How to Use Conditionals
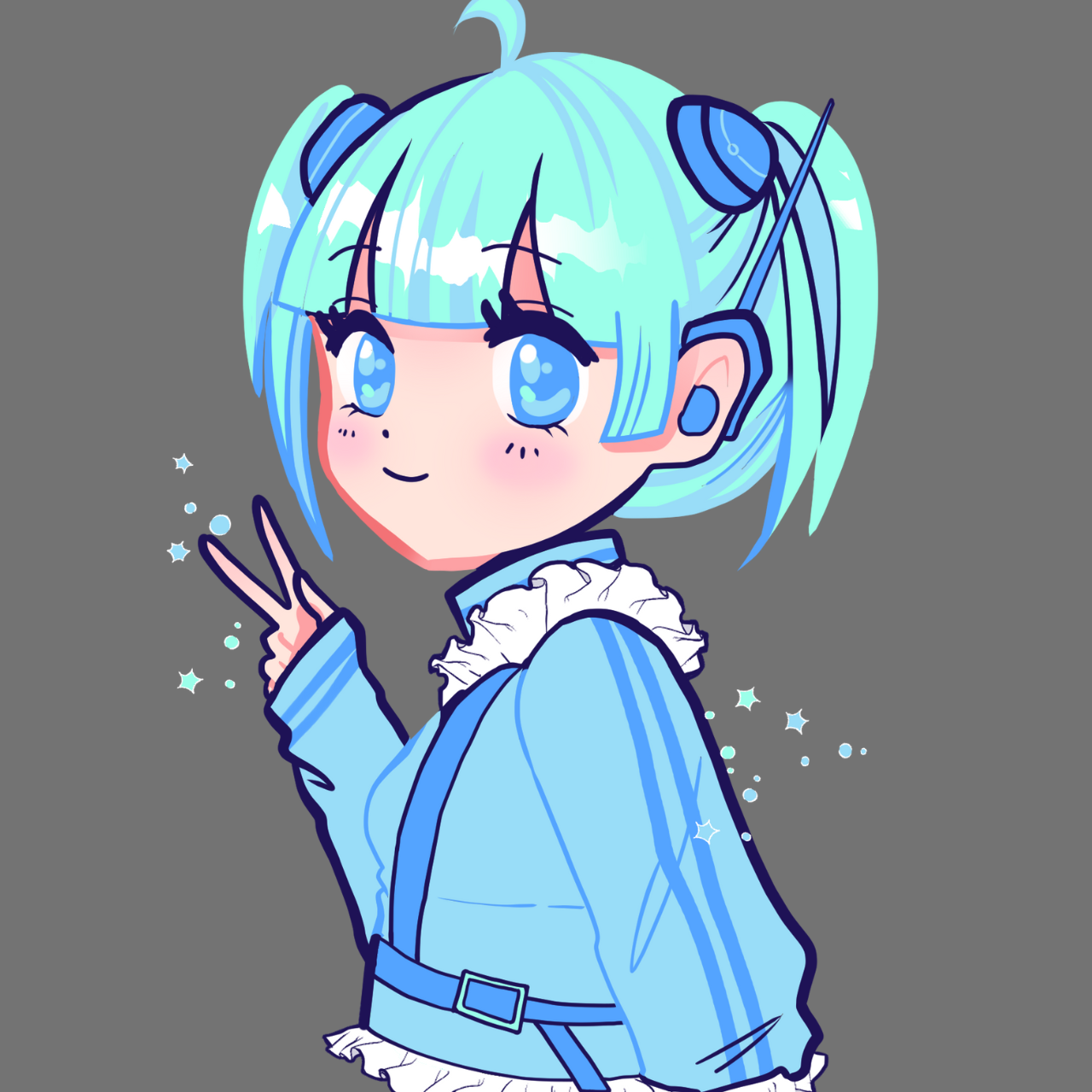
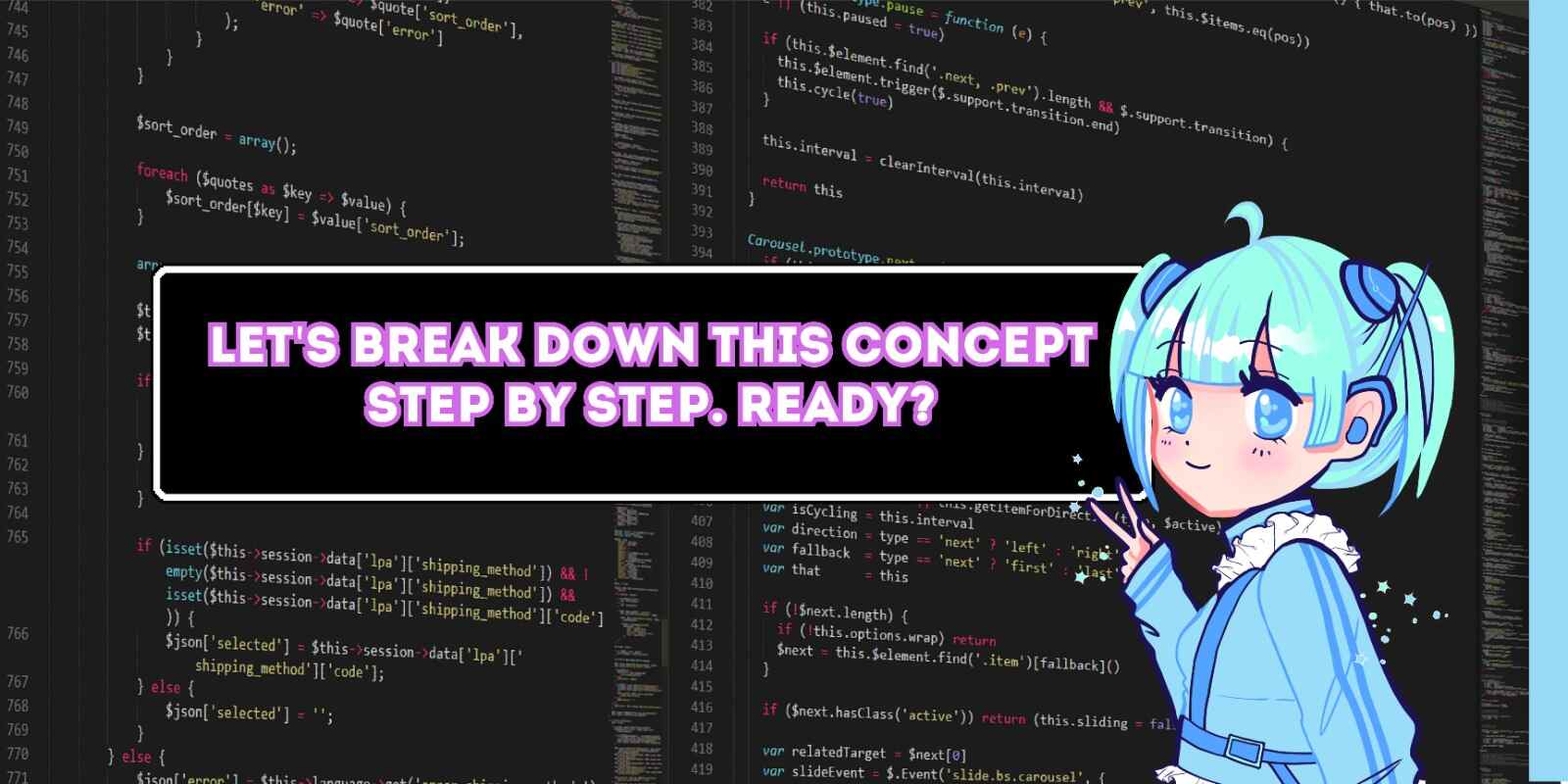
conditions are crucial concepts in programming as they help us save lines of code and manage memory efficiently.
CONDITIONALS
As the name suggests, conditionals are used to set rules and make decisions based on circumstances. For example, when driving a car, you follow traffic lights to determine your actions:
If the traffic light is red, then you stop.
Else if the traffic light is yellow, then you prepare to stop.
Else if the traffic light is green, then you proceed.
Similarly, computers use conditionals to make decisions. In games, NPCs (non-player characters) or enemies react based on the player's position. For instance, if the player is within range, the enemy attacks. This decision-making process relies on conditions to determine actions based on specific criteria.
There are four main types of conditionals:
if statement: Used to execute a block of code if a particular condition is true.
For example, "if I'm hungry, then eat."
if (hungry){ eat(); }
If the condition is not satisfied, nothing will happen and no code will be executed.
if-else statement: Executes one block of code if the condition is true and another block if it's false.
For instance, "if I'm hungry, eat; else, keep working."
if (hungry) { eat(); } else { keepWorking(); }
Here, even if the condition is not satisfied, it will still execute the else statement.
if-else if statement: Used for checking multiple conditions sequentially. Each
else if
condition is checked only if the previous conditions were false.
if (hungry && haveFood) {
System.out.println("Eat food that you have.");
} else if (hungry && haveMoney) {
System.out.println("Buy food to eat.");
} else if (hungry) {
System.out.println("Look for food options.");
} else {
System.out.println("Keep working.");
}
Suppose condition 2 is true, then the subsequent conditions will not be evaluated. This means the program will short-circuit and bypass the remaining conditions, optimizing performance and avoiding unnecessary operations.
switch statement: This is a multiple-choice decision-making statement used to select one case out of multiple cases. It checks from top to bottom and executes the corresponding block of code when a case is true. Once a case is true, it skips the rest of the ladder.
switch (condition) { case 1: System.out.println("Eat food that you have."); break; case 2: System.out.println("Buy food to eat."); break; case 3: System.out.println("Look for food options."); break; case 4: System.out.println("Keep working."); break; default: System.out.println("Unexpected condition."); break;
In switch statement, default option is used when none of the conditions apply. This ensures there's always a plan to follow, no matter what happens.
Break tells the program to stop after it runs the code for a particular option (
case
). It keeps each option separate and prevents the program from running into the next one.
CONCLUSION
In summary, conditionals are essential tools in programming for making decisions based on different situations. Whether it's controlling traffic in a program or adjusting game actions based on player choices, conditionals help programs react intelligently. From basic if statements to detailed switch cases, each type of conditional offers a way to handle different scenarios efficiently.
Subscribe to my newsletter
Read articles from CODE SENSEI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
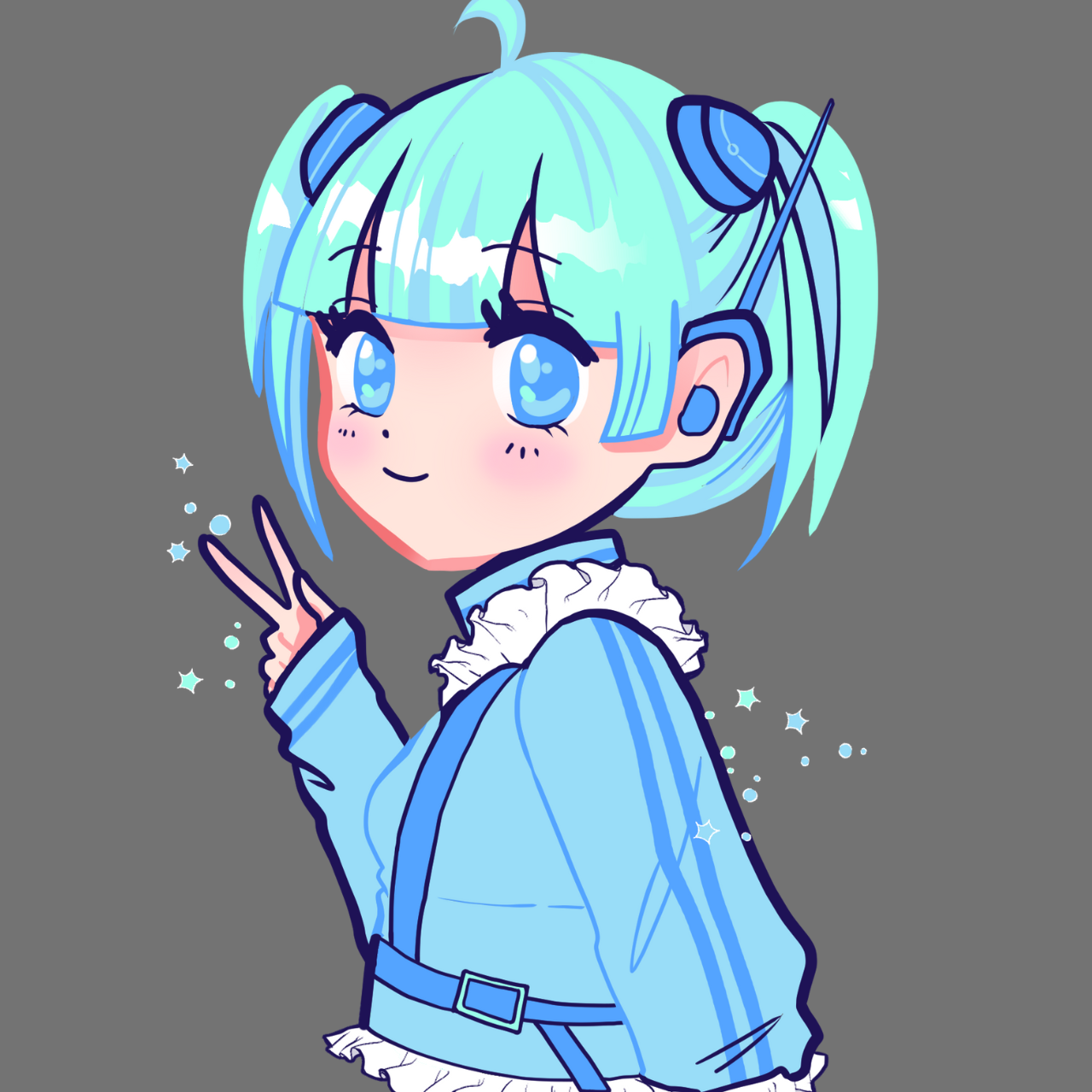
CODE SENSEI
CODE SENSEI
Hi there! I'm Ayush, currently diving into Data Structures and Algorithms (DSA) and learning Java. Join me as I navigate through these subjects, sharing what I learn along the way. Let's explore together!