Build a To-Do List App with Vanilla JS✍️
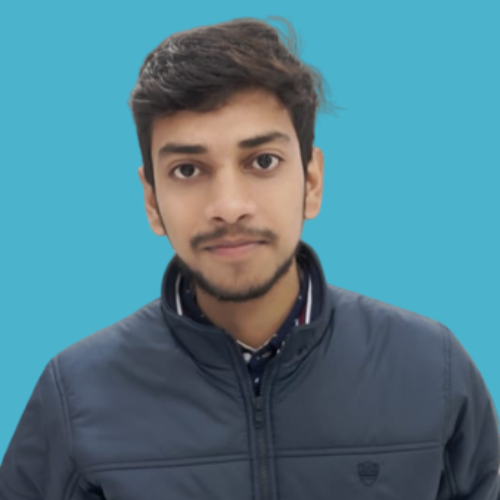
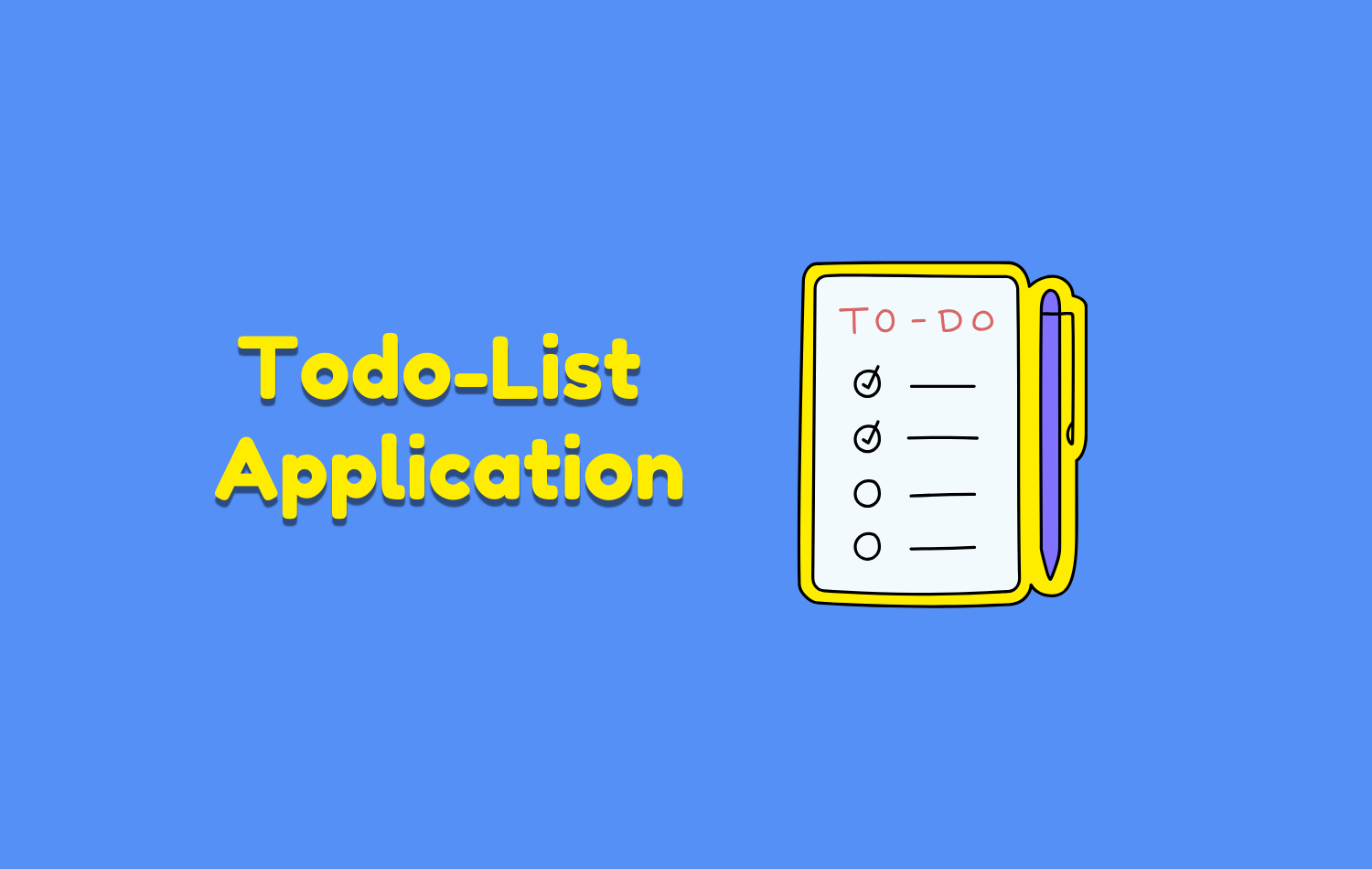
Introduction👋
In this blog, I will demonstrate illustrated steps on how to develop a to-do list application, as well as how I designed my to-do app before the coding phase, now let's get started.
Technology Used💻
For Frontend:html
, css
For Backend:javascript
For Deployment:Netlify
Figma Design🖍️
I have designed this to-do list web application on Figma as it is free software for designing.
Step 1: index.html file📙
Here is an HTML file that will be running on a browser as a frontend
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Homepage</title>
<link rel="shortcut icon" href="/Assests/favicon.png" type="image/x-icon" />
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Chivo&family=Kanit&display=swap"
rel="stylesheet"
/>
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Marhey:wght@500&display=swap"
rel="stylesheet"
/>
</head>
<body class="hp">
<nav id="navigation">
<div id="hamburger" onclick="onClickHamburger()">
<div id="bar1" class="bar"></div>
<div id="bar2" class="bar"></div>
<div id="bar3" class="bar"></div>
</div>
<ul class="navs" id="nav">
<h4>To-do It</h4>
<div class="icon-div">
<li>
<img src="Assests/github-sign.png" alt="" />
<a href="https://github.com/MOHDNEHALKHAN">My Github Profile</a>
</li>
<li>
<img src="Assests/linkedin.png" alt="" />
<a href="https://www.linkedin.com/in/mohd482/">LinkedIn Profile</a>
</li>
</div>
<p id="ham-para">Created With ❤️ By Mohd Nehal Khan</p>
</ul>
</nav>
<!-- Tasks will be added here -->
<div id="taskContainer"></div>
<!-- Add Task Modal -->
<form class="task-modal" id="form">
<div class="ts-add">
<label for="text" id="add-ts">Task</label>
<br />
<input type="text" name="" id="ts-text" />
<br />
<label for="text" id="add-ds">Description</label>
<br />
<textarea type="text" name="" id="ts-des"></textarea>
<div id="tp-btn">
<button type="button" id="close-btn" onclick="closeBtn()">
Close
</button>
<button type="submit" id="submit-btn">Save</button>
</div>
</div>
</form>
<!-- Homepage footer -->
<footer class="hp-btn">
<p class="CRtask">Create Task</p>
<a onclick="addTask()">
<img
src="Assests/taskadd-icon.png"
alt="task-add"
class="taskadd-icon"
/>
</a>
</footer>
<script src="script.js"></script>
</body>
</html>
Step 2: Style.css file📘
Here is a CSS file that will be running on a browser as a styling for our application
/* hamburger code */
#navigation {
width: auto;
height: 85px;
background: #faea58;
}
#hamburger {
width: 35px;
height: 30px;
padding: 30px 0px 0px 20px;
cursor: pointer;
z-index: 100;
}
.bar {
display: block;
width: 100%;
height: 5px;
border-radius: 10px;
background: #6cb9e5;
transition: 0.3s ease;
}
#bar1 {
transform: translateY(-4px);
}
#bar3 {
transform: translateY(4px);
}
.navs {
padding: 0;
margin: 0px 20px 0px 0px;
transition: 1s ease;
display: none;
}
.navs li a {
text-decoration: none;
padding: 8px 0px 0px 8px;
color: #636363;
}
.navs li a:active {
color: #636363;
}
.navs li {
list-style: none;
padding: 35px 0 0 32px;
display: flex;
flex-direction: row;
align-items: center;
}
#li-all {
padding-left: 37px;
}
.cross-icon .bar {
background-color: #6cb9e5;
}
.cross-icon #bar1 {
transform: translateY(4px) rotate(-45deg);
}
.cross-icon #bar2 {
opacity: 0;
}
.cross-icon #bar3 {
transform: translateY(-6px) rotate(45deg);
}
.change-ham {
background-color: #faea58;
height: 100%;
position: absolute;
margin: 0;
color: #636363;
font-family: "Marhey", sans-serif;
font-size: 24px;
font-style: normal;
font-weight: 400;
line-height: normal;
top: 0;
z-index: -100;
width: 100%;
display: flex;
flex-direction: column;
justify-content: center;
}
.CRtask {
color: #6cb9e5;
font-family: "Kanit", sans-serif;
font-size: 26px;
font-style: normal;
font-weight: 400;
line-height: normal;
text-align: center;
}
Step 3: Script.js📗
Here is a JavaScript file that will be running on the browser as a backend
// hamburger icon working
function onClickHamburger() {
document.getElementById("hamburger").classList.toggle("cross-icon");
document.getElementById("nav").classList.toggle("change-ham");
const hamBurger = document.getElementById("hamburger");
const navBar = document.getElementById("navigation");
const hpFooter = document.querySelector(".hp-btn");
if (hamBurger.classList.contains("cross-icon")) {
// Hamburger is now a cross, hide elements and set transparent background
if (hpFooter) hpFooter.style.display = "none";
if (navBar) navBar.style.background = "none";
} else {
// Hamburger is back, show elements and set yellow background
if (hpFooter) hpFooter.style.display = "flex";
if (navBar) navBar.style.background = "#faea58";
}
}
// Feature for Add-Task icon
function addTask() {
const TaskModal = document.querySelector(".task-modal");
const hpFooter = document.querySelector(".hp-btn");
if (TaskModal) TaskModal.style.display = "block";
if (hpFooter) hpFooter.style.display = "none";
}
//Close Modal
function closeBtn() {
let TaskModal = document.querySelector(".task-modal");
const hpFooter = document.querySelector(".hp-btn");
TaskModal.style.display = "none";
hpFooter.style.display = "flex";
}
// Function to create a new task
let form = document.getElementById("form");
let taskContainer = document.getElementById("taskContainer");
let taskHead = document.getElementById("ts-text");
let taskDes = document.getElementById("ts-des");
form.addEventListener("submit", function (e) {
e.preventDefault(); // refrain from refreshing a page
formValidation(); // calling the function
});
let formValidation = () => {
if (taskHead.value === "" || taskDes.value === "") {
alert("Please fill in all the fields");
} else {
console.log("Task Created");
acceptData();
}
};
let data = [];
let acceptData =() =>{
data.push({
taskHead: taskHead.value,
taskDes: taskDes.value,
});
localStorage.setItem("task", JSON.stringify(data));
console.log(data);
createTask();
};
let createTask = () =>{
taskDes.innerHTML = "";
data.map((x,y) => {
return taskContainer.innerHTML += `<div class="task">
<p class ="headTxt">${x.taskHead}</p>
<p class ="desTxt">${x.taskDes}</p>
<div class="Btn">
<button class="edit" onclick="editTask(this)" id = "editBtn">Edit</button>
<button class="delete" onclick="deleteTask(this)" id = "deleteBtn">Delete</button>
</div>
</div>`;
});
// Clear input fields
resetForm();
closeBtn();
};
let deleteTask = (e) =>{
e.parentElement.parentElement.remove();
data.splice(e,1);
localStorage.setItem("task", JSON.stringify(data));
};
let editTask = (e) => {
let selectedTask = e.parentNode.parentNode;
taskHead.value = selectedTask.children[0].innerText;
taskDes.value = selectedTask.children[1].innerText;
// Show the task modal for editing
const TaskModal = document.querySelector(".task-modal");
const hpFooter = document.querySelector(".hp-btn");
if (TaskModal) TaskModal.style.display = "block";
if (hpFooter) hpFooter.style.display = "none";
};
let resetForm = () =>{
taskHead.value = "";
taskDes.value = "";
};
(() => {
data = JSON.parse(localStorage.getItem("data")) || [];
console.log(data);
createTask();
})();
GitHub Repo👇🏼 Link for full code
Conclusion
Follow Me On Socials :
Like👍| Share📲| Comment💭
Subscribe to my newsletter
Read articles from MOHD NEHAL KHAN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
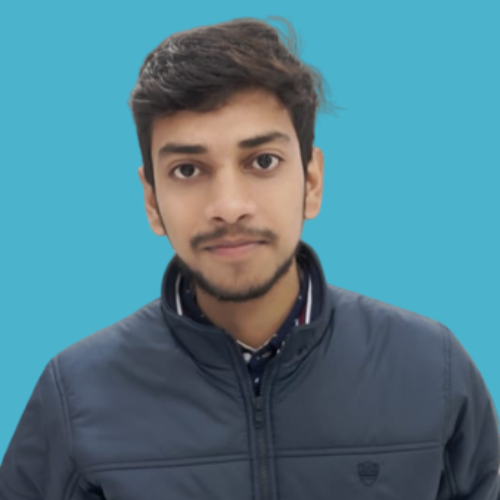
MOHD NEHAL KHAN
MOHD NEHAL KHAN
I am a student at Manipal University Jaipur and a web developer, learning and exploring tech stack in web development and implementing in my projects