Understanding Zustand: A Lightweight State Management Library for React

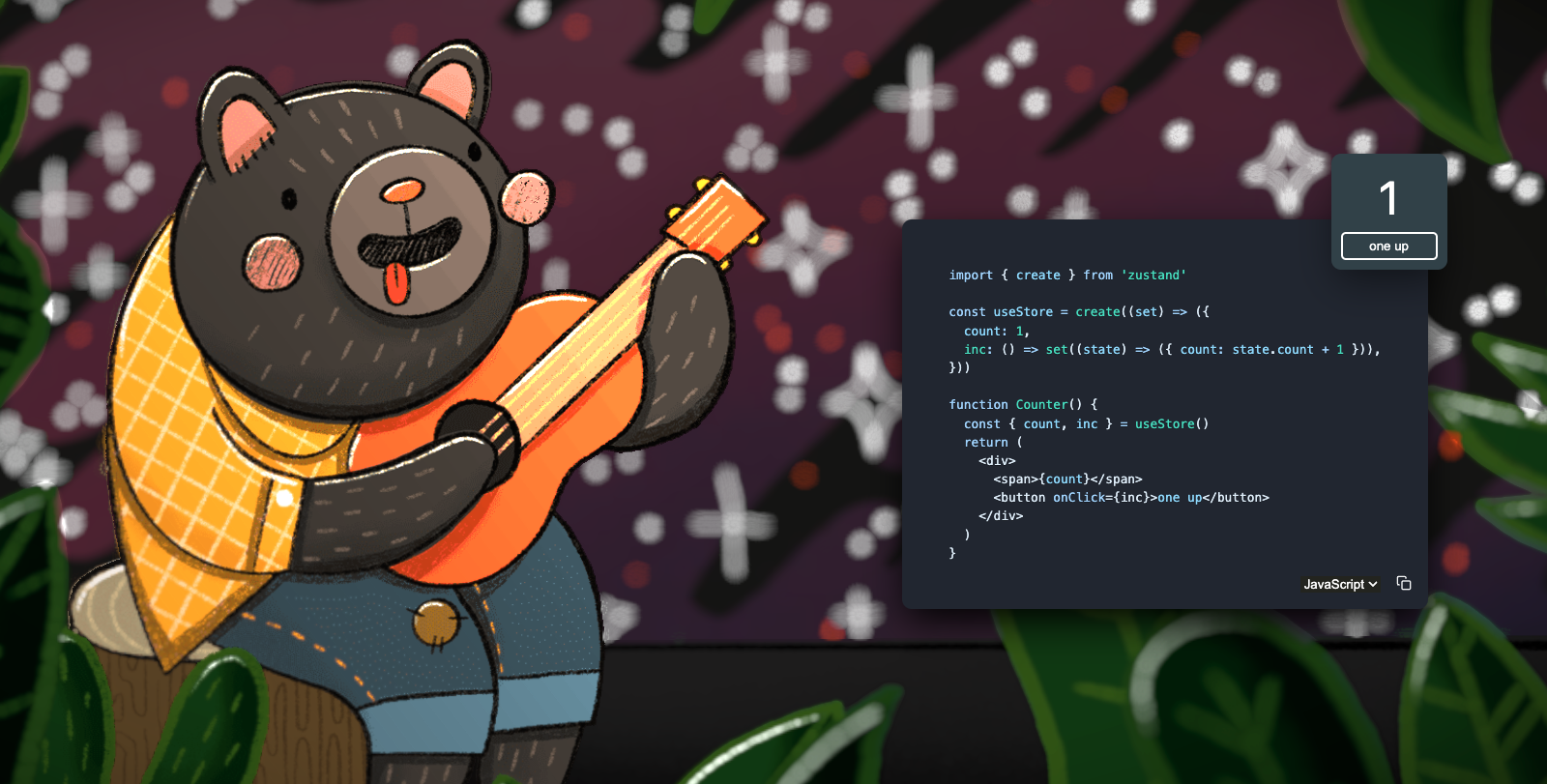
State management is a crucial aspect of building robust and scalable React applications. While there are numerous state management libraries available, Zustand has emerged as a lightweight and flexible alternative that provides a simple yet powerful way to manage state in React applications. This article delves into what Zustand is, its use cases, and some examples of its promising APIs, all in TypeScript.
What is Zustand?
Zustand is a small, fast, and scalable state management library for React. Unlike more complex libraries such as Redux, Zustand is designed to be minimalistic, with an API that's easy to use and integrate into any React application. It uses hooks to manage state, making it a perfect fit for modern React applications that leverage the power of hooks for state and side-effect management.
Key Features of Zustand
Minimalistic and Lightweight: Zustand has a small bundle size and minimal API surface, making it easy to learn and use.
No Boilerplate: It eliminates the need for boilerplate code, allowing developers to focus on application logic rather than wiring up actions and reducers.
Performance: Zustand is optimized for performance, ensuring that state updates are efficient and don't cause unnecessary re-renders.
Flexible and Scalable: It can be used for both simple and complex state management needs, making it suitable for a wide range of applications.
Use Cases for Zustand
Zustand can be used in various scenarios where state management is required. Here are a few common use cases:
Global State Management: Managing global state across the entire application, such as user authentication, theme settings, and application configuration.
Component State Management: Handling state that is shared between multiple components, such as form inputs, filters, and UI state.
Asynchronous State Management: Managing state that depends on asynchronous operations, such as fetching data from an API and handling loading and error states.
Getting Started with Zustand
To get started with Zustand, you need to install it in your React project:
npm install zustand
# or
yarn add zustand
Basic Example
Let's start with a basic example of using Zustand to manage the state of a counter:
import { create } from 'zustand';
interface State {
count: number;
increment: () => void;
decrement: () => void;
}
const useStore = create<State>((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
const Counter: React.FC = () => {
const { count, increment, decrement } = useStore();
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we define a store using the create
function from Zustand. The store has a state object with a count
property and two actions: increment
and decrement
. These actions update the state using the set
function. The Counter
component uses the useStore
hook to access the state and actions, allowing it to display and modify the counter value.
Advanced Example: Asynchronous State Management
Zustand can also handle asynchronous operations seamlessly. Here's an example of fetching data from an API:
import { create } from 'zustand';
interface State {
data: any;
loading: boolean;
error: string | null;
fetchData: () => Promise<void>;
}
const useStore = create<State>((set) => ({
data: null,
loading: false,
error: null,
fetchData: async () => {
set({ loading: true, error: null });
try {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
set({ data: result, loading: false });
} catch (error) {
set({ error: error.message, loading: false });
}
},
}));
const DataFetcher: React.FC = () => {
const { data, loading, error, fetchData } = useStore();
return (
<div>
<button onClick={fetchData}>Fetch Data</button>
{loading && <p>Loading...</p>}
{error && <p>Error: {error}</p>}
{data && <pre>{JSON.stringify(data, null, 2)}</pre>}
</div>
);
};
export default DataFetcher;
In this example, the store has additional state properties (data
, loading
, and error
) and an asynchronous action (fetchData
) that fetches data from an API. The DataFetcher
component uses the useStore
hook to access the state and action, allowing it to initiate the fetch operation and display the results.
Promising APIs in Zustand
Zustand offers several promising APIs that enhance its functionality:
- Selectors: Zustand supports selectors for efficient state selection, allowing components to subscribe to specific parts of the state.
const counter = () => useStore((state) => state.count);
const setCounter = () => useStore((state) => state.setCount);
- Middleware: Zustand allows middleware to be added to stores for advanced use cases such as logging, persisting state, and handling side effects.
import { create } from 'zustand';
import { persist } from 'zustand/middleware';
const useStore = create<State>(
persist(
(set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}),
{ name: 'counter' }
)
);
- Computed State: Zustand supports derived state, enabling the creation of computed properties based on the store's state.
const doubleCount = () => useStore((state) => state.count * 2);
- Devtools Integration: Zustand integrates with Redux DevTools for better debugging and state inspection.
import { devtools } from 'zustand/middleware';
const useStore = create<State>(
devtools((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}))
);
Real life example with slice pattern
Using the slice pattern with Zustand allows you to modularize and organize your state management logic into smaller, reusable pieces. This pattern is particularly useful for larger applications where you want to maintain a clear separation of concerns. Below, we'll enhance the contact form example by using the slice pattern.
Setting Up the Zustand Store with Slices
First, create slices for the contact form state. Create a file named contactStore.ts
:
import { create, type StateCreator } from 'zustand';
interface ContactFormSlice {
name: string;
email: string;
subject: string;
message: string;
setName: (name: string) => void;
setEmail: (email: string) => void;
setSubject: (subject: string) => void;
setMessage: (message: string) => void;
resetForm: () => void;
}
const createContactFormSlice: StateCreator<ContactFormSlice> = (set) => ({
name: '',
email: '',
subject: '',
message: '',
setName: (name) => set({ name }),
setEmail: (email) => set({ email }),
setSubject: (subject) => set({ subject }),
setMessage: (message) => set({ message }),
resetForm: () => set({
name: '',
email: '',
subject: '',
message: '',
}),
});
interface Store extends ContactFormSlice {}
export const useStore = create<Store>((set) => ({
...createContactFormSlice(set),
}));
Creating the Contact Form Component
Now, create a page or component for the contact form. Here’s an example using a functional component:
import { useStore } from '../store/contactStore';
import { FormEvent } from 'react';
const ContactForm = () => {
const { name, email, subject, message, setName, setEmail, setSubject, setMessage, resetForm } = useStore();
const handleSubmit = (e: FormEvent) => {
e.preventDefault();
// Here, you can handle the form submission, e.g., send the data to an API
console.log({ name, email, subject, message });
resetForm();
};
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="name">Name:</label>
<input
type="text"
id="name"
value={name}
onChange={(e) => setName(e.target.value)}
required
/>
</div>
<div>
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
</div>
<div>
<label htmlFor="subject">Subject:</label>
<input
type="text"
id="subject"
value={subject}
onChange={(e) => setSubject(e.target.value)}
required
/>
</div>
<div>
<label htmlFor="message">Message:</label>
<textarea
id="message"
value={message}
onChange={(e) => setMessage(e.target.value)}
required
/>
</div>
<button type="submit">Submit</button>
</form>
);
};
export default ContactForm;
Using the Contact form
You can now integrate the ContactForm
component into any page in your application. For example, if you want to display it on the home page, you can modify pages/home.tsx
:
import ContactForm from '../components/ContactForm';
const Home = () => {
return (
<div>
<h1>Contact Us</h1>
<ContactForm />
</div>
);
};
export default Home;
Using the slice pattern with Zustand, we have modularized the state management for a contact form in a React application. This approach helps in maintaining a clean and organized codebase, especially as the application grows. By defining slices and combining them into a single store, you can easily manage different parts of your application state in a scalable manner.
Conclusion
Zustand is a powerful and flexible state management library that offers a minimalistic API, making it an excellent choice for both simple and complex React applications. Its lightweight nature, combined with features like selectors, middleware, computed state, and devtools integration, makes it a compelling alternative to more heavyweight state management solutions. Whether you're building a small project or a large-scale application, Zustand provides the tools you need to manage state efficiently and effectively.
For more information visit there documentation from here. And still if you need help then let discuss and explore.
Subscribe to my newsletter
Read articles from Saiful Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saiful Alam
Saiful Alam
An Expert software engineer in Laravel and React. Creates robust backends and seamless user interfaces. Committed to clean code and efficient project delivery, In-demand for delivering excellent user experiences.