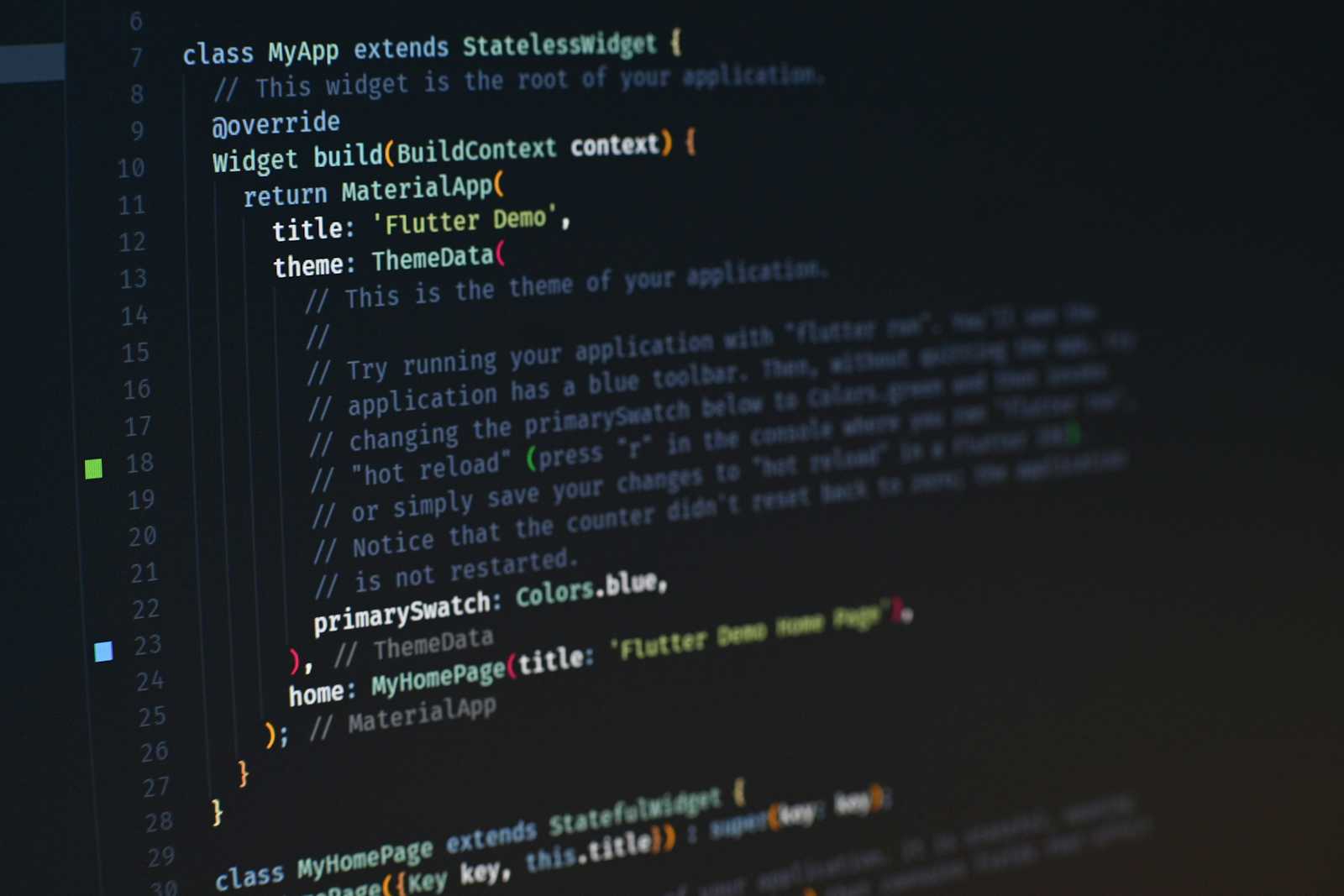
Introduction
As frontend developers, we often find ourselves knee-deep in code, trying to make sense of the complex code that powers modern web applications. Within this complexity, one of the most underrated yet powerful tools at our disposal is the simple comment tag. Commenting our code not only aids in readability and maintenance but also enhances collaboration and debugging. In this article, we will explore the significance of using comment tags in programming and delve into best practices to maximize their effectiveness.
What Are Comment Tags?
Comment tags are annotations within the source code that are ignored by the compiler or interpreter. They serve as notes or explanations for anyone reading the code, including the original developer, teammates, or even future maintainers. In the context of frontend development, comment tags can be used in HTML, CSS, and JavaScript to provide insights and clarify the purpose and functionality of the code.
Types of Comment Tags in Programming
A. Single-Line Comments
Single-line comments are typically used for brief explanations or notes. In JavaScript, they are denoted by //
, while in HTML, they are wrapped within <!-- -->
.
Example:
JavaScript:
// This function calculates the sum of two numbers
function add(a, b) {
return a + b;
}
HTML:
<!-- This is the main container -->
<div class="container">
<!-- Content goes here -->
</div>
B. Multi-Line Comments
Multi-line comments are useful for longer explanations or when commenting out blocks of code. In JavaScript, multi-line comments are enclosed within /* */
, while HTML comments remain within <!-- -->
.
Example:
JavaScript:
/*
* This function checks if a number is even or odd.
*/
function isEven(num) {
return num % 2 === 0;
}
HTML:
<!--
<div class="sidebar">
<p>Sidebar content</p>
</div>
-->
The Importance of Commenting Code
Commenting code is a crucial practice for several reasons:
Readability: Comments make the code more readable by providing context and explaining complex logic.
Maintainability: Well-commented code is easier to maintain and update, as the purpose and functionality are clearly documented.
Collaboration: Comments facilitate better collaboration among team members by providing clear communication about the code's intent.
Debugging: During debugging, comments help identify and understand the problem areas quickly.
Best Practices
A. Clarity and Conciseness
Comments should be clear and concise, providing just enough information to understand the code without overwhelming the reader.
B. Keeping Comments Up-to-Date
As the code evolves, it's essential to update the comments to reflect any changes. Outdated comments can be misleading and cause confusion.
C. Avoiding Over-Commenting
While comments are helpful, over-commenting can clutter the code and make it harder to read. Aim to comment only on complex or non-obvious parts of the code.
Commenting in Popular Frontend Frameworks
A. React
In React, comments can be used within JSX by wrapping them in curly braces and using the standard JavaScript comment syntax.
Example:
JSX:
return (
<div>
{/* This is a comment in JSX */}
<h1>Hello, world!</h1>
</div>
);
B. Vue.js
Vue.js templates use HTML comments, while script and style sections follow JavaScript and CSS commenting conventions.
Example:
<template>
<!-- This is a comment in a Vue template -->
<div>
<p>{{ message }}</p>
</div>
</template>
<script>
// This is a comment in a Vue script
export default {
data() {
return {
message: 'Hello, Vue!'
};
}
};
</script>
Conclusion
In the fast-paced world of frontend development, the power of using comment tags cannot be overstated. They are a vital tool for enhancing code readability, maintainability, and collaboration. By following best practices, developers can create more efficient and understandable source codes. Remember, comments are not just for others—they are a legacy we leave for our future selves, ensuring that we can always understand the complex structure we create.
Subscribe to my newsletter
Read articles from Oyindamola Abolaji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
